Choosing the Right UI Library for Your Java Web App
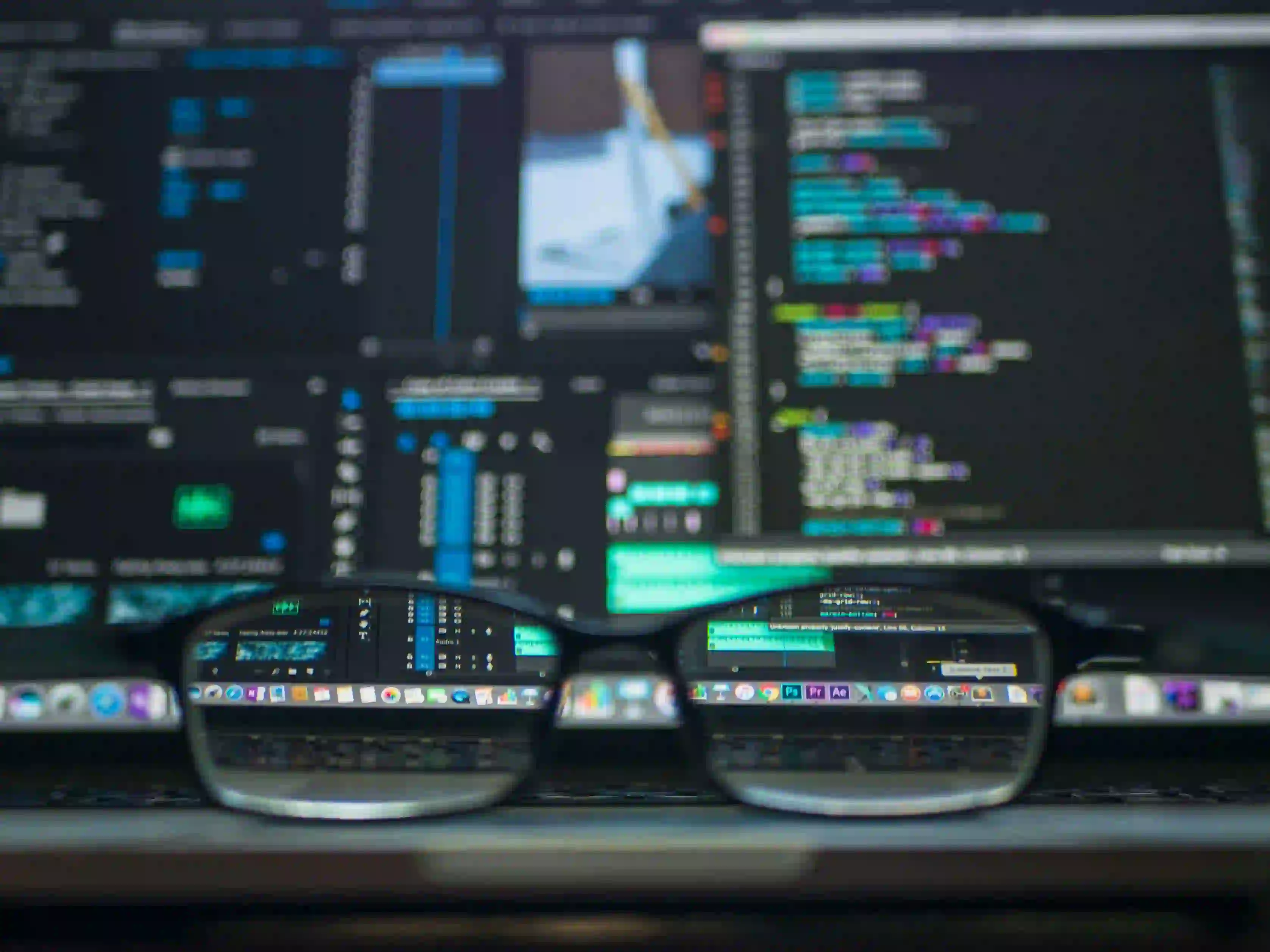
Choosing the Right UI Library for Your Java Web App
In the ever-evolving landscape of web development, selecting the right User Interface (UI) library for your Java web application is paramount. It can significantly impact both the performance of the application and the experience of the end-user. This article will guide you through the important considerations, popular UI libraries, and practical code snippets to help you navigate the complexities of this decision.
Why UI Libraries Matter
A UI library simplifies the process of creating interactive and dynamic user interfaces. By abstracting away a lot of the boilerplate code, these libraries allow developers to focus more on core functionalities rather than the intricacies of rendering UI components. Choosing the right library can enhance maintainability, increase speed, and improve the overall user experience.
Key Considerations While Choosing a UI Library
- Compatibility with Java Framework: Ensure that the UI library integrates well with the Java framework you are using (like Spring Boot, JavaServer Faces, or Vaadin).
- Performance: Look for libraries optimized for speed and efficiency. A responsive UI is critical for user satisfaction.
- Feature Set: Make sure the library offers the components you need, like charts, tables, forms, and modal dialogs.
- Community & Support: A large and active community will provide access to resources, updates, and tools.
- Documentation: Clear and detailed documentation makes it easier to learn and implement the library effectively.
Now, let’s explore some popular UI libraries that are widely used in Java applications.
Popular UI Libraries for Java Web Applications
1. Vaadin
Vaadin is a powerful Java framework that enables users to create rich web applications in Java. It allows developers to build UIs entirely in Java while accessing web technologies under the hood.
Example Code Snippet:
@Route("")
public class MainView extends VerticalLayout {
public MainView() {
Button button = new Button("Click Me", event -> {
Notification.show("Button Clicked!");
});
add(button);
}
}
Why?: This snippet creates a simple button that, when clicked, shows a notification. The Vaadin library abstracts away HTML and JavaScript complexities, allowing developers to work in a Java-centric way.
Check out more about Vaadin here.
2. JavaFX
JavaFX is the successor to Swing, providing a rich set of graphics and media packages for richer GUI applications. Though primarily used for desktop applications, JavaFX can also be leveraged for web applications with tools like JPro.
Example Code Snippet:
public class HelloWorld extends Application {
@Override
public void start(Stage stage) {
Button button = new Button("Click Me");
button.setOnAction(event ->
System.out.println("Button clicked!")
);
Scene scene = new Scene(new StackPane(button), 300, 250);
stage.setScene(scene);
stage.setTitle("Hello World");
stage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Why?: In this example, a button click triggers a print statement. JavaFX's modern API provides a more responsive interface for users compared to its predecessor, Swing.
3. JSF with PrimeFaces
JavaServer Faces (JSF) is well-known for component-based web applications. When paired with PrimeFaces, it unlocks a myriad of advanced UI components.
Example Code Snippet:
<h:form>
<p:inputText id="name" value="#{userBean.name}" />
<p:commandButton value="Submit" action="#{userBean.submit}" update="@form" />
</h:form>
Why?: This example shows how to create a simple form using JSF and PrimeFaces. The command button submits data, while the update attribute ensures that the form updates without a full page reload, providing a smoother user experience.
You can learn more about JSF and PrimeFaces components through the official PrimeFaces documentation.
Integration with Vue.js
With the growing popularity of JavaScript frameworks like Vue.js, you might consider integrating a UI library with your Java application to leverage the best of both worlds. For instance, if you're looking for a streamlined approach to select a UI library for Vue.js, the article "Struggling to Choose? Top Vue UI Libraries Simplified!" is an excellent resource. You can find it at infinitejs.com/posts/struggling-choose-top-vue-ui-libraries.
Pros of Integrating Vue.js with Java
- Enhanced Interactivity: Vue.js provides reactive components that make the UI elements dynamic and responsive.
- Rich Ecosystem: By combining Java with Vue.js, you can utilize a vast array of ready-made components and features.
- Flexibility: The modular nature of Vue.js allows you to attach or detach features and components easily.
Example Integration
If you wish to integrate Vue.js with your Java application, one common approach is to serve your Vue components through a RESTful API built with a Java framework (like Spring Boot).
@RestController
@RequestMapping("/api/users")
public class UserController {
@GetMapping
public List<User> getUsers() {
return userService.findAllUsers();
}
}
In this scenario, your Java application provides the backend data through an API, while Vue.js fetches and displays this data on the frontend.
Bringing It All Together
In conclusion, the right UI library can vastly improve the maintainability, performance, and user experience of your Java web application. Whether you choose Vaadin, JavaFX, or a combination of JSF with PrimeFaces, it’s crucial to weigh the pros and cons against your project requirements and future scalability.
With new libraries and technologies emerging regularly, keeping yourself informed is essential. Resources like the article mentioned earlier can offer guidance tailored to modern frameworks and libraries. Happy coding!