Debugging Push Notifications in Your Java Backend
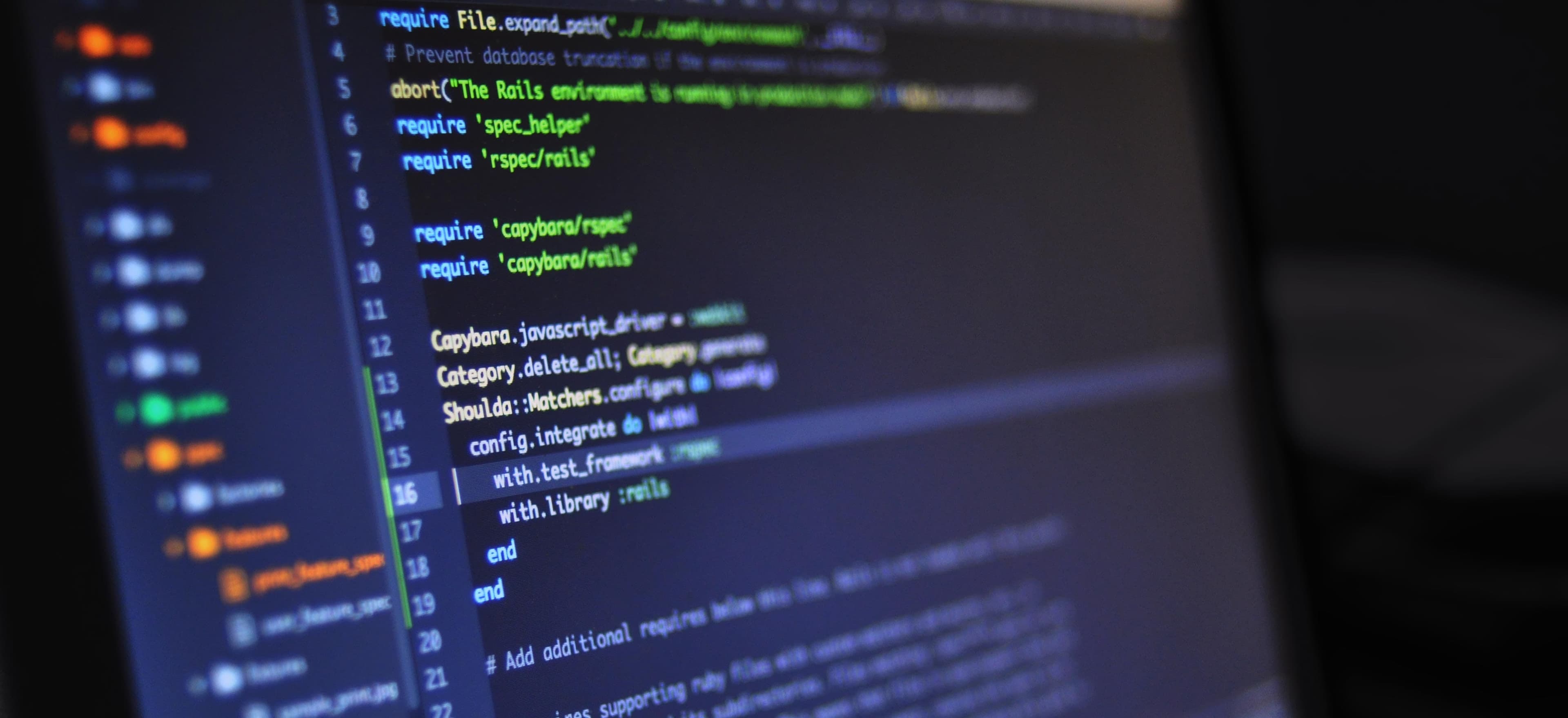
- Published on
Debugging Push Notifications in Your Java Backend
Push notifications have become an essential feature in modern applications, keeping users engaged and informed. However, setting up and troubleshooting push notifications can be a challenging task, especially when integrating with a mobile front-end like React Native. In this blog post, we will cover key aspects of debugging push notifications in your Java backend. We will walk you through common challenges, provide code snippets, and offer best practices, all while ensuring that you have the tools needed to succeed in this endeavor.
Understanding Push Notifications
Push notifications are messages sent from a server to a client app. They can trigger alerts, updates, or prompts even when the app is not actively running. In a typical setup, a mobile app subscribes to notifications from a backend service, which handles the logic for managing and sending these notifications.
The Architecture
The basic architecture for push notifications generally involves three main components:
- Mobile Application: This could be built using frameworks like React Native. It registers for push notifications and receives them.
- Push Notification Service: This could be Firebase Cloud Messaging (FCM), OneSignal, etc. It handles the transmission of notifications.
- Java Backend: This is responsible for sending requests to the push notification service and handling interactions with client applications.
Setting Up Your Java Backend
Setting up your Java backend for push notifications typically involves using libraries that facilitate communication with the push service. Here, we will use Firebase Cloud Messaging (FCM) as an example.
Maven Dependencies
First, ensure you have the necessary dependencies in your pom.xml
:
<dependency>
<groupId>com.google.firebase</groupId>
<artifactId>firebase-admin</artifactId>
<version>8.0.0</version>
</dependency>
Initializing Firebase Admin SDK
To interact with FCM, you'll need to initialize the Firebase Admin SDK. This is typically done in your application’s startup logic.
import com.google.firebase.FirebaseApp;
import com.google.firebase.FirebaseOptions;
import java.io.FileInputStream;
import java.io.IOException;
public class FirebaseInitializer {
public void initializeFirebase() {
try {
FileInputStream serviceAccount = new FileInputStream("path/to/serviceAccountKey.json");
FirebaseOptions options = new FirebaseOptions.Builder()
.setCredentials(GoogleCredentials.fromStream(serviceAccount))
.setDatabaseUrl("https://<DATABASE_NAME>.firebaseio.com")
.build();
FirebaseApp.initializeApp(options);
} catch (IOException e) {
e.printStackTrace();
// Log the error
}
}
}
Why this code is important: This code snippet shows how to initialize the Firebase connection using credentials. Without this setup, you'll face authentication failures when sending push notifications.
Sending a Notification
Once initialized, sending a notification is straightforward. Using the FCM library, you can easily create and send notifications.
import com.google.firebase.messaging.FirebaseMessaging;
import com.google.firebase.messaging.Message;
public void sendPushNotification(String token) {
Message message = Message.builder()
.setToken(token)
.putData("title", "Hello World!")
.putData("body", "This is a test push notification from Java backend.")
.build();
try {
String response = FirebaseMessaging.getInstance().send(message);
System.out.println("Successfully sent message: " + response);
} catch (FirebaseMessagingException e) {
e.printStackTrace();
// Handle the error gracefully here
}
}
Why this code is important: The sendPushNotification
method creates a notification and sends it using FCM. It is crucial to handle exceptions to gracefully deal with issues such as invalid tokens or network errors.
Debugging Steps
As with any backend service, debugging push notifications requires a systematic approach. Below are common issues you may encounter and how to troubleshoot them.
1. Invalid Registration Token
An invalid registration token is one of the most common errors. If a token is invalid, the push service cannot deliver messages.
Solution: Check the client’s token on the mobile application. Ensure that it is registered correctly and that it has not been expired or deleted.
Example Log Output: An invalid token will yield a NOT_FOUND
for the token in the logs. Consider implementing token lifecycle management to periodically remove old tokens.
2. Check Push Notification Service Status
When debugging, it is essential to ensure that your push notification service is operational.
Solution: Verify that FCM does not have any outages by checking their status page or logs.
3. Inspect Logs
Logging is your best friend while debugging. Capture the complete stack trace when an error occurs:
try {
String response = FirebaseMessaging.getInstance().send(message);
System.out.println("Successfully sent message: " + response);
} catch (FirebaseMessagingException e) {
System.err.println("Error sending notification: " + e.getMessage());
e.printStackTrace();
}
Why this code is important: Keeping an eye on the logs will help you quickly identify what part of your process is breaking down. Log specific messages, not just generic outputs.
Best Practices for Push Notifications
- Thorough Testing: Always test notifications in a production-like environment. This ensures that all components interact seamlessly.
- User Preferences: Allow users to manage their notification preferences. Not every user wants the same type of notifications, and respecting their choices can improve engagement.
- Error Handling: Implement robust error handling. React Native applications can use the response messages to inform users if a notification has failed.
- Consult External Resources: If you face persistent issues, consider consulting articles such as Troubleshooting Push Notification Setup in React Native, which covers related topics from the mobile application's perspective.
Final Thoughts
Debugging push notifications in your Java backend does not have to be an overwhelming task. By following a systematic approach—initializing the FCM service, sending notifications, inspecting logs, and applying best practices—you can efficiently handle push notifications. Continue learning and adapting as new tools and methods emerge; this field is ever-evolving.
By sharing the knowledge and tools at your disposal, you will not only enhance your application’s functionality but also improve user engagement. Happy coding!
Checkout our other articles