Troubleshooting Kafka Consumer Offset Issues: A Quick Guide
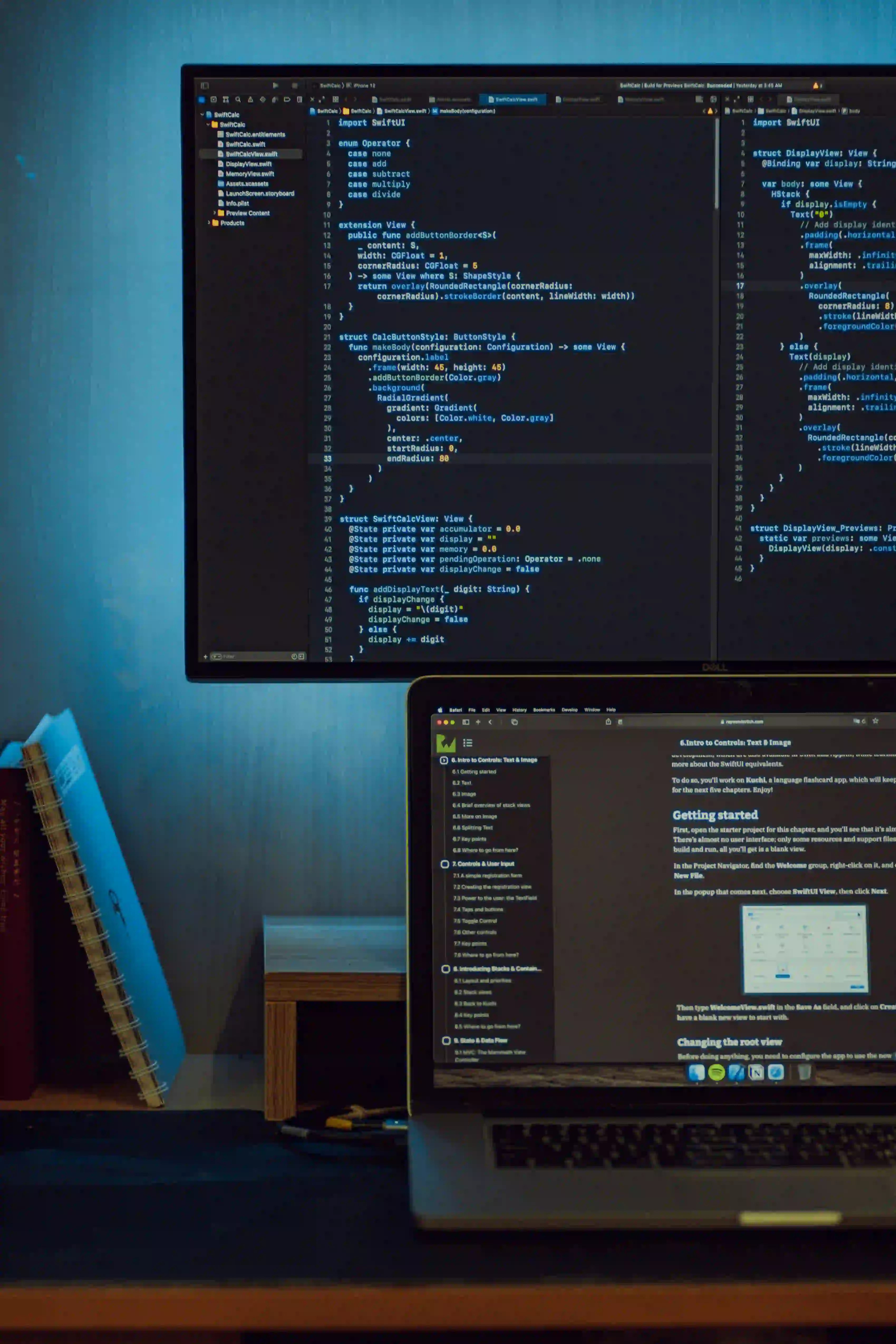
Troubleshooting Kafka Consumer Offset Issues: A Quick Guide
Apache Kafka has gained immense popularity as a distributed streaming platform suitable for building real-time data pipelines. However, like any complex system, it can present challenges. One common issue developers face is consumer offset management. In this blog post, we'll explore how to troubleshoot Kafka consumer offset issues effectively.
Understanding Kafka Offsets
Before diving into troubleshooting, let’s briefly understand what offsets are in Kafka. Each message in a Kafka partition has a unique offset, which is an integer value representing the position of the record within the partition. Consumers use this offset to track their progress in reading messages.
When consumers read messages from Kafka, they can control how offsets are managed. This can be done automatically or manually. Knowing how offsets affect consumption is crucial for resolving issues.
Key Concepts
- Consumer Groups: Groups of consumers that work together to process messages. Each consumer in a group reads from a unique set of partitions.
- Committing Offsets: The process in which a consumer signals that a message has been successfully processed.
- Rebalancing: Happens when consumers join or leave a group, leading to potential offset challenges.
Common Offset Issues and Their Solutions
Issue 1: Lagging Consumers
One frequent issue occurs when a consumer is not processing messages in real-time, leading to what is known as 'consumer lag'. This can result from slow processing, network outages, or misconfiguration.
Solution:
-
Monitor Consumer Lag: Use tools like Kakfa's built-in metrics or third-party monitoring solutions (like Prometheus) to monitor consumer lag.
🔧snippet.shkafka-consumer-groups.sh --bootstrap-server localhost:9092 --describe --group your-consumer-group
-
Check Processing Logic: Ensure that the processing logic is efficient. Optimize by breaking down tasks and using multi-threading where applicable.
-
Scaling Up: If lag continues, consider adding more consumers to the consumer group to share the load.
Issue 2: Offset Reset
Consumers might face a situation where offsets are reset unintentionally, leading to data duplication or skipping messages.
Solution:
-
Manual Offset Management: Use manual offset commits to regain control over which messages have been processed.
☕snippet.javaconsumer.commitSync(); // Ensures offsets are committed only after processing is complete
-
Check Configuration: Verify
auto.offset.reset
properties. It can be set to:- earliest: From the start of the log.
- latest: From the end of the log.
Example consumer configuration:
📄snippet.txtprops.put("auto.offset.reset", "earliest");
-
Use Consumer Rewind: If offsets are accidentally reset, you can use the
seek()
method to manually rewind the consumer to a specific offset.☕snippet.javaconsumer.seek(new TopicPartition("your-topic", partition), offset);
Issue 3: Uncommitted Offsets
Sometimes consumers might not commit offsets due to exceptions or other issues, resulting in re-processing some messages.
Solution:
-
Error Handling: Wrap message processing in try-catch blocks to ensure that exceptions are handled gracefully and offsets committed only upon successful processing.
☕snippet.javatry { ConsumerRecords<String, String> records = consumer.poll(Duration.ofMillis(100)); for (ConsumerRecord<String, String> record : records) { // Your processing logic // Commit only after successful processing consumer.commitSync(); } } catch (Exception e) { // Log the error }
-
Use idempotent producers: Another effective strategy to handle duplicate messages is to use idempotent producers, ensuring that reprocessing does not lead to unintended side effects.
Issue 4: Partition Assignment Issues
If consumers are not getting messages from assigned partitions or certain partitions appear empty, it may arise due to partition assignment problems.
Solution:
-
Check Consumer Group Coordinator: If a consumer fails, it may not join the group seamlessly. Use the following command to check the group’s status.
🔧snippet.shkafka-consumer-groups.sh --bootstrap-server localhost:9092 --describe --group your-consumer-group
-
Update Partition Assignment Strategy: If using a custom assignment strategy, ensure it is optimized for your use case. Kafka's default rack-aware strategy usually suffices.
-
Handle Unbalanced Partitions: Make sure partition distribution is balanced among consumers. Consider using
assign()
oversubscribe()
for more control over partition assignment.
Monitoring and Logging
Having robust monitoring can preemptively identify offset-related issues. Integrate tools like:
- Kafka Manager: Provides a web UI to monitor Kafka.
- Prometheus & Grafana: Powerful tools for real-time alerting and visualization.
- ElasticStack: For logging and monitoring consumer applications.
The Bottom Line
Understanding and troubleshooting Kafka consumer offset issues can significantly enhance your applications' performance and reliability. Ensure to keep your configurations optimal and monitor your consumers actively. With effective handling of offsets and a robust approach to error management, you'll mitigate many common Kafka pitfalls.
For more detailed insights into Kafka's inner workings and practical applications, check out Kafka’s official documentation and consider expanding your knowledge with more tutorials.
By implementing these strategies, you can maintain a highly effective Kafka consumer experience, reducing downtime and enhancing data processing efficiency. Happy coding!