Managing URL Stability in Java-Based Preview Environments
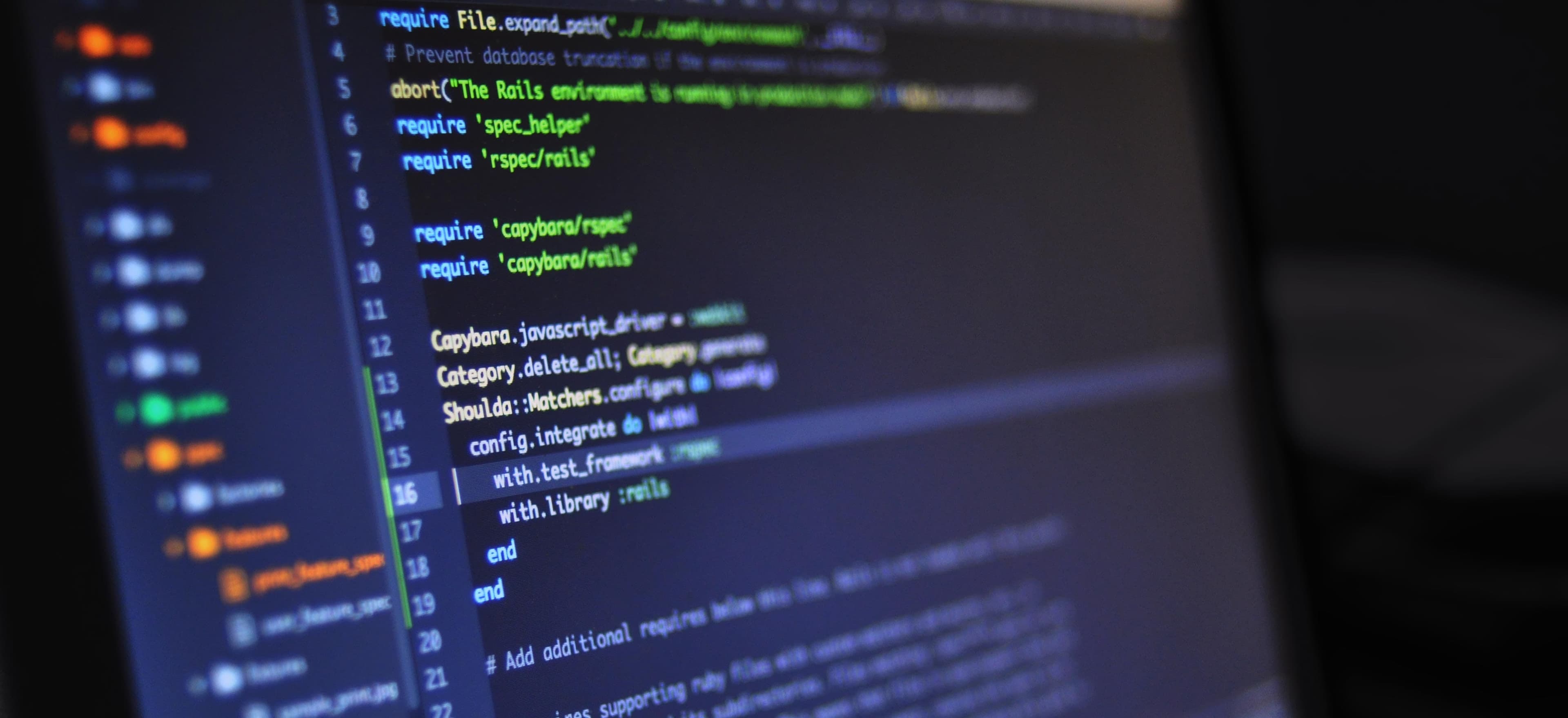
- Published on
Managing URL Stability in Java-Based Preview Environments
In modern software development, especially in agile environments, managing URL stability for various preview environments has become a significant challenge. These environments allow teams to test features before they go live, making them crucial in the CI/CD pipeline. However, as highlighted in the article The Problem with Auto-Generated URLs for Preview Environments, unstable URLs can lead to a myriad of issues, including broken links and difficulty in accessing the intended resources. In this blog post, we will explore how to manage URL stability effectively in Java-based environments.
Understanding URL Management
When discussing URL management, we primarily focus on how URLs are structured, created, and maintained within a web application. Stable URLs ensure that end-users, QA engineers, and developers can consistently access the same resources without hiccups. This becomes even more relevant in preview environments, where URLs can often change due to the dynamic nature of builds and releases.
Why URL Stability Matters
- User Experience: Inconsistent URLs can lead to frustration for users who need to test features.
- Integration: External services or teams relying on stable URLs may face disruptions, affecting overall productivity.
- Documentation: Stable URLs ease the process of documenting features, as developers don’t have to constantly update links.
To address these issues, we must employ strategies that prioritize static URL structures.
Designing Stable URLs
1. Use Meaningful Paths
Instead of auto-generating URLs with random strings or hashes, create meaningful paths that reflect the content. For example:
public class ApiUrlManager {
public String generateUrl(String feature) {
// Use meaningful path based on feature
return "/preview/" + feature.replaceAll(" ", "-").toLowerCase();
}
}
In this code snippet, we create a URL path that includes the feature name. This enhances readability and makes it easier to understand what the URL entails.
2. Versioning
Versioning your API URLs can help maintain stability. Changes to the API structure should not disrupt existing URLs. This approach can be done in various ways, but one common method is to include a version parameter in the URL.
public class ApiUrlManager {
private static final String VERSION = "v1";
public String generateVersionedUrl(String feature) {
return "/" + VERSION + "/preview/" + feature.replaceAll(" ", "-").toLowerCase();
}
}
By adding a version number, developers can iteratively work on new features without breaking existing links. Users can continue using the previous version while benefiting from enhancements or modifications made in the new version.
3. Configuration Files
For Java applications, externalizing URL paths into configuration files can aid in managing them. For instance, using a properties
file can allow easy updates without delving into the codebase.
config.properties
preview.base.url=/preview
Java Code
import java.io.FileReader;
import java.io.IOException;
import java.util.Properties;
public class ConfigurableUrlManager {
private String baseUrl;
public ConfigurableUrlManager() {
Properties properties = new Properties();
try {
properties.load(new FileReader("config.properties"));
baseUrl = properties.getProperty("preview.base.url");
} catch (IOException e) {
e.printStackTrace();
}
}
public String generateUrl(String feature) {
return baseUrl + "/" + feature.replaceAll(" ", "-").toLowerCase();
}
}
This separation allows different environments (development, testing, production) to have distinct configurations without changing the core codebase. If the base URL changes, you only need to modify the config.properties
file.
Implementing Redirection and Aliasing
In cases where a URL needs to change, implementing redirection can be a seamless way to maintain access to existing content. Java-based frameworks like Spring offer capabilities for URL redirection.
Example with Spring Boot
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
@RequestMapping("/old-feature")
public class UrlRedirectionController {
@GetMapping
public String redirectToNewFeature() {
return "redirect:/new-feature";
}
}
This redirection helps ensure that even if URLs change, users accessing old URLs are automatically sent to the new locations. This is critical for maintaining user trust and operational continuity.
Final Thoughts
Managing URL stability in Java-based preview environments is crucial. Using meaningful paths, implementing versioning, utilizing external configuration files, and leveraging redirection can all significantly enhance URL management. These steps not only improve user experience but also streamline the development and testing process.
As technologies evolve and the software development landscape shifts, it's vital to remain proactive about URL management. Staying informed and implementing best practices will empower teams to navigate the complexities of agile software development trends efficiently.
For further insights on the challenges associated with auto-generated URLs in preview environments, check out the article The Problem with Auto-Generated URLs for Preview Environments.
By implementing these URL management strategies, teams can enhance stability, improve collaboration, and ultimately create a more effective software development environment. Happy coding!