Troubleshooting Common Issues in Java Monitoring Stacks
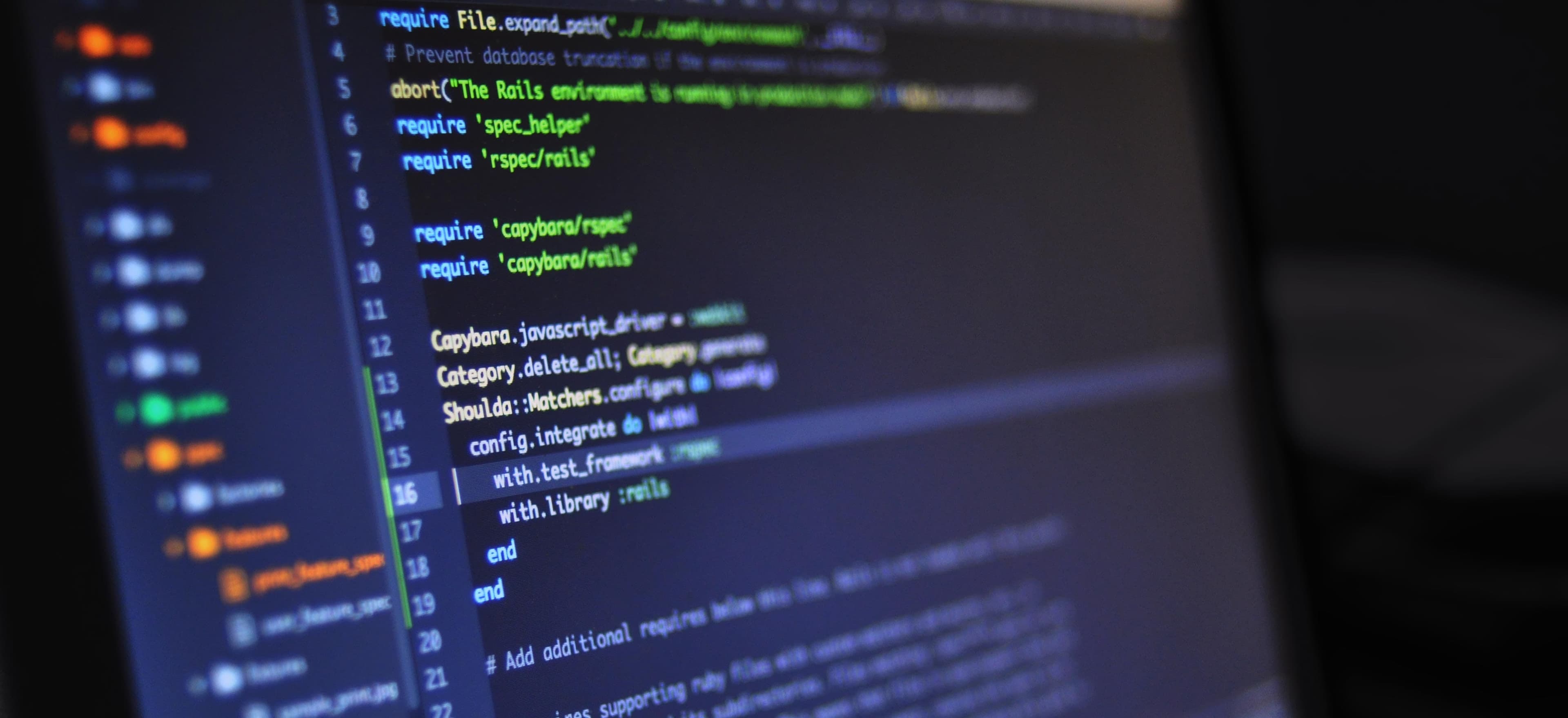
- Published on
Troubleshooting Common Issues in Java Monitoring Stacks
Java monitoring stacks are vital for identifying and diagnosing performance issues in Java applications. With the increasing complexity of distributed systems and microservices architecture, having a deep understanding of how to troubleshoot these stacks can significantly enhance the performance and reliability of your applications.
In this blog post, we will explore common issues associated with Java monitoring stacks, how to troubleshoot them, and effective strategies for resolving these issues.
Understanding Java Monitoring
Before diving into troubleshooting, let's first understand what Java monitoring entails. Monitoring involves the collection, analysis, and interpretation of performance data from your Java applications. This data often includes:
- Garbage Collector (GC) Activity: Information on memory allocation and deallocation.
- Thread Usage: Insights into thread pools, blockages, and contention.
- Application Performance Metrics: Response times, request rates, and error rates.
There are several popular tools available for monitoring Java applications, including:
- Java Management Extensions (JMX)
- VisualVM
- Prometheus
- AppDynamics
- New Relic
Each of these tools provides various metrics that you can analyze to troubleshoot potential issues.
Common Issues in Java Monitoring Stacks
- High Garbage Collection (GC) Pauses
- Thread Contention and High CPU Utilization
- Memory Leaks
- Poor Application Performance
- Network Latency and Failures
Let’s break down each of these issues, explore their causes, and present solutions.
1. High Garbage Collection (GC) Pauses
Symptoms: The application becomes unresponsive or sluggish.
Causes:
- Insufficient heap size.
- Inefficient object creation leading to excessive garbage.
Troubleshooting Steps:
- Analyze GC Logs: Use
-XX:+PrintGCDetails
,-XX:+PrintGCTimeStamps
, and-Xloggc:<file>
JVM flags to log garbage collection events.
java -Xmx2g -XX:+PrintGCDetails -XX:+PrintGCTimeStamps -Xloggc:gc.log MyApplication
- Evaluate GC Type: Consider changing the garbage collector. For instance, use G1 for large heaps, or ZGC for ultra-low latency.
Example of Switching GC:
java -XX:+UseG1Gc -jar MyApplication.jar
Why: G1 GC is designed for cost-effective garbage collection, especially suitable for applications that require predictable response times.
2. Thread Contention and High CPU Utilization
Symptoms: Your application exhibits high CPU usage, yet is performing slow.
Causes:
- Excessive locking in code.
- Too many threads competing for resources.
Troubleshooting Steps:
- Use Thread Dumps: Obtain a thread dump using JVisualVM or
jstack
to analyze thread states and identify contention points.
jstack <pid> > threaddump.txt
- Identify Synchronized Blocks: Look for threads that are stuck in synchronized blocks and optimize these areas to reduce contention.
Example of Reducing Locking:
// Possible contention
public synchronized void update() {
// perform operations
}
Refactored:
public void update() {
// use a non-blocking data structure or synchronized method
}
Why: Reducing synchronous blocks allows more threads to execute concurrently, thus using CPU more effectively.
3. Memory Leaks
Symptoms: Gradual increase in memory usage over time leading to OutOfMemoryErrors.
Causes:
- Holding on to references of objects that are no longer in use.
- Unbounded caches or collections.
Troubleshooting Steps:
- Heap Dumps: Capture heap dumps to analyze retained object sizes using tools like Eclipse MAT.
jmap -dump:live,format=b,file=heapdump.hprof <pid>
- Inspect for Leaks: Use MAT to pinpoint objects consuming excessive memory.
Tip: Look for static variables, singleton patterns, and large collections.
4. Poor Application Performance
Symptoms: Slow response times and high error rates.
Causes:
- Inefficient algorithms or poor database performance.
- External API call delays.
Troubleshooting Steps:
-
Use APM Tools: Tools like New Relic or AppDynamics provide transaction tracing. Study slow transaction traces for bottlenecks.
-
Optimize Database Queries: Use EXPLAIN plans to improve slow-running queries.
Example Query Optimization:
-- Before Optimization
SELECT * FROM users WHERE name LIKE '%john%';
-- Optimized
SELECT * FROM users WHERE name = 'john';
Why: Using precise filters such as exact matches can significantly improve query performance by leveraging indexes.
5. Network Latency and Failures
Symptoms: Increased latency in API requests and connection issues.
Causes:
- Faulty configurations in network settings.
- Issues with external services or dependencies.
Troubleshooting Steps:
-
Measure Network Latency: Use tools like Wireshark or tcptraceroute to diagnose network issues.
-
Connection Pool Monitoring: Monitor connection pools (e.g., with HikariCP) to ensure that connections are not being exhausted or wasted.
Example of Connection Pool Configuration:
HikariConfig config = new HikariConfig();
config.setJdbcUrl("jdbc:mysql://localhost:3306/mydb");
config.setUsername("user");
config.setPassword("password");
config.setMaximumPoolSize(10);
Why: Proper connection pooling ensures efficient database access while avoiding connection overhead.
The Closing Argument
Effective Java monitoring is much more than just collecting metrics. It is about understanding what those metrics mean and how they translate to application performance. By addressing common issues such as high garbage collection pauses, thread contention, memory leaks, poor performance, and network problems, developers can dramatically improve application reliability and performance.
For further reading, you might find these resources useful:
- Effective Java (3rd Edition)
- Java Performance Tuning
By remaining vigilant about monitoring and understanding your application's behavior, you can develop a more robust Java environment that delivers consistent performance.
Happy coding!