Navigating Auto-Generated URLs in Java Web Apps
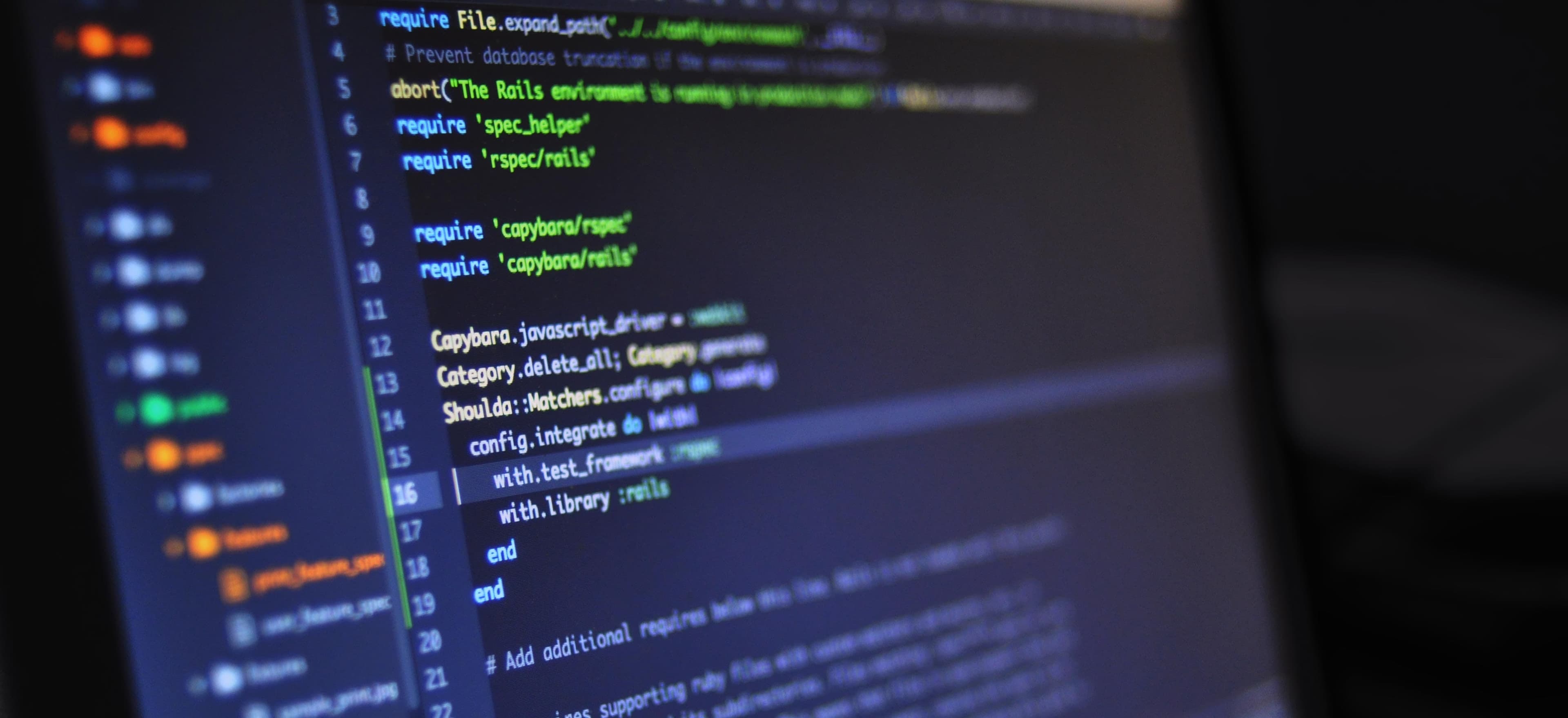
- Published on
Navigating Auto-Generated URLs in Java Web Apps
Web applications have become an essential part of modern software architecture, and with them comes the need for intelligent URL management. In Java web applications, the need for well-structured and meaningful URLs cannot be overstated. This post will delve into the world of auto-generated URLs, discussing their implications, advantages, and potential pitfalls. We will also link to a relevant article titled "The Problem with Auto-Generated URLs for Preview Environments" to provide further insights.
Understanding Auto-Generated URLs
Auto-generated URLs are typically created dynamically based on various parameters. For example, when a new resource is created in an online shopping application, the URL might automatically incorporate the product ID, name, and category.
Example of an Auto-Generated URL
Consider a simple product page URL like this:
https://example.com/products/12345
Here, "12345" is the product ID which serves both as an identifier and part of the URL structure. While this method simplifies routing, it poses challenges related to readability, maintainability, and search engine optimization (SEO).
The Need for Meaningful URLs
Meaningful URLs contribute significantly to the usability of your web app. They assist users in understanding the content they are about to engage with, thus enhancing user experience. Furthermore, meaningful URLs are favored by search engines, improving your application's visibility.
Why Meaningful URLs Matter
-
User Engagement: URLs that convey information can entice users to click. For instance, a URL like
https://example.com/products/fancy-watch
is likely to attract more clicks thanhttps://example.com/products/12345
. -
SEO Benefits: Search engines prioritize URLs that are descriptive. This can lead to better indexation and ranking.
-
Shareability: Users are more inclined to share meaningful URLs, spreading awareness of your site.
Custom URL Generation in Java
To address the challenges associated with auto-generated URLs, we can implement a custom URL generation strategy in Java. Here's a straightforward implementation.
Code Snippet: Custom URL Generator
public class Product {
private int id;
private String name;
private String category;
public Product(int id, String name, String category) {
this.id = id;
this.name = name;
this.category = category;
}
public String generateUrl() {
// Generate a slug from the product name for a cleaner URL
String slug = name.toLowerCase().replaceAll("\\s+", "-");
return String.format("https://example.com/products/%d-%s", id, slug);
}
}
Commentary
In the above code, we define a Product
class with a method to generate a user-friendly URL. The name
attribute is transformed into a slug, which replaces spaces with hyphens, creating a clean and readable URL. This method enhances both user experience and SEO.
Strategy for Handling Preview Environments
In development, auto-generated URLs can create inconsistencies and potential issues in preview environments. Static URLs can lead to unexpected behavior, especially when team members need to review different versions.
Reference to Existing Article
In the article titled "The Problem with Auto-Generated URLs for Preview Environments", the authors discuss how these URLs can obscure the actual state of a web application. Preview environments often introduce numerous versions, making it difficult to track changes.
To mitigate these issues, consider implementing unique identifiers or tags in your URLs that specify the environment. For instance, a URL could look like this:
https://example.com/dev/products/12345
Enhancing URL Readability with Environment Tags
Here is how you could extend the earlier Product
class to incorporate environment tags:
public class Product {
private int id;
private String name;
private String category;
private String environment;
public Product(int id, String name, String category, String environment) {
this.id = id;
this.name = name;
this.category = category;
this.environment = environment;
}
public String generateUrl() {
String slug = name.toLowerCase().replaceAll("\\s+", "-");
return String.format("https://example.com/%s/products/%d-%s", environment, id, slug);
}
}
Commentary
By adding an environment
parameter, you can tailor the URL to reflect the context in which it is generated. This method ensures clarity and usability, which is critical during development and testing.
Best Practices for URL Management
1. Keep URLs Short and Simple
A best practice is to keep URLs concise yet descriptive. Avoid excessive parameters or unnecessary complexity.
2. Use Hyphens Instead of Underscores
Search engines treat hyphens as word separators, which makes URLs using them easier to read and comprehend.
3. Implement Redirects
When changing URLs, ensure to set up 301 redirects from old URLs to new. This prevents 404 errors and maintains SEO rankings.
4. Manage Versioning
Versioning your APIs and web application routes (like the staging and production examples mentioned) is crucial for maintenance and future development.
Bringing It All Together
Auto-generated URLs in Java web applications offer an easy routing mechanism but can lead to problems if not handled wisely. By implementing strategies for meaningful URLs and understanding the implications of auto-generation, developers can create more user-friendly and SEO-optimized applications.
To avoid the pitfalls associated with auto-generated URLs, especially in environments with multiple versions, refer to the article "The Problem with Auto-Generated URLs for Preview Environments". Keeping your URLs manageable will enhance user experience, facilitate easier navigation, and preserve potential SEO benefits.
Navigating the intricacies of web app URL generation is key to launching a successful product. With proper techniques and considerations in place, you can create a more effective web application.