Overcoming Log Noise: Streamlining Java Log Analysis
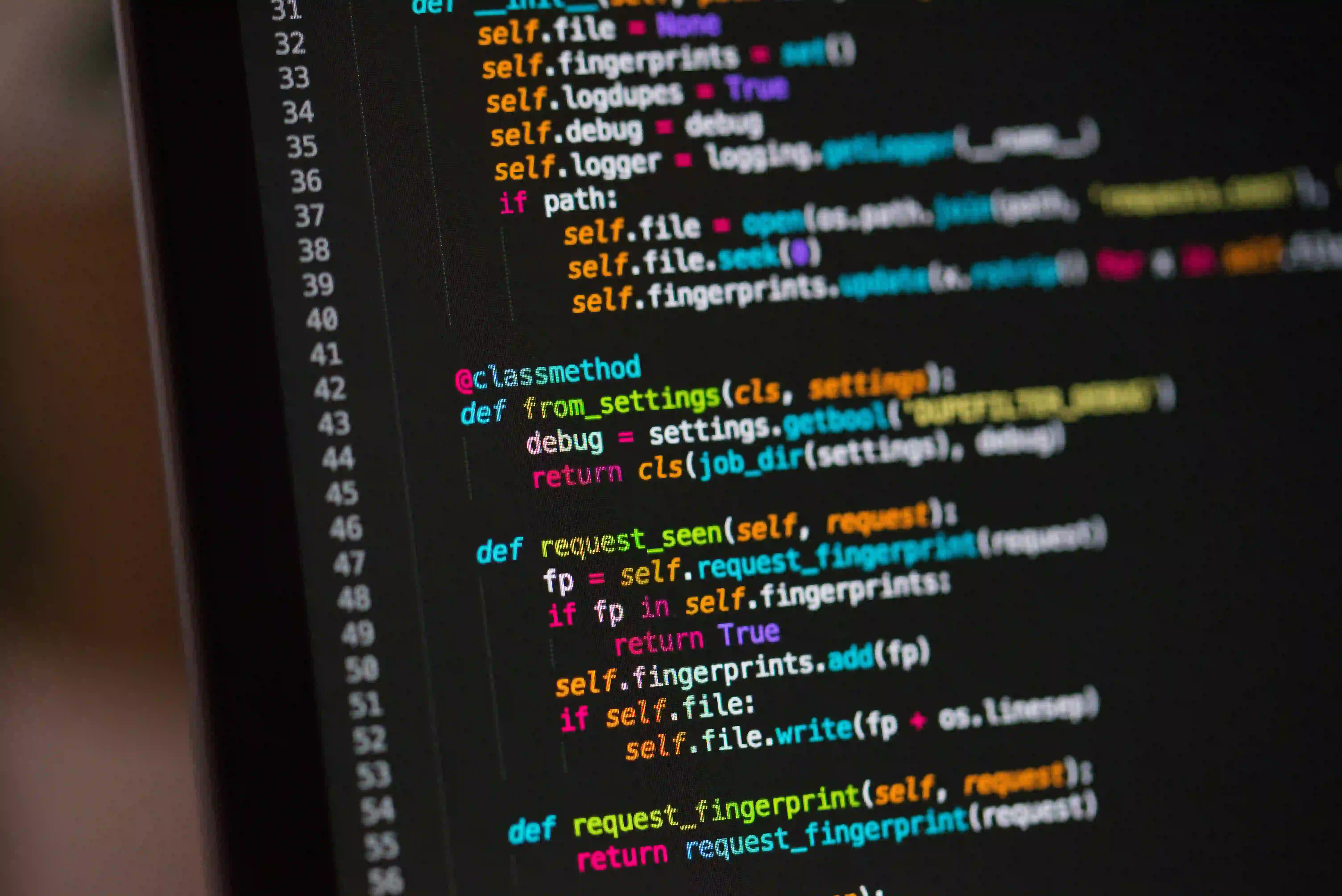
Overcoming Log Noise: Streamlining Java Log Analysis
Logging is an essential aspect of software development, especially when it comes to debugging and monitoring applications. However, in Java applications, logs can quickly become overwhelming due to significant noise. This blog aims to help you streamline Java log analysis by overcoming log noise.
Understanding Log Noise
Before diving deeper, let's define what log noise is. Log noise refers to unnecessary, verbose, or repetitive log entries that can obscure useful information. An abundance of log entries can hinder developers from effectively diagnosing issues, complicating the troubleshooting process.
Unraveling log noise is crucial for enhancing clarity and efficiency during log analysis. By minimizing irrelevant information, developers can quickly pinpoint problems and improve overall system reliability.
The Importance of Effective Logging in Java
Effective logging practices have profound benefits, including:
- Improved Debugging: Clear logs provide immediate insights into application operations.
- Performance Monitoring: Analyzing logs helps identify performance bottlenecks.
- Audit Trails: Logs maintain a history of system activities, useful for compliance.
As a Java developer, understanding how to structure and filter logs will prove pivotal in maintaining efficient software.
Choosing the Right Logging Framework
Java comes equipped with several logging frameworks. Choosing the right one is a fundamental step in minimizing log noise:
- Log4j: A powerful, flexible framework widely used in Java applications. It allows for configuration through XML or property files.
- SLF4J (Simple Logging Facade for Java): Serves as a facade or abstraction for various logging frameworks.
- Java Util Logging (JUL): Provides a basic logging capability.
When selecting a framework, consider performance, ease of integration, and configurability. For our example, we will use Log4j.
Setting Up Log4j
To begin, you first need to add the Log4j dependency to your project. If you're using Maven, add the following to your pom.xml
:
<dependency>
<groupId>org.apache.logging.log4j</groupId>
<artifactId>log4j-core</artifactId>
<version>2.17.1</version>
</dependency>
<dependency>
<groupId>org.apache.logging.log4j</groupId>
<artifactId>log4j-api</artifactId>
<version>2.17.1</version>
</dependency>
Sample Log4j Configuration
Below is an example of a Log4j configuration file (log4j2.xml
) minimalist for cleaner logs.
<?xml version="1.0" encoding="UTF-8"?>
<Configuration status="WARN">
<Appenders>
<Console name="Console" target="SYSTEM_OUT">
<PatternLayout pattern="%d{yyyy-MM-dd HH:mm:ss} %-5p %c{1} - %m%n"/>
</Console>
</Appenders>
<Loggers>
<Root level="info">
<AppenderRef ref="Console"/>
</Root>
</Loggers>
</Configuration>
Why This Configuration?
- Minimalist Layout: The
%d
,%p
,%c
, and%m
placeholders present timestamps, log levels, class names, and messages, respectively. This format ensures logs are easy to read while stripping unnecessary information. - Level Set to Info: By setting the root logger to 'info', we avoid debug and trace-level logs that contribute to noise in production environments.
Logging Best Practices
Once you have configured your logging framework, it's time to establish best practices to enhance clarity while reducing noise.
1. Log at Appropriate Levels
Java logging provides several levels, including DEBUG, INFO, WARN, ERROR, and FATAL. Understanding the hierarchy is crucial.
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
public class LoggingExample {
private static final Logger logger = LogManager.getLogger(LoggingExample.class);
public void performOperation() {
logger.debug("Operation started"); // Debug - detailed information for troubleshooting.
try {
// Perform task...
logger.info("Operation performed successfully"); // Info - high-level information about the process.
} catch (Exception e) {
logger.error("An error occurred: {}", e.getMessage()); // Error - issues that disrupt normal flow.
}
}
}
Why Log at Different Levels?
Logging at appropriate levels offers clarity. Critical information appears clearly while background details remain available during development/debugging.
2. Use Meaningful Messages
The clarity of log messages is paramount. Avoid vague terms and instead provide context.
logger.info("User with ID {} has logged in successfully.", userId);
Why This Matters?
Using meaningful messages helps teams quickly understand application behavior without needing to dig through code or documentation to decipher the logs.
3. Avoid Logging Sensitive Data
In today's security-focused environment, be vigilant about what you log. Avoid logging passwords, personal information, or any sensitive data that could be exploited.
4. Implement Structured Logging
Structured logging involves outputting logs in a structured format (e.g., JSON). This technique aids in filtering and searching logs in log aggregation tools.
logger.info("User login event", Map.of("userId", userId, "timestamp", System.currentTimeMillis()));
Why Structured Logging?
The structured format enhances the capability for parsing, especially in systems using tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Splunk, where having structured logs improves the indexing and searching process.
5. Utilize Filters
Log filters enable you to refine what gets logged based on specified criteria. You can filter logs dynamically to eliminate noise.
<Logger name="com.yourpackage" level="warn" additivity="false">
<AppenderRef ref="Console"/>
</Logger>
6. Regular Log Cleanup
Logs should be regularly archived or deleted. Unnecessary logs can consume excessive disk space, thus impacting performance and making analysis more cumbersome.
The Last Word
Overcoming log noise is a blend of choosing the right framework, utilizing best practices, and continuously reviewing your logging strategy. By adopting these measures, you'll streamline your Java log analysis and lead your team towards more effective debugging and monitoring processes.
For further reading on best practices in logging and monitoring, check out Log4j Documentation and Structured Logging in Java.
Implement these strategies today, and transform log noise into actionable insights!