Overcoming Microservices Challenges with Project Valhalla
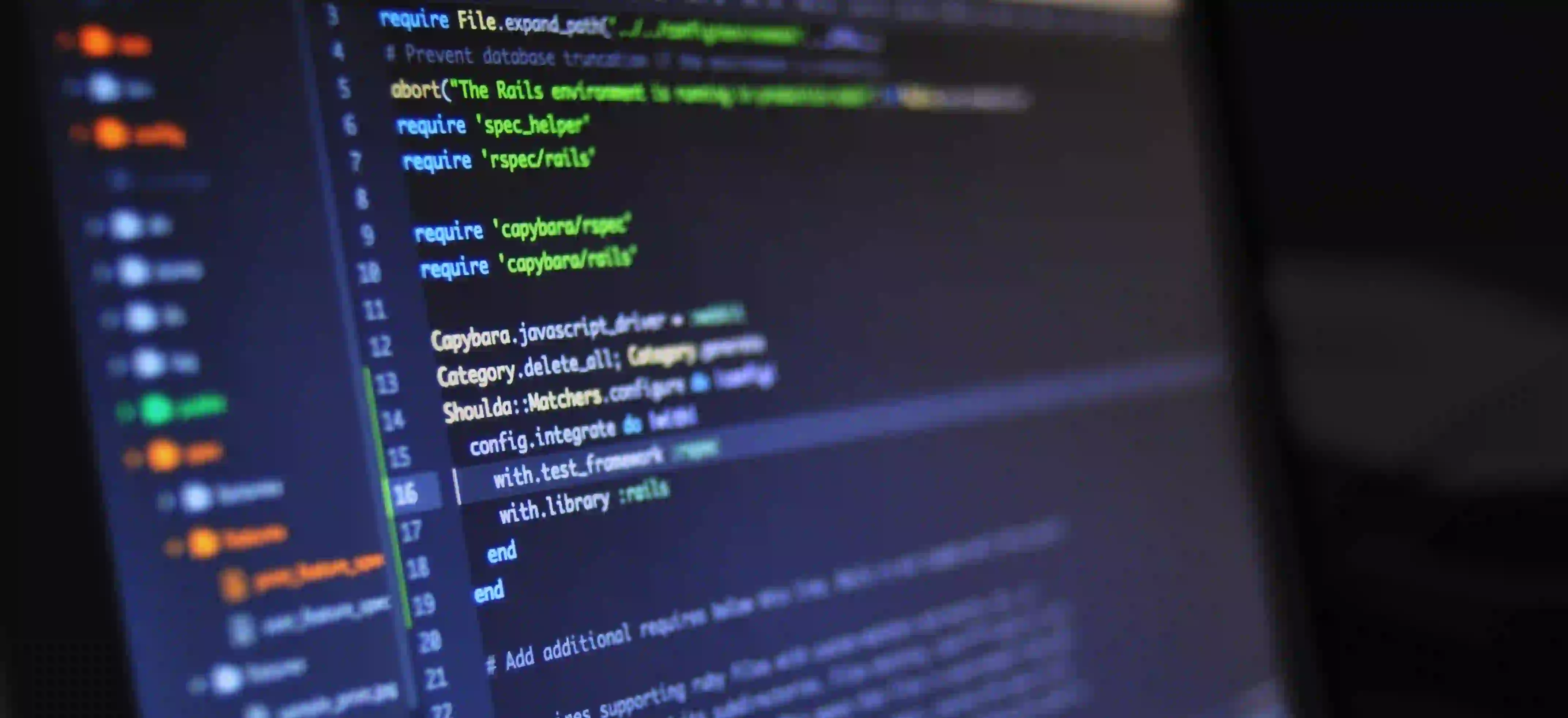
Overcoming Microservices Challenges with Project Valhalla
Microservices architecture has transformed the way applications are built and scaled. However, with this transformation comes a set of unique challenges. In this blog post, we'll explore how Project Valhalla, the ongoing effort within the Java community to bring more flexibility and performance to Java, can help overcome these challenges. We'll dive deep into the key features of Project Valhalla and provide practical examples that can be applied to real-world microservices scenarios.
What are Microservices?
Microservices architecture is an approach to software development where an application is constructed as a collection of loosely coupled, independently deployable services. Each service focuses on a specific business capability, which allows for faster development, easy scaling, and improved resilience. However, this architecture isn't without its drawbacks.
Challenges Facing Microservices
-
Complexity: Managing multiple services can lead to increased complexity in deployment, communication, and data management.
-
Latency and Performance: Each microservice communicates over the network, introducing latency compared to monolithic applications.
-
Data Consistency: Ensuring data consistency across services can be tricky, especially in distributed systems.
-
Deployment and Monitoring: Deploying multiple services requires robust CI/CD pipelines, and monitoring these services efficiently can be a daunting task.
Enter Project Valhalla
Project Valhalla aims to bring support for value types to Java, enabling developers to create more performant and flexible data structures. With these enhancements, Java can better address the challenges associated with microservices.
Key Features of Project Valhalla
-
Value Types: Allows the definition of lightweight data structures that can be manipulated in a more performance-efficient manner.
-
Specialization: The JIT (Just-In-Time) compiler can optimize code based on the types involved, leading to increased performance.
-
Generics Overhaul: Enhancements in generics will allow for more flexibility and robustness in code design.
How Project Valhalla Solves Microservices Problems
1. Reduced Complexity with Value Types
Microservices often require data structures that are shared across services. Using value types can help simplify the models we work with in these services.
@ValueType
public class Money {
private final int amount;
private final String currency;
// Constructor
public Money(int amount, String currency) {
this.amount = amount;
this.currency = currency;
}
// Adding two Money instances
public Money add(Money other) {
if (!this.currency.equals(other.currency)) {
throw new IllegalArgumentException("Currency mismatch");
}
return new Money(this.amount + other.amount, this.currency);
}
}
In this example, the Money
class is defined as a value type. Its immutability results in safer code when used across multiple microservices. The add
method ensures that we avoid currency mismatches, simplifying error handling.
2. Enhanced Performance
In a microservice environment, performance can be a significant concern, especially when services communicate over the network. Project Valhalla allows for specialization of types, ensuring that you get better JIT optimization.
Consider an application where you're handling various data transformations in a microservice:
public <T> T processData(T data) {
// Some processing logic
return transformData(data);
}
public Integer transformData(Integer number) {
return number * 2;
}
public String transformData(String text) {
return text.toUpperCase();
}
Using specialized generics with Project Valhalla, the JIT compiler can optimize these method calls based on the actual type being processed, eliminating the overhead associated with polymorphism.
3. Better Data Consistency
Microservices often interact with databases, and maintaining data consistency can be challenging. By using value types, we can enforce stricter type checks and create more predictable data flows.
Example: Redux Pattern in Microservices
Imagine a scenario where several services need to work together to modify an order. Using value types, these services can maintain their own state without worrying about external changes.
// Immutable Order as a Value Type
@ValueType
public class Order {
private final String orderId;
private final List<Item> items;
// Constructor and other methods
}
// Service Interaction
public class OrderService {
public Order processOrder(Order order) {
// Process order
return new Order(order.getOrderId(), modifyItems(order.getItems()));
}
}
In this illustration, every service operates with an immutable Order
object. This immutability enhances data consistency, as the original object remains unchanged, preventing accidental modifications between services.
4. Streamlined Deployment and Monitoring
With better performance and reduced complexity, deploying and monitoring microservices can also be simplified. The uniformity provided by value types sets clear contracts around data structures—making them easier to monitor.
For instance, using standardized value types across services can streamline logging and tracing:
public void logOrder(Order order) {
System.out.println("Processing order: " + order.getOrderId());
// Further logging logic...
}
With consistent logging structures, tools like Zipkin or Prometheus can more easily track service performance, detect anomalies, and provide insights into system operations.
Closing Remarks
Microservices architecture offers immense benefits in terms of scalability and flexibility. Nevertheless, it introduces notable challenges related to complexity, performance, data consistency, and deployment. Project Valhalla presents a robust solution for these challenges by introducing advanced value types, specialization, and a comprehensive overhaul of generics.
As Java developers, embracing Project Valhalla means not only tackling existing pain points but also allowing us to write simpler, more efficient code. By leveraging these advancements, teams can build microservices that are easier to manage, faster to deploy, and more resilient to failure.
For further reading on Project Valhalla and its ongoing developments, visit the OpenJDK Project Valhalla page. Embrace these powerful tools and elevate your microservices architecture to new heights!
References
- Microservices Architecture Overview
- OpenJDK Project Valhalla
- Zipkin - Distributed tracing
- Prometheus - Monitoring system
By understanding and implementing these practices in your Java microservices, you will be prepared to face the challenges head-on, ensuring smooth performance and a robust application architecture.