Troubleshooting Java Network Issues: A Beginner's Guide
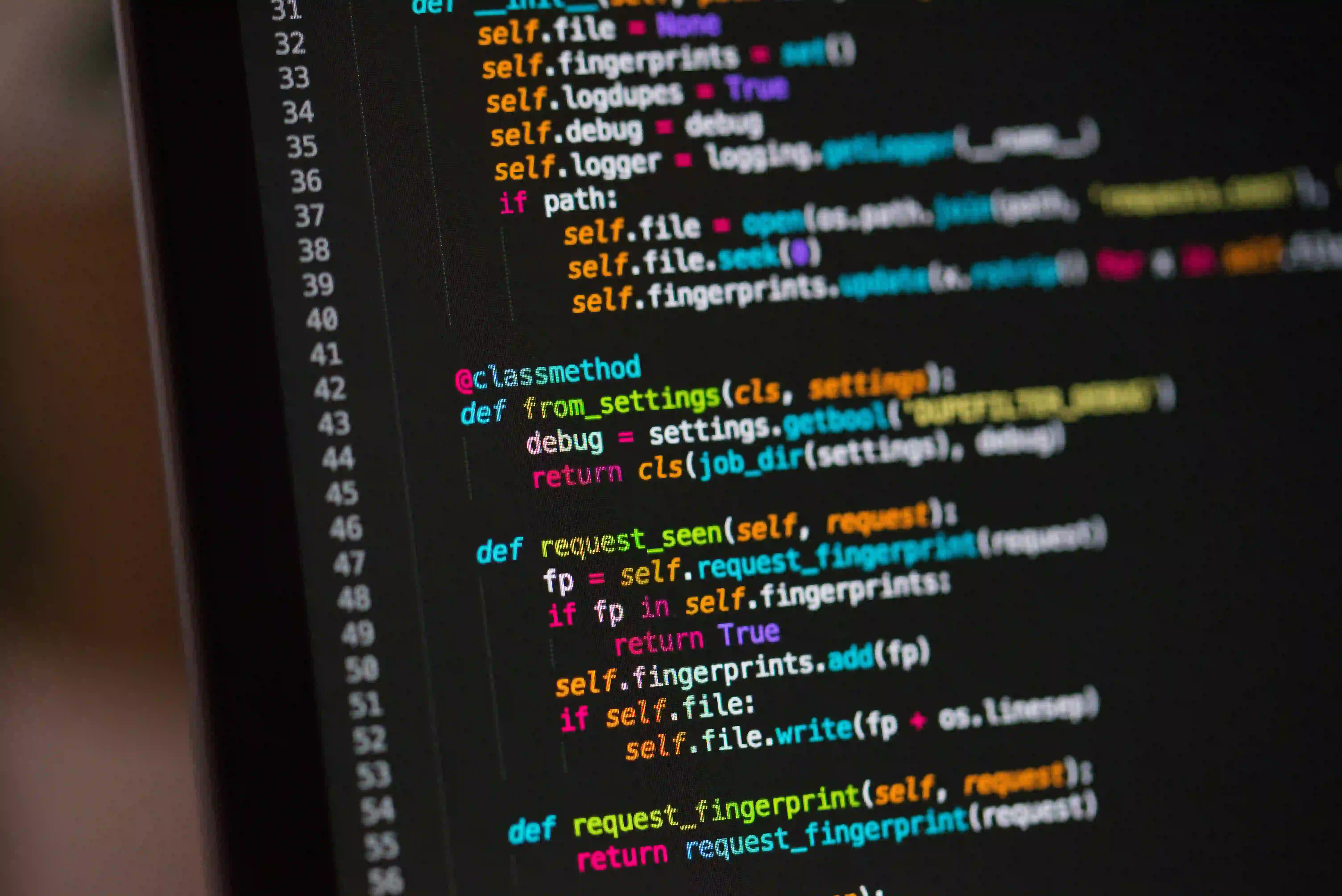
Troubleshooting Java Network Issues: A Beginner's Guide
When you dive into the world of Java, one of the significant hurdles you may encounter is networking. Java provides robust capabilities for networking, allowing developers to create distributed applications. However, issues can arise that can stump even experienced developers. In this guide, we will discuss common Java networking problems and how to resolve them, serving as a navigational tool for beginners interested in becoming proficient in Java networking.
Understanding Java Networking
Java's networking capabilities are built into the core Java platform. The java.net
package includes classes for implementing networking applications. Here, you can make HTTP requests, create sockets for TCP/IP communication, and much more.
This versatility is often accompanied by a host of network-related issues that can prevent applications from running smoothly. Before we dive into troubleshooting specifics, let’s brush up on a few essential networking concepts.
Key Networking Concepts
-
Sockets: Endpoints for sending and receiving data. You can think of them as communication channels between two applications.
-
IP Address and Port Number: Together, these identify a specific service running on a networked device.
-
Protocols: Rules governing communication over a network, for instance, TCP/IP or HTTP.
Understanding these concepts is crucial, as they are often the source of problems in Java networking applications.
Identifying Common Networking Issues
Network issues can often be classified into several categories. Below, I'll outline some of the most common problems and their solutions.
1. Connection Refused
One of the most frequent issues you'll encounter is the "Connection Refused" error. This typically happens when your application tries to connect to a server that isn't listening on the specified port.
Example Scenario:
You try to connect to a server on localhost:8080
but the server isn't running.
Solution:
Ensure that the server application is up and running. If you’re developing locally, double-check that you’ve started the server.
Code Snippet:
import java.io.IOException;
import java.net.Socket;
public class ServerConnection {
public static void main(String[] args) {
String hostname = "localhost";
int port = 8080;
try (Socket socket = new Socket(hostname, port)) {
System.out.println("Connected to the server!");
} catch (IOException e) {
System.err.println("Connection failed: " + e.getMessage());
}
}
}
This code attempts to connect to a server. If it fails, it will print the error message, hinting at what might be wrong.
2. Timeout Exception
Another common problem in network applications is a timeout exception. This means that your request to connect took too long, and the system gave up.
Example Scenario:
You're trying to reach an external API but your request isn't getting any response.
Solution:
Check the network connection, ensure that the API is live, and increase the timeout settings if necessary.
Code Snippet:
import java.net.HttpURLConnection;
import java.net.URL;
public class TimeoutExample {
public static void main(String[] args) {
String urlString = "http://example.com/api";
try {
URL url = new URL(urlString);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setConnectTimeout(5000); // 5 seconds timeout
connection.setReadTimeout(5000); // 5 seconds read timeout
connection.connect();
System.out.println("Response Code: " + connection.getResponseCode());
} catch (IOException e) {
System.err.println("Request failed: " + e.getMessage());
}
}
}
In this snippet, we configure timeouts for both connection and reading. If either fails or exceeds the specified time, an exception is thrown.
3. Security Exception
Java enforces strict security policies. If your application tries to make a network connection but lacks the necessary permissions, a Security Exception will be thrown.
Solution:
If you're running your application in a restricted environment (like an applet), ensure that you have granted the application the right permissions.
Code Snippet:
import java.io.IOException;
import java.net.Socket;
public class SecureConnection {
public static void main(String[] args) {
String hostname = "localhost";
int port = 8080;
try {
System.setSecurityManager(new SecurityManager());
Socket socket = new Socket(hostname, port);
System.out.println("Securely connected to the server!");
} catch (SecurityException e) {
System.err.println("Security exception: " + e.getMessage());
} catch (IOException e) {
System.err.println("I/O exception: " + e.getMessage());
}
}
}
This example demonstrates how you might create a socket connection while complying with Java's security restrictions.
Tips for Effective Troubleshooting
-
Use Logging: Implement logging mechanisms to help identify points of failures. This will provide you insight into your application's behavior.
-
Check for Updates: Libraries and frameworks are frequently updated to patch bugs and improve performance. Ensure you're using the latest version.
-
Monitor Network Health: Use tools or services that can monitor your network health for interruptions or latency issues.
-
Consult Documentation: Java's extensive documentation can be a valuable resource. When in doubt, review the Java networking documentation.
Connecting with Community Resources
If you find yourself baffled by a network issue, you're not alone. Communities such as Stack Overflow or Java forums can provide peer support.
Additionally, ensure to reference articles and blogs. The article titled "5 Common Network Issues Beginners Face and Fix" on configzen.com provides further context and solutions to common networking issues.
Understanding Java networking can dramatically enhance your application’s robustness and reliability.
My Closing Thoughts on the Matter
Navigating Java networking issues can be challenging, especially for beginners. By understanding common pitfalls and applying the above troubleshooting methods, you can streamline your development process. Remember, practice is key. As you experiment with different networking scenarios, your skills will improve, enabling you to build more complex applications with confidence.
Don't let networking issues hold you back—embrace them as an opportunity to grow and learn in the expansive world of Java!