Overcoming Common Pitfalls in Library Development
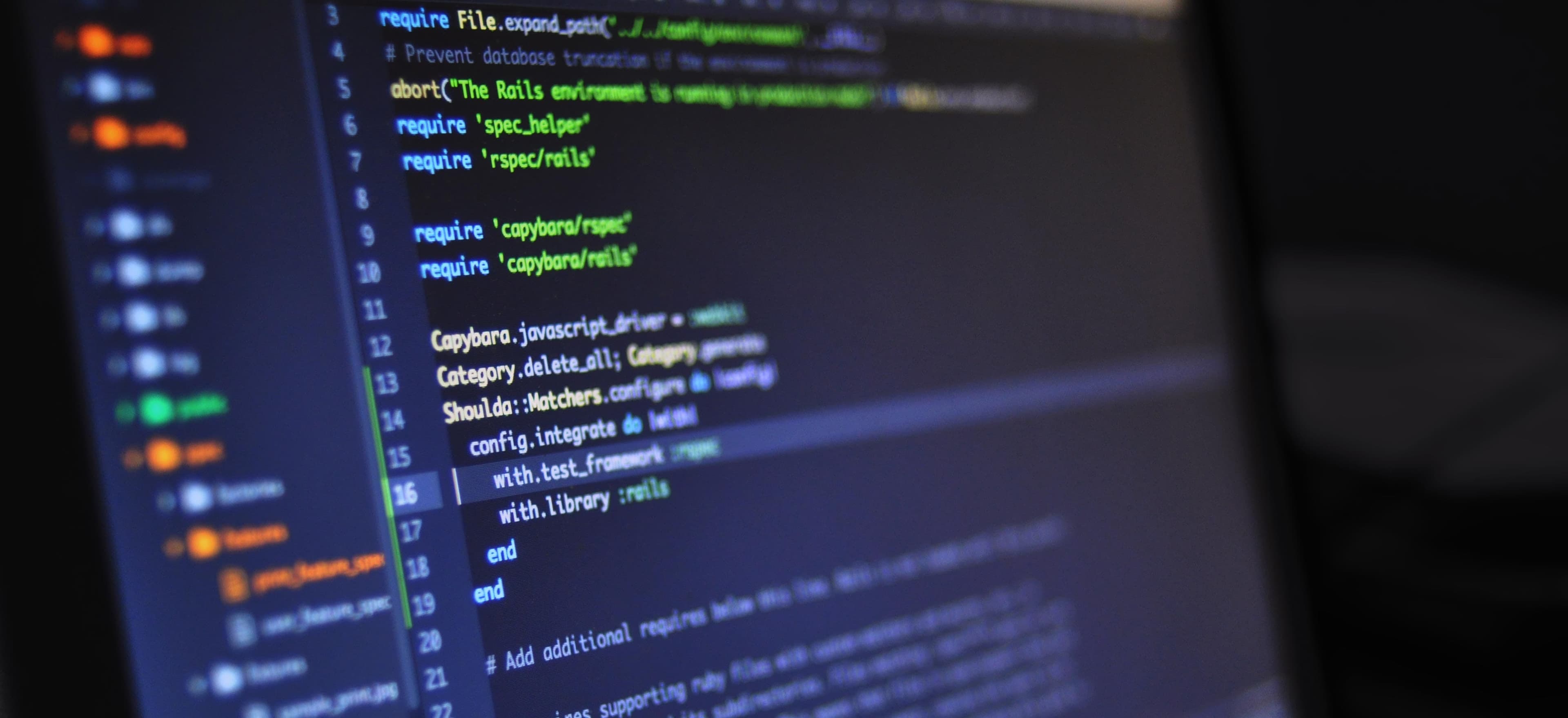
- Published on
Overcoming Common Pitfalls in Library Development
In the world of software development, libraries play an instrumental role. They encapsulate functionality, promote code reuse, and enable developers to build applications more efficiently. However, library development is not without its challenges. In this blog post, we'll explore common pitfalls in library development and provide practical solutions to help you navigate these obstacles effectively.
Understanding Library Development
Before diving into specific pitfalls, it's essential to understand what a library is in the context of software development. A library is a set of pre-written code that developers can use to optimize their applications. It can define functions, classes, variables, and more, which can be reused across different programs. Libraries can significantly reduce development time and enhance code quality.
Common Pitfalls in Library Development
1. Lack of Documentation
One of the most significant pitfalls in library development is the lack of proper documentation. Effective documentation not only helps users understand how to implement the library but also reduces the number of issues and questions they may have.
Solution: Invest time in creating comprehensive documentation. This should include:
- Installation Instructions: Clear steps on how to integrate the library into a project.
- Usage Examples: Code snippets demonstrating how to use the library's features.
- API References: Detailed explanations of the functions and classes provided by the library.
Example:
# MyLibrary
## Installation
To install MyLibrary, run:
```bash
pip install mylibrary
Example Usage
from mylibrary import MyFunction
result = MyFunction(arg1, arg2)
print(result)
### 2. Inconsistent API Design
Another common issue is an inconsistent API design. Users can become confused or frustrated when different parts of the library adhere to various design principles.
**Solution**: Follow a consistent style guide. Determine naming conventions, parameter types, and return values and stick to them throughout the library. Good practices can be found in [Google's Python Style Guide](https://google.github.io/styleguide/pyguide.html).
**Example**:
If you decide on camelCase for function names, ensure all function names follow this convention:
```python
# Inconsistent naming
def my_function(): pass
def AnotherFunction(): pass
# Consistent naming
def myFunction(): pass
def anotherFunction(): pass
3. Ignoring Error Handling
Many developers overlook error handling in their libraries, leading to potential crashes or unexpected behavior in applications that use them.
Solution: Ensure that your library anticipates possible errors and exceptions. Always validate inputs and provide informative error messages.
Example:
def divide(a, b):
if b == 0:
raise ValueError("The denominator cannot be zero.")
return a / b
In this example, we validate the denominator to prevent division by zero, providing essential feedback to the user when an error occurs.
4. Insufficient Testing
Testing is fundamental to any software development process. Libraries, in particular, must be robust and well-tested, given that other applications rely on them.
Solution: Implement a thorough testing strategy using frameworks like JUnit (for Java) or PyTest (for Python). Aim for unit tests, integration tests, and end-to-end tests to ensure all parts of the library function correctly.
Example (Python):
import pytest
from mylibrary import MyFunction
def test_my_function():
assert MyFunction(1, 2) == 3
assert MyFunction(-1, -1) == -2
5. Not Considering Performance
Performance is crucial, especially for libraries that will be used in a variety of applications. Neglecting performance can lead to slow applications and user dissatisfaction.
Solution: Profile your library's performance using tools like VisualVM for Java or cProfile for Python. Optimize algorithms where necessary, and avoid unnecessary computations.
Example:
# Inefficient
def get_even_numbers(numbers):
return [num for num in numbers if num % 2 == 0]
# Improved
def get_even_numbers(numbers):
return list(filter(lambda x: x % 2 == 0, numbers))
# Including performance testing
import time
start_time = time.time()
get_even_numbers(range(1, 1000000))
print("Execution time:", time.time() - start_time)
6. Failing to Keep Dependencies Updated
Libraries often interact with other packages or libraries. Failing to keep these dependencies updated can lead to security vulnerabilities and compatibility issues.
Solution: Regularly check for updates to the dependencies your library relies on. Tools like Dependabot can automate this process for you.
7. Neglecting User Feedback
Many developers develop in a vacuum, forgetting to solicit feedback from actual users of their libraries. Failing to do so can lead to poor user experience and the potential loss of users.
Solution: Create a feedback loop. Encourage developers to recommend features, report bugs, and share their experiences. Use platforms like GitHub Issues to manage this feedback.
8. Ignoring Versioning
Versioning is vital for any library's evolution. Many developers fail to employ a clear versioning strategy, which can make it hard for users to understand the changes.
Solution: Follow Semantic Versioning (SemVer) principles. This way, users can quickly identify breaking changes, new features, or bug fixes.
Example:
- Major version: Indicates breaking changes (e.g., 1.0.0 to 2.0.0)
- Minor version: Backward-compatible functionality (e.g., 1.0.0 to 1.1.0)
- Patch version: Backward-compatible bug fixes (e.g., 1.0.0 to 1.0.1)
A Final Look
Library development offers exciting possibilities, but it also comes with its challenges. By being aware of potential pitfalls and implementing the solutions outlined above, developers can create libraries that are not only robust and efficient but also user-friendly. The benefits of sound library development are vast: they lead to better code quality, happier users, and a flourishing development community.
To dive deeper into best practices for library development, consider exploring resources like The Twelve-Factor App and Clean Code.
By keeping these strategies in mind, you will not only avoid common pitfalls but also contribute positively to the software development landscape. Happy coding!