Realign Your Code: Stop Making Excuses for Bugs
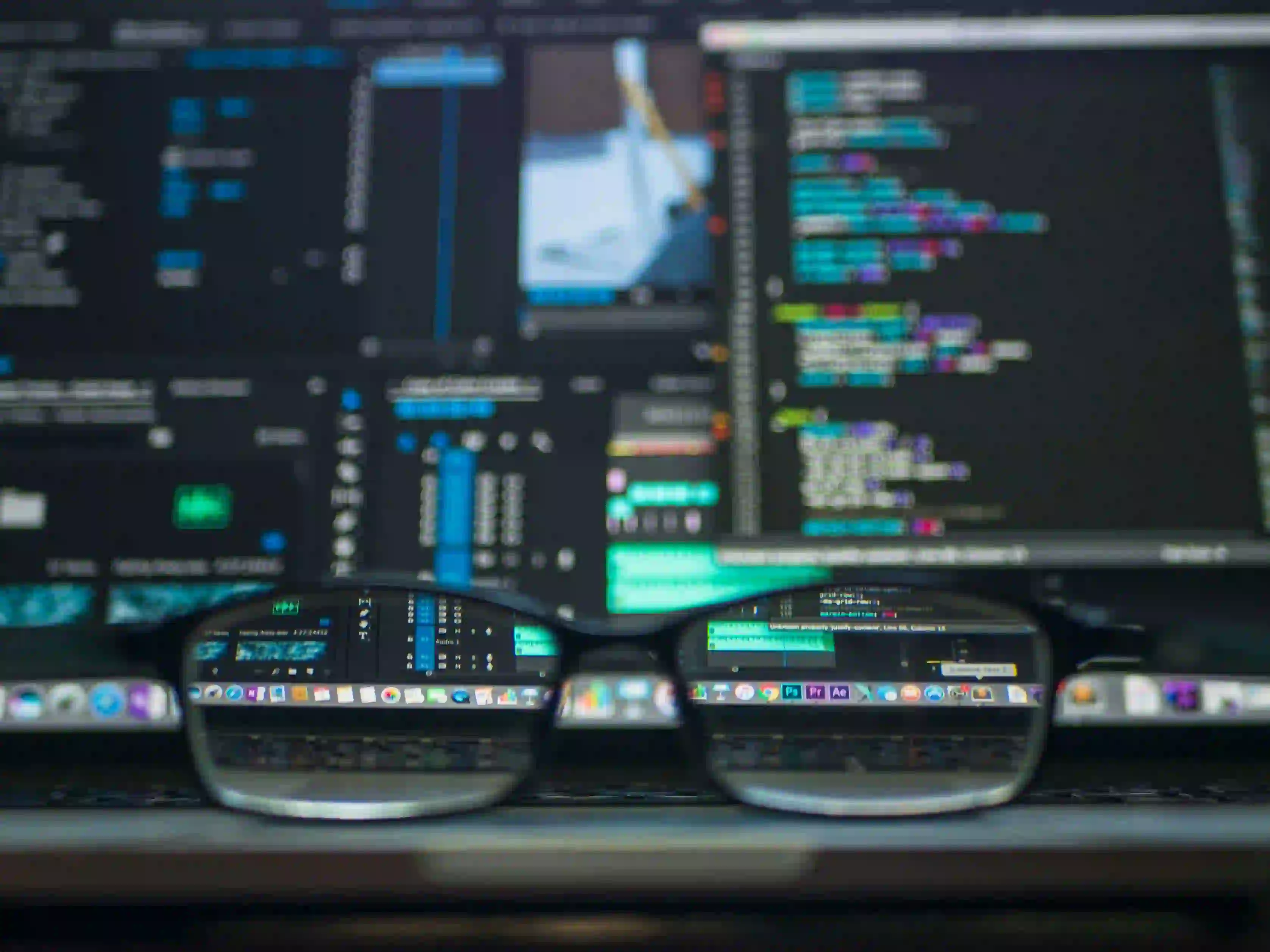
Realign Your Code: Stop Making Excuses for Bugs
In the realm of software development, bugs are an unavoidable reality. Every developer encounters them; they are part of the coding life cycle. However, the narrative surrounding bugs shouldn’t be about acceptance or resignation. Instead, we should focus on understanding, preventing, and fixing them effectively. In this blog post, we will discuss strategies to minimize bugs in your Java applications.
Understanding the Root Causes of Bugs
Before diving into prevention techniques, it's essential to understand what causes bugs. These can range from syntactical errors to logical errors and even environmental discrepancies.
Some common causes include:
- Poor design: Not planning your application architecture leads to convoluted code that is prone to errors.
- Inadequate testing: Skipping unit testing makes it difficult to catch problems early.
- Wrong assumptions: Misunderstanding the specifications or requirements can lead to significant bugs.
Being aware of these causes allows you to develop strategies tailored to mitigate them.
1. Embrace Clean Code Principles
One of the most effective ways to reduce bugs is through clean coding practices. Clean code is not just about aesthetics; it enhances readability and maintenance, which in turn helps in identifying issues.
Here are a few principles to follow:
- Meaningful Naming: Choose descriptive names for your variables and methods.
// Poor naming example
int x;
// Better naming
int userCount; // Clear indication of what the variable is holding
- Keep Functions Small: Ideally, a function should do one thing, and do it well. This makes debugging more manageable.
// Poor function structure
void process() {
fetchData();
saveData();
sendNotification();
}
// Better function structure
void fetchData() {
// Fetch data from the source
}
void saveData() {
// Save data to the database
}
void sendNotification() {
// Notify the user
}
Following these principles not only invites more maintainable code but also enhances team collaboration. If every developer adheres to consistent naming and structure, code reviews and bug tracking become significantly easier.
2. Implement Automated Testing
Don’t rely solely on manual testing. Automated testing is crucial for detecting regressions that might introduce new bugs. In Java, you can utilize various frameworks for this purpose, such as JUnit and Mockito.
Example: JUnit Test Case
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class CalculatorTest {
@Test
public void testAdd() {
Calculator calculator = new Calculator();
assertEquals(5, calculator.add(2, 3));
}
}
In this snippet, we are checking if the add
method in our Calculator
class works correctly. By running this JUnit test after any changes, we ensure that previous functionality remains intact. This proactive approach minimizes the risk of bugs making their way into production.
3. Code Reviews: Never Underestimate Peer Feedback
Engaging in code reviews is an effective way to ensure the quality of your code. Another set of eyes can spot potential issues you might have overlooked.
How to Conduct Effective Code Reviews
- Be Constructive: Offer feedback in a manner that encourages improvement rather than discouragement.
- Focus on Intent: Understanding why the code was written a certain way can lead to actionable insights.
- Use Tools: Leverage platforms like GitHub or Bitbucket that provide features for inline comments.
Example:
In a hypothetical code review, a developer might raise a concern:
// Original Logic
public boolean isEven(int number) {
return number % 2 == 1; // Incorrect logic
}
The reviewer could point out the logic error, leading to a quick fix:
// Fixed Logic
public boolean isEven(int number) {
return number % 2 == 0; // Corrected logic
}
4. Continuous Integration (CI) Tools
Utilizing Continuous Integration tools can significantly improve your development workflow. CI systems like Jenkins or Travis CI will ensure that your tests run on every commit, thus catching issues before they reach the production environment.
Simple Jenkins Pipeline Example
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'mvn clean package'
}
}
stage('Test') {
steps {
sh 'mvn test'
}
}
}
}
This simple Jenkins pipeline automatically builds your project and runs your tests. Automating these tasks helps to maintain the integrity of your codebase and reduces the window of opportunity for bugs to be introduced.
5. Effective Logging and Debugging
Even with the best practices in place, bugs will occur. When they do, clear logging practices will aid in quickly identifying and fixing them.
Example of Simple Logging Using Log4j
First, add the Log4j dependency to your pom.xml
.
<dependency>
<groupId>log4j</groupId>
<artifactId>log4j</artifactId>
<version>1.2.17</version>
</dependency>
Then set up logging in your application.
import org.apache.log4j.Logger;
public class UserService {
private final static Logger logger = Logger.getLogger(UserService.class);
public void createUser(String username) {
logger.info("Creating user: " + username);
// User creation logic
}
}
In this example, we log a message when a new user is being created. If something goes wrong, you can trace back through the logs to identify where the failure occurred. Effective logging gives you the insight needed to address bugs quickly.
Closing the Chapter
There is no magic formula for completely eradicating bugs from your Java applications, but that doesn't mean you have to let them run rampant either. By embracing clean code principles, implementing thorough testing, conducting peer reviews, utilizing CI tools, and enhancing your logging practices, you can significantly reduce the frequency and impact of bugs in your projects.
Remember, accountability is key. Instead of making excuses for bugs, take ownership of your code. Continuous learning and improvement should be at the forefront of your coding practices. By doing so, you’ll not only enhance your own skills but also contribute positively to your team and the larger development community.
For more insights on Java development and best practices, consider exploring additional resources: Oracle Java Documentation and Effective Java.
Happy coding!