Common Pitfalls When Creating DynamoDB Tables with Java
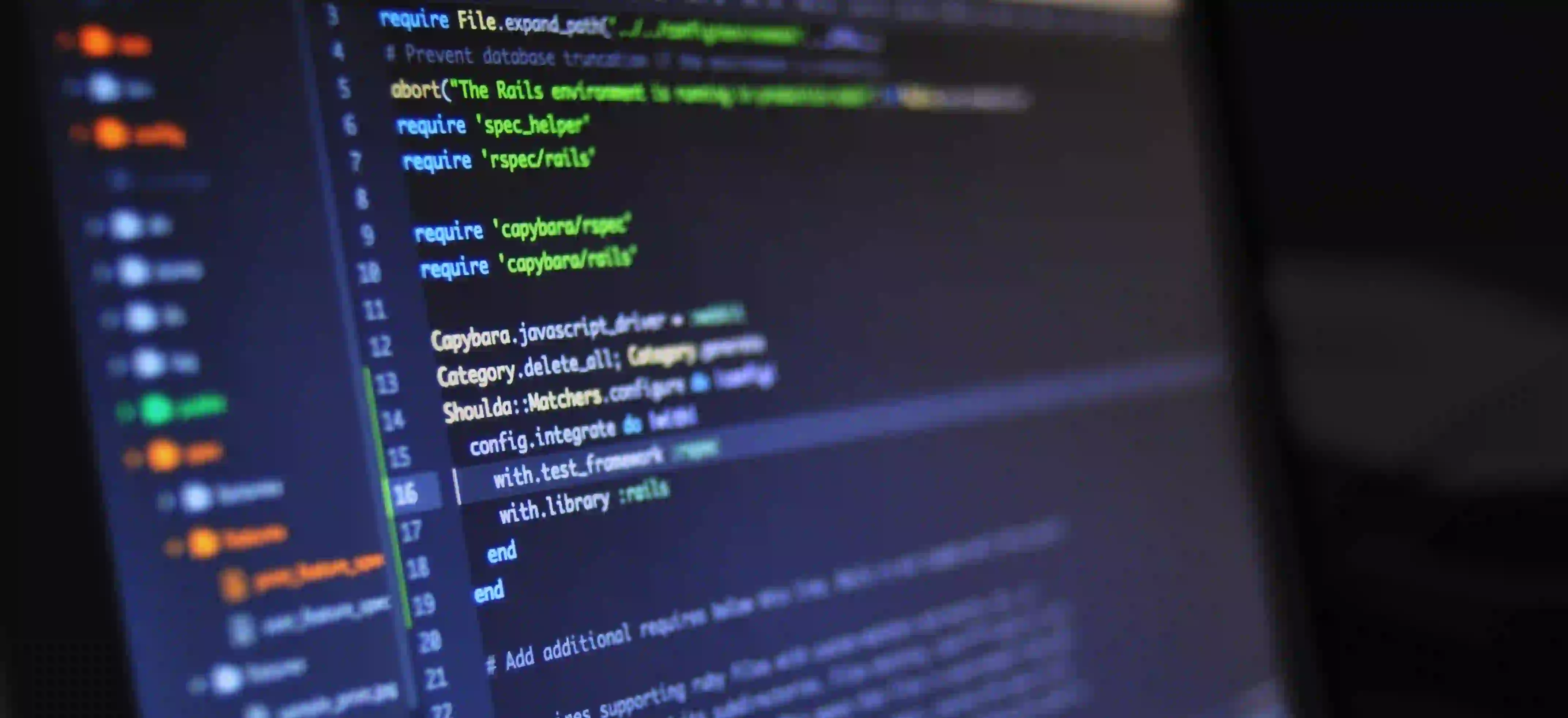
Common Pitfalls When Creating DynamoDB Tables with Java
Amazon DynamoDB is a powerful NoSQL database service that provides high performance and scalability. Its flexible data model enables developers to build applications that can handle a variety of data types and workloads. However, when creating DynamoDB tables, developers often encounter pitfalls that can lead to suboptimal performance or operational difficulties. In this blog post, we will explore some of these common pitfalls and how to avoid them, particularly when using the AWS SDK for Java.
Understanding DynamoDB's Data Model
Before we delve into the pitfalls, it's essential to understand DynamoDB's data model. In DynamoDB, data is organized into tables, items (similar to records), and attributes (similar to fields in records). Each table must have a primary key, which can be either a simple partition key or a composite key made up of a partition key and a sort key.
Essential Concepts to Keep in Mind
- Primary Key Choices: Choose a primary key that allows for efficient querying. A poorly chosen primary key can lead to performance bottlenecks.
- Data Types: DynamoDB supports various data types, including strings, numbers, and binary data. Data type selection can affect performance and storage costs.
- Capacity Modes: Be aware of on-demand versus provisioned capacity modes, as they impact both performance and cost.
Common Pitfalls
1. Ignoring Provisioned Throughput
When creating a DynamoDB table, you can choose between provisioned and on-demand capacity modes. A common pitfall is not understanding the implications of provisioned throughput.
Provisioned Throughput
Provisioned throughput allows you to specify the number of reads and writes per second. Setting these values too low can lead to throttling errors, while setting them too high can incur unnecessary costs.
Code Example
import software.amazon.awssdk.services.dynamodb.model.*;
import software.amazon.awssdk.services.dynamodb.DynamoDbClient;
public class CreateTableExample {
public static void createTable(DynamoDbClient dynamoDbClient) {
CreateTableRequest request = CreateTableRequest.builder()
.tableName("MyTable")
.keySchema(KeySchemaElement.builder()
.attributeName("id")
.keyType(KeyType.HASH) //Partition key
.build())
.attributeDefinitions(AttributeDefinition.builder()
.attributeName("id")
.attributeType(ScalarAttributeType.S) // String type
.build())
.provisionedThroughput(ProvisionedThroughput.builder()
.readCapacityUnits(5L) // Choose according to expected traffic
.writeCapacityUnits(5L)
.build())
.build();
dynamoDbClient.createTable(request);
}
}
Why This Matters
Choosing the right capacity ensures that your application operates smoothly without incurring unexpected charges. Always monitor your actual usage and adjust accordingly.
2. Underestimating the Impact of Composite Keys
Using composite keys involves both a partition key and a sort key. A common mistake is to not properly consider how your application will query the data.
Missing Sort Key Benefits
Failing to utilize a sort key can lead to inefficient data retrieval when you want to query items within the same partition.
Code Example
CreateTableRequest request = CreateTableRequest.builder()
.tableName("MyTable")
.keySchema(
KeySchemaElement.builder()
.attributeName("userId")
.keyType(KeyType.HASH) // Partition Key
.build(),
KeySchemaElement.builder()
.attributeName("orderId")
.keyType(KeyType.RANGE) // Sort Key
.build()
)
.attributeDefinitions(
AttributeDefinition.builder()
.attributeName("userId")
.attributeType(ScalarAttributeType.S)
.build(),
AttributeDefinition.builder()
.attributeName("orderId")
.attributeType(ScalarAttributeType.S)
.build()
)
.provisionedThroughput(ProvisionedThroughput.builder()
.readCapacityUnits(5L)
.writeCapacityUnits(5L)
.build())
.build();
Why This Matters
Selecting appropriate keys can drastically improve query performance by allowing you to retrieve related items efficiently.
3. Not Leveraging Secondary Indexes
Another common oversight is failing to use Global Secondary Indexes (GSIs) or Local Secondary Indexes (LSIs) effectively.
Indexing for Enhanced Query Flexibility
By not creating secondary indexes, you limit your ability to query data flexibly. Queries become less efficient, leading to potentially higher read costs and slower performance.
Code Example
GlobalSecondaryIndex gsi = GlobalSecondaryIndex.builder()
.indexName("UserRoleIndex")
.keySchema(KeySchemaElement.builder()
.attributeName("role")
.keyType(KeyType.HASH)
.build())
.projection(Projection.builder()
.projectionType(ProjectionType.ALL)
.build())
.provisionedThroughput(ProvisionedThroughput.builder()
.readCapacityUnits(5L)
.writeCapacityUnits(5L)
.build())
.build();
CreateTableRequest request = CreateTableRequest.builder()
.tableName("MyTable")
.keySchema(...)
// Add other details
.globalSecondaryIndexes(gsi)
.build();
Why This Matters
Secondary indexes allow you to query additional attributes and retrieve data efficiently without needing to scan the entire table.
4. Not Considering Data Serialization Favorites
When storing complex objects in DynamoDB, developers often overlook how to serialize objects properly.
Serialization Pitfalls
Using inappropriate data structures can lead to increased complexity in querying and can even raise cost implications due to larger item sizes.
Code Example
import software.amazon.awssdk.services.dynamodb.model.*;
Map<String, AttributeValue> itemValues = new HashMap<>();
itemValues.put("id", AttributeValue.builder().s("1").build());
itemValues.put("userInfo", AttributeValue.builder().m(userInfoObject).build()); // Ensure you serialize correctly
PutItemRequest putItemRequest = PutItemRequest.builder()
.tableName("MyTable")
.item(itemValues)
.build();
Why This Matters
Choosing the right serialization strategy affects both the cost and complexity of data manipulation within DynamoDB.
5. Not Planning for Future Growth
Future-proofing your data model is critical. As your application scales, the limitations you impose during the table creation can become bottlenecks.
Code Example
Consider future steel changes, such as more attributes or larger data storage:
// Instead of a fixed schema, consider designing for future attributes.
// e.g., Avoid reliance on hardcoded attributes, and instead allow for flexible item structures
Map<String, AttributeValue> itemValues = new HashMap<>();
itemValues.put("dynamicAttribute", AttributeValue.builder().s("value").build());
Why This Matters
Scalable designs ensure that you do not have to refactor your database frequently, leading to less downtime and maintenance effort.
Bringing It All Together
Creating tables in DynamoDB with Java can be an efficient process if appropriate care is taken. Understanding the fundamental aspects and avoiding common pitfalls—such as improper capacity planning, inefficient key selection, neglecting secondary indexes, poor serialization, and lack of future planning—can lead to a robust infrastructure and a seamless user experience.
By thoughtfully addressing these areas, developers can maximize both performance and cost-effectiveness in their applications using DynamoDB.
For more information on DynamoDB design patterns, consult AWS Documentation. Happy coding!