Mastering URL Variable Attachment in Java Applications
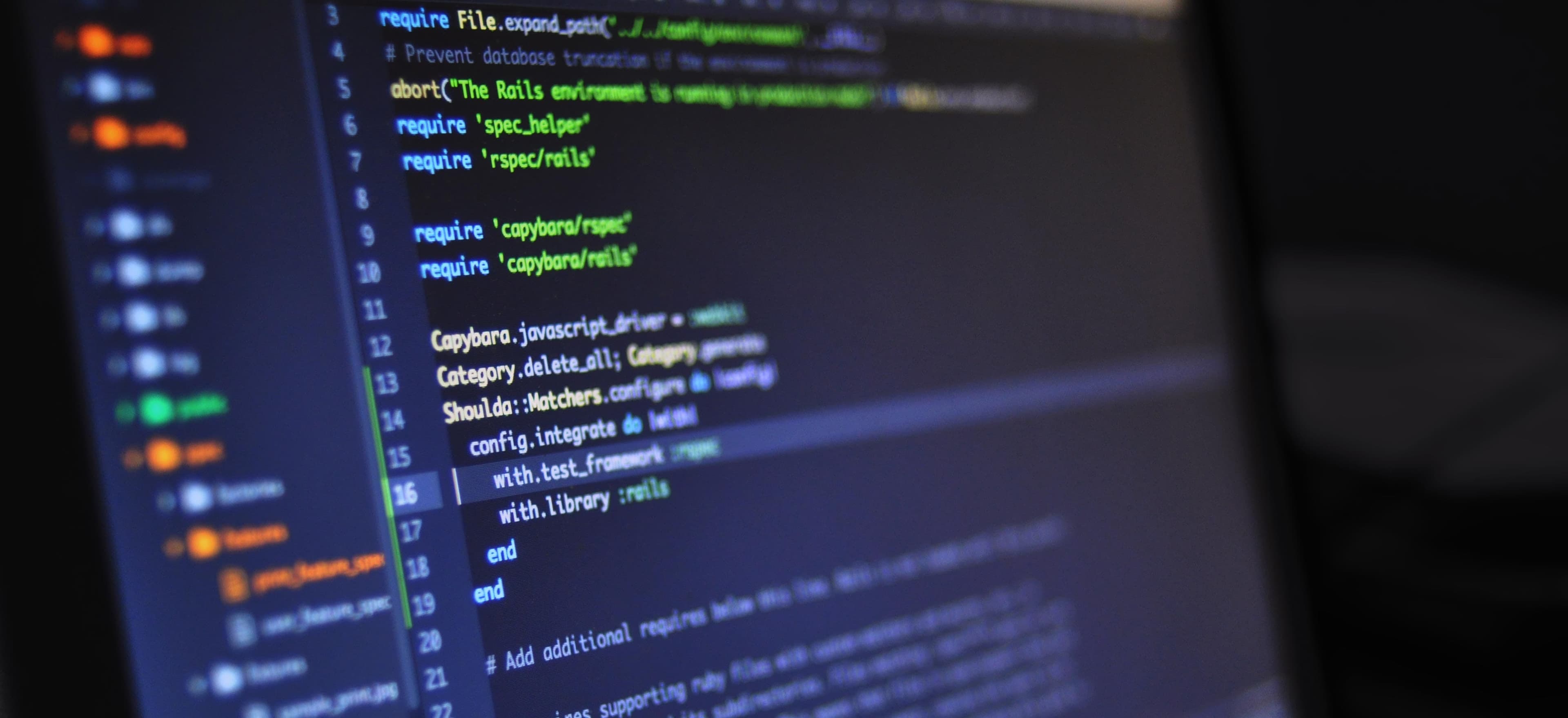
- Published on
Mastering URL Variable Attachment in Java Applications
In the world of web development, understanding how to effectively attach URL variables is crucial. Whether creating a RESTful API, a simple web application, or a complex backend system, mastering this technique can greatly enhance how your application interfaces with its users and other services. In this blog post, we'll dive deep into using URL variables in Java applications, exploring various methods and best practices to ensure smooth integration.
What Are URL Variables?
URL variables, often called query parameters, are a way to pass data to the server through URLs. They can significantly influence how APIs function and how web pages display dynamic content.
Basic Structure of URL Variables
A typical URL can include variables organized as key-value pairs after the question mark. For example:
https://example.com/api/users?id=123&name=JohnDoe
In this example, id
and name
are variable names, while 123
and JohnDoe
are their respective values.
Why Use URL Variables?
Using URL variables has a multitude of benefits:
- Dynamic Data Handling: They enable the processing of dynamic data without the need for additional endpoints.
- SEO Benefits: Well-structured URLs can enhance search engine optimization by making content more discoverable.
- Simplified State Management: It allows applications to maintain state or filter information based on user input.
For a deeper understanding of the common pitfalls when attaching URL variables, check out the article titled Overcoming Common Pitfalls in URL Variable Attachment.
How to Attach URL Variables in Java
Using HttpURLConnection
A straightforward way to attach URL variables in Java is through the HttpURLConnection
class. This approach is particularly effective for simple API calls.
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class APIClient {
public static void main(String[] args) {
String baseURL = "https://example.com/api/users";
String id = "123";
String name = "JohnDoe";
try {
// Constructing the URL with query parameters
String query = String.format("?id=%s&name=%s", id, name);
URL url = new URL(baseURL + query);
// Open a connection
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("GET");
// Read the response
BufferedReader in = new BufferedReader(new InputStreamReader(conn.getInputStream()));
String inputLine;
StringBuilder response = new StringBuilder();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
// Output the response
System.out.println("Response: " + response.toString());
} catch (IOException e) {
e.printStackTrace();
}
}
}
Commentary
- URL Construction: The
String.format()
method is a clean way to construct the URL with variables. This method makes it easy to embed variables directly into the string. - HttpURLConnection: This class handles the connection to the server. Remember to set the request method appropriately (e.g., GET, POST).
- Reading the Response: Utilizing a BufferedReader allows for efficient reading of the input stream.
Using a Library - Apache HttpClient
While HttpURLConnection
is straightforward, using a third-party library like Apache HttpClient can streamline your code, especially for complex requests.
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils;
public class ApacheHttpClientDemo {
public static void main(String[] args) {
String baseURL = "https://example.com/api/users";
String id = "123";
String name = "JohnDoe";
try (CloseableHttpClient httpClient = HttpClients.createDefault()) {
String fullUrl = String.format("%s?id=%s&name=%s", baseURL, id, name);
HttpGet httpGet = new HttpGet(fullUrl);
try (CloseableHttpResponse response = httpClient.execute(httpGet)) {
String responseString = EntityUtils.toString(response.getEntity());
System.out.println("Response: " + responseString);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
Commentary
- Library Usage: The
Apache HttpClient
API offers more features and flexibility, making it ideal for handling complex scenarios. - Resource Management: The try-with-resources statement ensures that resources like
CloseableHttpClient
are appropriately managed. - Improved Readability: This code is more concise and easier to read, emphasizing the importance of using robust libraries.
Best Practices for URL Variable Attachment
-
Always Use URL Encoding: To avoid issues with special characters and spaces, encode your query parameters using
URLEncoder.encode()
.String encodedName = URLEncoder.encode(name, "UTF-8");
-
Limit the Length of URLs: Browsers and servers might have constraints on URL length. Be mindful of how much data you include as URL variables.
-
Keep Variables Meaningful: Name your variables in a way that makes sense for what they represent. This practice helps with code readability and maintainability.
-
Validate Input: Always validate any input from URL variables to protect against injection attacks and ensure the integrity of your application.
-
Utilize Logging: Integrating logging for the incoming requests can help debug issues related to variable attachments effectively.
Final Thoughts
Mastering URL variable attachment in Java applications is an essential skill for any developer working with APIs or web services. By understanding how to construct URLs properly and using the right tools, you can create robust applications that elegantly handle a variety of dynamic data scenarios. For more advanced insights into common issues and solutions, revisit the article Overcoming Common Pitfalls in URL Variable Attachment.
With proper coding practices and a keen understanding of your tools, you can enhance your Java applications significantly. Happy coding!
Checkout our other articles