Managing Kubernetes ConfigMaps in Java Applications Effectively
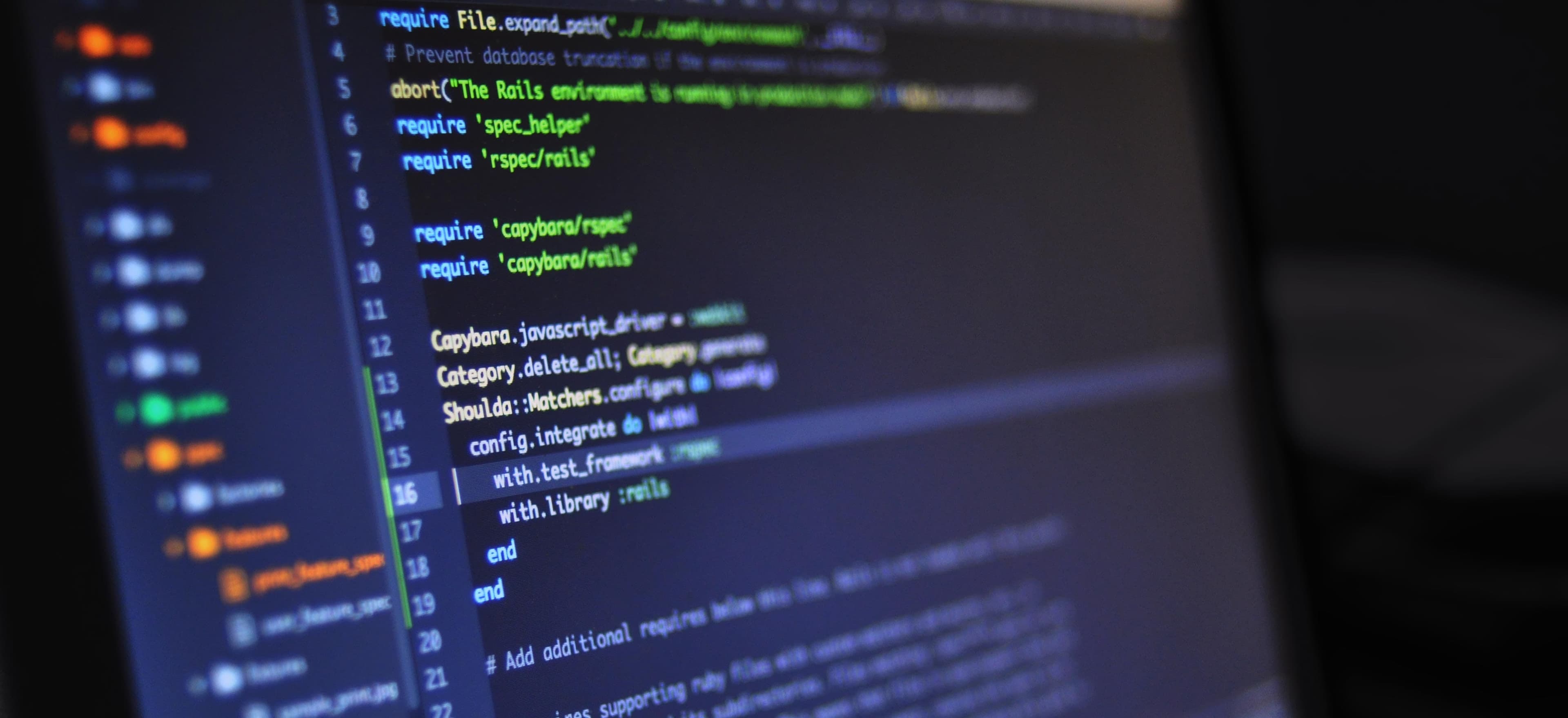
- Published on
Managing Kubernetes ConfigMaps in Java Applications Effectively
Kubernetes has taken the IT world by storm, and for good reason. It provides robust frameworks for deploying, scaling, and managing containerized applications. Among the various components in Kubernetes, ConfigMaps play a pivotal role, especially when it comes to application configuration management. In this blog post, we will delve into the effective management of ConfigMaps in Java applications, covering best practices, code snippets, and real-world scenarios.
What Are ConfigMaps?
Before diving into management strategies, let’s clarify what ConfigMaps are. A ConfigMap in Kubernetes is an API object that lets you store configuration data, such as environment variables, command-line arguments, and configuration files, separately from container images. This externalizes configuration details, which allows for flexibility and ease of updates without needing to rebuild application images.
Why Use ConfigMaps?
-
Decoupling Configuration from Code: By externalizing configuration, you can modify your application's configuration without changing the code or rebuilding Docker images.
-
Version Control: You can keep configurations versioned and apply changes in a controlled manner.
-
Dynamic Updates: ConfigMaps can be updated easily, and depending on your application’s handling, this can allow dynamic reconfiguration.
Creating and Using ConfigMaps
Creating a ConfigMap is a straightforward process. You can do this via the Kubernetes command line, configuration files, or programmatically. Below is a simple example of creating a ConfigMap using a YAML file:
apiVersion: v1
kind: ConfigMap
metadata:
name: my-config
data:
DATABASE_URL: "jdbc:mysql://localhost:3306/mydb"
DATABASE_USER: "dbuser"
DATABASE_PASSWORD: "dbpassword"
Command Line Creation
You can also create a ConfigMap directly using the command line:
kubectl create configmap my-config --from-literal=DATABASE_URL="jdbc:mysql://localhost:3306/mydb" \
--from-literal=DATABASE_USER="dbuser" \
--from-literal=DATABASE_PASSWORD="dbpassword"
By using kubectl
, you can quickly deploy the ConfigMap without needing to write a configuration file.
Accessing ConfigMaps in Java
In a Java Spring Boot application, you can access ConfigMaps as environment variables once you properly link the ConfigMap to your deployment. Here’s an example of how to fetch these properties:
Step 1: Declare Environment Variables
Add the environment variables in your Kubernetes Deployment YAML:
apiVersion: apps/v1
kind: Deployment
metadata:
name: my-app
spec:
replicas: 1
template:
metadata:
labels:
app: my-app
spec:
containers:
- name: my-container
image: my-image
env:
- name: DATABASE_URL
valueFrom:
configMapKeyRef:
name: my-config
key: DATABASE_URL
- name: DATABASE_USER
valueFrom:
configMapKeyRef:
name: my-config
key: DATABASE_USER
- name: DATABASE_PASSWORD
valueFrom:
configMapKeyRef:
name: my-config
key: DATABASE_PASSWORD
Step 2: Access Environment Variables in Java
Now you can access these environment variables in your Spring application:
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Component;
@Component
public class DatabaseConfig {
@Value("${DATABASE_URL}")
private String databaseUrl;
@Value("${DATABASE_USER}")
private String databaseUser;
@Value("${DATABASE_PASSWORD}")
private String databasePassword;
// Getter methods
public String getDatabaseUrl() {
return databaseUrl;
}
public String getDatabaseUser() {
return databaseUser;
}
public String getDatabasePassword() {
return databasePassword;
}
}
This allows you to seamlessly inject your configuration values into your beans, making it easy to manage your application's settings.
Updating ConfigMaps
Given the dynamic nature of most applications, the need to update ConfigMaps is inevitable. You can update a ConfigMap by using either the command line or YAML files.
Using Command Line
You can update a ConfigMap with:
kubectl create configmap my-config --from-literal=DATABASE_URL="jdbc:mysql://newhost:3306/mydb" --dry-run -o yaml | kubectl apply -f -
This command line ensures that the changes are applied without needing to completely recreate the ConfigMap.
Code for Dynamic Reload
If your application needs to reload changes from a ConfigMap dynamically, consider using Spring Cloud Kubernetes, which provides seamless integration and auto-configurations.
Example with Spring Cloud Kubernetes
Here’s how you might implement a refresh listener:
import org.springframework.cloud.context.config.annotation.RefreshScope;
import org.springframework.stereotype.Component;
@RefreshScope
@Component
public class ConfigListener {
// Inject your configuration here
}
By annotating your bean with @RefreshScope
, it will automatically refresh its configuration when changes are detected in the ConfigMap.
Best Practices for ConfigMaps
-
Organize Configurations: Use meaningful names and organize ConfigMaps logically. This makes them easier to manage. For example, you could have
app-logging-config
,app-db-config
, etc. -
Limit Size: Ensure ConfigMaps are not too large. Kubernetes has restrictions on the size of objects, so keep your configurations concise.
-
Use Separate ConfigMaps for Different Profiles: Consider having different ConfigMaps for production, development, and testing. This avoids accidentally deploying the wrong configurations.
-
Automate Cleanup: As highlighted in the article "Eliminate Orphaned ConfigMaps: A Step-by-Step Guide" available at infinitejs.com/posts/eliminate-orphaned-configmaps-guide, automate the cleanup process of unused or orphaned ConfigMaps through scripts or CI/CD pipelines.
A Final Look
Managing ConfigMaps effectively will allow you to decouple configuration from your application, provide flexibility, and facilitate easier updates. By following best practices and leveraging Spring Boot features for configuration management, you can enhance the maintainability of your Java applications running in a Kubernetes environment.
With Kubernetes consistently evolving, keeping informed on how to leverage its capabilities will help maintain smooth application operations. For a deeper dive into managing ConfigMaps, consult the detailed guide referenced above.
Now that you have a solid understanding and implementation of ConfigMaps in Kubernetes with Java, go forth and enhance your applications!