Mastering Merging Collectors in JDK 12: A Practical Guide
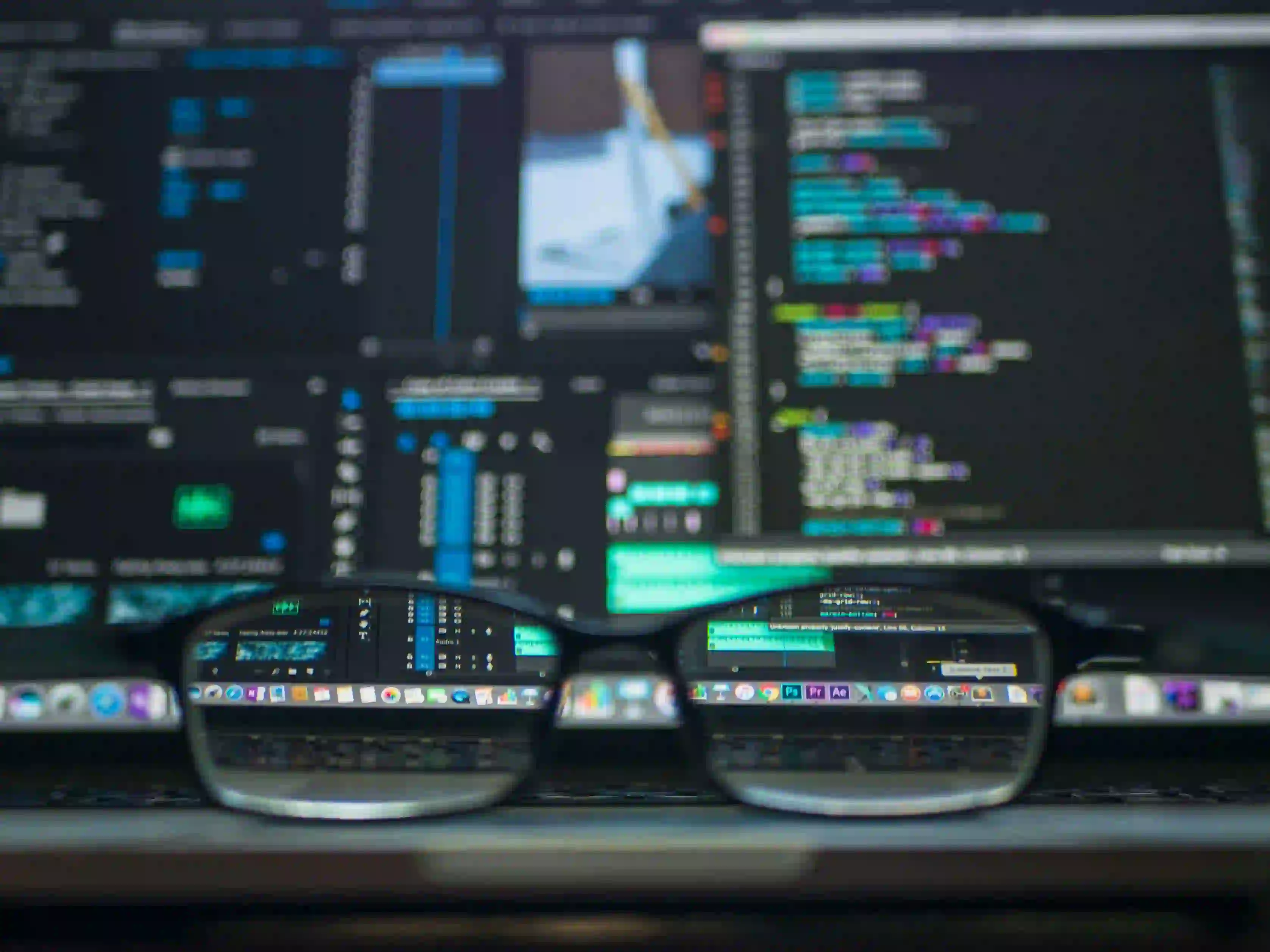
Mastering Merging Collectors in JDK 12: A Practical Guide
In the rapidly evolving world of Java, the introduction of new features can significantly impact how developers approach common programming tasks. One such feature that emerged in JDK 12 is the ability to create merging collectors. This functionality allows developers to aggregate and manipulate data in new and creative ways, enhancing the overall power of the Streams API. In this blog post, we'll dive deep into merging collectors, explore examples, and understand why they are a game-changer in modern Java programming.
Understanding Collectors
Before diving into merging collectors, it's essential to understand what collectors are in Java. Collectors are a part of the Streams API, which provides a method to accumulate elements into a collection. The Collectors
class in Java offers several pre-defined collectors like toList()
, toSet()
, and toMap()
.
Here's a quick example to illustrate this:
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class Main {
public static void main(String[] args) {
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
// Using the toList collector
List<String> nameList = names.stream()
.collect(Collectors.toList());
System.out.println(nameList); // Output: [Alice, Bob, Charlie]
}
}
In this snippet, we convert a list of names into a new list using the toList
collector. It's simple, straightforward, and efficient. However, with the introduction of merging collectors, we can address more complex scenarios.
What are Merging Collectors?
Merging collectors allow you to combine values in a way that they can be reduced or transformed into a single outcome. They flourished into existence primarily for situations where you need to merge two or more accumulating results.
In JDK 12, the method Collectors.teeing()
was introduced, which provides a significant advantage—merging results from two different collectors into one. This means we can concurrently collect data in separate ways and then merge those results into a single outcome, making it immensely handy for diverse data aggregation tasks.
Use Case: Merging Numeric Values
Let's explore an example where we want to count the even and odd numbers in a list and compute their sums separately. Using merging collectors, we can achieve that seamlessly.
Example Code Snippet:
import java.util.Arrays;
import java.util.List;
import java.util.IntSummaryStatistics;
import java.util.stream.Collectors;
public class MergingCollectorsDemo {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
IntSummaryStatistics evenStats = numbers.stream()
.filter(num -> num % 2 == 0)
.collect(Collectors.summarizingInt(num -> num));
IntSummaryStatistics oddStats = numbers.stream()
.filter(num -> num % 2 != 0)
.collect(Collectors.summarizingInt(num -> num));
// Merging results
IntSummaryStatistics combinedStats = Collectors.teeing(
Collectors.summarizingInt(num -> num),
Collectors.summarizingInt(num -> num),
(even, odd) -> new IntSummaryStatistics(
even.getCount() + odd.getCount(),
even.getSum() + odd.getSum(),
Math.min(even.getMin(), odd.getMin()),
Math.max(even.getMax(), odd.getMax()),
even.getCount() + odd.getCount())
);
IntSummaryStatistics stats = numbers.stream().collect(combinedStats);
System.out.println("Combined Stats: " + stats);
}
}
Explanation:
- Filtering: We first filter the even and odd numbers from the
numbers
list. - Statistics: We collect statistics for even and odd numbers separately using
summarizingInt
. - Merging: We use
Collectors.teeing()
to merge the two summaries—this method takes two collectors and a merging function. It combines the results into a singleIntSummaryStatistics
instance.
The final output provides a comprehensive summary of both even and odd numbers, revealing their counts, sums, and ranges. This example highlights how merging collectors enable more elegant solutions without complicating the logic.
Real-world Applications
Merging collectors are particularly useful in scenarios such as:
- Data Analysis: When processing large sets of data, merging multiple results can reduce the need for additional passes over data.
- Reporting: Aggregating different summary information (like counts and averages) into a single report.
- Finance: In financial applications, you could merge results from different financial calculations and statistics, providing a detailed overview in a consolidated format.
Performance Considerations
While the allure of merging collectors is strong, developers must remain aware of performance trade-offs. When combining multiple streams, they could potentially lead to higher computational complexity, depending on the volume of data. Always test and profile your applications to ensure you're achieving the desired performance, especially in production environments.
The Closing Argument
The introduction of merging collectors in JDK 12 is a significant addition to the Java language, empowering developers to write cleaner and more efficient code. By learning how to leverage these merging capabilities, you can transform how you collect and aggregate data in Java.
Accustomed to the traditional ways of using collectors? It's time to embrace the complexities and unlock the full potential that merging collectors provide. For more detailed information on Java Streams and Collectors, you can also check out the official Java documentation.
As Java continues to evolve, mastering new features like merging collectors will differentiate you as a proficient and agile developer. Happy coding!