Overcoming Latency Issues in Multi-DC Cassandra Setups
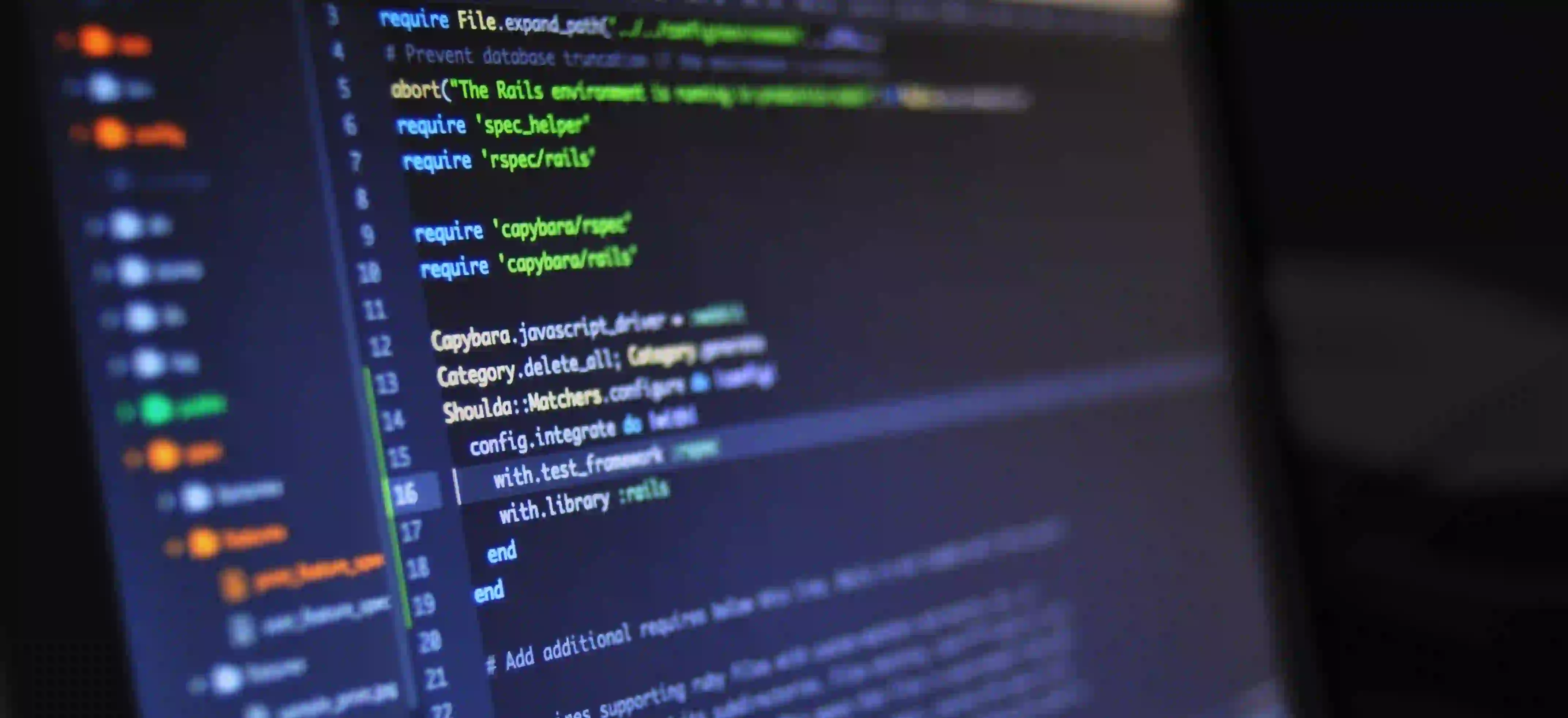
Overcoming Latency Issues in Multi-DC Cassandra Setups
Cassandra is a powerful distributed NoSQL database renowned for its scalability and high availability. But when configuring it for multi-data center (DC) deployments, latency issues can arise that challenge application performance and user experience. This blog post will dive into the common sources of latency issues in multi-DC Cassandra setups and explore strategies to overcome them.
Understanding Multi-DC Deployments
In a multi-DC deployment, Cassandra clusters span multiple geographical locations. This architecture helps ensure data redundancy and availability in the face of regional outages. However, it inherently introduces latency due to the increased distance between data centers.
Key Benefits of Multi-DC Configurations
- High Availability: Availability during outages in one data center ensures constant access to data.
- Disaster Recovery: In the event of a catastrophic failure, data can be retrieved from another data center.
- Localized Reads/Writes: Users can connect to the nearest data center for improved performance.
- Data Locality: Data can be stored in a region closer to specific user bases, enhancing response times.
Common Sources of Latency
Before we can effectively tackle latency, understanding its sources is critical. Here are a few major culprits:
- Network Latency: The most apparent factor is the physical distance between data centers and the associated network latency.
- Write and Read Consistency Levels: High consistency levels requiring coordination across data centers can introduce delay.
- Replica Placement Strategy: Poor choice of replica placement can lead to inefficient access patterns.
- Load Balancing: Unbalanced load among nodes can lead to some nodes being overwhelmed while others remain idle.
Strategies to Overcome Latency
1. Evaluate and Optimize Consistency Levels
Cassandra allows for adjustable consistency levels that determine how many replicas must respond for a read or write operation. For multi-DC setups, consider the following common consistency levels:
- One: Only one replica must acknowledge the read or write. This level provides the fastest response time but the least consistency.
- Local ONE: This level ensures that only a replica in the local data center must respond. It mitigates cross-DC latency for reads and writes.
- Local Quorum: Requires a majority of replicas in the local data center to acknowledge, striking a balance between speed and consistency.
Example:
// Set consistency level to Local ONE for fast reads
Statement statement = new SimpleStatement("SELECT * FROM your_table")
.setConsistencyLevel(ConsistencyLevel.LOCAL_ONE);
This approach minimizes cross-data-center round trips and offers quicker read responses.
2. Optimize Replica Placement Strategy
The replica placement strategy you choose directly impacts how data distribution occurs across the nodes in your cluster.
- NetworkTopologyStrategy: This strategy is recommended for multi-DC setups. It allows you to specify replica counts for each data center.
Example:
# cassandra.yaml configuration
replication_strategy: NetworkTopologyStrategy
replication_factor:
<dc1>: 3
<dc2>: 3
Choose appropriate replication factors for your use case, ensuring enhanced availability without data staleness.
3. Use Data Locality for Reads and Writes
Leverage the Local Data Center option in your client application to route requests to the closest data center, reducing latency. Many drivers enable this feature easily.
Example in Java using DataStax Driver:
Cluster cluster = Cluster.builder()
.addContactPoint("your_dc_ip")
.withLocalDatacenter("your_local_dc") // Specific to local DC
.build();
This change allows the application to always prefer servicing requests from the local data center, enhancing performance significantly.
4. Enable Client-Side Load Balancing
Implement client-side load balancing to distribute requests efficiently across nodes. By doing so, you can avoid overloading any single node, creating a more balanced load across your multi-DC environment.
Example:
LoadBalancingPolicy policy = new DCAwareRoundRobinPolicy.Builder()
.withLocalDc("your_local_dc") // Use the local DC first
.build();
Cluster cluster = Cluster.builder()
.addContactPoint("your_dc_ip")
.withLoadBalancingPolicy(policy)
.build();
Incorporating a solid load balancing policy is essential for keeping response times in check.
5. Monitor and Optimize Network Performance
Ensure that your network infrastructure is robust and capable of handling cross-DC traffic. Regular monitoring tools and metrics can help identify bottlenecks. Use tools like Prometheus or Grafana to visualize performance and detect issues early.
Key Metrics:
- Latency for cross-DC calls
- Read and write performance across all data centers
- Load distribution among nodes
6. Tune JVM and Node Settings
Ensure your JVM and Cassandra are properly tuned. Parameters such as heap size, garbage collection strategies, and configured thread pools could significantly impact performance.
- Heap Size: Always align your heap size with data requirements. A good rule of thumb is 50% of your total available RAM, with a maximum of 32GB to avoid performance penalties in garbage collection.
7. Asynchronous Processing
Where possible, implement asynchronous processing for write operations. This can help the application continue functioning while waiting for write acknowledgements, ultimately improving user experience.
Example:
// Asynchronous insert in Cassandra
session.executeAsync(new SimpleStatement("INSERT INTO your_table (id, data) VALUES (?, ?)", id, data));
This approach can vastly improve performance, especially under high load.
To Wrap Things Up
While multi-DC setups amplify the resilience and availability of Cassandra databases, they also introduce unique latency challenges. By following the strategies outlined above—evaluating consistency levels, choosing the right replica placement strategy, enhancing data locality, employing effective load balancing, monitoring network performance, tuning settings, and embracing asynchronous processing—you can mitigate latency issues effectively.
For further reading, consider checking out the following resources:
- Apache Cassandra Documentation
- Cassandra Client Drivers
- Common Cassandra Performance Tuning Practices
Now go forth and optimize your multi-DC Cassandra deployment, ensuring a seamless and responsive experience for users across the globe!