Mastering Java: Overcoming Zoom SDK Integration Challenges
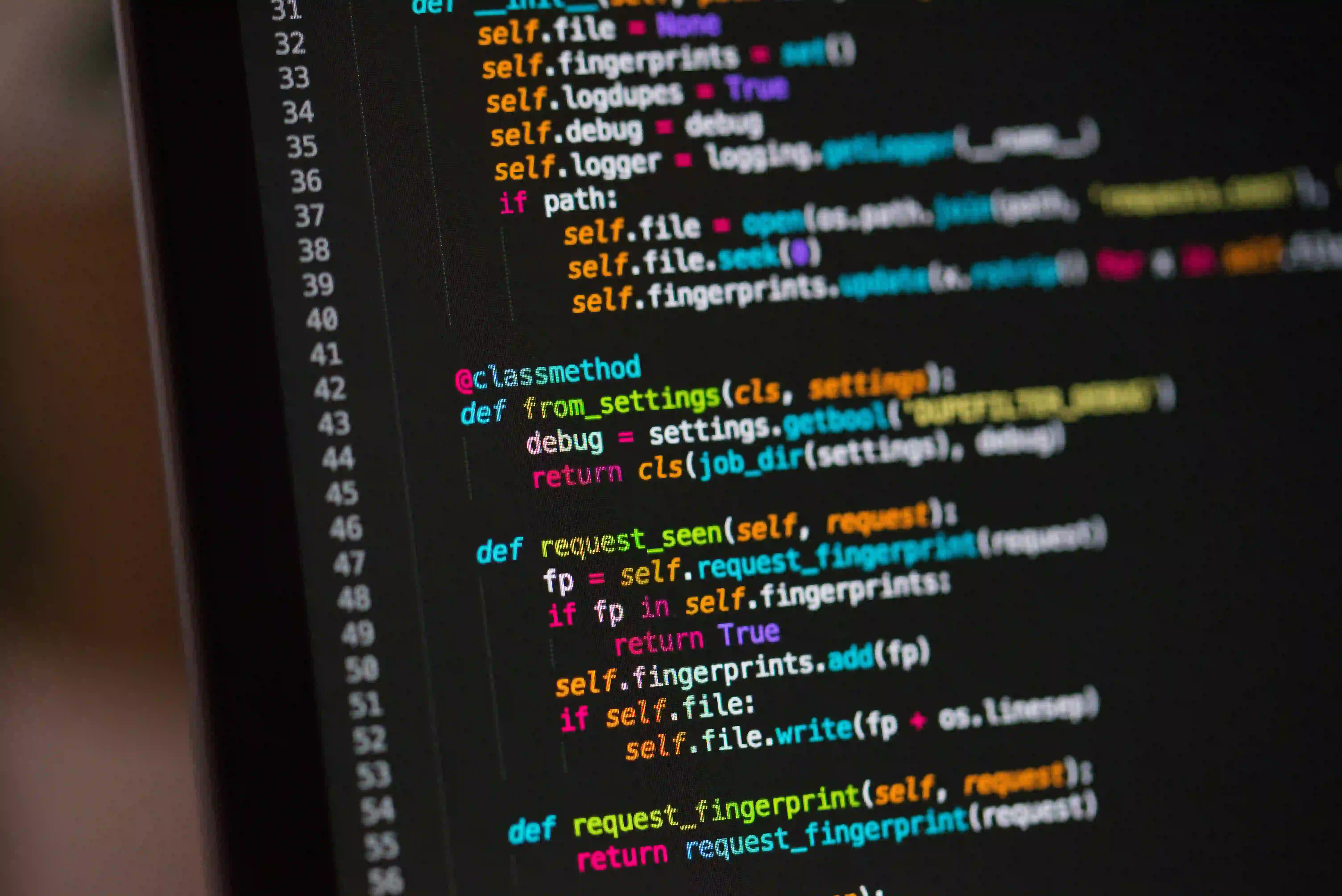
Mastering Java: Overcoming Zoom SDK Integration Challenges
Integrating third-party services into your applications can be daunting, especially when dealing with complex SDKs like Zoom's. However, with a systematic approach and a clear understanding of the challenges, you can master the art of integration. In this blog post, we will explore the common hurdles developers face when integrating the Zoom SDK in Java applications and provide effective solutions to overcome them.
Understanding Zoom SDK Integration
Before diving into the hurdles and their solutions, it's essential to grasp what the Zoom SDK offers. The Zoom Video SDK enables developers to integrate video conferencing capabilities directly into their applications, providing features like real-time audio, video, and chat.
Why Use the Zoom SDK?
- Rich Features: It allows features such as screen sharing, live annotations, and breakout rooms.
- Customization: Unlike using Zoom’s web application, integrating the SDK lets you tailor the user experience.
- Cross-Platform Support: Functionality is available on various platforms, including web, mobile, and desktop apps.
Having laid the groundwork, let’s discuss some of the common challenges developers encounter when integrating the Zoom SDK and how to navigate them efficiently.
Challenge 1: Authentication & Authorization
Explanation
One of the first hurdles developers face is managing authentication and authorization. The Zoom SDK requires valid credentials and access tokens to utilize its features. Without correct configurations, you might run into security issues, or worse, you’ll be unable to access the video capabilities altogether.
Solution
To authenticate properly, ensure you follow the OAuth flow provided by Zoom. Here's a basic Java snippet demonstrating how to retrieve an OAuth token:
import java.io.IOException;
import java.util.Base64;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.Response;
import okhttp3.ResponseBody;
public class ZoomAuth {
private static final String CLIENT_ID = "your_client_id";
private static final String CLIENT_SECRET = "your_client_secret";
private static final String TOKEN_URL = "https://zoom.us/oauth/token";
public String getOAuthToken() throws IOException {
OkHttpClient client = new OkHttpClient();
String credentials = CLIENT_ID + ":" + CLIENT_SECRET;
String basicAuth = "Basic " + Base64.getEncoder().encodeToString(credentials.getBytes());
Request request = new Request.Builder()
.url(TOKEN_URL)
.header("Authorization", basicAuth)
.post(RequestBody.create(null, new byte[0]))
.build();
try (Response response = client.newCall(request).execute()) {
ResponseBody responseBody = response.body();
if (response.isSuccessful() && responseBody != null) {
return responseBody.string();
} else {
throw new IOException("Failed to retrieve token: " + response.message());
}
}
}
}
Commentary
In this code snippet, we're using OkHttpClient
to send an HTTP request to Zoom's token endpoint. The token is essential for subsequent requests. Notice how we utilize Base64 encoding for authorization—this step is crucial to maintain security while communicating with the Zoom API.
Challenge 2: SDK Initialization
Explanation
Once authenticated, you may face issues initializing the SDK correctly. This can lead to various runtime errors, and the SDK may not function as expected.
Solution
Refer to the official Zoom documentation to ensure proper initialization. Here’s a streamlined approach to initializing the Zoom SDK in Java:
import com.zoom.sdk.ZoomSDK;
import com.zoom.sdk.ZoomSDKInitializeListener;
public class ZoomInitialization {
public void initializeZoomSDK() {
ZoomSDK.getInstance().initialize(new ZoomSDKInitializeListener() {
@Override
public void onInitialized() {
System.out.println("Zoom SDK initialized successfully!");
}
@Override
public void onFailed(int errorCode) {
System.err.println("Failed to initialize Zoom SDK: " + errorCode);
}
});
}
}
Commentary
In the above code, we are leveraging the ZoomSDKInitializeListener
to determine the state of SDK initialization. A successful initialization triggers a callback, while failure provides an error code for debugging. Understanding these callbacks can help debug issues quickly, enhancing the overall integration process.
Challenge 3: Handling Errors and Exceptions
Explanation
Managing runtime exceptions and errors effectively is vital in ensuring a smooth user experience. Errors can occur due to network issues, incorrect parameters, or API changes.
Solution
Implement proper error handling using try-catch blocks throughout your SDK interaction. Example:
public class ZoomMeetingManager {
public void startMeeting(String meetingId) {
try {
// Logic to start a meeting
// If there is an issue
throw new RuntimeException("Simulated start meeting error.");
} catch (Exception e) {
System.err.println("Error starting meeting: " + e.getMessage());
// Consider notifying users or logging the error
}
}
}
Commentary
This approach captures any exceptions thrown during the meeting startup sequence. Not only does this assist with user feedback, but it also provides insights into what went wrong, allowing developers to debug accordingly.
Challenge 4: User Experience Design
Explanation
The frontend interface can be equally challenging. Users expect a seamless experience, and poorly designed interfaces can deter them from using your application.
Solution
Focus on creating a user-friendly UI that intuitively guides users through steps like joining or creating a meeting. Make consistent use of tooltips, buttons, and loading indicators. Additionally, refer to UI design best practices to maintain high usability standards.
Example
While specific JavaFX or Swing code examples aren’t included here, it is crucial to implement responsiveness and easy navigation in your user interface. Use libraries specifically designed for video UI to enhance user interactions.
For more on common integration hurdles, check out "Zoom SDK Integration: Navigating Common Hurdles" at infinitejs.com/posts/zoom-sdk-integration-navigating-hurdles.
Final Considerations
Integrating the Zoom SDK into your Java application doesn’t have to be an insurmountable task. By understanding the common pain points—authentication, SDK initialization, error handling, and user experience—you can navigate the integration process more effectively. What's essential is continued learning, practice, and adaptation to updates from Zoom as they enhance their SDK offerings.
With the skills discussed in this guide, you're well on your way to creating seamless, interactive applications that elevate user experiences through video conferencing capabilities. Happy coding!