Surviving Java Development: Bug-In vs. Bug-Out Strategies
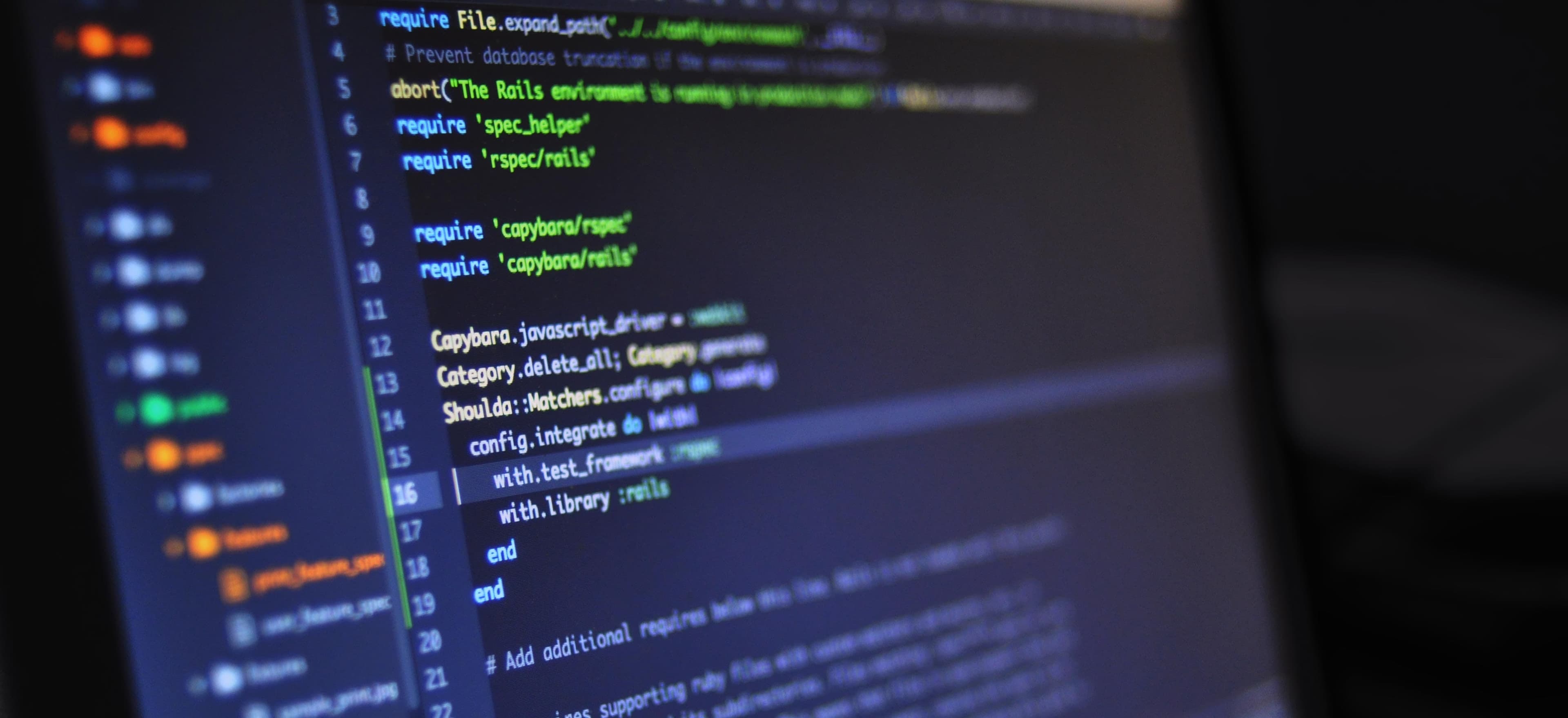
- Published on
Surviving Java Development: Bug-In vs. Bug-Out Strategies
The world of Java development is an ever-evolving landscape filled with challenges, unexpected bugs, and the thrill of solving intricate problems. Much like survival strategies in uncertain environments, developers must choose between two contrasting approaches when it comes to handling bugs in their Java applications: Bug-In and Bug-Out strategies.
In this blog post, we will delve deep into these strategies, exploring their significance, code best practices, and lessons learned from the real world. Let's get started!
Understanding the Bug-In Strategy
What is Bug-In?
The Bug-In strategy is akin to fortifying your position. It encourages developers to tackle the issue head-on, fixing bugs with a local approach and striving to improve the code incrementally. It emphasizes improving code quality, writing thorough tests, and ensuring robust deployment.
When Should You Use Bug-In?
You should consider this strategy when:
- You face minor bugs that can be easily addressed without extensive refactoring.
- The development environment is stable, and there is a high likelihood that the fix will succeed.
- You have a strong testing framework in place that allows for thorough validations.
Example of Bug-In Approach
Let’s look at a common bug: a NullPointerException in Java. Here’s a simple example to illustrate the Bug-In approach:
public class User {
private String username;
public User(String username) {
this.username = username;
}
public String getUsername() {
return username;
}
}
public class UserService {
public void printUserDetails(User user) {
// Checking for null before accessing methods
if (user != null) {
System.out.println("Username: " + user.getUsername());
} else {
System.out.println("User is null");
}
}
}
Why This Code Works?
- Null Check: By checking if the
user
object is null before accessing it, we prevent a NullPointerException. - Maintainability: This method keeps the code understandable and maintainable, adhering to Java best practices.
- User Feedback: Informing the user when the object is null provides a better experience and aids in debugging.
Bug-In Best Practices
- Incremental fixes over wholesale changes help maintain stability.
- Refactoring should occur as needed, not just when convenient.
- Always employ test-driven development (TDD) principles to catch issues early.
Understanding the Bug-Out Strategy
What is Bug-Out?
Bug-Out implies evacuating from a problematic scenario. This proactive approach entails acknowledging the need to abandon the current code or solution due to pervasive issues, allowing teams to pivot without getting bogged down.
When Should You Use Bug-Out?
This strategy is a go-to when:
- A pervasive and consistent pattern of bugs appears, stemming from the existing architecture.
- New requirements dictate a significant overhaul of the system.
- Development velocity is hampered by legacy issues that outweigh the benefits of short-term fixes.
Example of Bug-Out Approach
In the case of an outdated application that consistently conflicts with new libraries, here's how you might consider Bug-Out instead of persisting in fixing the old code:
// Existing code with many legacy issues
public class LegacyUserService {
public void displayUserData() {
// Legacy code that is rapidly becoming unstable
...
}
}
// New refactored UserService using modern Java features
import java.util.Optional;
public class UserService {
public void displayUserData(Optional<User> user) {
user.ifPresentOrElse(
u -> System.out.println("Username: " + u.getUsername()),
() -> System.out.println("No user found")
);
}
}
Why This Code Works?
- Using Optional: This modern Java feature encourages safe handling of potentially absent values.
- Eliminating Legacy Issues: By rewriting parts of the application, the team avoids well-known pitfalls associated with old code.
- Improved Readability: The use of lambda expressions makes the code concise and clearer.
Bug-Out Best Practices
- Don’t fear rewrites. Sometimes a fresh start is more efficient than patchwork fixes.
- Utilize pair programming during re-architecture for shared knowledge and enhanced quality.
- Document the process so everyone understands why significant changes were made.
Comparing Bug-In and Bug-Out: Choosing Your Path
The choice between Bug-In and Bug-Out strategies is pivotal and should align with the situation at hand.
- When Resources are Limited: If you find yourself in a resource-constrained environment, Bug-In may seem more viable as it often requires less investment in time and manpower.
- When Ethical Decisions Must be Made: Consider your company's direction and future maintenance when deciding to Bug-Out. If legacy support burdens outweigh benefits, it may be time to move forward.
Gauging Long-Term Impact
Teams must also assess the potential long-term impact of their chosen strategy. For organic, steady growth of a software product, the Bug-In strategy may yield favorable results when integrated with Continuous Integration and Continuous Deployment practices. Conversely, in a rapidly changing landscape, embracing a Bug-Out strategy could promote agility and adaptability.
You can draw parallels with survival strategies, as highlighted in the article Bug-In vs. Bug-Out: Making the Right Survival Choice. The essence is survival—not least as a developer facing the inevitable bugs that come along.
A Final Look
Navigating the developer landscape calls for dexterity in handling bugs and challenges. Choosing between Bug-In and Bug-Out strategies is not just a tactical decision but a reflection of the team’s philosophy towards problem-solving.
Emphasizing best practices and understanding the nature of your code and environment will help prepare you for the inevitable challenges that lie ahead in your Java development journey. Whether you fortify your position with Bug-In or strategically withdraw with Bug-Out, remember to gather lessons from each approach. Meanwhile, stay prepared, as your coding environment might require a swift change at any moment.
By fostering a culture of innovation, understanding, and adaptability, you can confidently tackle any bug that dares cross your path. Happy coding!
Checkout our other articles