Conquering Lazy Image Loading: Boost Your App's Performance!
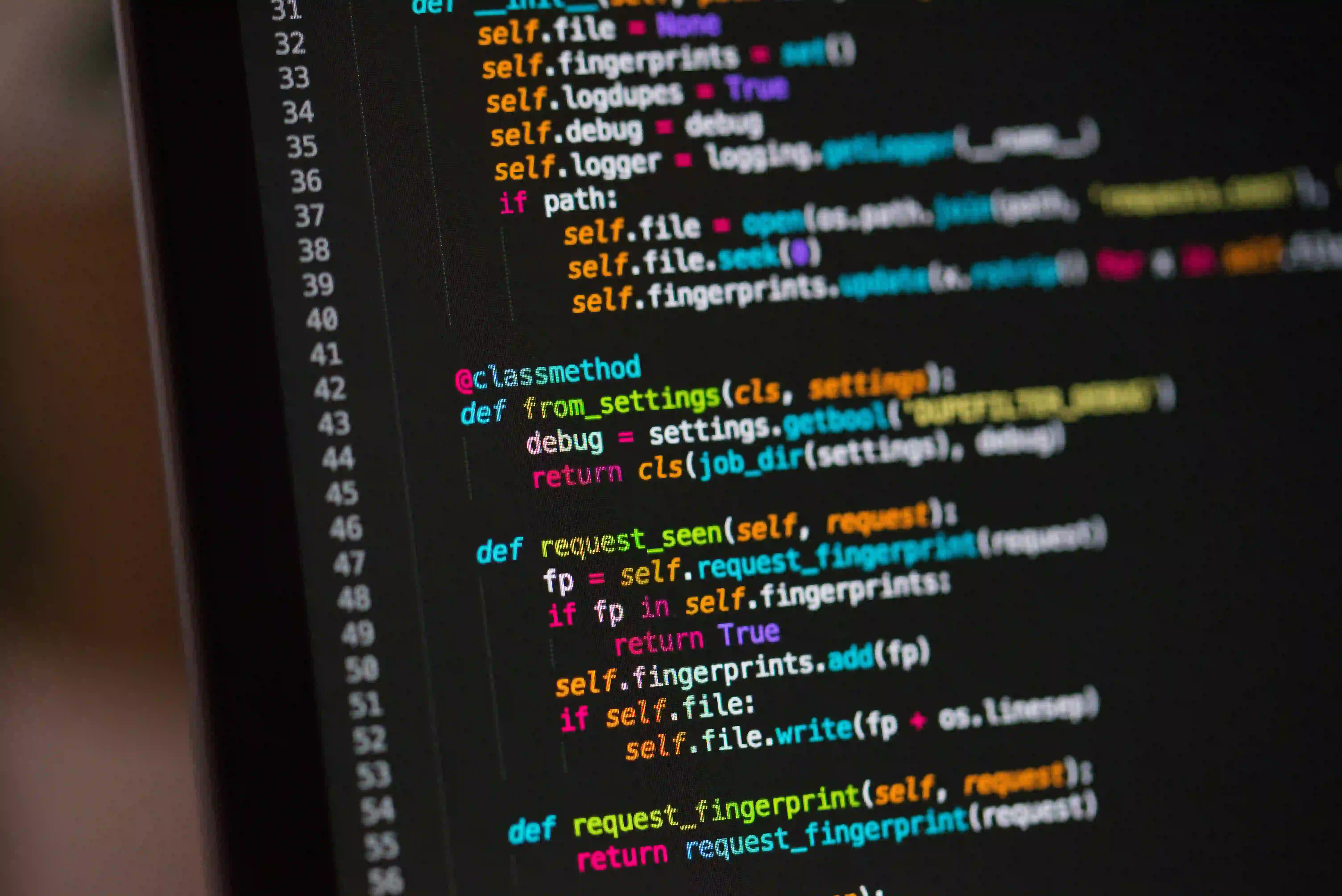
Conquering Lazy Image Loading: Boost Your App's Performance!
In web and mobile applications, one of the key factors affecting user experience is how quickly images load. High-resolution images can lead to significant performance slowdowns. Fortunately, lazy loading is an effective strategy to improve performance by loading images only when needed, such as when they enter the viewport. In this blog post, we will explore the concept of lazy image loading, its benefits, the implementation process, and how it can dramatically boost your application's performance.
What is Lazy Loading?
Lazy loading is a design pattern that postpones loading of non-essential resources at the point of page load. Instead, these resources are loaded only when they are actually needed. In the context of images, this translates to not loading any images until they are about to be visible on the user's screen.
Benefits of Lazy Loading
-
Improved Performance: By deferring image loading, you reduce the initial page load time. You improve user experience significantly, especially for mobile users on slower networks.
-
Reduced Bandwidth Usage: Loading fewer images means less data consumption, which is crucial for users with limited data plans.
-
Enhanced SEO: Fast-loading pages are favored by search engines, which can lead to higher rankings. By employing lazy loading, you can minimize loading times, enhancing SEO efforts.
-
Better User Engagement: Faster load times lead to better engagement, as users are less likely to abandon your site or app due to slow performance.
Implementation Steps in Java
Lazy loading can be implemented in various programming languages, including Java. Below, we will go through how to implement lazy loading in a Java-based application. We will primarily focus on web applications using Servlets and JSPs.
Step 1: Setup Your Project
You'll want to set up a Maven project if you haven't done so. In this step, create a simple web application structure. Make your pom.xml
file include dependencies for Servlet API if not already present:
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>4.0.1</version>
<scope>provided</scope>
</dependency>
Step 2: Create a Sample JSP Page
Create a JSP page that will serve content. For lazy loading purposes, we will include data-src
attributes in our image tags that point to where the images will be fetched when they come into view.
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>Lazy Load Images</title>
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
<script>
$(document).ready(function() {
$('img[data-src]').each(function() {
var img = $(this);
img.attr('src', img.data('src'));
});
});
</script>
</head>
<body>
<h1>Welcome to Our Image Gallery</h1>
<div>
<img data-src="path/to/first_image.jpg" alt="First Image" width="300" height="200">
<img data-src="path/to/second_image.jpg" alt="Second Image" width="300" height="200">
<img data-src="path/to/third_image.jpg" alt="Third Image" width="300" height="200">
<img data-src="path/to/fourth_image.jpg" alt="Fourth Image" width="300" height="200">
</div>
</body>
</html>
Code Explanation
-
HTML Structure: The
img
tags are equipped with a custom attributedata-src
instead of the usualsrc
. This prevents the browser from loading the images immediately. -
jQuery Script: The jQuery code runs when the document is ready and changes the
src
attribute of each image to the URL specified indata-src
. This effectively loads the image when the page is displayed.
Step 3: Use Intersection Observer for Improved Performance
While the aforementioned approach is easy and efficient for small projects, using the Intersection Observer API is much more performance-friendly. This API allows you to defer loading images until they are in or about to enter the viewport.
Here’s how you can implement it:
document.addEventListener('DOMContentLoaded', function() {
let lazyImages = document.querySelectorAll('img[data-src]');
const imgObserver = new IntersectionObserver((entries, observer) => {
entries.forEach(entry => {
if (entry.isIntersecting) {
const img = entry.target;
img.src = img.dataset.src;
imgObserver.unobserve(img);
}
});
});
lazyImages.forEach(image => {
imgObserver.observe(image);
});
});
Code Explanation
-
IntersectionObserver: This monitors the entry of images into the viewport. It’s highly efficient for lazy loading as it allows for batch processing of image loads, rather than initiating a load every time an image is in view.
-
Performance Benefits: By only loading images when they are close to being seen, you save bandwidth and reduce initial load times, enhancing user experience significantly.
Key Takeaways
In today’s fast-paced digital world, the performance of your web applications is paramount. Implementing lazy image loading in your Java applications can drastically improve load times, reduce bandwidth usage, and elevate user engagement.
Using simple approaches with custom attributes and jQuery can help implement the basic concept, whereas adopting the Intersection Observer API can provide even better performance for modern applications.
For further reading, check out the following articles that delve deeper into optimizing web performance and techniques like lazy loading:
- Web Performance Optimization by Google Developers
- Lazy loading images - A guide from Google on the best practices and implementation.
By conquering lazy image loading, you're not only enhancing user experience but also paving the way for better performance metrics which can translate into higher search engine rankings. Start integrating lazy loading and watch your app's performance soar!