Java Solutions for Handling Push Notifications in Mobile Apps
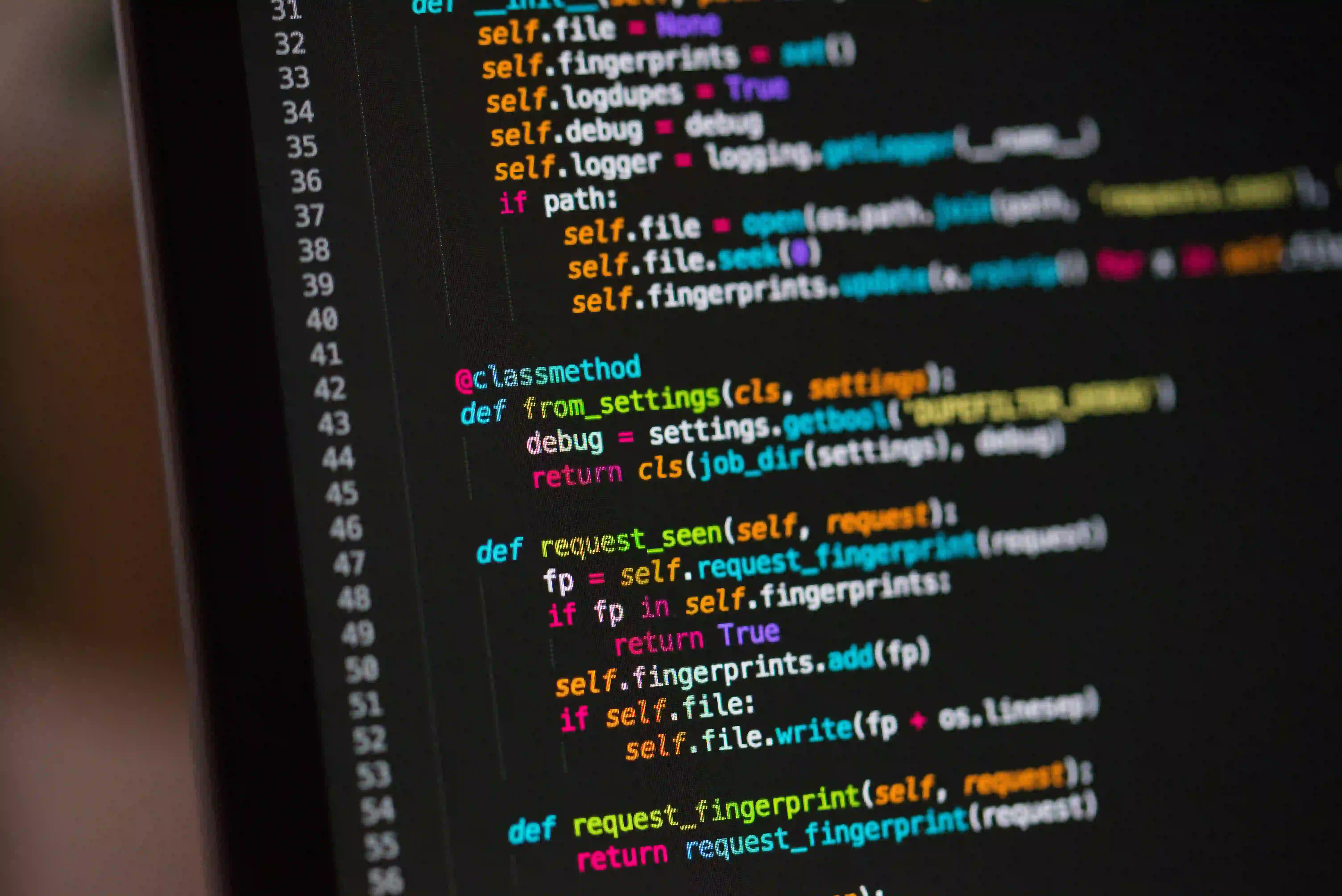
Java Solutions for Handling Push Notifications in Mobile Apps
Push notifications have become an integral part of mobile applications, engaging users and delivering timely information. However, managing push notifications can be challenging, particularly when developing apps in Java. In this blog post, we will explore effective Java solutions for handling push notifications, ensuring smooth user experiences across various platforms.
What are Push Notifications?
Push notifications are messages sent from a server to an application on a device. They can alert users about new messages, promotional offers, updates, and more, even when the app is not actively being used. They can significantly enhance user retention and engagement when implemented correctly.
Why Use Java for Push Notifications?
Java is a versatile and widely-used programming language, particularly in Android development. With its rich framework support and community, Java provides robust solutions for handling push notifications:
- Cross-Platform Compatibility: Java applications can run on any device with a Java Virtual Machine (JVM).
- Strong Community Support: There is an abundance of resources, libraries, and tools available to assist developers.
- Scalability: Java is known for its scalable architecture, ideal for applications requiring push notifications at scale.
Overview of Push Notification Mechanism
Before diving into Java solutions, it's crucial to understand how push notifications work:
- Registration: The client app registers with a push notification service (like Firebase Cloud Messaging or Amazon SNS) to obtain a unique device token.
- Payload Creation: The server creates a payload that contains the message and metadata for the notification.
- Sending the Notification: The server sends the notification payload to the push notification service, which forwards it to the client app.
- User Interaction: The client app receives the notification and can display it, even if the app isn't running.
Setting Up Push Notifications with Firebase in Java
Firebase Cloud Messaging (FCM) is one of the most popular services for implementing push notifications. Below are steps and code snippets to set it up using Java.
Step 1: Add Firebase to Your Android Project
To start, include the Firebase dependencies in your build.gradle
file:
implementation 'com.google.firebase:firebase-messaging:23.0.0'
This will add the necessary libraries for using Firebase Cloud Messaging in your Android app.
Step 2: Register for Push Notifications
In your main Java activity class, initialize FCM and request a device token:
import com.google.firebase.messaging.FirebaseMessaging;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Get device token
FirebaseMessaging.getInstance().getToken()
.addOnCompleteListener(task -> {
if (!task.isSuccessful()) {
Log.w(TAG, "Fetching FCM registration token failed", task.getException());
return;
}
// Get new FCM registration token
String token = task.getResult();
Log.d(TAG, "FCM Registration Token: " + token);
// Send the token to your server for target notifications
});
}
}
Why this code? This code initializes Firebase messaging and retrieves a unique device token for further communication with the Firebase server. This token will be utilized for sending targeted notifications.
Step 3: Handle Incoming Notifications
To handle incoming notifications, create a service that extends FirebaseMessagingService
:
import android.app.NotificationChannel;
import android.app.NotificationManager;
import android.app.PendingIntent;
import android.content.Intent;
import android.os.Build;
import androidx.core.app.NotificationCompat;
import com.google.firebase.messaging.FirebaseMessagingService;
import com.google.firebase.messaging.RemoteMessage;
public class MyFirebaseMessagingService extends FirebaseMessagingService {
@Override
public void onMessageReceived(RemoteMessage remoteMessage) {
// Handle FCM messages here.
if (remoteMessage.getNotification() != null) {
sendNotification(remoteMessage.getNotification().getBody());
}
}
private void sendNotification(String messageBody) {
Intent intent = new Intent(this, MainActivity.class);
intent.addFlags(Intent.FLAG_ACTIVITY_CLEAR_TOP);
PendingIntent pendingIntent = PendingIntent.getActivity(this, 0, intent, PendingIntent.FLAG_ONE_SHOT);
NotificationCompat.Builder notificationBuilder = new NotificationCompat.Builder(this, "your_channel_id")
.setSmallIcon(R.drawable.ic_notification)
.setContentTitle("My Notification")
.setContentText(messageBody)
.setAutoCancel(true)
.setContentIntent(pendingIntent);
NotificationManager notificationManager = (NotificationManager) getSystemService(NOTIFICATION_SERVICE);
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) {
NotificationChannel channel = new NotificationChannel("your_channel_id", "Channel human readable title", NotificationManager.IMPORTANCE_DEFAULT);
notificationManager.createNotificationChannel(channel);
}
notificationManager.notify(0, notificationBuilder.build());
}
}
Why this code? The service overrides onMessageReceived
to handle incoming notifications. When a notification arrives, it builds and displays it using an NotificationCompat
builder. This encapsulates best practices for notifications on Android and ensures they adhere to Android's notification channels.
Working with a Java Backend to Send Notifications
Now that we have set up the client-side, let’s discuss the server aspect of sending notifications using Java.
Using Spring Boot to Send Push Notifications
You can set up a simple Spring Boot application to send notifications via FCM. Here’s a basic example:
import org.springframework.web.bind.annotation.*;
import org.springframework.http.ResponseEntity;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpEntity;
import org.springframework.http.HttpMethod;
import org.springframework.web.client.RestTemplate;
@RestController
@RequestMapping("/api")
public class NotificationController {
private final String FCM_URL = "https://fcm.googleapis.com/fcm/send";
private final String SERVER_KEY = "YOUR_SERVER_KEY";
@PostMapping("/sendNotification")
public ResponseEntity<String> sendNotification(@RequestBody NotificationRequest request) {
RestTemplate restTemplate = new RestTemplate();
HttpHeaders headers = new HttpHeaders();
headers.add("Authorization", "key=" + SERVER_KEY);
headers.add("Content-Type", "application/json");
String body = String.format("{\"to\": \"%s\", \"notification\": {\"title\": \"%s\", \"body\": \"%s\"}}",
request.getToken(), request.getTitle(), request.getBody());
HttpEntity<String> entity = new HttpEntity<>(body, headers);
String response = restTemplate.exchange(FCM_URL, HttpMethod.POST, entity, String.class).getBody();
return ResponseEntity.ok(response);
}
}
class NotificationRequest {
private String token;
private String title;
private String body;
// getters and setters
}
Why this code? This Spring Boot example demonstrates how to create an API endpoint to send notifications using Firebase Cloud Messaging. The REST
API constructs and sends a JSON payload, which contains the target device token, title, and body of the notification.
Best Practices for Push Notifications
When integrating push notifications, consider the following best practices:
- Be Relevant: Ensure notifications provide real value to users.
- Don't Overwhelm Users: Too many notifications can trigger app uninstalls. Focus on quality over quantity.
- Allow Users to Control Preferences: Provide settings in your app where users can manage notification preferences.
Troubleshooting Push Notification Issues
As referenced in the article "Troubleshooting Push Notification Setup in React Native", issues around push notifications can arise due to several factors such as API keys, device tokens, or when notifications are blocked by the OS. Always ensure that your keys are correctly configured and that you are handling token refreshes appropriately on the client side.
Key Takeaways
Push notifications in Java applications provide a pathway for rich user engagement. Whether you're using Firebase for Android or a Java backend like Spring Boot, understanding how to efficiently implement and manage notifications will benefit both users and developers. By following the outlined steps and best practices, you will be able to enhance your application's communication strategy significantly.
Feel free to dive deeper into each library and practice implementing push notifications tailored to your specific requirements.