Surviving Bugs in Java: The Right Debugging Strategy
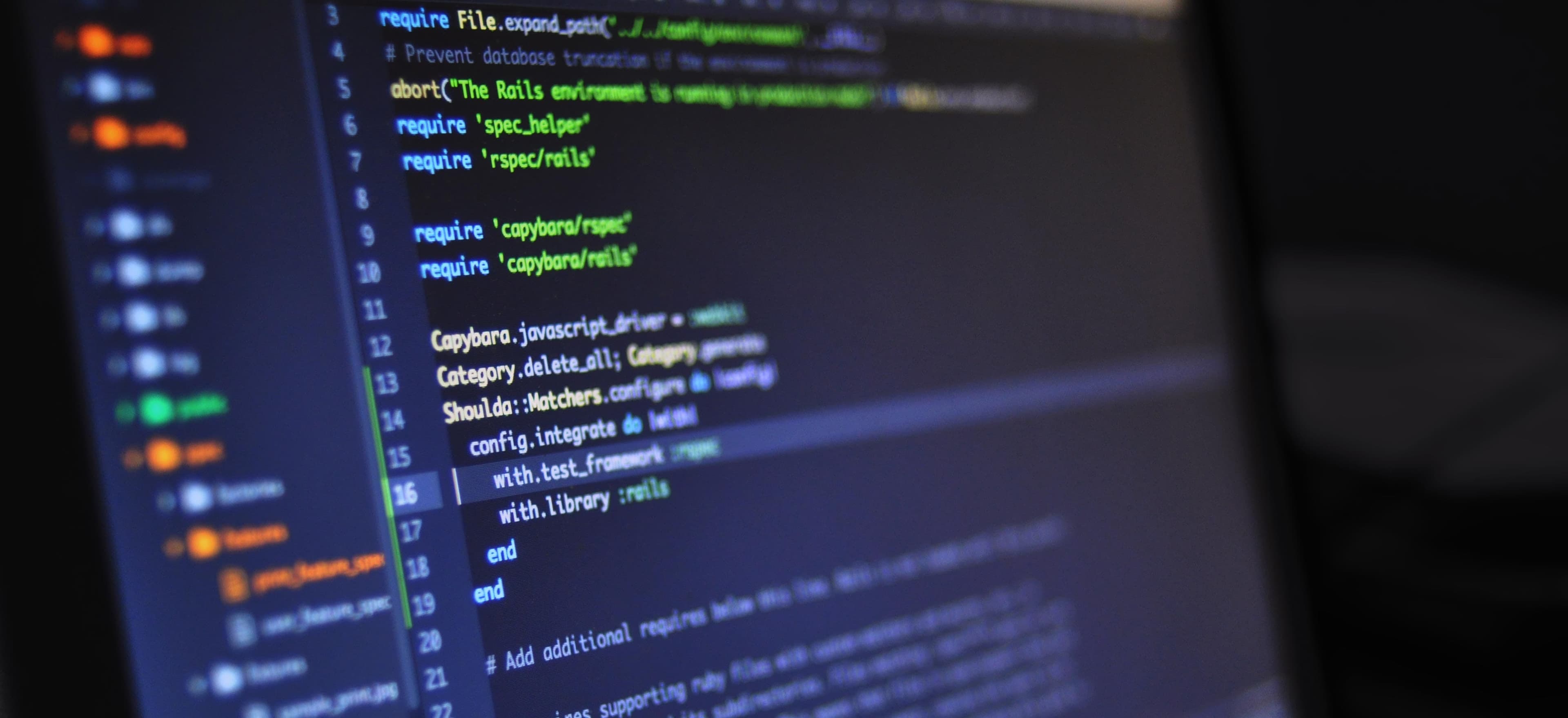
- Published on
Surviving Bugs in Java: The Right Debugging Strategy
Debugging is an essential skill for any Java developer. Whether you are a seasoned programmer or just starting your coding journey, understanding the nuances of debugging can save you time, frustration, and ultimately, improve the quality of your software. In this blog post, we will explore different debugging strategies in Java and what makes each one effective. We will also discuss best practices, tools, and techniques that can elevate your debugging skills.
Understanding the Importance of Debugging
To start, it's crucial to comprehend why debugging is not just about finding and fixing bugs. Debugging is a way to enhance the quality of your code and ensure your application behaves as expected.
When we think about debugging in terms of survival, we are reminded of the article "Bug-In vs. Bug-Out: Making the Right Survival Choice". In the same spirit, debugging requires you to decide on the best approach—whether to "bug in" and sharpen your current code or to "bug out" and refactor or even rewrite segments of your application. You can check out the article here for more insights.
Common Bugs in Java
Before discussing strategies, let's briefly cover the types of bugs you might encounter in Java:
-
Syntax Errors: These errors are due to incorrect syntax that violates the language's rules. They are usually caught by the compiler.
-
Runtime Exceptions: These occur during the execution of your program. Example: accessing an array out of bounds.
-
Logic Errors: These occur when the program runs without crashing but produces incorrect results, often due to an error in the algorithm.
Code Snippet: Syntax Error Example
public class Main {
public static void main(String[] args {
System.out.println("Hello, World!")
}
}
Commentary: In this example, a missing parenthesis and semicolon will lead to syntax errors. The Java compiler won't execute the program until all syntax issues are resolved.
Debugging Strategies in Java
1. Code Review and Pair Programming
One traditional yet effective method is undergoing code reviews or working with a peer (pair programming). This serves as a double check, often catching issues one might overlook.
Benefits:
- Fresh Perspective: Fresh eyes can spot bugs.
- Knowledge Sharing: You learn from each other.
2. Print Statements
Often the simplest debugging technique involves placing print statements throughout your code. While old-fashioned, this method can break down complicated logic.
Example:
public class Calculator {
public int add(int a, int b) {
System.out.println("Adding " + a + " and " + b); // Debugging Statement
return a + b;
}
}
Commentary: The print statement here helps you track the values at a specific stage. You can replace it with a logging library later for better maintainability.
3. Using a Debugger Tool
Integrated Development Environments (IDEs) like IntelliJ IDEA or Eclipse provide powerful debugging tools. You can step through your code, inspect variables, or set breakpoints.
A Simple Walkthrough:
- Set Breakpoint: Click on the gutter next to the line number.
- Run Debug Mode: Start your program in debug mode.
- Step Into/Over: Control the flow to see how variables change.
Example Use Case:
If you have the following simple loop that calculates a sum:
public class SumCalculator {
public int calculateSum(int n) {
int sum = 0;
for (int i = 0; i <= n; i++) {
sum += i;
}
return sum;
}
}
Debugging Strategy: Place a breakpoint at sum += i;
and step through the code. Monitor how i
and sum
change with each iteration.
4. Unit Testing
Writing unit tests not only helps in validating your code but can also pinpoint the location of bugs. Using testing frameworks like JUnit makes your code robust and bug-resistant.
Example Test Case:
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class CalculatorTest {
@Test
public void testAdd() {
Calculator calculator = new Calculator();
assertEquals("Adding 1 + 2 should equal 3", 3, calculator.add(1, 2));
}
}
Commentary: Here, the JUnit test case assists in ensuring that your add
method behaves as expected. If it fails, it quickly signals a problem.
5. Log Analysis
Logging frameworks like Log4j or SLF4J allow for a more refined logging mechanism than print statements. Logs can be set at different levels (info, debug, error), giving you granular control over what to record.
Example: Logging a Method Entry and Exit
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class UserService {
private static final Logger logger = LoggerFactory.getLogger(UserService.class);
public void registerUser(String username) {
logger.info("Entering registerUser method with username: {}", username);
// Registration logic here...
logger.info("Exiting registerUser method");
}
}
Commentary: Using logs here will provide insights into the method's flow without affecting the performance significantly.
6. Static Code Analysis
Tools like SonarQube or Checkstyle analyze your code for potential errors or bad practices without executing the program. It can catch issues early in the development process.
Why Use Static Analysis?
- Early bug detection: Catch potential errors before runtime.
- Code quality improvement: Enforce coding standards.
Closing the Chapter
In the realm of Java programming, debugging is a skill that every developer must possess. By employing strategies such as code reviews, using a debugger, incorporating unit tests, and leveraging logging, you can overcome the challenges of debugging effectively.
Ultimately, just like the insights from the article "Bug-In vs. Bug-Out: Making the Right Survival Choice", you must assess your situation and approach each debugging scenario with the right mindset and tools.
As you continue your journey in Java programming, these debugging strategies can become invaluable. They not only save time but also enhance the code quality and maintainability of your applications.
For further reading, consider exploring the importance of unit testing and best practices in this JUnit Guide.
Happy coding and debugging!
Checkout our other articles