Streamlining Java Apps: Optimize Docker Images for Speed
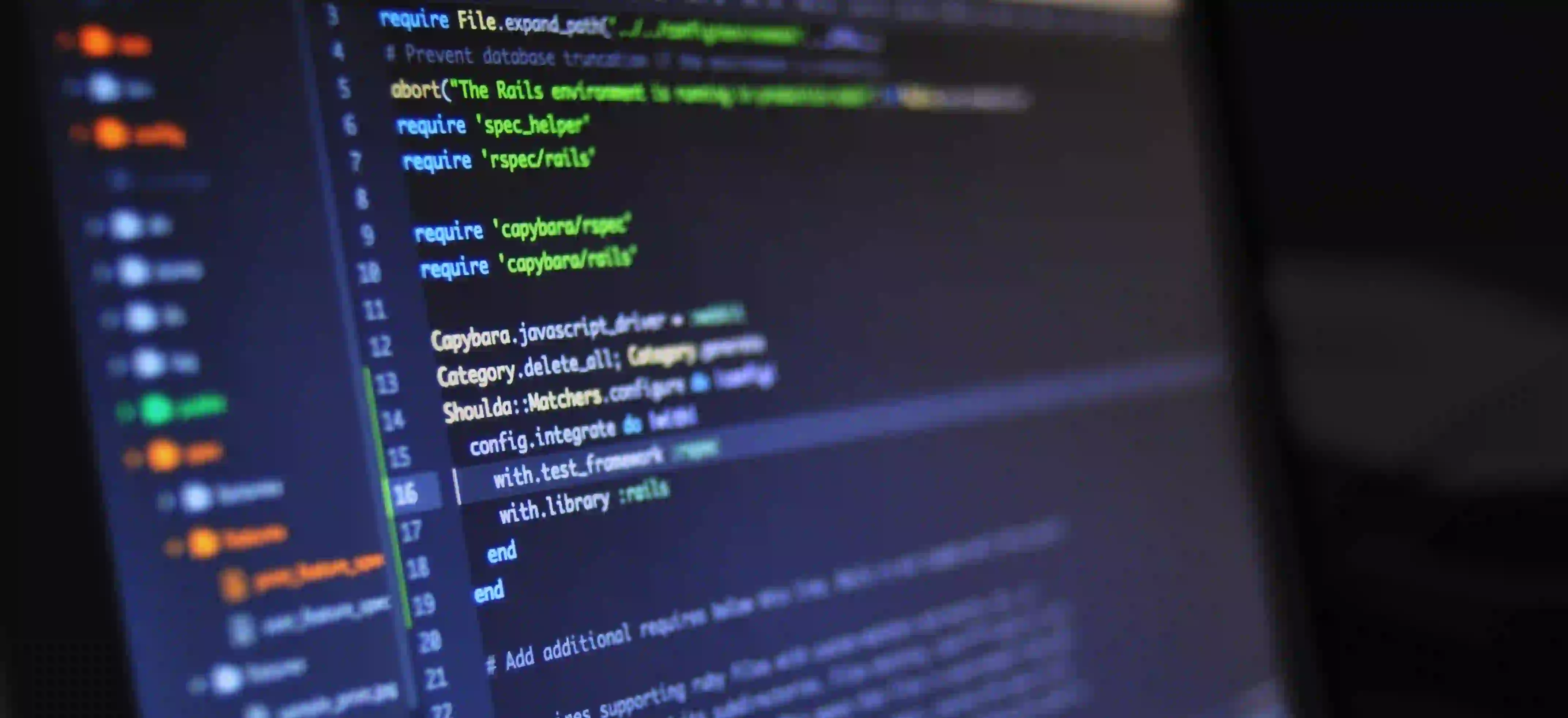
Streamlining Java Apps: Optimize Docker Images for Speed
In the world of modern software development, performance and efficiency are paramount. With the rise of containerization, developers are increasingly turning to Docker to streamline application deployment. However, a common challenge arises when it comes to optimizing the size of Docker images, especially for Java applications. This guide will walk you through techniques for optimizing your Docker images for Java apps, ensuring faster deployment and improved performance.
Understanding Docker Basics
Docker simplifies the process of creating, deploying, and managing applications in lightweight containers. A Docker image is a snapshot of your application and its environment, while a container is a running instance of that image.
However, larger Docker images can lead to slower builds, longer deployment times, and increased resource usage. Hence, optimizing your Docker images is a crucial step in the development lifecycle.
The Importance of Image Optimization
Optimizing Docker images is essential for several reasons:
- Faster Deployments: Smaller images can be pulled from the registry more quickly.
- Reduced Bandwidth: Smaller images consume less bandwidth during transfers.
- Lower Resource Consumption: Less disk space and memory usage during the runtime.
Techniques for Docker Image Optimization
Let’s dive into some effective techniques for streamlining your Java applications by optimizing Docker images.
1. Use a Multi-Stage Build
Multi-stage builds allow you to separate the build environment from the runtime environment. This helps in reducing the final image size by excluding unnecessary files from the production image.
# First stage: Build
FROM maven:3.8.1-openjdk-11 AS builder
WORKDIR /app
COPY pom.xml .
COPY src ./src
RUN mvn clean package -DskipTests
# Second stage: Run
FROM openjdk:11-jre-slim
WORKDIR /app
COPY --from=builder /app/target/myapp.jar myapp.jar
ENTRYPOINT ["java", "-jar", "myapp.jar"]
Why Multi-Stage Builds? Using a multi-stage build reduces the final image size by excluding build tools and dependencies not needed at runtime. The base image for the runtime (openjdk:11-jre-slim) is lightweight, which directly contributes to a faster deployment.
2. Leverage Base Images Wisely
Choosing the right base image can impact the size and efficiency of your Docker image. Using a minimal base image, such as alpine
, can drastically reduce the image size.
FROM adoptopenjdk:11-jre-hotspot
COPY target/myapp.jar /usr/local/myapp/myapp.jar
CMD ["java", "-jar", "/usr/local/myapp/myapp.jar"]
Why a Minimal Base Image? Alpine images are small, lean, and designed to reduce overall size. When you use it in your Dockerfile, you benefit from quicker build times and reduced resource consumption.
3. Clean Up Unnecessary Files
Docker images can accumulate unnecessary files over time, some of which may be included from the build process. Cleaning up these files is crucial to optimizing your images.
RUN apt-get update && apt-get install -y \
package_name \
&& rm -rf /var/lib/apt/lists/*
Why Clean Up? Removing temporary files can significantly reduce the size of your image. Every step in your Dockerfile contributes to the final image; keeping it clean translates to better optimization.
4. Minimize Layer Count
Each command in a Dockerfile creates a new layer. Thus, combining commands into fewer RUN statements can help minimize the number of layers and, consequently, the image size.
RUN apt-get update && apt-get install -y \
package_name_1 \
package_name_2 \
&& rm -rf /var/lib/apt/lists/*
Why Minimize Layers? Fewer layers lead to a smaller image size. Each Docker layer encapsulates file changes and is stored as a separate entity, leading to larger images with unnecessary bloat.
5. Configure .dockerignore
Using a .dockerignore
file helps in excluding files and folders from the build context that aren't needed for your Docker image.
target/
*.war
*.jar
*.log
*.iml
*.ds_store
Why Use .dockerignore? Just as a .gitignore
file excludes files from a Git repository, .dockerignore
prevents unnecessary files from being added to the Docker image, further optimizing its size.
Java Application Optimization Tips
In addition to Docker image optimization, it's essential to consider the efficiency of your Java application itself.
1. Optimize Your Dependencies
Minimize the number of libraries your application relies on. Opt for lighter alternatives when possible.
2. Use ProGuard or Similar Tools
Tools like ProGuard can be used to shrink, optimize, and obfuscate your code, thereby improving startup times and reducing memory usage.
3. JVM Optimization
Running your Java application with the right JVM options can lead to improved performance. Experiment with parameters such as heap size, garbage collection strategies, and more to suit your use case.
Closing Remarks
Optimizing Docker images for your Java applications is not just a matter of size; it’s about improving the entire development lifecycle, from build times to deployment speeds. By employing multi-stage builds, choosing the right base images, cleaning up unnecessary files, and minimizing layers, you can significantly enhance the performance of your Java applications.
For further reading and advanced optimization techniques, consider checking out the article titled Mastering Scratch: Trim Docker Images for Faster Deployment. This article provides additional insights that can complement your understanding of Docker image optimization.
By prioritizing the principles outlined in this article, you position your Java applications for success in a competitive and demanding tech landscape. Happy coding and dockerizing!