Java Developers: Integrating Vue UI Libraries Effortlessly
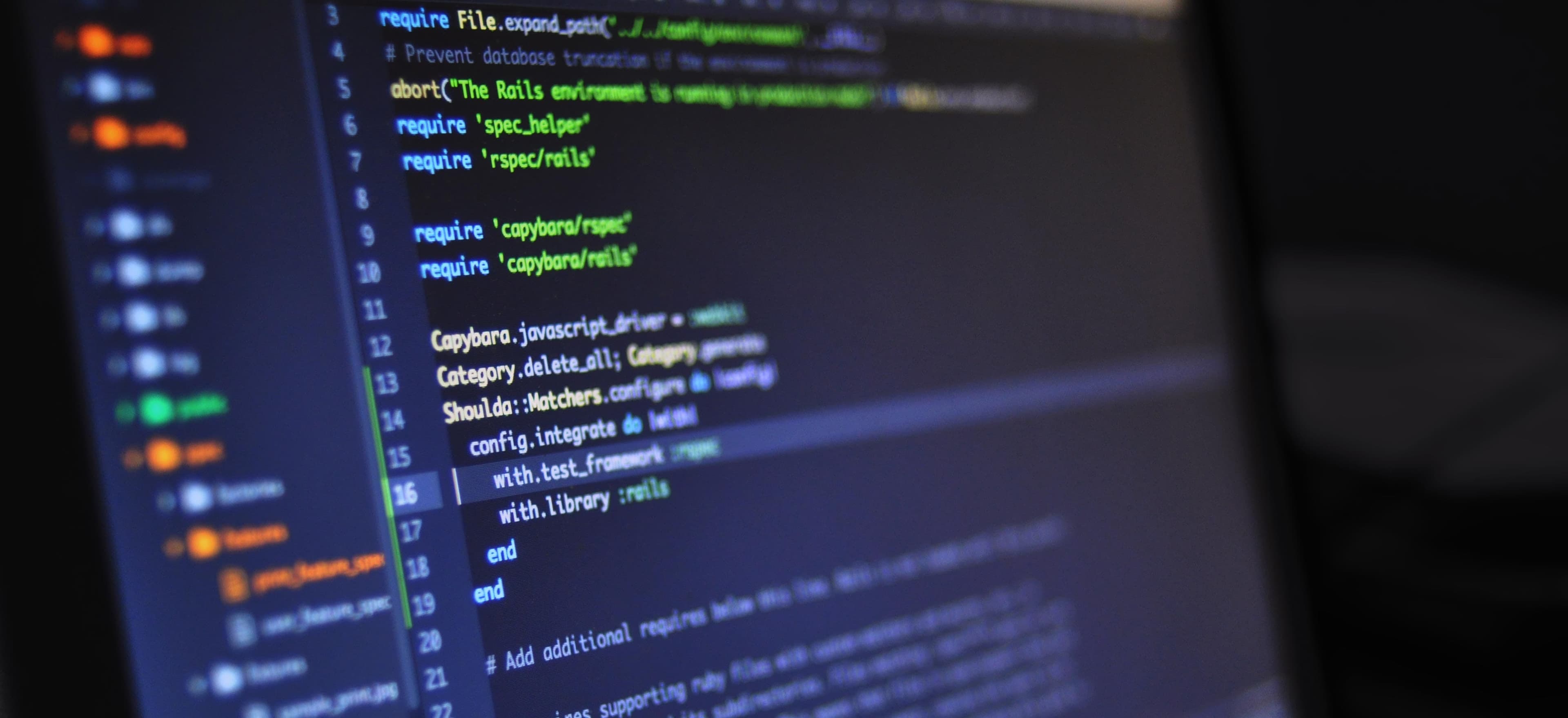
- Published on
Java Developers: Integrating Vue UI Libraries Effortlessly
Java has long been a staple in the world of programming, powering backend services and robust applications. Yet, with the rise of modern frontend frameworks like Vue.js, many Java developers find themselves at the intersection of backend logic and dynamic user interfaces. This blog post will be your guide to integrating Vue UI libraries with Java applications seamlessly. We'll explore essential concepts, provide practical code snippets, and share best practices along the way.
The Need for a Frontend Framework
Front-end frameworks like Vue.js have revolutionized the way developers build interactive user interfaces. They allow developers to create reactive, component-driven designs that enhance user experiences. Java, typically utilized in backend development, can leverage these frameworks to deliver rich client-side functionalities. By integrating Vue UI libraries, Java applications can be more engaging and visually appealing.
Why Choose Vue?
-
Reactive Data Binding: Vue enables developers to create data-driven applications effortlessly. Any changes in the data will automatically update the UI without manual DOM manipulation.
-
Component-Based Architecture: Vue promotes reusable components, which can simplify your codebase and enhance maintainability.
-
Growing Ecosystem: With a wealth of Vue UI libraries available, developers have many tools at their disposal to craft beautiful interfaces.
Choosing the Right UI Library
Before integrating Vue UI libraries, it's critical to choose one that complements your application requirements. As highlighted in the article "Struggling to Choose? Top Vue UI Libraries Simplified!", evaluating libraries based on criteria such as documentation quality, community support, and UI component variety can streamline your decision.
Some popular Vue UI libraries include:
- Vuetify
- Element UI
- BootstrapVue
- Quasar
Example: Integrating Vuetify
In this guide, we will focus on integrating Vuetify, a popular Material Design component framework for Vue.js. Below are the steps to set up Vuetify in a Java-based web application.
Step-by-Step Integration Guide
1. Setting Up the Java Backend
First, establish your Java backend using a framework like Spring Boot. Here’s a simple setup using Maven.
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
</dependencies>
This configuration will set up a RESTful backend that serves your Vue frontend. Spring Boot simplifies dependency management and provides a solid foundation for Java applications.
2. Creating a Vue Project
Next, create a Vue project using Vue CLI. Open your terminal and run:
npm install -g @vue/cli
vue create my-vue-app
Follow the prompts to set up your preferred settings for the project.
3. Adding Vue and Vuetify
Navigate to your newly created Vue project and install Vuetify:
cd my-vue-app
vue add vuetify
This command will guide you through the process, allowing you to choose a preset. Just like that, Vuetify has been integrated.
4. Creating a Sample Component
Next, let’s create a component to display a list of users. Here's an example of a simple Vue component utilizing Vuetify:
<template>
<v-container>
<v-row>
<v-col v-for="user in users" :key="user.id" cols="12" md="4">
<v-card>
<v-card-title>{{ user.name }}</v-card-title>
<v-card-subtitle>{{ user.email }}</v-card-subtitle>
</v-card>
</v-col>
</v-row>
</v-container>
</template>
<script>
export default {
data() {
return {
users: []
};
},
mounted() {
this.fetchUsers();
},
methods: {
async fetchUsers() {
const response = await fetch('/api/users');
this.users = await response.json();
}
}
};
</script>
Why This Component Matters
-
Data Fetching: The mounted lifecycle hook is used to fetch users right after the component is created. This way, when the component loads, it immediately queries the backend.
-
Responsive Design: The component uses Vuetify's grid system, ensuring that the layout adjusts based on screen size.
-
Dynamic Data: By binding the
users
array to the UI, any updates to the component will automatically reflect in the view, thanks to Vue's reactivity.
5. Connecting Vue to the Java Backend
With the Java backend serving RESTful endpoints, ensure you have a method in your Spring Boot application that provides user data.
@RestController
@RequestMapping("/api")
public class UserController {
@GetMapping("/users")
public List<User> getUsers() {
// Mock data for example
return List.of(
new User(1, "John Doe", "john@mail.com"),
new User(2, "Jane Doe", "jane@mail.com")
);
}
}
Explanation of the Java Code
-
REST API: We create a simple REST API endpoint that returns a list of users. This way, our Vue component can fetch user data directly from this endpoint.
-
Mock Data: For demonstration purposes, we return a list of mock user objects. In a real-world application, you would typically fetch this data from a database.
Best Practices for Integration
-
CORS Configuration: If you're serving your Vue frontend from a different domain than your Java backend, make sure to handle CORS (Cross-Origin Resource Sharing) properly to allow requests.
-
Error Handling: Implementing proper error handling both in the frontend (Vue) and backend (Java) is crucial for creating a robust application.
-
Build Process: Use the
npm run build
command to generate production-ready static files for Vue, which you can then serve through your Java servlet. -
Testing: Ensure that you conduct unit tests for both your backend and frontend to maintain a high standard of quality assurance.
-
Performance Optimization: Both Vue and Spring Boot have techniques and best practices to improve performance, such as lazy loading, caching responses, and minimizing API calls.
My Closing Thoughts on the Matter
Integrating Vue UI libraries like Vuetify with Java applications can drastically enhance user experiences while allowing developers to maintain clean and efficient codebases. By leveraging the strengths of both platforms, Java developers can deliver robust applications that meet modern user expectations.
For further insights, don't forget to check out the article "Struggling to Choose? Top Vue UI Libraries Simplified!".
By following this guide, you will be well-equipped to start building visually stunning Vue applications with a Java backend, effectively navigating the realms of both server-side and client-side programming. Happy coding!
Checkout our other articles