Unlocking Performance: Latency Lock vs. Synchronization Issues
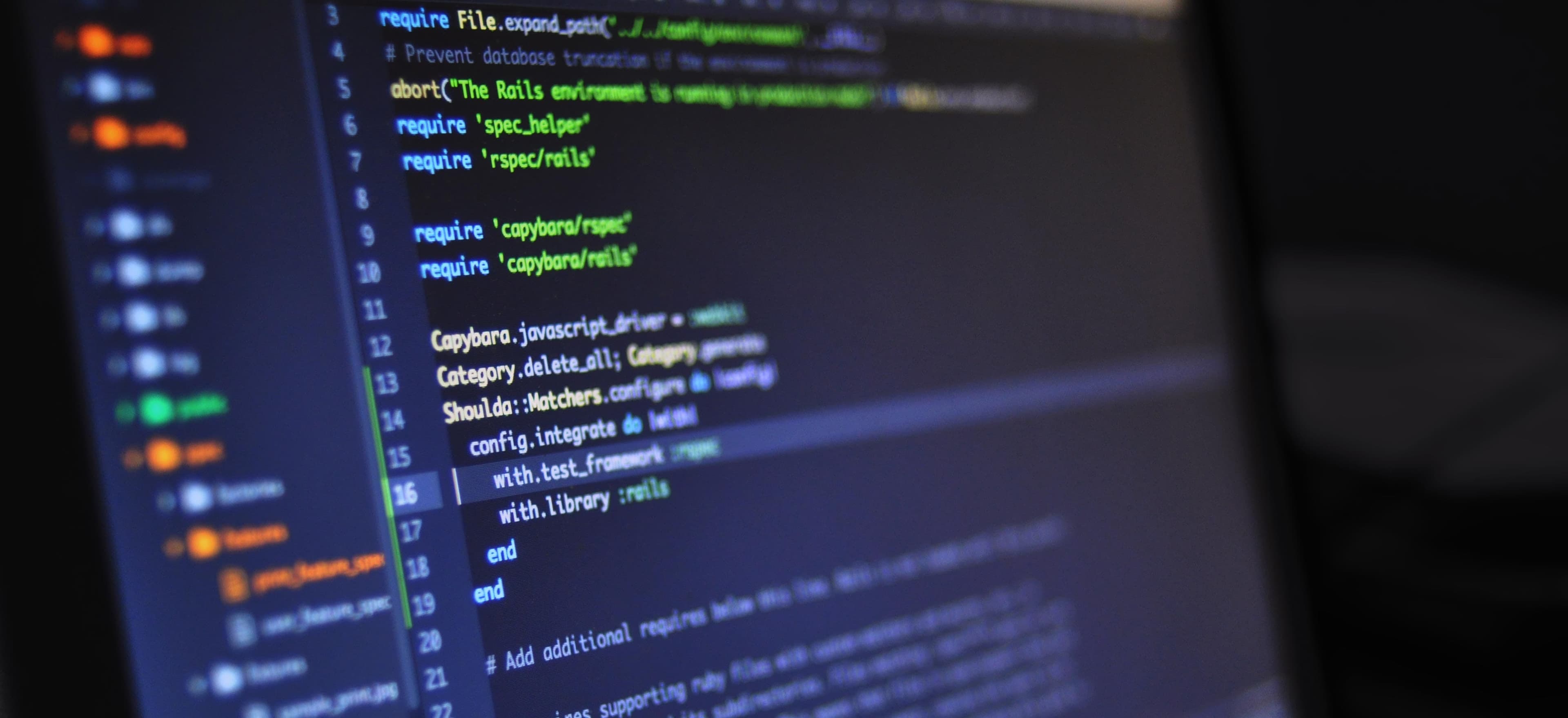
- Published on
Unlocking Performance: Latency Lock vs. Synchronization Issues in Java
Java is widely recognized for its robust architecture and cross-platform capabilities. However, as with any programming language, performance bottlenecks can emerge, particularly concerning latency locks and synchronization issues. Understanding how these two factors interplay can significantly enhance your application's performance. Let's delve deeper into both concepts and explore their implications on Java concurrency.
Understanding the Basics
Before diving into the core of the topic, it's essential to understand what latency locks and synchronization issues are.
What is a Latency Lock?
A latency lock occurs when a thread must wait for a resource that is currently locked due to another thread's operation. This waiting can lead to increased wait times, negatively affecting overall performance. As your application scales, latency locks can become increasingly problematic, resulting in user dissatisfaction due to slow response times.
What are Synchronization Issues?
Synchronization issues arise when multiple threads access shared resources concurrently. Without proper synchronization, threads may lead to inconsistent views of data or even data corruption. This becomes particularly crucial in Java, where thread safety is essential for working with mutable shared objects.
Here's a simple example to illustrate synchronization:
public class SynchronizedCounter {
private int count = 0;
public synchronized void increment() {
count++;
}
public int getCount() {
return count;
}
}
In the code above, increment()
is synchronized, meaning only one thread can execute it at any given time. This prevents other threads from accessing the count
variable while one thread is incrementing it, thus avoiding inconsistencies. However, excessive synchronization can lead to performance bottlenecks.
The Trade-offs Between Latency Locks and Synchronization Issues
Performance vs. Consistency
In an ideal scenario, we want to maximize performance while ensuring data consistency. However, achieving both simultaneously can be challenging. Understanding the dynamics between latency locks and synchronization will help you make informed decisions when designing your Java applications.
-
Latency Locks:
- Pros: Efficient for a single-threaded context or when access contention is minimal.
- Cons: Can lead to increased wait times, especially under heavy loads.
-
Synchronization:
- Pros: Ensures data integrity and consistency when shared resources are accessed.
- Cons: Can introduce overhead, causing threads to be held up.
Example of Latency Lock
Consider the following example of a potential latency lock scenario:
public class LatencyLockDemo {
private final Object lock = new Object();
public void criticalSection() {
synchronized(lock) {
// Simulate long-running operation
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
}
}
In this code, if multiple threads need to enter the criticalSection
method, they will experience significant latency as they wait for the lock to be freed. Consequently, this can lead to performance degradation in applications that require high throughput.
Effective Strategies to Minimize Latency Locks and Synchronization Issues
Achieving optimal performance involves several techniques to balance between latency locks and synchronization issues.
1. Reduce Lock Scope
One of the simplest ways to minimize the impact of latency locks is reducing the scope of synchronized code:
public void executeCriticalOperation() {
int data = performOperation(); // Perform operation outside of synchronized block
synchronized(lock) {
updateSharedResource(data);
}
}
By keeping the synchronized block small, you allow multiple threads to execute the non-critical parts concurrently, thus reducing wait times.
2. Use Concurrent Collections
Java provides several collections explicitly designed for concurrent access, such as ConcurrentHashMap
, which alleviates the need for external synchronization in many scenarios. Here's an example:
import java.util.concurrent.ConcurrentHashMap;
public class ConcurrentMapExample {
private final ConcurrentHashMap<String, Integer> map = new ConcurrentHashMap<>();
public void countWords(String word) {
map.merge(word, 1, Integer::sum);
}
public int getCount(String word) {
return map.getOrDefault(word, 0);
}
}
In this case, the merge
operation is atomic, eliminating the need for explicit locks and thereby improving performance in concurrent scenarios.
3. Consider Using Read/Write Locks
If your application has a higher ratio of read operations compared to write operations, consider utilizing ReadWriteLock
. This allows multiple threads to read the resource simultaneously while still ensuring exclusive access for write operations.
import java.util.concurrent.locks.ReentrantReadWriteLock;
public class ReadWriteLockExample {
private final ReentrantReadWriteLock lock = new ReentrantReadWriteLock();
private int sharedResource;
public void write(int value) {
lock.writeLock().lock();
try {
sharedResource = value;
} finally {
lock.writeLock().unlock();
}
}
public int read() {
lock.readLock().lock();
try {
return sharedResource;
} finally {
lock.readLock().unlock();
}
}
}
In this implementation, multiple threads can read simultaneously, improving efficiency according to their needs while still protecting against write-induced inconsistencies.
4. Use Atomic Variables
Java's java.util.concurrent.atomic
package includes several atomic classes designed to simplify creating thread-safe applications. These classes avoid the need for explicit synchronization. Here's an example using AtomicInteger
:
import java.util.concurrent.atomic.AtomicInteger;
public class AtomicCounter {
private final AtomicInteger count = new AtomicInteger(0);
public void increment() {
count.incrementAndGet();
}
public int getCount() {
return count.get();
}
}
The atomic operations provided by AtomicInteger
enable concurrent updates without explicit locking, significantly enhancing performance.
Summary
Latency locks and synchronization issues are critical considerations when working with Java's concurrency model. Striking the right balance between performance and data consistency is essential for building responsive applications.
Utilize techniques like reducing lock scope, leveraging concurrent collections, adopting read/write locks, and employing atomic variables to optimize your Java code.
For an in-depth understanding of Java concurrency, check out these resources:
- Java Concurrency in Practice - A comprehensive guide by Brian Goetz that covers thread management and concurrency.
- Oracle's Java Tutorials on Concurrency - A detailed resource from Oracle that covers core concurrency concepts.
By implementing these best practices and understanding the interplay of latency locks and synchronization issues, you can unlock the performance potential of your Java applications, delivering optimal user experiences.
Checkout our other articles