Common Pitfalls Setting Up Vaadin on Google App Engine
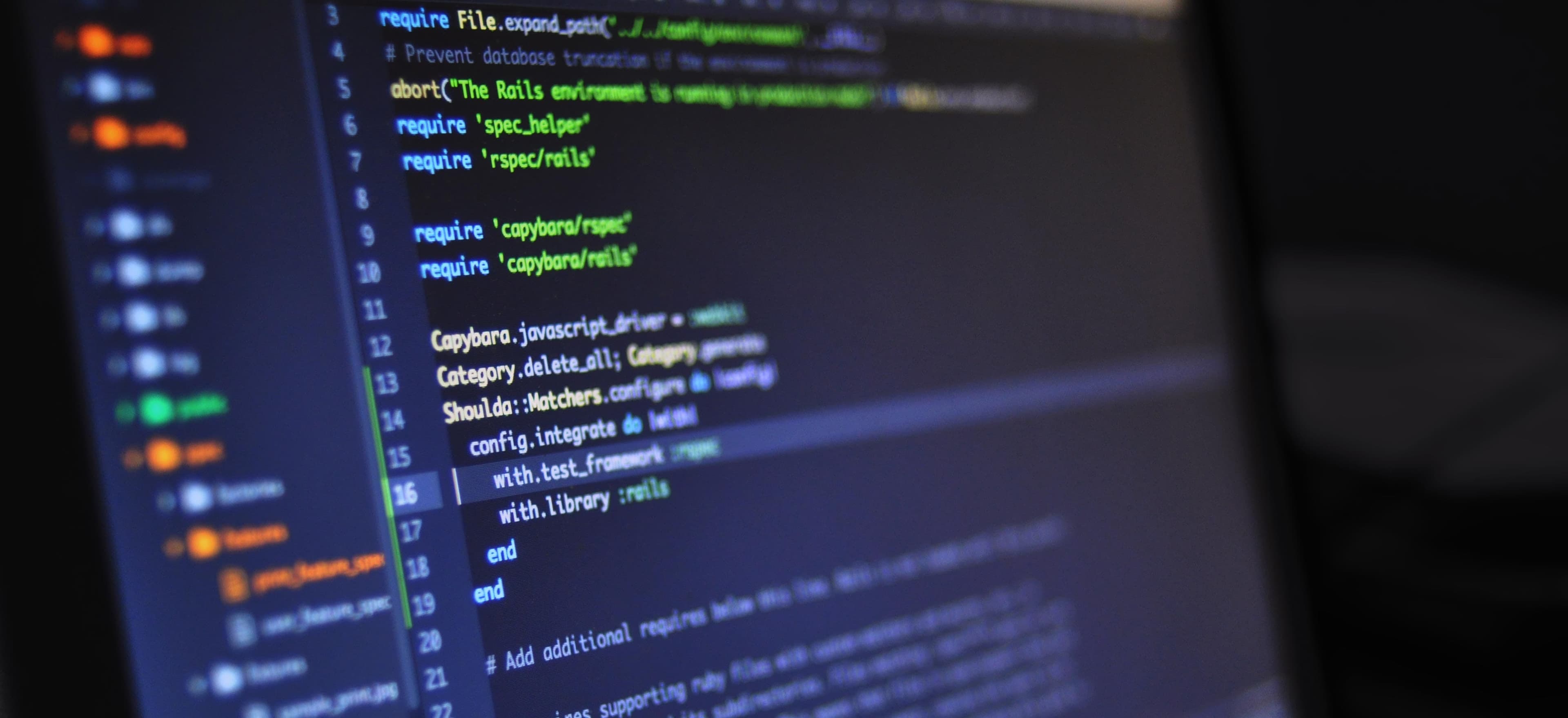
- Published on
Common Pitfalls Setting Up Vaadin on Google App Engine
If you're working with Java and looking to build modern web applications, Vaadin is a framework that deserves your attention. It allows you to create powerful user interfaces with ease. However, deploying your Vaadin application on Google App Engine (GAE) may come with unexpected challenges. In this post, we will explore common pitfalls when setting up Vaadin on GAE, along with actionable solutions to help you navigate smoothly through the process.
Overview of Vaadin and Google App Engine
Vaadin is an open-source web application framework that simplifies the development of single-page applications. It allows developers to build front-end components in Java while abstracting many of the complexities of traditional web development. On the other hand, Google App Engine is a fully managed serverless platform that allows developers to build scalable web applications with minimal infrastructure management.
Before diving into the common pitfalls, let’s briefly outline the key steps involved in setting up a Vaadin project on GAE.
Setting Up Vaadin on Google App Engine: Basic Steps
-
Create a New Vaadin Project: You can use the Vaadin Starter or tools like Vaadin CLI to initiate a new project.
-
Add Dependencies: Make sure to include Vaadin dependencies in your
pom.xml
file if you are using Maven. -
Configure App Engine: You need to set up
app.yaml
, which contains the configuration for your GAE deployment. -
Deploy the Application: Use the Google Cloud SDK to deploy your application to GAE.
This flow is simple, but let's examine some of the common pitfalls you might encounter.
Common Pitfalls
1. Library Compatibility
Pitfall: Incompatibility between Vaadin and Google App Engine libraries can lead to runtime errors.
Solution: Always check the compatibility matrix provided by Vaadin for the version you're using. Keeping your libraries up to date is essential. Note that some older Vaadin versions may not be compatible with the latest GAE Java Runtime.
<dependency>
<groupId>com.vaadin</groupId>
<artifactId>vaadin-core</artifactId>
<version>14.8.6</version>
</dependency>
The above snippet includes the Vaadin Core dependency in your Maven project. Be sure to check for the latest version on the Vaadin Directory.
2. Environment Variables
Pitfall: Failing to configure the necessary environment variables can result in configuration failures or unexpected behavior.
Solution: Define all required environment variables in your app.yaml
file. This helps ensure that your application can access external services like databases.
env_variables:
DATABASE_URL: 'your_database_url'
API_KEY: 'your_api_key'
By specifying your environment variables this way, you ensure that your application can retrieve essential information at runtime without hardcoding sensitive data in your codebase.
3. Static Resources Handling
Pitfall: Sometimes, static resources such as CSS or JavaScript files may not load correctly in GAE.
Solution: Make sure to declare these resources in your app.yaml
properly. Use the static_files
directive to point to any directories containing static assets, like this:
handlers:
- url: /my-static-files
static_dir: static/
This configuration ensures that GAE knows where to serve your static content, improving the loading speed and performance of your application.
4. Session Handling
Pitfall: Vaadin relies on session handling, which may differ in a serverless environment like GAE.
Solution: Utilize the built-in session management provided by Vaadin, but also consider using User ID tokens stored in a lightweight database or in-memory cache.
@Route("")
public class MainView extends VerticalLayout {
public MainView() {
Button button = new Button("Click Me", e -> {
UI.getCurrent().getSession().setAttribute("user", "username");
Notification.show("Session attribute is set.");
});
add(button);
}
}
In the above example, the session attribute can be used across different views, ensuring a seamless user experience.
5. Database Configuration
Pitfall: Misconfiguration of the database can lead to connection issues or performance bottlenecks.
Solution: Use Google Cloud SQL or another managed database solution, and ensure your JDBC driver is in place.
Here is a code snippet for connecting to a Cloud SQL database:
String url = "jdbc:mysql://<INSTANCE_CONNECTION_NAME>/<DATABASE_NAME>";
Connection connection = DriverManager.getConnection(url, "<USER>", "<PASSWORD>");
Ensure you replace <INSTANCE_CONNECTION_NAME>
, <DATABASE_NAME>
, <USER>
, and <PASSWORD>
with your actual database parameters. Your SQL driver must be properly included in your pom.xml
.
6. Network Configuration
Pitfall: Network issues can arise if your application relies on external APIs or microservices.
Solution: Configure your GAE application to allow outbound requests to necessary services. You may also need to deal with CORS to facilitate communication between your frontend and backend services.
@CrossOrigin(origins = "*")
@RestController
public class ApiController {
@GetMapping("/api/data")
public ResponseEntity<Data> getData() {
// Fetch your data
return new ResponseEntity<>(data, HttpStatus.OK);
}
}
This simple controller demonstrates how you can allow access from various origins, making it easier to interact with your Vaadin application.
7. Performance Optimization
Pitfall: Applications that run slowly or inefficiently can frustrate users.
Solution: Utilize GAE's built-in performance monitoring tools to analyze your application's behavior. Optimize your resource usage by limiting the number of active components in your Vaadin UI.
You may consider lazy-loading components or using @Push
to only update specific parts of your application when needed.
@Push
public class LazyLoadedView extends HorizontalLayout {
public LazyLoadedView() {
// Only some components are initialized at start.
}
}
In this example, @Push
enables real-time updates while keeping the application responsive by reducing the number of components rendered initially.
My Closing Thoughts on the Matter
Setting up Vaadin on Google App Engine can be rewarding but fraught with challenges. By avoiding common pitfalls such as library incompatibility, inadequate session management, and improper database configuration, you can streamline your development process and ensure your application performs at its best.
For detailed documentation on Vaadin, visit the Vaadin Documentation. If you want to dive deeper into deploying applications on Google Cloud, check out the Google Cloud Documentation.
With the right preparation and knowledge, deploying your Vaadin application on Google App Engine will be a smoother and more enjoyable experience. Happy coding!
Checkout our other articles