Resolving Java Application MySQL Connection Problems
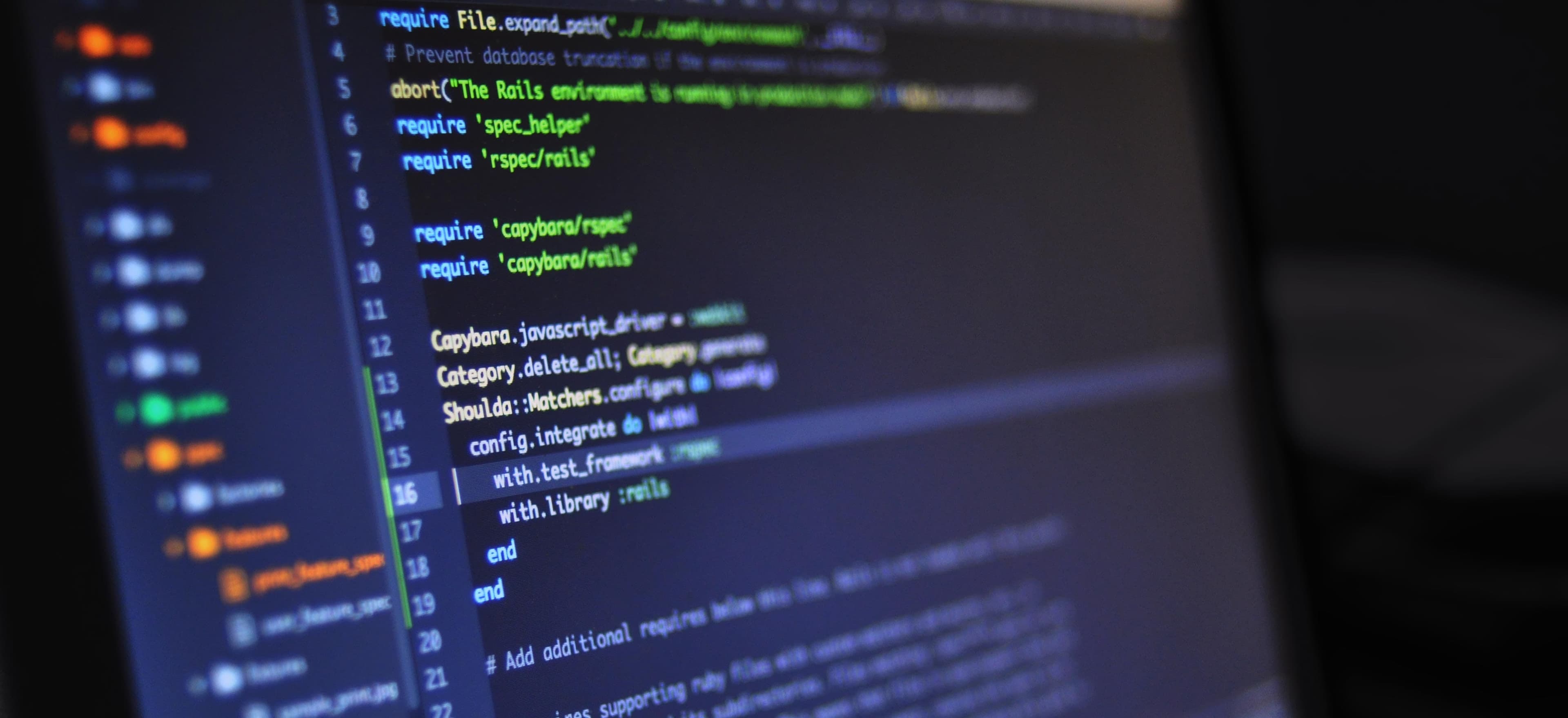
- Published on
Resolving Java Application MySQL Connection Problems
When developing Java applications that need to interact with a MySQL database, establishing a reliable connection is paramount. However, developers often encounter various issues that can lead to connection failures. This blog post will explore the common causes of MySQL connection problems in Java applications and provide practical solutions to overcome these challenges.
In this guide, we will cover:
- Typical connection issues and their causes
- Essential steps to debug and troubleshoot MySQL connections
- Code examples to implement effective database connection management
- Documentation references to deepen your understanding
Understanding MySQL Connection Issues
Before diving into solutions, it’s crucial to understand the common problems that plague developers trying to connect their Java applications to MySQL:
- Incorrect JDBC URL: The URL format must align precisely with MySQL's expectations.
- Authentication Errors: Mistyping the username or password can easily lock you out.
- Driver Issues: Not having the correct MySQL JDBC driver in your project can result in a world of headaches.
- Network Problems: Local environment configurations and firewall settings can block access.
- Database Server Not Running: A simple oversight, but essential to check.
Understanding these can help debug issues more effectively.
Steps to Debug MySQL Connection
Now that we know what might be going wrong, let’s explore how to troubleshoot these problems. The following steps are vital in the debugging process.
Step 1: Verify Your Database Credentials
Ensure that the username, password, and database name provided in the Java application match those configured in your MySQL server. Here's a quick snippet demonstrating how to set up the connection:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class DatabaseConnection {
private static final String URL = "jdbc:mysql://localhost:3306/yourDatabaseName";
private static final String USER = "yourUsername";
private static final String PASSWORD = "yourPassword";
public static Connection getConnection() {
Connection connection = null;
try {
connection = DriverManager.getConnection(URL, USER, PASSWORD);
System.out.println("Connection successful!");
} catch (SQLException e) {
System.err.println("Connection failed: " + e.getMessage());
}
return connection;
}
}
Code Explanation
- DriverManager: This manages the JDBC drivers. It is essential for establishing connections.
- URL: The JDBC URL must specify the hostname (localhost), port number (3306), and database name.
- Connection Handling: Always wrap the connection process in a try-catch block to handle any exceptions gracefully.
Step 2: Test the JDBC Driver
Make sure that your JDBC driver is included in your project’s classpath. If using Maven, include it as a dependency in your pom.xml
:
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.26</version>
</dependency>
Not having the correct version can lead to compatibility issues.
Step 3: Check Network Settings
If using a remote server, check your network settings. Firewalls and security groups may block access to the database. Use telnet or a similar tool to confirm connectivity:
telnet your-database-host 3306
If you don't get a response, there's likely a networking issue that needs resolution.
Step 4: Confirm Server Status
Run the following command in your terminal to ensure the MySQL server is up and running:
sudo systemctl status mysql
You can also check your logs for any reported issues:
tail -f /var/log/mysql/error.log
Step 5: Review Connection String Parameters
Ensure the connection string is correctly formatted. An incorrect parameter can lead to connection failures. Here's an example:
String url = "jdbc:mysql://localhost:3306/yourDatabaseName?useSSL=false&serverTimezone=UTC";
Why use these parameters?
useSSL=false
: If you do not require SSL, it can simplify development and prevent unnecessary connection attempts.serverTimezone=UTC
: Ensures your application correctly handles time zones, avoiding potential errors.
For a more extensive discussion on potential database connection errors, consider checking out the article titled Troubleshooting MySQL Connection Issues in EasyPHP, which can provide additional guidance.
Connection Pooling
For professional-grade applications, it is advisable to implement connection pooling to reuse connections instead of opening a new one each time. This can improve performance significantly.
Example of Connection Pooling using HikariCP
<dependency>
<groupId>com.zaxxer</groupId>
<artifactId>HikariCP</artifactId>
<version>4.0.3</version>
</dependency>
import com.zaxxer.hikari.HikariConfig;
import com.zaxxer.hikari.HikariDataSource;
public class DataSourceManager {
private static HikariDataSource dataSource;
static {
HikariConfig config = new HikariConfig();
config.setJdbcUrl("jdbc:mysql://localhost:3306/yourDatabaseName");
config.setUsername("yourUsername");
config.setPassword("yourPassword");
config.setMaximumPoolSize(10);
dataSource = new HikariDataSource(config);
}
public static Connection getConnection() throws SQLException {
return dataSource.getConnection();
}
}
Explanation of HikariCP
- HikariConfig: Manages configuration parameters for the connection pool.
- Maximum Pool Size: Configured to manage how many connections can be actively maintained.
Final Considerations
MySQL connection problems in Java applications can be a source of frustration, but with the right knowledge and tools, they can be efficiently resolved. Always start by verifying your credentials, testing your JDBC driver, and checking for server status and network settings.
Implementing connection pooling can further bolster your application’s efficiency. Remember to follow best practices regarding error handling and connection management in your Java applications.
For more information on addressing specific MySQL connection problems, review the Troubleshooting MySQL Connection Issues in EasyPHP, as it presents valuable insights and resolutions.
By using the guidelines presented here, you can effectively troubleshoot and resolve MySQL connection problems, allowing your Java applications to perform optimally.
Checkout our other articles