Mastering JCommando: Overcoming Common Command Issues
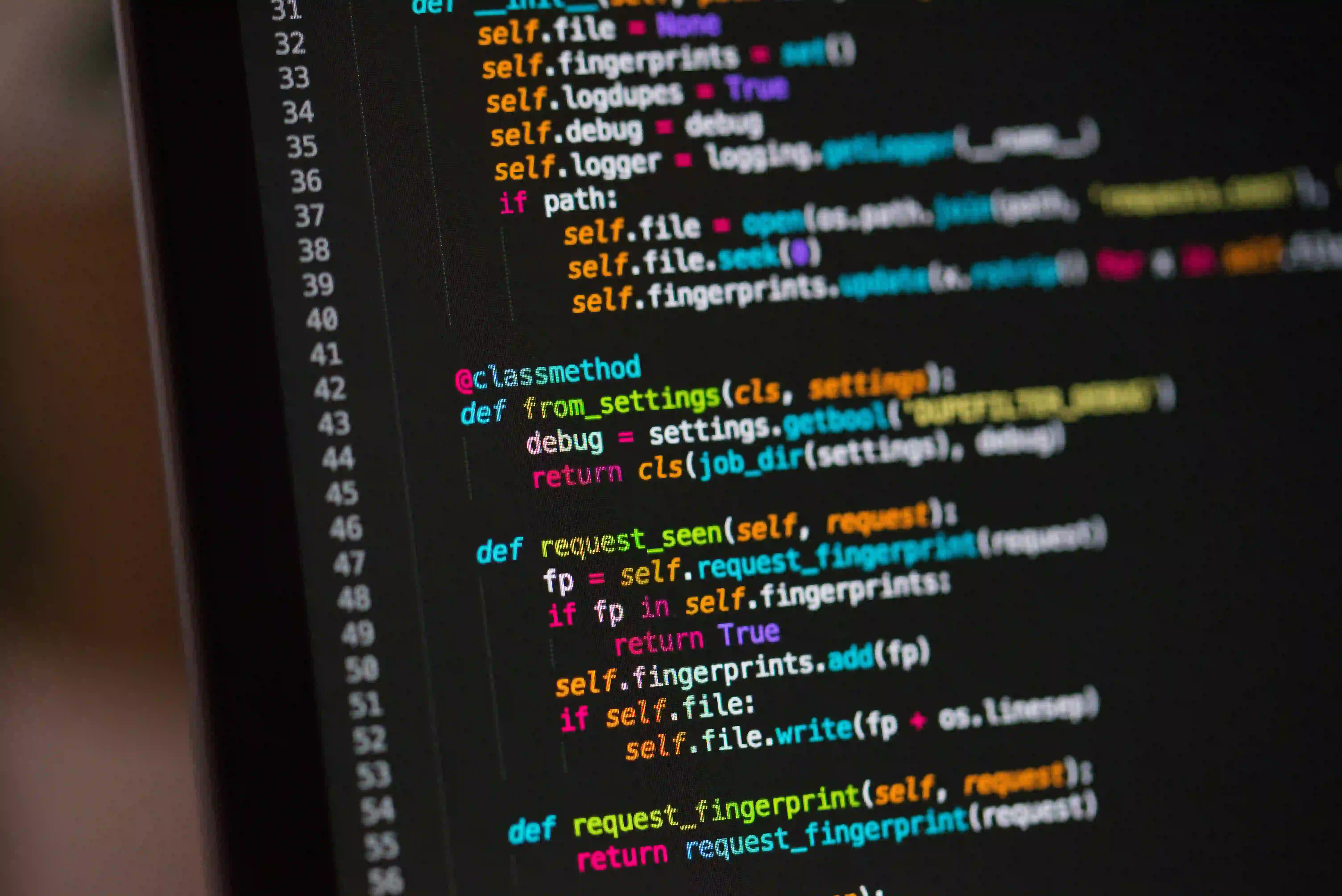
Mastering JCommando: Overcoming Common Command Issues
In the world of modern Java development, command-line utilities play a crucial role in creating flexible and efficient applications. One such utility that has gained traction recently is JCommando, a lightweight command-line argument parser for Java applications. While JCommando is relatively easy to use, developers can still encounter common issues that may hinder their productivity. This blog post aims to address those common command issues and provide Java developers with effective solutions.
What is JCommando?
JCommando simplifies the process of parsing command-line arguments in Java applications. Its design allows for easy integration, minimal overhead, and clear syntax, making it an attractive option for developers. By leveraging annotations, developers can effortlessly define commands, options, and arguments.
To get started with JCommando, first include it in your project. If you're using Maven, add the following dependency to your pom.xml
:
<dependency>
<groupId>com.github.JCommando</groupId>
<artifactId>JCommando</artifactId>
<version>1.0.4</version>
</dependency>
Why Use JCommando?
- Simplicity: Its API is straightforward, making integration quick.
- Rich Feature Set: Supports various types of command options.
- Documentation and Examples: Comprehensive resources are available to get you started effectively.
For more information on how to get started, visit the JCommando GitHub page.
Common Command Issues in JCommando
Although JCommando works seamlessly in many cases, it is not uncommon for developers to face certain issues while implementing it. Below, we will discuss some of the most frequent problems and their corresponding solutions.
1. Parsing Errors: Check Your Annotations
One common issue developers face is misconfigured annotations. When commands and options are not correctly annotated, JCommando will fail to parse the input, leading to runtime errors.
Solution: Validate Annotations
Ensure that every command and option is properly defined. For example:
import jcommando.Command;
import jcommando.Option;
public class MyApplication {
@Command(description = "Greets the user")
public void greet(@Option(shortName = 'n', description = "User's name") String name) {
System.out.println("Hello, " + name + "!");
}
}
In this example, ensure that @Command
and @Option
are imported correctly from the JCommando library. A simple oversight can lead to frustrating debug sessions.
2. Missing Required Options
Another issue arises when a required option is not provided, causing the application to fail.
Solution: Use Built-in Validations
You can set options as required, instructing JCommando to check for their presence. For example:
@Option(required = true, shortName = 'f', description = "Input file")
private String inputFile;
By marking the inputFile
option as required
, JCommando will prompt the user if it is missing.
3. Confusion Between Short and Long Options
Using both short and long options can lead to clashes or confusion among developers and users.
Solution: Be Consistent
Make sure to maintain a consistent style across your commands. For instance:
@Option(shortName = 'v', longName = "verbose", description = "Enable verbose output")
private boolean verboseMode;
Always document your commands to minimize confusion. Refer to clear guidelines in your project documentation for using command-line options.
4. Order of Arguments Matters
A common misconception is that the order of command-line arguments doesn't matter. With JCommando, the order can affect how the parser interprets the input.
Solution: Document Argument Order
Clearly document the expected argument order. For example:
@Command(description = "Start the application")
public void start(@Option(shortName = 'm', description = "Mode to run") String mode,
String additionalArgument) {
// additionalArgument should always come after '-m'
}
Educate users on how to input commands correctly, which will facilitate a smoother user experience.
5. Handling Invalid Input Gracefully
What happens when a user enters an invalid option or argument? It is vital to handle these situations gracefully to prevent unexpected crashes.
Solution: Catch Exceptions
Utilize exception handling around your command-run methods. Here is an example:
try {
jCommando.run(args);
} catch (IllegalArgumentException e) {
System.err.println("Error: " + e.getMessage());
System.err.println("Please refer to the documentation for correct usage.");
}
By providing feedback on the error, you can guide users back on track.
Best Practices for Using JCommando
To enhance your experience with JCommando, consider the following best practices:
-
Write Comprehensive Help Messages: Make sure that the help output is informative. This encourages users to seek assistance when needed.
-
Version Control for Command Parsing: If your application changes regularly, version your commands so that users are aware of updates and modifications.
-
Unit Testing Your Commands: Implement unit tests to verify that your commands are behaving as expected. This will help catch potential errors before they reach production.
-
Use Default Values: Provide sensible default values for optional parameters to reduce user input burden.
-
Encourage Feedback: Involve your user base in providing feedback on command interfaces. This enables continuous improvement.
Bringing It All Together
Mastering JCommando can significantly enhance your command-line tool's user experience. By understanding its common pitfalls and applying the solutions provided, you will empower your Java applications to be more efficient and user-friendly. Remember that the goal is not just to make things work but to create a smooth user experience.
For more advanced readings, check out this comprehensive guide on Java command-line interfaces that dives deeper into strategies and methodologies for command-line handling.
With the practices mentioned above, you'll be on your way to conquering JCommando and enjoying the flexibility it offers.