Capturing the Soul: Java Frameworks for Digital Photography
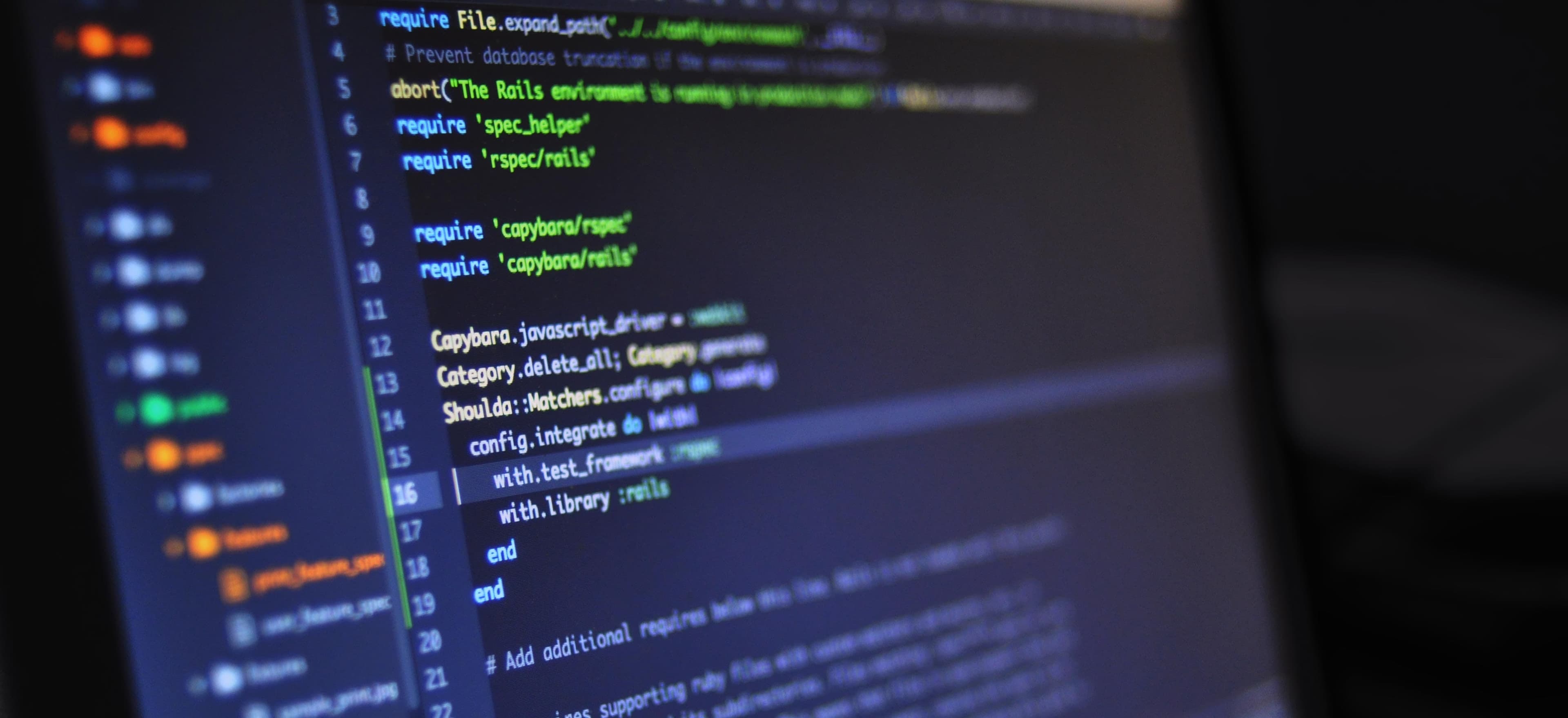
- Published on
Capturing the Soul: Java Frameworks for Digital Photography
In the ever-evolving world of digital photography, technology plays a crucial role in how we capture and manipulate images. The Java programming language has established itself as a reliable tool for developing applications that can enhance digital photography workflows. This blog post delves into popular Java frameworks designed for photography applications and discusses their unique features, benefits, and best practices, particularly with respect to the Java 8 and beyond ecosystem.
Understanding Java's Role in Digital Photography
Java is a versatile programming language known for its portability, object-oriented structure, and vast ecosystem of libraries and frameworks. Its ability to run on any platform that supports the Java Virtual Machine (JVM) makes it ideal for developing cross-platform applications, including those tailored for digital photographers. Whether you're looking to create image editing software, manage photo metadata, or analyze images, Java provides numerous libraries to get the job done.
Popular Java Frameworks for Digital Photography
1. Java Image Processing Cookbook
The Java Image Processing Cookbook offers various techniques for manipulating images using Java's built-in libraries. From simple tasks such as resizing and rotating images to more advanced techniques like histogram equalization, this collection of recipes provides an excellent architecture for creating photography applications.
Example: Resizing an Image
import javax.imageio.ImageIO;
import java.awt.*;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
public class ImageResizer {
public BufferedImage resizeImage(File file, int newWidth, int newHeight) throws IOException {
BufferedImage originalImage = ImageIO.read(file);
BufferedImage resizedImage = new BufferedImage(newWidth, newHeight, BufferedImage.TYPE_INT_ARGB);
Graphics2D g = resizedImage.createGraphics();
g.drawImage(originalImage, 0, 0, newWidth, newHeight, null);
g.dispose();
return resizedImage;
}
}
In this code snippet, we import necessary libraries, create a resizeImage
method that reads an image file, and resize it to new dimensions. The benefits of resizing include optimizing image load times and improving performance in photography applications.
2. OpenCV for Java
OpenCV (Open Source Computer Vision Library) is an open-source computer vision and machine learning software library. It has native support for Java, allowing developers to perform complex image analysis tasks. From face detection to image segmentation and optical flow calculations, OpenCV offers a wide range of functionalities suitable for advanced photography applications.
Example: Face Detection
import org.opencv.core.*;
import org.opencv.imgcodecs.Imgcodecs;
import org.opencv.objdetect.CascadeClassifier;
public class FaceDetection {
static { System.loadLibrary(Core.NATIVE_LIBRARY_NAME); }
public void detectFaces(String imagePath) {
Mat image = Imgcodecs.imread(imagePath);
CascadeClassifier faceDetector = new CascadeClassifier("haarcascade_frontalface_default.xml");
MatOfRect faceDetections = new MatOfRect();
faceDetector.detectMultiScale(image, faceDetections);
for (Rect rect : faceDetections.toArray()) {
Imgproc.rectangle(image, new Point(rect.x, rect.y),
new Point(rect.x + rect.width, rect.y + rect.height),
new Scalar(0, 255, 0));
}
Imgcodecs.imwrite("face-detected.png", image);
}
}
In this example, we use the OpenCV library for face detection. After loading the image, we apply a pre-trained model (haarcascade_frontalface_default.xml
) to identify faces. The detection can be beneficial for photographers in grouping shots or identifying key individuals in images.
3. JMagick
JMagick is a Java wrapper for the well-known ImageMagick library, which is widely used for creating, editing, and converting bitmap images. With JMagick, developers can manipulate images with ease, allowing for rapid development of applications that require complex image transformations.
Example: Converting Image Formats
import magick.*;
public class ImageConverter {
public void convertImage(String inputPath, String outputPath) throws MagickException {
MagickImage image = new MagickImage(inputPath);
image.writeImage(new ImageInfo(outputPath));
image.destroyImages();
}
}
This small yet effective code snippet shows how to convert images from one format to another. The benefit of using JMagick lies in its powerful image manipulation capabilities, which can simplify bulk-processing tasks for digital photographers.
Integrating Metadata Handling
An often-overlooked aspect of photography is managing image metadata. Photographers typically embed important information within image files, such as Exposure time, F-stop, or camera model.
Apache Commons Imaging
Apache Commons Imaging provides a robust way to read and write image metadata across different formats. It helps developers access this critical information without needing to dive deep into the specifics of each image format.
Example: Reading Image Metadata
import org.apache.commons.imaging.*;
import org.apache.commons.imaging.formats.exif.ExifImageMetadata;
import java.io.File;
public class MetadataReader {
public void readMetadata(String imagePath) {
try {
File file = new File(imagePath);
ExifImageMetadata metadata = (ExifImageMetadata) Imaging.getMetadata(file);
System.out.println(metadata);
} catch (ImageReadException | IOException e) {
e.printStackTrace();
}
}
}
In this example, we read metadata from an image file. The ability to access metadata can elevate a photographer’s workflow, allowing them to make better-informed decisions based on previously captured settings.
My Closing Thoughts on the Matter: Bringing Photography and Technology Together
The world of digital photography is rich with possibilities when empowered by programming languages like Java. By utilizing frameworks such as the Java Image Processing Cookbook, OpenCV for Java, JMagick, and Apache Commons Imaging, developers can create robust applications that aid photographers in their creative pursuits.
As we navigate through advancements in photography and technology, it's essential to explore how different frameworks can enhance our workflows. Tools like those mentioned in this article help you not only elevate your work but allow you to capture the essence of subjects, akin to the intriguing concept of "Aural Photography" discussed in the existing article "Aurafotografie: Fenster zur Seele oder Science-Fiction?" available at auraglyphs.com/post/aurafotografie-fenster-zur-seele-oder-science-fiction.
Reflecting on the marriage of technical skill and artistic talent, Java's role in this realm is not just beneficial; it's fundamental. Whether you're a seasoned developer or an aspiring photographer, there's much to explore in the intersection of these two worlds.
Checkout our other articles