Why Java 8 Functional Programming Can Confuse Developers
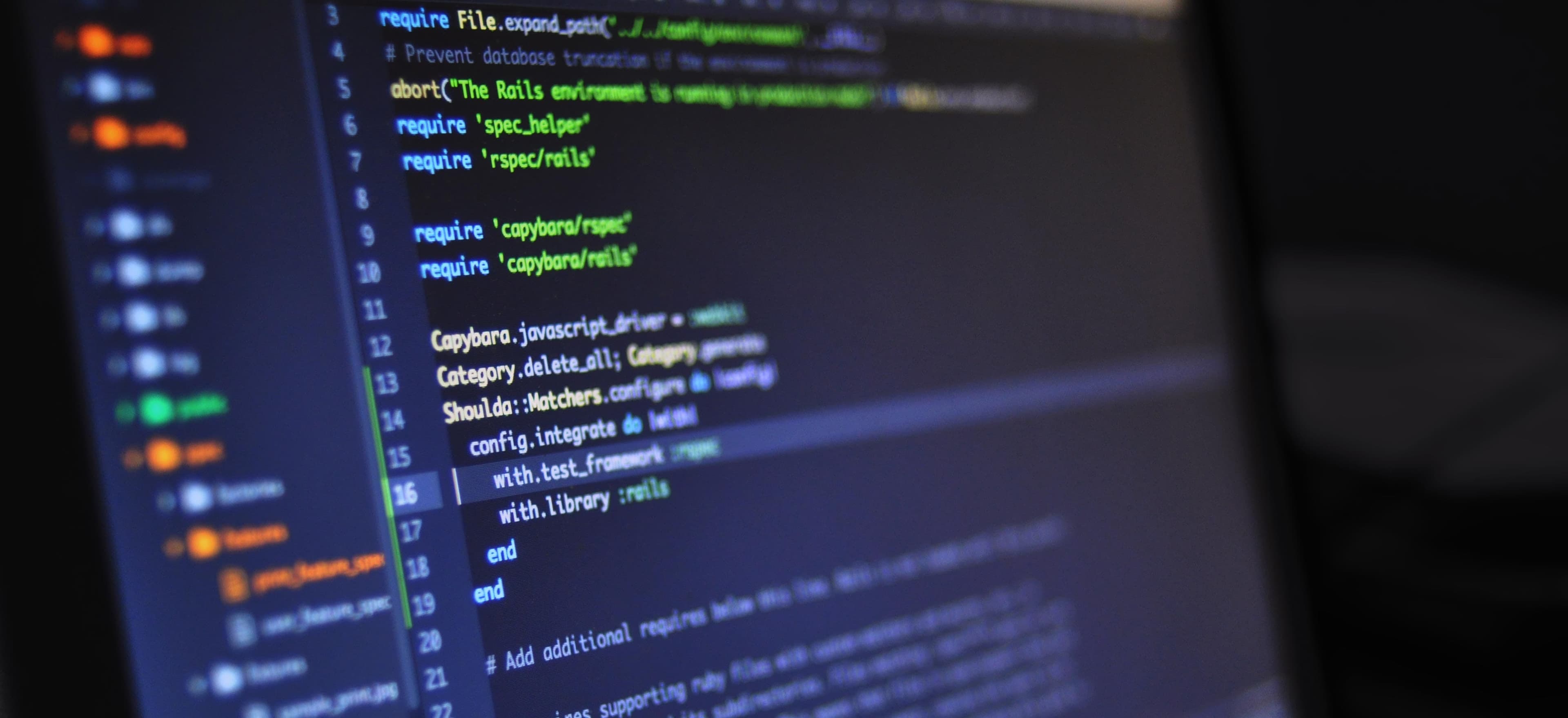
- Published on
Why Java 8 Functional Programming Can Confuse Developers
Java 8 introduced significant changes to the language by incorporating functional programming concepts. While this shift opens up new avenues for writing cleaner and more efficient code, it can lead to confusion, especially for developers who are accustomed to the traditional object-oriented style of Java. In this blog post, we will explore why these changes can be disorienting. We will dissect key features of functional programming in Java 8—like lambdas, streams, and method references—and provide illustrative code snippets to clarify these concepts.
Understanding Functional Programming
Functional programming (FP) is a programming paradigm that treats computation as the evaluation of mathematical functions and avoids changing state and mutable data. This paradigm is very different from the object-oriented programming (OOP) model, which focuses on using objects to represent state and behavior.
Java has traditionally been an OOP language, so the introduction of FP in Java 8 marked a significant paradigm shift. Here are three crucial features of Java 8 that encapsulate this shift.
1. Lambda Expressions
What it is: A lambda expression is a new and concise way to represent a function interface.
Why it can confuse: Developers coming from a traditional OOP background may struggle to understand the syntax and the concept of function interfaces.
Example of Lambda Expression
// Traditional way
Runnable runnable = new Runnable() {
@Override
public void run() {
System.out.println("Hello, World!");
}
};
// Lambda expression way
Runnable runnableLambda = () -> System.out.println("Hello, World!");
Commentary: As seen in the example above, the lambda expression syntax is much more compact and readable. However, its concise nature might lead developers to overlook the underlying function interface it implements—Runnable
. Understanding this connection is essential for leveraging lambdas effectively.
2. Streams API
What it is: The Streams API allows developers to process collections of data in a functional style.
Why it can confuse: The way streams are chained together can be unfamiliar to OOP developers, who may be more accustomed to for-loops and manual data manipulation.
Example of Streams in Action
import java.util.Arrays;
import java.util.List;
public class StreamExample {
public static void main(String[] args) {
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "David");
// Using Streams API
names.stream()
.filter(name -> name.startsWith("A"))
.map(String::toUpperCase)
.forEach(System.out::println);
}
}
Commentary: In this example, we filter out names that start with "A", convert them to uppercase, and print them. The fluent style enables cascading operations, which can feel alien to developers used to imperative programming. Learning how to read and interpret this chaining is pivotal for adapting to FP.
3. Method References
What it is: Method references provide a way to refer to methods without executing them.
Why it can confuse: This feature can seem like syntactical sugar, leading to misunderstandings about its unique advantages and use cases.
Example of Method References
import java.util.Arrays;
import java.util.List;
public class MethodReferenceExample {
public static void main(String[] args) {
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
// Using method reference
names.forEach(System.out::println);
}
}
Commentary: The method reference System.out::println
is a concise alternative to using a lambda expression like name -> System.out.println(name)
. Understanding when and how to utilize method references can greatly enhance code clarity and maintainability, a significant shift from verbose OOP practices.
Common Pitfalls for Developers
With these powerful features come some typical pitfalls that can lead to confusion among developers:
1. Understanding the Functional Interface
A functional interface is an interface with a single abstract method. Lambda expressions can only be assigned to functional interfaces.
Pitfall: Many developers struggle with recognizing a functional interface at first glance, which can result in runtime errors or misunderstandings about lambda applicability.
2. Stream Processing and Short-Circuiting
Streams can efficiently process large data sets. However, some operations are stateful and could lead to unexpected results.
Pitfall: For example, using distinct()
or sorted()
can change how a subsequent operation behaves. Understanding the state of the stream’s data, given its lazy nature, is vital for maintaining code correctness.
3. Implicit vs Explicit Types in Lambda Expressions
Developers used to explicitly defining types in OOP may overlook type inference in lambda expressions.
Pitfall: Omitting type annotations can sometimes lead to misunderstandings about what the lambda is expected to do—or even result in type mismatch errors.
Embracing Functional Programming in Java 8
While functional programming can initially seem confusing, the benefits of using it in Java 8 far outweigh the initial learning curve. By mastering its features, developers can write cleaner, more efficient, and more maintainable code.
Here are some tips to embrace FP:
-
Practice Regularly: Write small programs focusing solely on using lambdas, streams, and method references. Familiarity will breed comfort.
-
Learn Through Examples: Study well-documented Java 8 projects on platforms like GitHub to see how other developers manage these concepts.
-
Leverage Resources: Utilize online resources, such as Java Documentation, and tutorials dedicated to Java 8 features to deepen your understanding.
-
Refactor Existing Codes: Try refactoring traditional Java code into functional-style code, which can be an enlightening exercise to grasp the differences.
-
Community Engagement: Participate in forums such as Stack Overflow or the Java subreddit to engage with fellow developers and resolve issues collaboratively.
Final Considerations
Java 8’s introduction of functional programming concepts may pose challenges but also serves as a gateway to more efficient and elegant coding practices. By understanding and embracing these concepts, traditional Java developers can significantly enhance their skill sets and contribute to more sophisticated applications.
As you dive into Java 8's functional programming, remember that mastery comes with time and practice. Embrace the journey, and soon you may find that the power of functional programming becomes a valuable part of your Java toolkit.
Checkout our other articles