Simplifying Apache Camel 3.2 Configurations Without Reflection
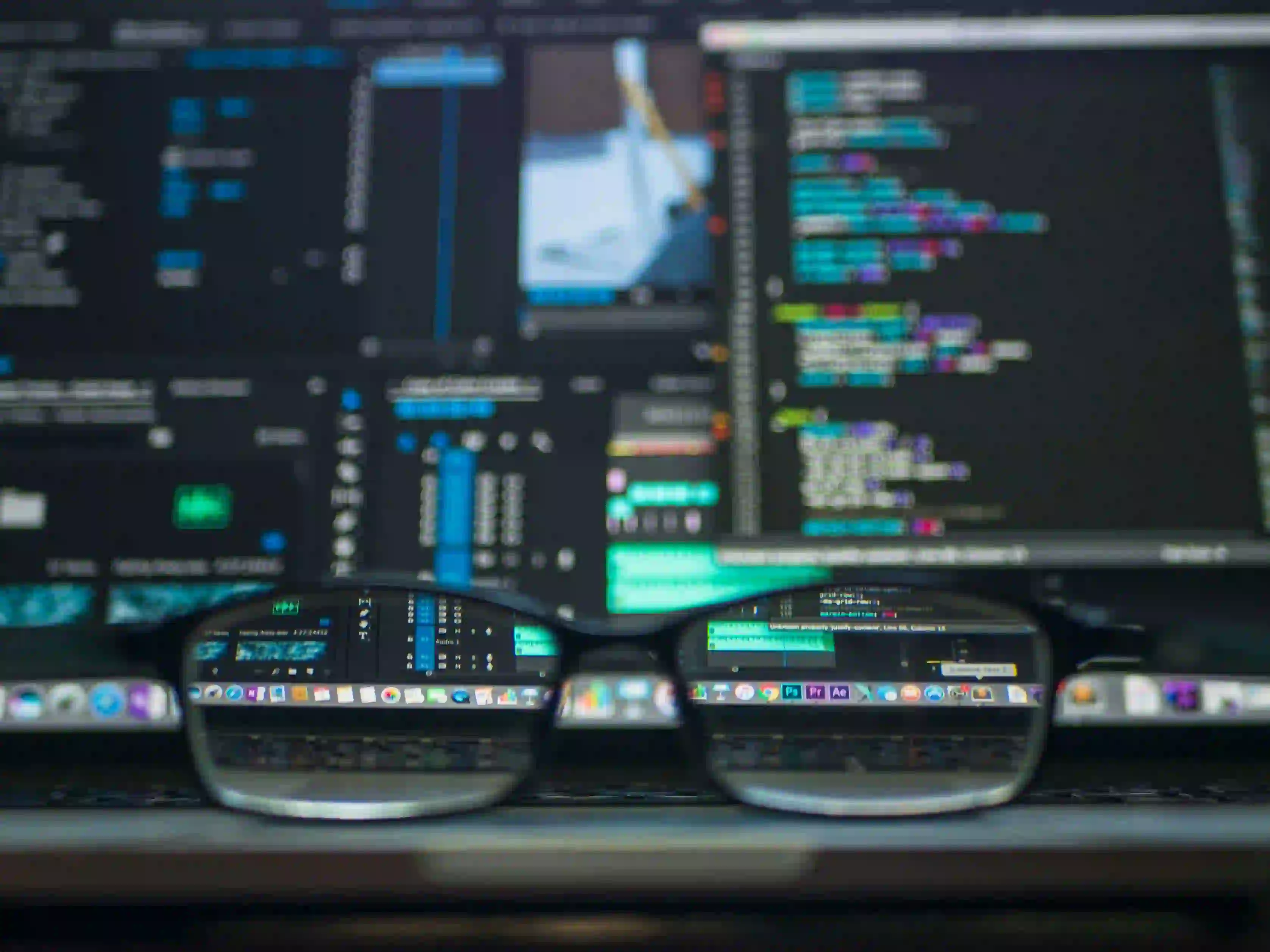
Simplifying Apache Camel 3.2 Configurations Without Reflection
Apache Camel is a powerful integration framework that helps developers create robust message-driven applications. With its support for various Enterprise Integration Patterns (EIPs) and a multitude of components, Camel makes it easy to integrate different systems. However, as your applications grow, the complexity of your Camel configuration can increase rapidly.
In version 3.2, Apache Camel introduced capabilities that simplify its configuration, but there are still many developers who rely on reflection to manage their settings. This blog post will explore how to streamline your Apache Camel 3.2 configurations without resorting to reflection.
Why Avoid Reflection in Apache Camel?
Reflection allows for dynamic access to classes and their fields at runtime, but it comes with a set of drawbacks:
- Performance Overhead: Reflection can be slower than direct method calls or field access.
- Increased Complexity: Using reflection can make code more difficult to understand and maintain.
- Type Safety: Reflection does not offer compile-time type checking, leading to potential runtime errors.
With these factors in mind, we will discuss alternative approaches for configuration management in Apache Camel 3.2 that are both efficient and easy to understand.
Understanding Apache Camel Configurations
Apache Camel allows you to define routes and components usually in XML, Java, or using its DSL (Domain Specific Language). Traditionally, developers might use reflection to load properties or dynamically modify routes or beans at runtime. However, Apache Camel's 3.2 release offers several new features that minimize the need for such complexity.
Key Features in Camel 3.2
- Kotlin DSL: Offers a more concise and expressive way to define routes.
- Simplified Property Handling: Enhanced support for configuration properties via typesafe builders.
- Enhanced Camel K: Streamlined process for deployments, especially in a Kubernetes environment.
Configuring Camel with Properties
One of the simplest ways to manage your Apache Camel configurations is by using a properties file. This method enhances readability and maintainability.
Step 1: Create a Properties File
Create a camel.properties
file in the src/main/resources
directory:
# camel.properties
myService.endpoint=http://localhost:8080/api
myService.timeout=5000
Step 2: Load Properties in Your Route
Next, load these properties directly into your Camel routes without reflection. Below is an example of how to achieve that using the Java DSL:
import org.apache.camel.builder.RouteBuilder;
public class MyCamelRoute extends RouteBuilder {
@Override
public void configure() {
// Retrieve properties
String endpoint = getContext().getPropertiesComponent().parseUri("{{myService.endpoint}}");
String timeout = getContext().resolvePropertyPlaceholders("{{myService.timeout}}");
// Log the endpoint and timeout
log.info("Service Endpoint: {}", endpoint);
log.info("Service Timeout: {}", timeout);
// Define the route
from("direct:start")
.to(endpoint)
.log("Request sent to: " + endpoint)
.setHeader("CamelHttpResponseCode")
.to("http://localhost:8080/api?timeout=" + timeout);
}
}
Explanation of Code
- getContext().getPropertiesComponent(): This allows direct access to properties without reflection.
- parseUri(...): Uses Camel's built-in capability to replace placeholders in URIs with property values.
- resolvePropertyPlaceholders(...): This method helps to retrieve values while ensuring they're parsed.
Benefits of Direct Property Access
By using Camel's property handling, you're able to simplify your routes and make them more readable. Developers can easily modify configurations in the properties file without diving into the route logic, thereby reducing the maintenance overhead.
Using Camel's Java DSL for Concise Configurations
Camel's Java DSL provides a fluent and expressive way to define routes, which can simplify or even eliminate the need for reflection.
Defining a Route with Java DSL
Consider this straightforward example that demonstrates how to define a route using the Java DSL:
import org.apache.camel.builder.RouteBuilder;
public class SimpleRouteBuilder extends RouteBuilder {
@Override
public void configure() {
from("timer:foo?repeatCount=5")
.routeId("TimerRoute")
.log("Tick at ${header.fireTime}")
.to("log:info");
}
}
Key Characteristics
- Fluency: The method chaining style makes it clear what happens at each step.
- Clarity: No need to use reflection to manipulate class properties or manage route states.
Using Configuration Classes
Another excellent way to avoid reflection while simplifying your configuration is by defining your settings using configuration classes.
Step 1: Define Configuration Class
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.stereotype.Component;
@Component
@ConfigurationProperties(prefix = "myService")
public class MyServiceConfig {
private String endpoint;
private int timeout;
// Getters and Setters
public String getEndpoint() {
return endpoint;
}
public void setEndpoint(String endpoint) {
this.endpoint = endpoint;
}
public int getTimeout() {
return timeout;
}
public void setTimeout(int timeout) {
this.timeout = timeout;
}
}
Step 2: Use Configuration Class in a Route
You can now inject this configuration into your Camel routes without needing to resort to reflection:
import org.apache.camel.builder.RouteBuilder;
import org.springframework.beans.factory.annotation.Autowired;
public class MyServiceRoute extends RouteBuilder {
@Autowired
private MyServiceConfig myServiceConfig;
@Override
public void configure() {
from("direct:start")
.to(myServiceConfig.getEndpoint())
.log("Request sent to: " + myServiceConfig.getEndpoint() + " with timeout: " + myServiceConfig.getTimeout());
}
}
Benefits of Configuration Classes
- Type Safety: With this approach, you benefit from compile-time type checking.
- Environment-Specific Configuration: You can use profiles to manage different configurations, making it easier to work across different environments.
- Clear Separation: It promotes a clean architectural design by separating configuration logic from business logic.
The Closing Argument
In a world where readability and maintainability are crucial, avoiding reflection in Apache Camel 3.2 can lead to better-structured code and improved performance. By leveraging the framework's improved property handling, utilizing the powerful Java DSL, and implementing configuration classes, you can simplify your Camel configurations.
Each of these strategies will result in clearer, more concise configurations that reduce the potential for bugs and enhance the overall development experience.
By investing time in simplifying your configurations, you're not just promoting code on a micro level; you're enhancing collaboration and scalability on an organizational level too.
For further reading on Apache Camel, you can explore their official documentation on Apache Camel and Spring Boot integration.
Happy coding!