Resolving MySQL Connection Hurdles in Java Applications
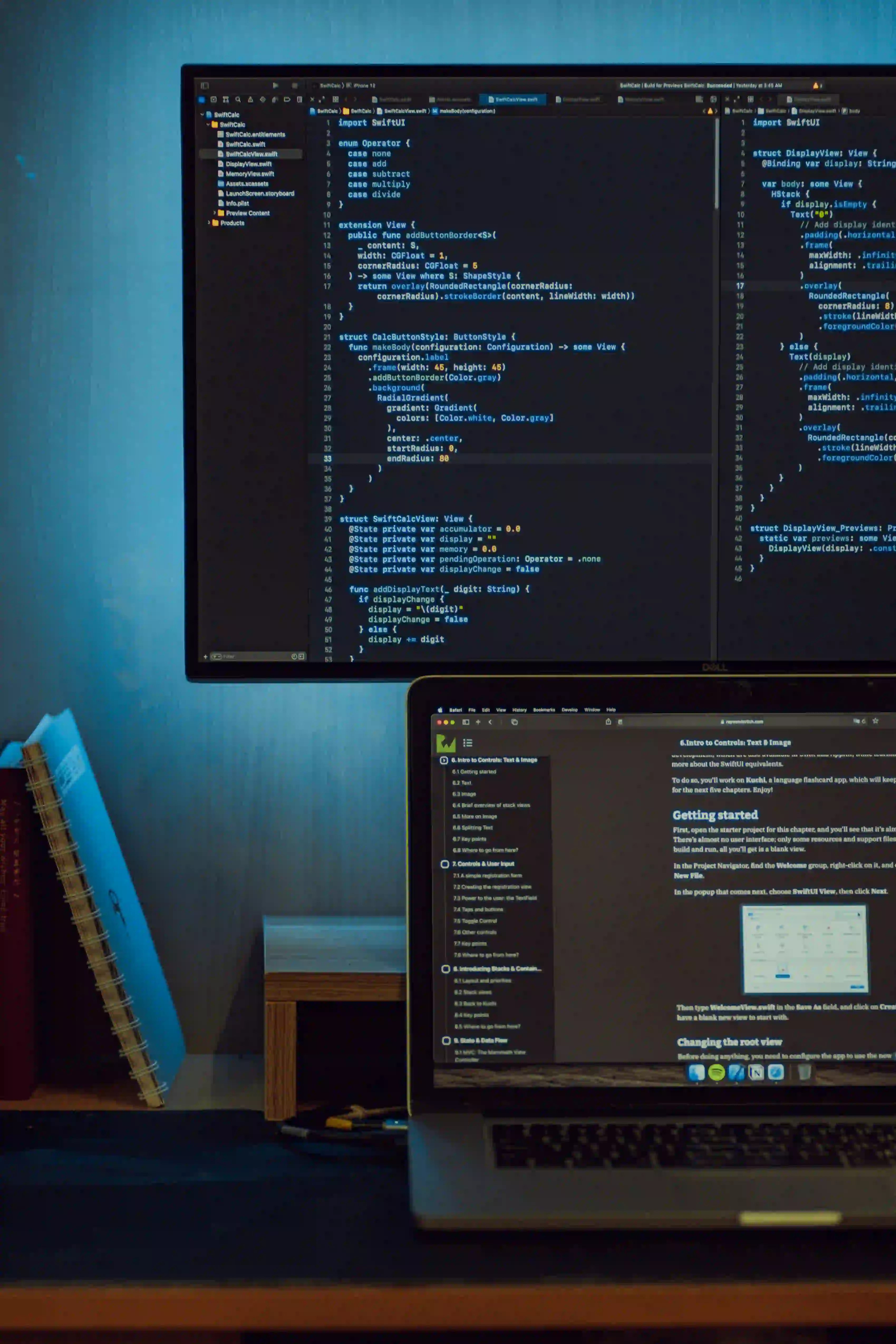
Resolving MySQL Connection Hurdles in Java Applications
In today's fast-paced digital landscape, database connectivity is crucial for web applications, data-driven systems, and enterprise solutions. Among the various databases, MySQL remains one of the most popular choices due to its performance, reliability, and community support. However, developers often encounter connection issues that can significantly hinder application performance. In this blog post, we will delve into common MySQL connection hurdles specifically in the context of Java applications and provide practical solutions to resolve these issues.
Understanding MySQL and JDBC
Before we jump into troubleshooting connection issues, it's important to understand how Java connects to a MySQL database. The Java Database Connectivity (JDBC) API facilitates this interaction, providing a standard method for connecting and executing SQL queries on databases.
Code Snippet: Simple JDBC Connection
Here's a simple example of how to establish a connection to a MySQL database using JDBC:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class MySQLConnection {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/mydatabase";
String user = "username";
String password = "password";
try {
Connection connection = DriverManager.getConnection(url, user, password);
System.out.println("Connection Successful!");
// Your logic here
} catch (SQLException e) {
e.printStackTrace();
}
}
}
Explanation
- DriverManager: This is used to establish a connection to the database.
- Connection URL: It includes the database type (jdbc:mysql), host (localhost), port (3306), and database name (mydatabase).
- Credentials: User credentials are required to authenticate access to the database.
In a real-world application, consider handling credentials securely, possibly using environment variables or encrypted storage.
Common Connection Issues
Many issues can disrupt your MySQL connection. Let’s explore some common ones:
1. Incorrect JDBC URL
The JDBC URL is the gateway to the database. Simple typographical errors can lead to connection failures.
Solution
Confirm that your JDBC URL adheres to the expected format:
jdbc:mysql://hostname:port/databasename
Be sure to replace hostname
, port
, and databasename
with the accurate values.
2. MySQL Server Not Running
It is critical that the MySQL server is running. You can test this by executing the following command in your terminal:
mysqladmin -u username -p status
Solution
If the server is not running, start it using the appropriate command for your operating system. For example, in Linux, it may be:
sudo service mysql start
3. Firewall Issues
Firewalls may block access to your MySQL server, especially in production environments or cloud-hosted applications.
Solution
Ensure that the port used by MySQL is open. The default port for MySQL is 3306. You might need to allow access through your firewall settings.
4. Driver Issues
A missing or incompatible JDBC driver will result in connection failures. Always ensure that you have the correct version of the MySQL Connector/J library.
Solution
You can download the JDBC driver from the official MySQL site. Include it in your project dependencies:
-
For Maven users, the dependency in your
pom.xml
would look like:📄snippet.txt<dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <version>8.0.26</version> </dependency>
5. SSL Connection Requirement
Some MySQL servers have SSL connections enforced. Attempting to connect without SSL will result in failure.
Solution
You can add SSL parameters to your JDBC URL:
String url = "jdbc:mysql://localhost:3306/mydatabase?useSSL=true&requireSSL=true";
Handling Connection Exceptions
Managing SQL exceptions effectively is essential. Here's a refined example that handles exceptions in a meaningful way:
try {
Connection connection = DriverManager.getConnection(url, user, password);
System.out.println("Connection Successful!");
} catch (SQLException e) {
System.err.println("Connection Failed! Check output console");
e.printStackTrace();
}
Explanation
- Meaningful Error Messages: Providing clear messages helps developers troubleshoot effectively.
- Error Stack Trace: The stack trace provides insight into what went wrong, aiding debugging efforts.
Connection Pooling
For applications that require frequent connections to the database, consider implementing connection pooling. This approach improves performance significantly.
Code Snippet: Using HikariCP for Connection Pooling
Here's an example of setting up HikariCP, a robust connection pooling library:
import com.zaxxer.hikari.HikariConfig;
import com.zaxxer.hikari.HikariDataSource;
public class DataSource {
private static HikariDataSource dataSource;
static {
HikariConfig config = new HikariConfig();
config.setJdbcUrl("jdbc:mysql://localhost:3306/mydatabase");
config.setUsername("username");
config.setPassword("password");
config.setMaximumPoolSize(10);
dataSource = new HikariDataSource(config);
}
public static HikariDataSource getDataSource() {
return dataSource;
}
}
Explanation
- HikariConfig: You configure properties such as JDBC URL, user credentials, and the maximum pool size.
- HikariDataSource: Use the getter method to retrieve a data source instance whenever needed.
Additional Resources
For more specific troubleshooting related to MySQL and PHP, you may find the article titled "Troubleshooting MySQL Connection Issues in EasyPHP" helpful. You can access it here.
A Final Look
Resolving MySQL connection hurdles in Java applications requires a good understanding of JDBC, the underlying MySQL server, and best practices for error handling and connection pooling. By addressing the common issues outlined in this article, developers can improve application robustness and user experience.
Whether your application is large or small, mastering these connection techniques is essential. Test your configurations thoroughly, manage exceptions carefully, and don’t hesitate to seek help from communities and resources when needed.
Happy coding, and may your connections remain strong and stable!