Managing ConfigMaps in Spring Boot: Avoiding Orphans
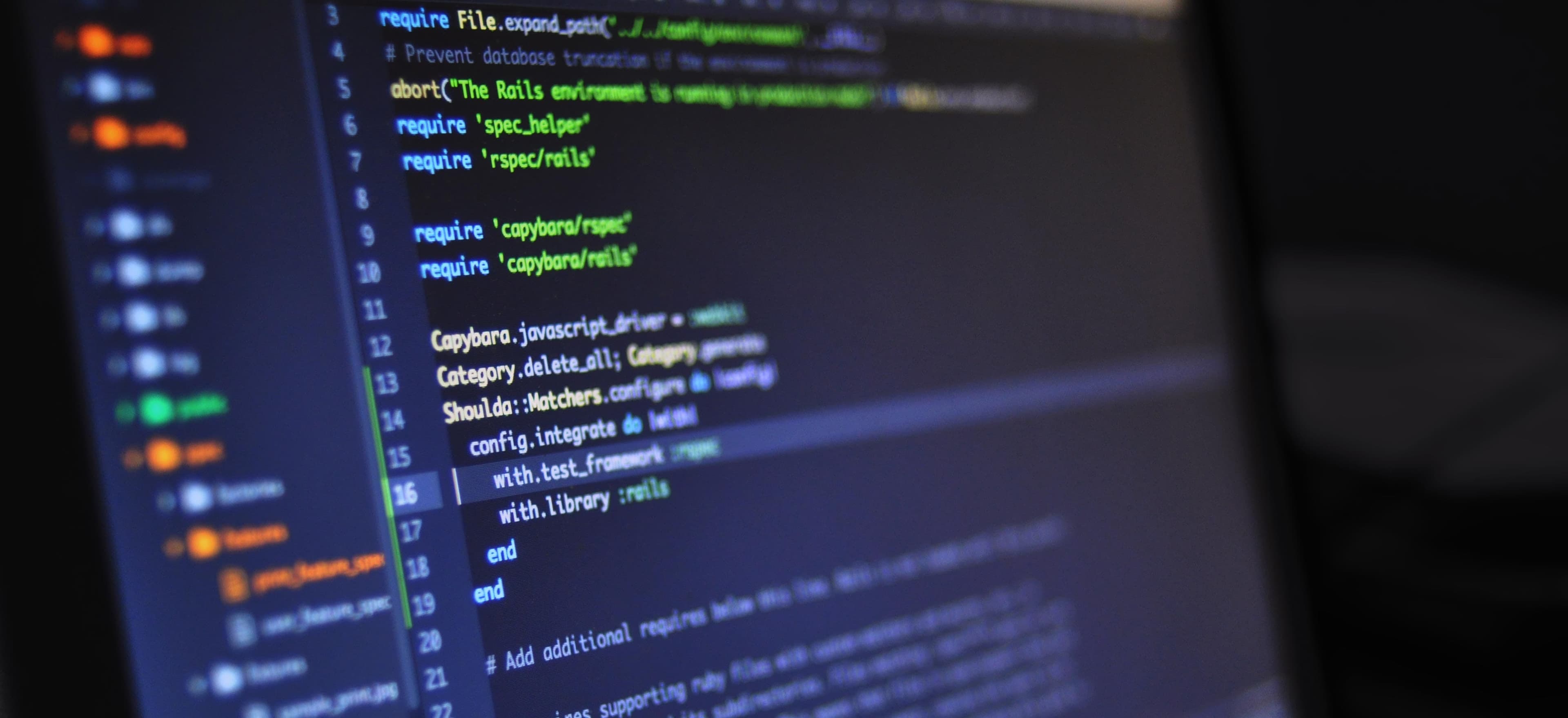
- Published on
Managing ConfigMaps in Spring Boot: Avoiding Orphans
In today's cloud-native world, Kubernetes has become a fundamental platform for deploying and managing applications effectively. One important aspect of Kubernetes is the use of ConfigMaps, which allow you to decouple configuration artifacts from image content in your containerized applications. In this blog post, we will discuss how to manage ConfigMaps effectively in a Spring Boot application, ensuring that you avoid orphaned ConfigMaps.
ConfigMaps help you keep configuration separate from the application code, enhancing the flexibility and maintainability of your applications. However, without proper management, your application can accumulate orphaned ConfigMaps, leading to clutter, confusion, and unnecessary resource consumption.
This post draws on the insights detailed in the existing article titled Eliminate Orphaned ConfigMaps: A Step-by-Step Guide.
Understanding ConfigMaps in Kubernetes
A ConfigMap in Kubernetes is a key-value pair that can be used to pass configuration data to your application. Using ConfigMaps allows you to change configurations without rebuilding your application.
Why Use ConfigMaps?
- Decoupling Configuration from Code: This makes your application more portable and easier to manage.
- Dynamic Updates: You can change configurations on the fly without stopping your application.
- Enhanced Security: Sensitive data can be managed more efficiently using secrets alongside ConfigMaps.
Creating a ConfigMap
Creating a ConfigMap in Kubernetes is relatively straightforward. You can create it from literal values, files, or directories.
Here's a simple example:
kubectl create configmap my-config --from-literal=app.name=myapp --from-literal=app.version=1.0
In this command:
my-config
is the name of the ConfigMap.app.name
andapp.version
are keys holding configuration data.
Utilizing ConfigMap in Spring Boot
In a Spring Boot application, you can inject values from ConfigMaps directly into your application properties. Spring Boot's support for Kubernetes simplifies this process.
To fetch the ConfigMap values, add the following properties in your application.yml
:
spring:
cloud:
kubernetes:
config:
name: my-config
Accessing ConfigMap Values
Once the ConfigMap is created and referenced in your Spring Boot configuration, you can access these values in your code using Spring's @Value
annotation:
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Component;
@Component
public class AppConfig {
@Value("${app.name}")
private String appName;
@Value("${app.version}")
private String appVersion;
public String getAppName() {
return appName;
}
public String getAppVersion() {
return appVersion;
}
}
Why This Matters
Using ConfigMaps effectively allows your application to adapt to different environments without modifying the code base. Therefore, it enhances the DevOps practices by promoting better CI/CD workflows.
Managing and Cleaning Up ConfigMaps
Orphaned ConfigMaps
An orphaned ConfigMap arises when the configuration is no longer in use, but Kubernetes has not removed it automatically. Such leftover ConfigMaps can lead to confusion and wasted resources.
To avoid this, it is essential to keep track of your ConfigMaps accurately. The guide Eliminate Orphaned ConfigMaps: A Step-by-Step Guide outlines effective techniques for managing this aspect.
Techniques to Manage ConfigMaps
- Naming Conventions: Use consistent and meaningful names for your ConfigMaps to easily relate them to the application.
kubectl get configmaps --namespace=my-namespace
- Labeling: Use labels to categorize your ConfigMaps based on the application, version, or environment.
kubectl label configmap my-config app=myapp version=1.0
- Periodic Cleanup: Implement a regular review cycle of your ConfigMaps to identify and delete orphans.
kubectl delete configmap my-obsolete-config
Automating ConfigMap Management
To enhance efficiency and avoid human error, consider automating your ConfigMap management workflow using tools like Helm or Argo CD. These tools can help package and track your application configurations.
Example: Building a Spring Boot Application with ConfigMaps
Below is a simple Spring Boot application code to demonstrate a basic use case of ConfigMaps.
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@SpringBootApplication
public class MyApp {
public static void main(String[] args) {
SpringApplication.run(MyApp.class, args);
}
@RestController
public class HomeController {
private final AppConfig appConfig;
public HomeController(AppConfig appConfig) {
this.appConfig = appConfig;
}
@GetMapping("/")
public String greet() {
return "Welcome to " + appConfig.getAppName() + " version " + appConfig.getAppVersion();
}
}
}
Why This Example Matters
This simple application illustrates how you can leverage ConfigMaps in your Spring Boot application. It shows how to externalize configuration and keep code clean and maintainable.
To Wrap Things Up
Managing ConfigMaps in a Spring Boot application is crucial for ensuring that your application remains clean, efficient, and adaptable to changes. By following the best practices outlined above and leveraging insights from resources like Eliminate Orphaned ConfigMaps: A Step-by-Step Guide, you can effectively avoid orphaned ConfigMaps, streamline your applications, and improve your overall deployment strategy.
By adopting these strategies and making use of available tools, you can ensure your applications are more manageable and sustainable. Happy coding!
Checkout our other articles