Optimize Java Applications: Trim Docker Images for Speed
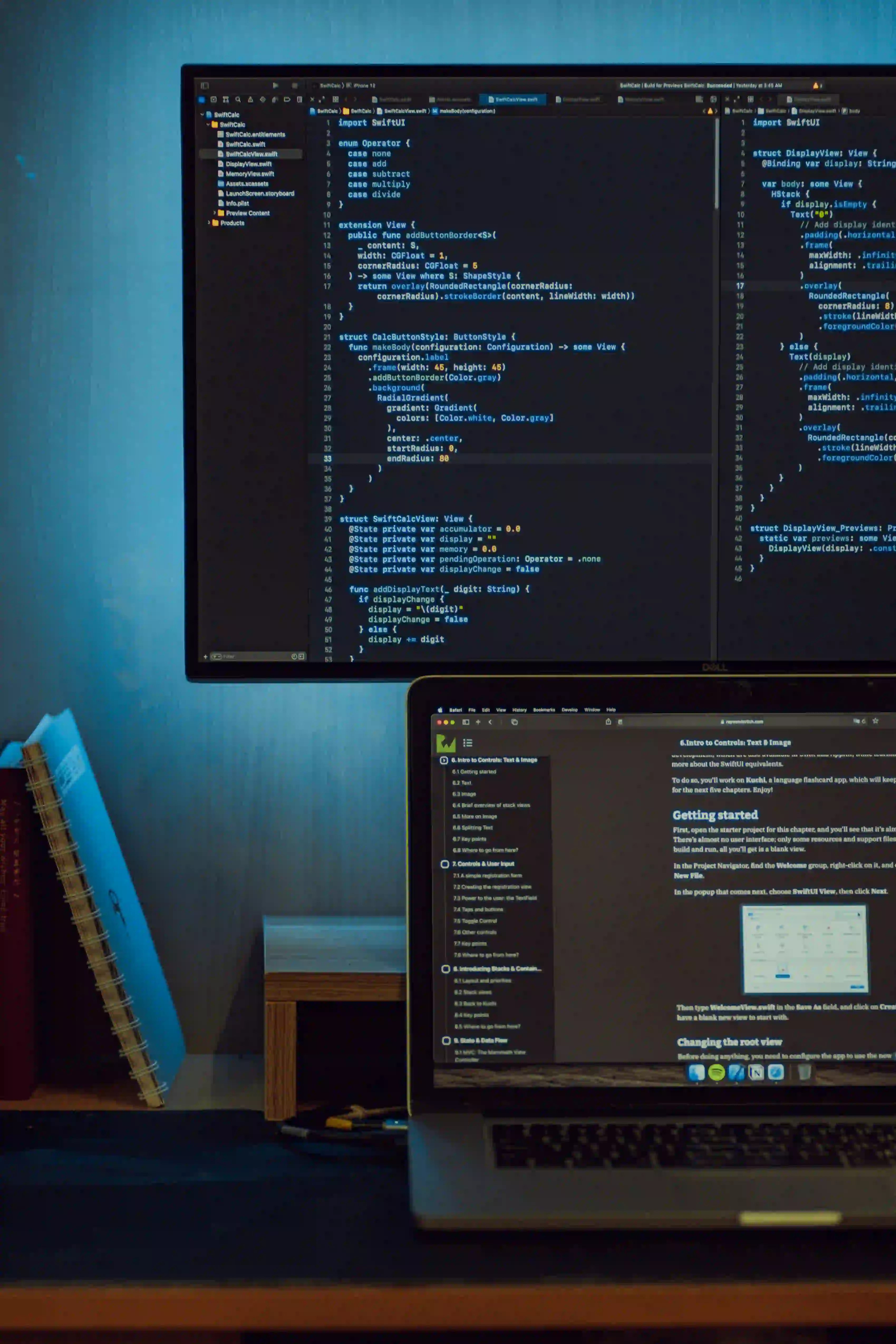
Optimize Java Applications: Trim Docker Images for Speed
In today's cloud-native ecosystem, containerization has become an essential practice for developers. Specifically, Docker allows us to package applications and their dependencies into a standardized unit for software development. However, the speed of deployment and the efficiency of resource usage can become bottlenecks if Docker images are not optimized. In this blog post, we will discuss techniques for optimizing Java applications by trimming Docker images, ultimately leading to faster deployment times and reduced resource consumption.
Understanding Docker Images
Before diving into optimization strategies, it's important to grasp what Docker images are. A Docker image is a lightweight, standalone, executable package that includes everything needed to run a piece of software: the code, runtime, libraries, environment variables, and configuration files.
Why Optimize Docker Images?
- Faster Builds: Smaller images reduce build times, making continuous integration and delivery more efficient.
- Reduced Deployment Times: Lightweight images can be pushed and pulled faster in repositories, speeding up deployment cycles.
- Lower Resource Usage: Smaller images take up less disk space and memory, which is crucial for environments with resource constraints.
With these benefits in mind, let's explore strategies to optimize Docker images for Java applications.
Use Multi-Stage Builds
One of the most effective ways to trim down Docker images is through multi-stage builds. This feature allows you to create multiple build stages within a single Dockerfile, enabling you to copy only the artifacts you need into your final image.
Example of Multi-Stage Builds
# Stage 1: Build the application
FROM maven:3.8.1-openjdk-11-slim AS builder
WORKDIR /app
COPY pom.xml .
COPY src ./src
RUN mvn clean package -DskipTests
# Stage 2: Create a lightweight image
FROM openjdk:11-jre-slim
WORKDIR /app
COPY --from=builder /app/target/my-java-app.jar ./my-java-app.jar
CMD ["java", "-jar", "my-java-app.jar"]
Why Use Multi-Stage Builds?
Using multi-stage builds means you only include the JRE and your application, without unnecessary build dependencies like Maven. This drastically reduces the final image size and accelerates deployment time.
Optimize Your Base Image
Java applications often run on OpenJDK images, which can be hefty. Consider opting for distroless images. These are minimal images that contain only your application and its runtime dependencies.
Example of a Distroless Image
# Using a distroless base image
FROM gcr.io/distroless/java:11
WORKDIR /app
COPY my-java-app.jar ./my-java-app.jar
ENTRYPOINT ["java", "-jar", "my-java-app.jar"]
Why Choose a Distroless Image?
Distroless images do not include unnecessary files and executables, thereby minimizing the attack surface and the performance implications of unused libraries. As a result, you get a more secure and faster deployment.
Reduce Layer Complexity
Each instruction in your Dockerfile creates a new layer in your image. To minimize the number of layers, consider combining related commands.
Example of Layer Reduction
# Avoid creating unnecessary layers
FROM openjdk:11-jre-slim
WORKDIR /app
COPY . .
RUN mvn clean package -DskipTests && \
rm -rf /app/src /app/pom.xml
Why Minimize Layers?
Fewer layers lead to smaller images, which translates to faster transfer speeds. More importantly, it reduces the complexity of the image, making updates and maintenance easier down the road.
Clean Up Unnecessary Files
After building your application, be sure to delete unnecessary files and dependencies before creating the final image. This includes folders related to the build process.
Example of Clean Up in Dockerfile
FROM maven:3.8.1-openjdk-11-slim AS builder
WORKDIR /app
COPY pom.xml .
COPY src ./src
RUN mvn clean package -DskipTests
# Second stage
FROM openjdk:11-jre-slim
WORKDIR /app
COPY --from=builder /app/target/my-java-app.jar ./my-java-app.jar
RUN rm -rf /app/src /app/pom.xml # Cleaning up build files
CMD ["java", "-jar", "my-java-app.jar"]
Why Clean Up?
Removing unnecessary files helps to further reduce the size of your Docker image. This practice not only conserves space but also enhances the security of your application by eliminating potential vulnerabilities from leftover files.
Leverage Caching
Docker utilizes layer caching to speed up builds. For effective caching, put the less frequently changing commands toward the top of your Dockerfile.
Example of Effective Caching
# This structure benefits from layer caching
FROM maven:3.8.1-openjdk-11-slim AS builder
WORKDIR /app
COPY pom.xml .
RUN mvn dependency:go-offline
COPY src ./src
RUN mvn clean package -DskipTests
Why Leverage Caching?
By separating out the commands that are less likely to change (like dependencies), you can avoid redundant work when rebuilding your images. This can save a significant amount of time during the development lifecycle.
Lessons Learned
Optimizing Docker images for Java applications is a critical step towards enhancing application performance and efficiency. Utilizing multi-stage builds, selecting lightweight base images, minimizing layers, and cleaning up unnecessary files are just a few essential strategies you can adopt.
For those looking to dive deeper into optimization strategies, consider reading the insightful article "Mastering Scratch: Trim Docker Images for Faster Deployment" at configzen.com. It provides valuable insights that can complement this blog post and further accelerate your journey toward optimized deployments.
By applying these best practices, you will not only reduce build and deployment times but also contribute to a more efficient use of resources in your development stack. Start optimizing today, and witness the transformation in your Java application's Docker images!