Securing Your Java Web Apps: Don’t Miss These Common Flaws
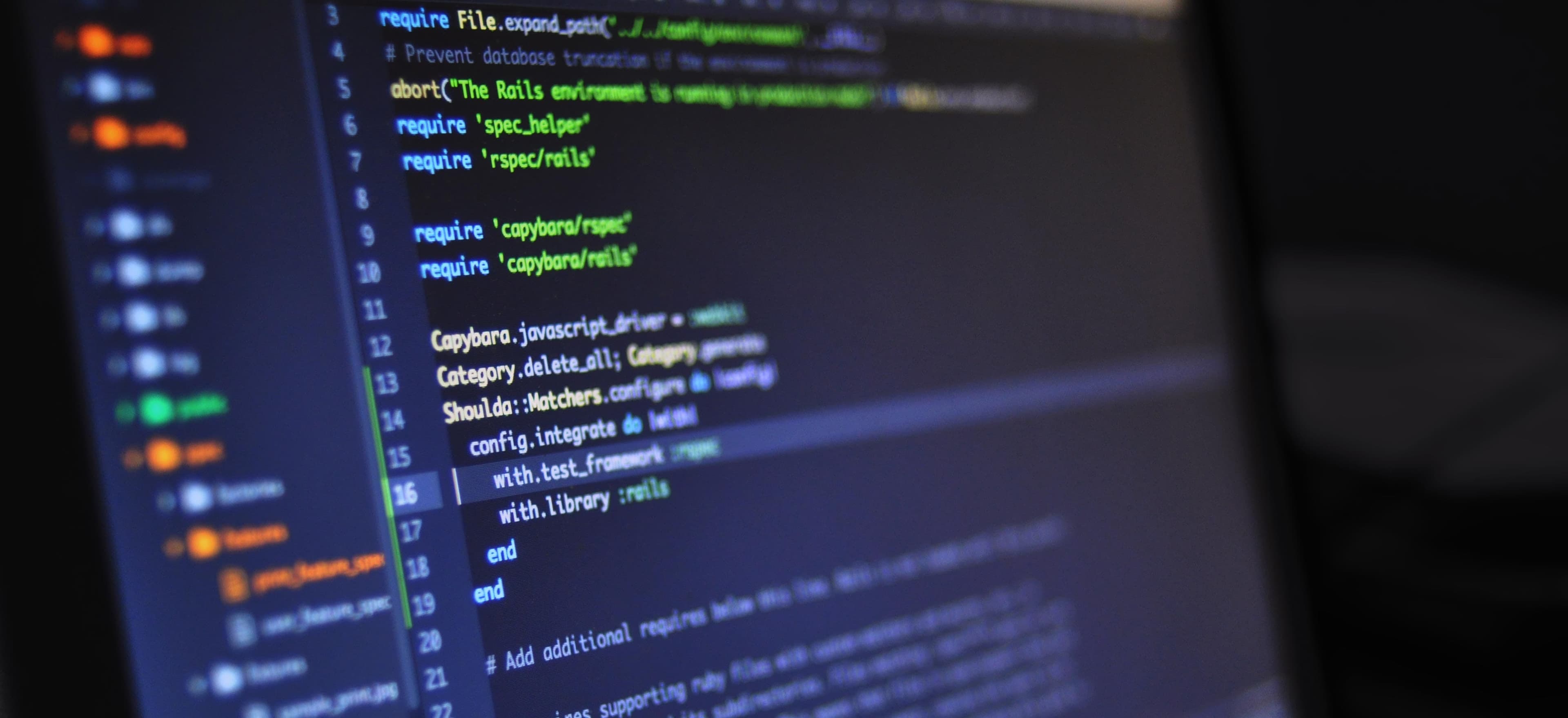
- Published on
Securing Your Java Web Apps: Don't Miss These Common Flaws
Web applications have become the backbone of the digital world, serving millions of users daily. With this growth, however, comes the increasing threat of security vulnerabilities. Java, as one of the most widely-used programming languages for building web applications, demands special attention when it comes to security. In this post, we will explore common security flaws in Java web applications and provide practical advice on how to mitigate these risks.
Understanding Secure Coding Practices
When writing Java code, developers should always adopt secure coding practices. This not only protects users but also enhances the reputation of the application. A recent article titled Top 5 Overlooked Security Flaws in Web Apps 2021 emphasizes the importance of being aware of potential security gaps. Let’s now dive into those crucial elements of security.
1. SQL Injection
What is SQL Injection?
SQL Injection (SQLi) is a code injection technique wherein an attacker interferes with the queries that an application makes to its database. This can lead to unauthorized data access or manipulation.
Prevention: Always use Prepared Statements or ORM frameworks.
Example:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
public class SecureDatabaseAccess {
public void getUser(String username) throws Exception {
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "user", "password");
String query = "SELECT * FROM users WHERE username = ?";
PreparedStatement stmt = conn.prepareStatement(query);
stmt.setString(1, username); // Set user input as a parameter
ResultSet rs = stmt.executeQuery();
while (rs.next()) {
System.out.println("User found: " + rs.getString("username"));
}
rs.close();
stmt.close();
conn.close();
}
}
Why It Matters:
Using Prepared Statements prevents SQL injection because the input is treated as a variable rather than part of the SQL command. This means that even if an attacker attempts to inject SQL code, it will not execute.
2. Cross-Site Scripting (XSS)
What is XSS?
Cross-Site Scripting occurs when an application includes untrusted data on a web page without proper validation or escaping. This can allow attackers to execute scripts in a user's browser.
Prevention: Always validate and sanitize user inputs.
Example:
import javax.servlet.http.HttpServletRequest;
import org.owasp.html.HtmlPolicyBuilder;
public class XSSPrevention {
public String sanitizeUserInput(HttpServletRequest request) {
String unsafeInput = request.getParameter("userInput");
String safeInput = new HtmlPolicyBuilder().toFactory().sanitize(unsafeInput);
return safeInput;
}
}
Why It Matters:
In this snippet, we use a library that sanitizes user input by removing any malicious code. By validating and escaping data before including it in the response, you mitigate the risk of XSS attacks.
3. Insecure Deserialization
What is Insecure Deserialization?
This vulnerability occurs when untrusted data is injected into a program and is later deserialized. Attackers can craft serialized objects that can modify application logic or execute arbitrary code.
Prevention: Avoid using Java's native serialization for untrusted data.
Example:
import java.io.*;
public class SecureObjectManager {
public MyObject deserializeObject(byte[] data) {
try (ByteArrayInputStream byteInput = new ByteArrayInputStream(data);
ObjectInputStream objectInput = new ObjectInputStream(byteInput)) {
return (MyObject) objectInput.readObject(); // Unsafe without validation
} catch (IOException | ClassNotFoundException e) {
e.printStackTrace();
}
return null;
}
}
Why It Matters:
This example illustrates the inherent risk of deserializing untrusted data directly. Instead, developers should implement their custom serialization mechanisms that validate input before deserializing.
4. Security Misconfiguration
What is Security Misconfiguration?
This vulnerability occurs when an application is deployed with default settings or overly permissive permissions. Attackers can exploit these configurations to gain unauthorized access.
Prevention: Conduct regular security reviews and audit configurations.
Example:
- Ensure that sensitive directories are not publicly accessible in the web server configuration.
- Regularly update dependencies and remove unused services.
Why It Matters:
Security misconfigurations can lead to a higher attack surface than necessary. By maintaining best practices in configuration and having a robust deployment checklist, you can minimize potential vulnerabilities.
5. Broken Authentication
What is Broken Authentication?
This vulnerability arises when authentication methods are poorly implemented. Attackers can exploit weak passwords or predictable session management to hijack user sessions.
Prevention: Use strong hashing algorithms and implement multi-factor authentication.
Example:
import java.security.MessageDigest;
public class PasswordManager {
public String hashPassword(String password) throws Exception {
MessageDigest md = MessageDigest.getInstance("SHA-256");
return bytesToHex(md.digest(password.getBytes("UTF-8")));
}
private String bytesToHex(byte[] bytes) {
StringBuilder sb = new StringBuilder();
for (byte b : bytes) {
sb.append(String.format("%02x", b));
}
return sb.toString();
}
}
Why It Matters:
Using secure hashing algorithms (like SHA-256) ensures that even if a password database is compromised, the actual passwords remain protected. Multi-factor authentication adds another layer, making it harder for unauthorized users to gain access.
Key Takeaways
In conclusion, security should be at the forefront of every Java web application development process. By being educated on common vulnerabilities like SQL Injection, XSS, Insecure Deserialization, Security Misconfiguration, and Broken Authentication, developers can build robust and secure applications.
For enthusiasts looking to deepen their understanding, referencing works like the Top 5 Overlooked Security Flaws in Web Apps 2021 can provide context and additional security insights.
Ensure your Java applications are built on a solid foundation of security practices and watch as the trust of your users grows, paving the way for a successful and safe digital presence.