Overcoming Common API Rate Limiting Challenges
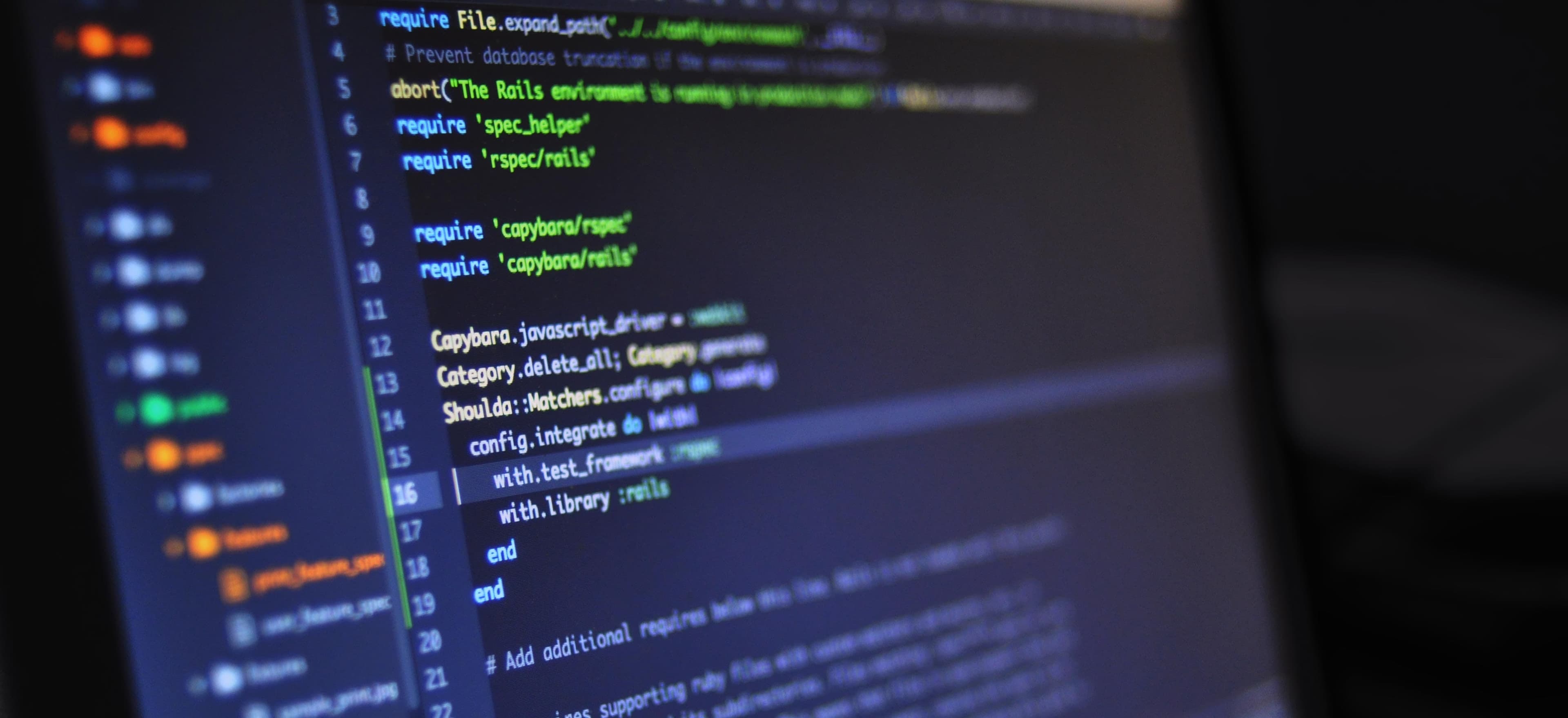
- Published on
Overcoming Common API Rate Limiting Challenges
In today's interconnected world, APIs (Application Programming Interfaces) play a crucial role in enabling various services to communicate with one another. However, with this powerful capability comes the challenge of API rate limiting. Rate limiting is a mechanism that restricts the number of requests a user can make to a particular API within a specified time frame. This post will delve into the intricacies of API rate limiting and explore practical solutions for overcoming common challenges.
Understanding API Rate Limiting
APIs often impose limits on the frequency of requests for several reasons:
- Resource Management: To prevent abuse and manage server workload.
- Performance Stability: To ensure all users receive a stable level of service.
- Security: To mitigate the risk of denial-of-service attacks.
While rate limiting is essential, it can pose significant challenges for developers. Let's explore the common issues and how to tackle them effectively.
Common Challenges with Rate Limiting
1. Unexpected Request Limits
When working with third-party APIs, developers may encounter surprising rate limits. Each service has distinct rules and limits, which can change anytime. For example, Twitter’s API operates on a rate limit model that allows a specific number of requests per 15 minutes. Failing to account for these limits can result in unexpected errors and downtime.
Solution: Always refer to the API documentation before implementation. Make use of tools like Postman or cURL to experiment with the API and understand its limits better. Here’s a quick code snippet leveraging the Twitter API in Java:
public class TwitterApiExample {
public static void main(String[] args) {
try {
// Assuming necessary libraries and authentication setup is done
Twitter twitter = TwitterFactory.getSingleton();
List<Status> statuses = twitter.getUserTimeline("your_username");
System.out.println("Got " + statuses.size() + " tweets.");
} catch (TwitterException e) {
// Catching the rate limit exception
if (e.getErrorCode() == 88) {
System.out.println("Rate limit exceeded. Please try again later.");
}
}
}
}
This code snippet demonstrates how to handle a rate limit exception by checking the error code.
2. Error Handling and Backoff Strategies
When your application exceeds the rate limit, APIs will typically respond with an error code (like HTTP 429). Appropriate error handling becomes necessary here. A common strategy in this context is to implement an exponential backoff algorithm.
Solution: Use an exponential backoff approach to retry failed requests. This strategy involves waiting longer periods between retries after each subsequent failure. Here’s an example:
public void fetchDataWithBackoff() {
int retryCount = 0;
while (retryCount < MAX_RETRIES) {
try {
// Place your API call here
makeApiCall();
break; // Exit if successful
} catch (ApiRateLimitException e) {
retryCount++;
long waitTime = (long) Math.pow(2, retryCount) * 1000; // Exponential backoff
System.out.println("Rate limit reached, waiting for " + waitTime + " ms.");
try {
Thread.sleep(waitTime);
} catch (InterruptedException ie) {
Thread.currentThread().interrupt();
}
}
}
}
In this snippet, we use an exponential backoff strategy to handle ApiRateLimitException
. The retry will wait longer after each failure, minimizing the chances of hitting the limit again.
3. Throttling Client-Side
Throttling requests on the client side is crucial to stay within the limit and ensure an efficient user experience. Implement a queueing mechanism to manage requests effectively, especially if multiple requests are initiated simultaneously.
Solution: Use a request queue with a delay between requests. Here’s an illustrative example:
import java.util.concurrent.*;
public class ApiThrottler {
private final ScheduledExecutorService scheduler = Executors.newScheduledThreadPool(1);
public void scheduleApiCalls(Runnable apiCall, int delayInSeconds) {
scheduler.schedule(apiCall, delayInSeconds, TimeUnit.SECONDS);
}
public static void main(String[] args) {
ApiThrottler throttler = new ApiThrottler();
Runnable apiCall = () -> {
// Your API call logic here
System.out.println("Making API call at " + System.currentTimeMillis());
};
for (int i = 0; i < 10; i++) {
throttler.scheduleApiCalls(apiCall, i * 1); // 1 second apart
}
}
}
In this example, we utilize ScheduledExecutorService
to delay API calls. This ensures you stay under your allowed request limit by controlling how quickly requests are made.
4. Monitoring and Logging
Constant monitoring and logging of your API requests is vital. It allows you to analyze usage patterns, anticipate potential rate limiting, and strategize accordingly.
Solution: Implement logging that captures the response codes and any important metrics. Here’s how you might do this:
import java.util.logging.*;
public class ApiLogger {
private static final Logger logger = Logger.getLogger(ApiLogger.class.getName());
public void logApiCall(String endpoint, int responseCode) {
logger.log(Level.INFO, "API Call to {0} returned response code: {1}", new Object[]{endpoint, responseCode});
}
}
Using a logging framework helps keep track of API interactions, making debugging easier and providing useful feedback on rate-limiting issues.
5. Leveraging Caching Mechanisms
Reduce the number of API requests by implementing caching logic. When you frequently access the same data, it can be more efficient to cache the result rather than fetching it again immediately.
Solution: Store responses locally for a short period. For instance, if you get user data from an API that doesn't change often, you can cache that data for a few minutes. Here is a simple caching mechanism:
import java.util.HashMap;
import java.util.Map;
public class Cache {
private Map<String, String> cache = new HashMap<>();
private long cacheExpirationTime = 300000; // 5 minutes in milliseconds
private long lastFetchTime;
public String getData(String key) {
if (cache.containsKey(key) && (System.currentTimeMillis() - lastFetchTime < cacheExpirationTime)) {
return cache.get(key);
}
String data = fetchDataFromApi(key);
cache.put(key, data);
lastFetchTime = System.currentTimeMillis();
return data;
}
private String fetchDataFromApi(String key) {
// Implement API call here
return "Fetched Data"; // Mocked response
}
}
The Last Word
API rate limiting can indeed be challenging, but with the right strategies and tools, developers can navigate these hurdles efficiently. From acknowledging varying request limits and implementing robust error handling techniques to employing caching mechanisms and request throttling, there are numerous ways to design your applications to withstand these constraints.
For more information and resources on understanding API usage and limitations, consider checking out the Twitter API documentation or explore the GitHub API guidelines.
Embrace these best practices, and you will find a smoother journey in API interactions! Happy coding!