Debugging Common Issues in Java 8 Lambda Expressions
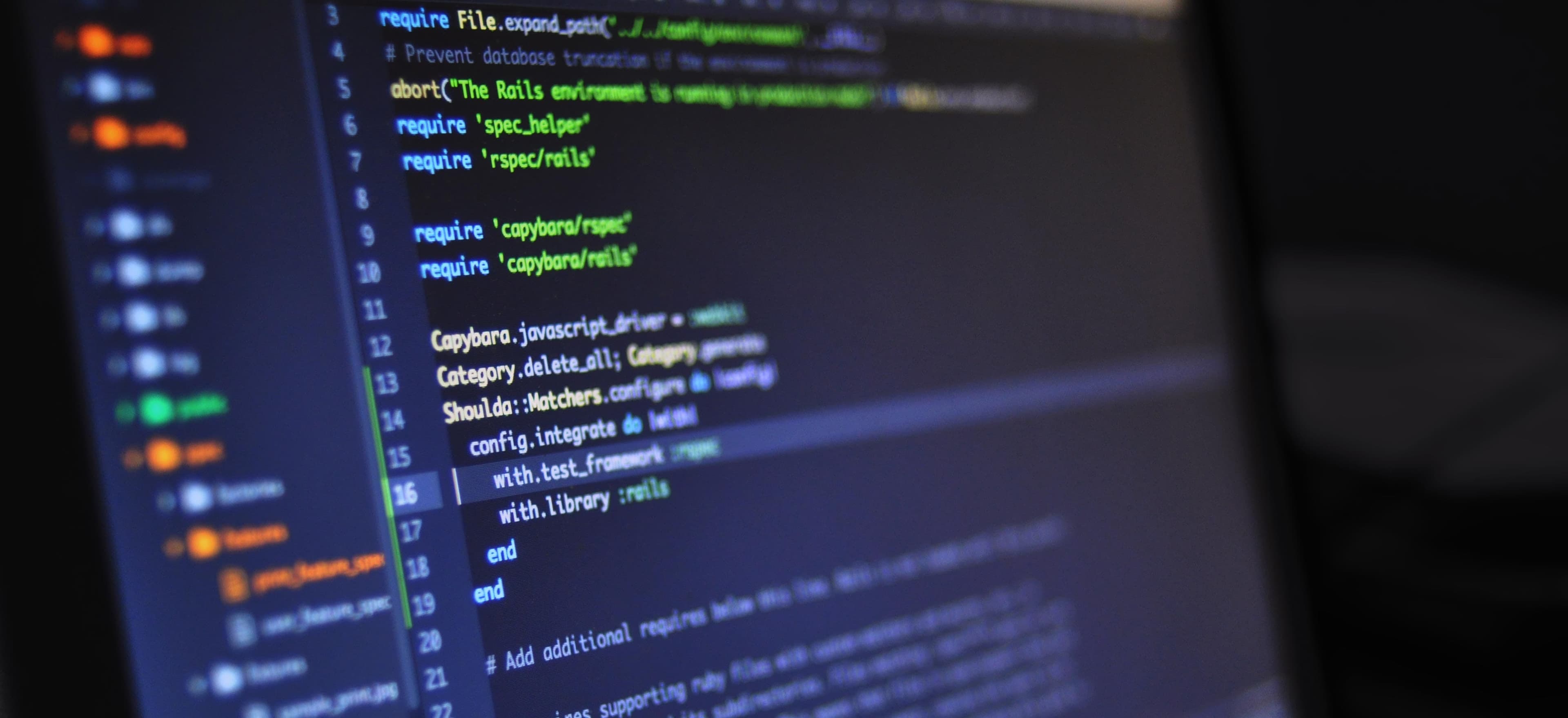
- Published on
Debugging Common Issues in Java 8 Lambda Expressions
Java 8 introduced lambda expressions, a significant enhancement that allows developers to implement functional programming concepts in Java. However, as developers adapt to this new feature, they may encounter some common issues and debugging challenges. This blog post serves as a guide to identify, understand, and rectify these issues effectively. Let’s dive into the fascinating world of lambda expressions and unlock the potential they offer!
Understanding Lambda Expressions
Before we delve into debugging, let us briefly understand lambdas. Lambda expressions are syntactic sugar for representing anonymous functions. They provide a clear and concise way to express behaviors — primarily used in functional programming interfaces.
Syntax of Lambda Expressions
The basic syntax of a lambda expression in Java is:
(parameters) -> expression
or
(parameters) -> { statements; }
Basic Example
Here’s a simple example to illustrate how a lambda expression works with the Runnable
interface:
Runnable runTask = () -> System.out.println("Running in lambda");
runTask.run(); // Output: Running in lambda
In this example, instead of implementing the Runnable
interface the traditional way (by creating a class that implements Runnable
), we use a lambda expression, making the code more concise and readable.
Common Issues and Debugging Techniques
While lambda expressions can greatly simplify your code, issues can arise. Below, we discuss a few common problems and the best strategies for debugging them.
1. Type Inference Problems
Issue: Java uses type inference to determine the types of parameters in lambda expressions. However, sometimes, it fails to infer the correct types, leading to compilation errors.
Example:
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
names.forEach(name -> System.out.println(name.length())); // Works fine
However, if you incorrectly provide a parameter type:
names.forEach((Integer name) -> System.out.println(name.length())); // Compilation Error
Solution: Ensure that the parameter types are compatible. If necessary, explicitly annotate the types within the lambda:
names.forEach((String name) -> System.out.println(name.length()));
2. Accessing Non-Final Variables
Issue: In lambda expressions, you can only access "effectively final" variables from the enclosing scope. A variable is effectively final if it is not modified after its initialization.
Example:
String suffix = "!";
Runnable greet = () -> System.out.println("Hello" + suffix);
suffix = "?"; // Compilation Error: Variable suffix is already defined as final
Solution: To work around this limitation, make sure the variable doesn't change after its creation, or use fields from a containing class.
String suffix = "!";
Runnable greet = () -> System.out.println("Hello" + suffix);
// No changes to suffix after initialization
3. Use of this
Keyword
Issue: Inside a lambda expression, this
refers to the enclosing class, not the lambda itself. This can create confusion when trying to access instance variables.
Example:
class Foo {
int x = 10;
void doSomething() {
Runnable r = () -> System.out.println(this.x);
r.run(); // Output: 10
}
}
But, if you want to capture a variable in lambda:
class Bar {
int x = 10;
void doSomething() {
Runnable r = () -> {
int x = 20; // New variable
System.out.println(this.x); // Refers to Bar's x
System.out.println(x); // Refers to lambda's x
};
r.run(); // Output: 10, 20
}
}
Solution: Be aware of the scope of this
. For instance, to refer to the lambda expression itself, use a separate variable.
4. Error Propagation
Issue: When exceptions are thrown inside a lambda expression, they can be tricky to handle. Rather than being caught at the lambda level, they propagate back to the caller, possibly causing runtime failures.
Example:
List<Integer> numbers = Arrays.asList(1, 2, 3);
numbers.forEach(num -> {
if (num == 2) throw new RuntimeException("Exception for 2");
});
Solution: Wrap the lambda in a try-catch
block to manage exceptions gracefully.
numbers.forEach(num -> {
try {
if (num == 2) throw new RuntimeException("Exception for 2");
} catch (RuntimeException e) {
System.out.println(e.getMessage());
}
});
5. Using Streams Incorrectly
Streams are a powerful feature that complements lambda expressions but can lead to convolutions if not used correctly.
Example of an Incorrect Stream Usage:
List<String> names = Arrays.asList("Anna", "Bob", "Charlie");
names.stream()
.filter(name -> name.length() > 3)
.forEach(System.out::println); // Works Fine
// Reasonable Usage
List<String> filteredNames = names.stream()
.filter(name -> name.length() > 3)
.collect(Collectors.toList());
Solution: Understand the lifecycle of streams. They are not reusable, so don’t try to use the same stream more than once.
In Conclusion, Here is What Matters
Java 8's lambda expressions have revolutionized the way we approach functional programming in Java. However, like any powerful tool, they come with their set of challenges that require understanding and careful implementation. By being aware of the common issues discussed in this guide, you will not only debug effectively but also leverage lambda expressions to their full potential.
For more resources and in-depth discussions on functional programming in Java, consider exploring the following links:
- Java 8 Official Documentation
- Effective Java (3rd Edition) by Joshua Bloch
- Java Lambdas: Learn to Use Java Lambdas Features
Now that you're equipped with knowledge and debugging strategies, happy coding with Java 8 lambdas!