Common Resteasy Mistakes You Should Avoid in Tutorials
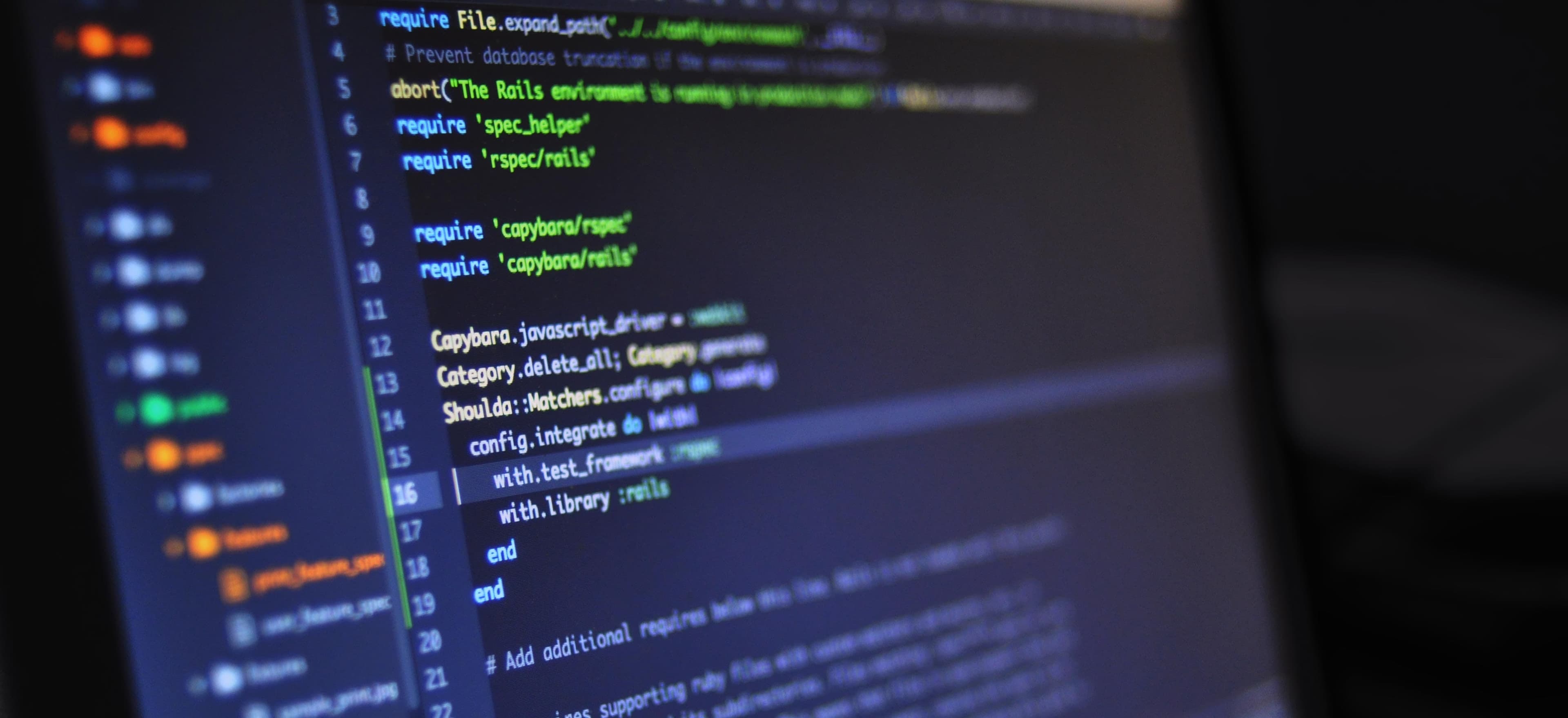
- Published on
Common Resteasy Mistakes You Should Avoid in Tutorials
When diving into the world of Java EE and RESTful web services, Resteasy is often the go-to framework for many developers. It's powerful, flexible, and integrates seamlessly with JAX-RS. However, new developers often make mistakes while following tutorials, which can lead to confusion and frustration. In this blog post, we will explore some common mistakes you should avoid when using Resteasy, while providing actionable tips to improve your RESTful services.
What is Resteasy?
Resteasy is an open-source JAX-RS implementation from Red Hat. It allows Java developers to build RESTful web services with ease, using annotations to simplify endpoint creation and request handling. With Resteasy, you can expose Java objects as REST endpoints, making it easier to communicate with web clients.
1. Ignoring Dependency Management
One of the most frequent oversights is not properly managing dependencies. Using Maven, Gradle, or another build tool, ensure that you import the correct versions of Resteasy and its dependencies.
<dependency>
<groupId>org.jboss.resteasy</groupId>
<artifactId>resteasy-jaxrs</artifactId>
<version>4.7.1.Final</version>
</dependency>
Why: A mismatch in versions can lead to runtime errors that are difficult to debug. Always check the Resteasy documentation for the latest dependencies.
2. Neglecting the Proper Application Path
Another common mistake is failing to set the proper application path for your REST API. When creating a JAX-RS application, it’s vital to define the @ApplicationPath
.
import javax.ws.rs.ApplicationPath;
import javax.ws.rs.core.Application;
@ApplicationPath("/api")
public class MyApplication extends Application {
}
Why: If you don’t set this correctly, your endpoints might not be accessible at the intended URL, leading to 404 Not Found
errors. Always validate your API path by testing it after deployment.
3. Forgetting to Handle HTTP Methods
Developers often overlook the importance of correctly handling HTTP methods such as GET, POST, PUT, DELETE, etc. Using annotations wrong can result in unresponsive endpoints.
import javax.ws.rs.GET;
import javax.ws.rs.Path;
@Path("/items")
public class ItemResource {
@GET
public List<Item> getItems() {
return itemService.getAll();
}
}
Why: Ensure you utilize the right HTTP method for the intended action. Misusing these can lead to unintended behaviors and make your API less intuitive for users.
4. Not Handling Exceptions Properly
Upon an exceptional case, Resteasy might simply return a 500 Internal Server Error without providing any detail. This can frustrate users trying to debug what went wrong.
import javax.ws.rs.*;
@Provider
public class CustomExceptionMapper implements ExceptionMapper<Throwable> {
@Override
public Response toResponse(Throwable exception) {
return Response.status(Response.Status.INTERNAL_SERVER_ERROR)
.entity("Error occurred: " + exception.getMessage())
.build();
}
}
Why: Proper exception handling helps users understand what went wrong. Using custom exception mappers can greatly enhance the usability of your API.
5. Ignoring Content Negotiation
If your API does not support content negotiation, it may lead to confusion among clients expecting a specific content type.
import javax.ws.rs.Produces;
import javax.ws.rs.core.MediaType;
@Produces(MediaType.APPLICATION_JSON)
@Path("/products")
public class ProductService {
@GET
public List<Product> getProducts() {
return productService.getAll();
}
}
Why: Correctly specifying the media type ensures your API reliably communicates the expected response format. This greatly enhances the client experience.
6. Not Using Annotations Correctly
Each JAX-RS annotation has its purpose (e.g., @Path
, @GET
, @POST
). Misusing these annotations can lead to functionality that is hard to grasp and maintain.
@Path("/users")
public class UserResource {
@POST
@Consumes(MediaType.APPLICATION_JSON)
public Response createUser(User user) {
// Logic to create a user
return Response.status(Response.Status.CREATED).build();
}
}
Why: Understanding the purpose of each annotation makes your code cleaner and prevents misinterpretation. Be sure to familiarize yourself with the JAX-RS annotations.
7. Missing Unit Tests and Integration Tests
Skipping unit tests is a critical error many ignore. Unit tests ensure your methods behave as expected.
import org.junit.Test;
import static org.mockito.Mockito.*;
public class ItemResourceTest {
@Test
public void testGetItems() {
ItemService service = mock(ItemService.class);
ItemResource resource = new ItemResource(service);
when(service.getAll()).thenReturn(new ArrayList<>());
List<Item> items = resource.getItems();
assertNotNull(items);
}
}
Why: Testing guarantees that your API endpoints work as intended and help catch errors early in the development process.
8. Not Documenting the API
Finally, neglecting to document your API can be detrimental. Tools like OpenAPI (formerly Swagger) allow you to provide your users with a clear understanding of how to interact with your service.
openapi: 3.0.1
info:
title: My API
version: 1.0.0
paths:
/api/items:
get:
summary: Returns a list of items
responses:
'200':
description: A JSON array of items
Why: Clear documentation is indispensable for both internal and external users. Consider using tools like Springdoc OpenAPI to create documentation automatically.
To Wrap Things Up
Navigating the Resteasy framework for Java EE can be a rewarding experience if approached correctly. By avoiding these common mistakes, you can enhance your development process, improve your API's usability, and ensure a smoother integration experience for clients. Remember to keep your dependencies updated, properly handle HTTP methods and exceptions, employ content negotiation, and document your APIs thoroughly.
For further information, resources, and community support on Resteasy, visit Resteasy's GitHub page or the official documentation.
By keeping these considerations in mind, you're not just writing code; you're creating reliable and robust RESTful web services that stand the test of time. Happy coding!
Checkout our other articles