JavaFX: Creating Captivating UI for Your Visual Storytelling
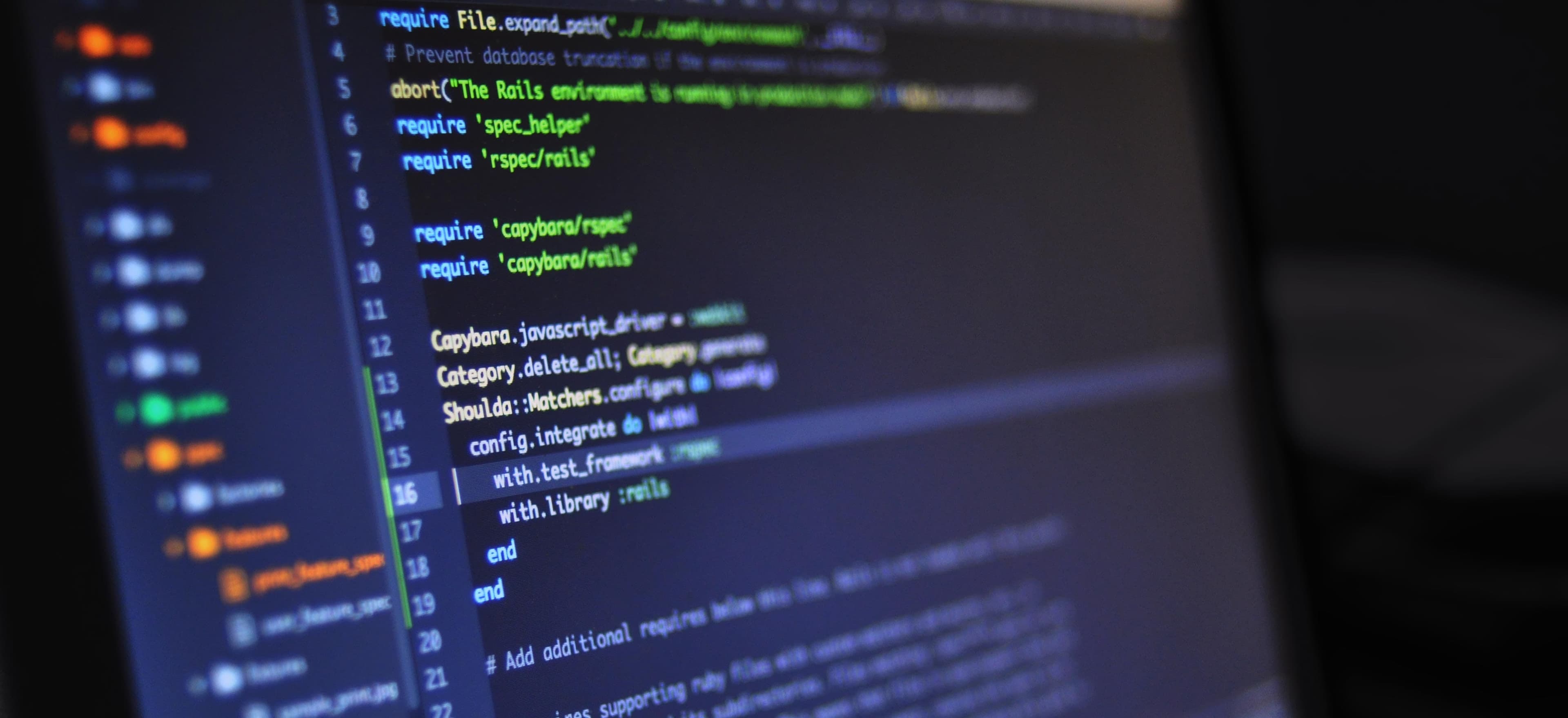
- Published on
JavaFX: Creating Captivating UI for Your Visual Storytelling
When embarking on a journey through the digital landscape, having a compelling user interface (UI) is essential for storytelling. As developers, we have the ability to create interfaces that not only function well but also capture the imagination of users. In this blog post, we'll explore how JavaFX can be leveraged to design stunning visual storytelling applications.
Along the way, we'll discuss various JavaFX components, layout management, event handling, and even delve into some code snippets to illustrate these concepts. Whether you are an experienced Java developer or just starting with JavaFX, this guide will enrich your understanding and inspire your next project.
What is JavaFX?
JavaFX is a rich client platform for Java, designed for creating modern user interfaces. It allows developers to build visually appealing applications that can run on various platforms, including desktops, browsers, and mobile devices. The power of JavaFX lies in its ability to produce rich graphics, animations, and media that can enhance any storytelling experience.
Setting Up Your JavaFX Environment
Before we dive into creating captivating UIs, ensure that you have JavaFX set up correctly in your development environment:
-
Install Java Development Kit (JDK): Download and install the latest version of the JDK from Oracle’s official website.
-
Set Up JavaFX SDK: Download the JavaFX SDK from Gluon. Follow the installation instructions provided.
-
Configure Your IDE: If you’re using IntelliJ IDEA or Eclipse, you'll need to configure the IDE to recognize JavaFX libraries.
Example of JavaFX Application Structure
Fostering an understanding of a basic JavaFX application structure is crucial. Below is a minimal code snippet that demonstrates the essentials of a JavaFX application:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class HelloWorldApp extends Application {
@Override
public void start(Stage primaryStage) {
Label helloLabel = new Label("Hello, JavaFX!");
StackPane root = new StackPane();
root.getChildren().add(helloLabel);
Scene scene = new Scene(root, 300, 200);
primaryStage.setTitle("Hello World!");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Code Commentary
- Application Class: The
HelloWorldApp
class extends theApplication
class, which is the primary entry for JavaFX applications. - Stage and Scene:
Stage
represents the primary window, whileScene
contains all the visual elements. - Layout:
StackPane
is used for layout management, allowing simple stacking of UI elements.
Creating Engaging UI with Controls and Layouts
Once you grasp the basic structure, you’ll want to enhance the user experience with various controls and layouts. JavaFX provides numerous options, including buttons, text fields, and sliders, which you can use in your applications.
Example: Adding Buttons and Event Handling
Buttons can elicit actions and lead users on a colorful journey through your application. Here’s how to add a button and handle events:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class ButtonExample extends Application {
@Override
public void start(Stage primaryStage) {
Button button = new Button("Click Me");
button.setOnAction(e -> System.out.println("Button clicked!"));
VBox vbox = new VBox(10, button);
Scene scene = new Scene(vbox, 300, 200);
primaryStage.setTitle("Button Example");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Code Commentary
- Event Handling: The
setOnAction()
method is crucial here as it binds the button to an action. This method adds interactivity to your UI, making it feel alive. - VBox Layout: Using
VBox
gives vertical spacing to UI elements, which is important for clarity in UI design.
Visual Storytelling with Graphics and Animation
To captivate users, incorporating graphics and animation can transform your application from ordinary to extraordinary. JavaFX supports 2D and 3D graphics and provides smooth animations.
Example: Simple Animation
import javafx.animation.RotateTransition;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.paint.Color;
import javafx.scene.shape.Circle;
import javafx.stage.Stage;
import javafx.util.Duration;
public class CircleAnimation extends Application {
@Override
public void start(Stage primaryStage) {
Circle circle = new Circle(50, Color.BLUE);
RotateTransition rotation = new RotateTransition(Duration.seconds(2), circle);
rotation.setByAngle(360);
rotation.setCycleCount(RotateTransition.INDEFINITE);
rotation.setAutoReverse(true);
rotation.play();
Scene scene = new Scene(circle, 300, 200);
primaryStage.setTitle("Circle Animation");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Code Commentary
- RotateTransition: This creates a simple rotation effect on the circle. It makes the UI dynamic, increasing user engagement.
- Color Customization: The circle's color can be customized to suit the theme of your storytelling, enhancing visual appeal.
Integrating Multimedia for Rich Experiences
Incorporating sound, images, and videos can enrich your application. JavaFX easily allows the use of media elements.
Example: Playing a Video
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.media.Media;
import javafx.scene.media.MediaPlayer;
import javafx.scene.media.MediaView;
import javafx.stage.Stage;
public class VideoPlayerExample extends Application {
@Override
public void start(Stage primaryStage) {
String path = "path/to/video.mp4"; // Ensure the path is correct
Media media = new Media(new File(path).toURI().toString());
MediaPlayer mediaPlayer = new MediaPlayer(media);
MediaView mediaView = new MediaView(mediaPlayer);
mediaPlayer.play();
Scene scene = new Scene(mediaView, 800, 600);
primaryStage.setTitle("Video Player");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Code Commentary
- Media and MediaPlayer: These classes facilitate video playback, which can be a captivating aspect of your visual storytelling.
- Path Handling: Ensure the media path is accurate for smooth execution, enhancing your user experience.
In Conclusion, Here is What Matters
JavaFX provides an extensive set of features to create captivating user interfaces that are integral to visual storytelling. By using its diverse controls, graphics capabilities, and multimedia integration, you can build applications that are not only functional but also engaging and visually appealing.
As you craft your digital narratives, remember that your UI can serve as the window for your audience into the story you want to tell, much like the concept of "Aurafotografie: Fenster zur Seele oder Science-Fiction?" found at auraglyphs.com/post/aurafotografie-fenster-zur-seele-oder-science-fiction.
Continue experimenting and pushing the boundaries of what’s possible with JavaFX to create visually stunning applications that capture the essence of your storytelling! Happy coding!
Checkout our other articles