Troubleshooting Java Applications: Solving Network Issues
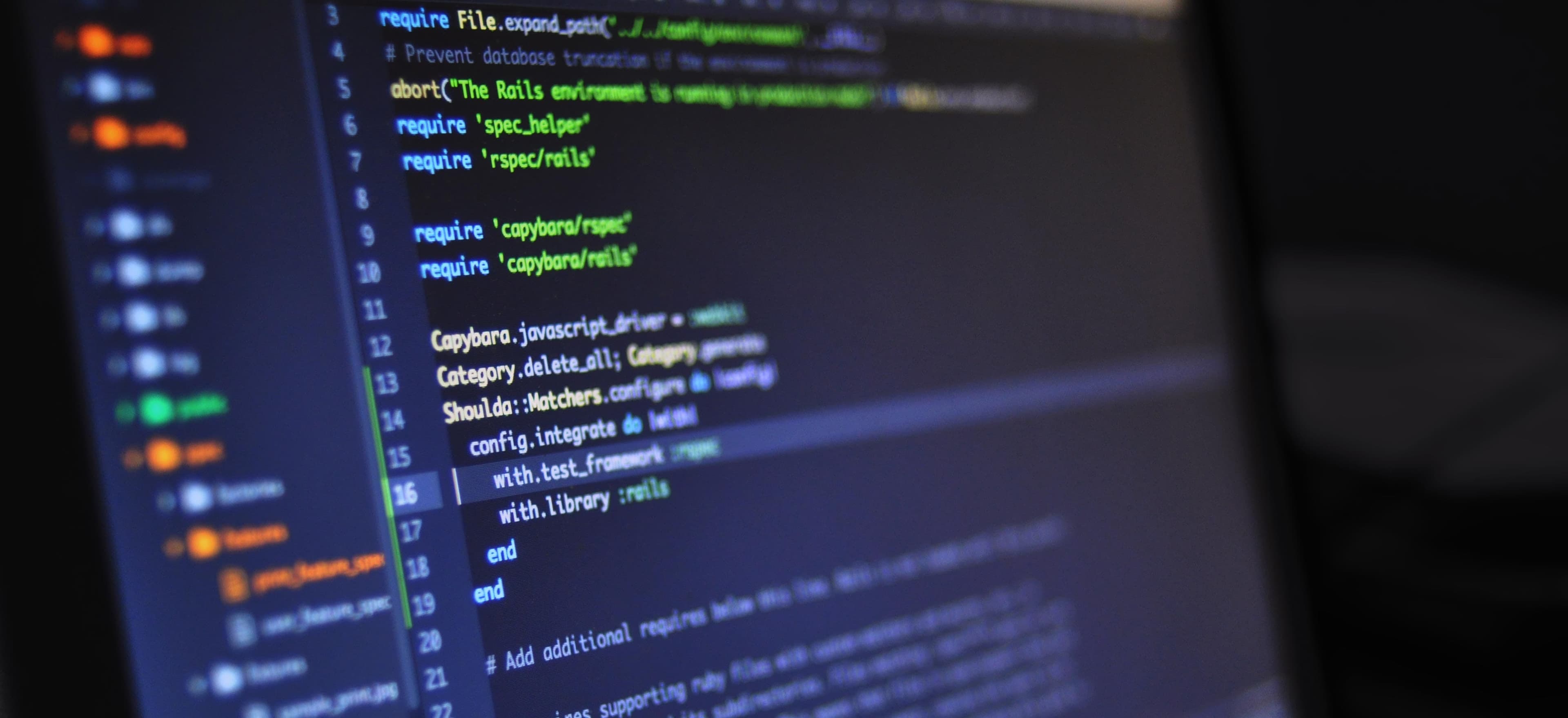
- Published on
Troubleshooting Java Applications: Solving Network Issues
As Java developers, we often face a multitude of challenges that come with building networked applications. Smart developers anticipate potential network issues; those who don't find themselves knee-deep in troubleshooting. In this post, we will explore common network problems encountered in Java applications and strategies for resolving them effectively.
Understanding Network Architecture in Java Applications
Before diving into troubleshooting techniques, it is critical to understand how network communication works in Java. Java provides a rich set of libraries that facilitate network programming; these include the standard Java networking APIs like java.net.Socket
, java.net.ServerSocket
, and java.net.URL
.
Example: Basic Socket Communication
Consider the following code that demonstrates how to establish a simple client-server communication using sockets.
// Server Code
import java.io.*;
import java.net.*;
public class SimpleServer {
public static void main(String[] args) {
try (ServerSocket serverSocket = new ServerSocket(8080)) {
System.out.println("Server is listening on port 8080");
while (true) {
Socket socket = serverSocket.accept();
new ClientHandler(socket).start();
}
} catch (IOException ex) {
ex.printStackTrace();
}
}
}
class ClientHandler extends Thread {
private Socket socket;
public ClientHandler(Socket socket) {
this.socket = socket;
}
public void run() {
try (PrintWriter out = new PrintWriter(socket.getOutputStream(), true);
BufferedReader in = new BufferedReader(new InputStreamReader(socket.getInputStream()))) {
String inputLine;
while ((inputLine = in.readLine()) != null) {
System.out.println("Received: " + inputLine);
out.println("Echo: " + inputLine);
}
} catch (IOException ex) {
ex.printStackTrace();
}
}
}
Commentary
In this example, the server opens a socket on port 8080 and continuously listens for incoming connections. When a connection is accepted, it spawns a new thread for handling the client. This approach allows multiple clients to connect simultaneously.
Understanding these basics helps diagnose problems that arise as applications scale and complexity increases.
Common Network Issues in Java Applications
1. Connection Timeouts
Issue: A connection attempt to a remote server can fail if the server is unreachable or slow to respond. Java applications will throw a SocketTimeoutException
.
Resolution: Adjust the socket timeout settings when you create your socket.
Socket socket = new Socket();
socket.connect(new InetSocketAddress("hostname", port), timeout); // timeout in milliseconds
Why: Setting an appropriate timeout prevents your application from hanging indefinitely when attempting to connect to a server that may be down.
2. Unresolved Hostnames
Issue: Sometimes, your application cannot resolve a hostname to an IP address, leading to a UnknownHostException
.
Resolution: Ensure that the hostname is correctly specified and reachable. Use DNS tools to validate.
Example Code:
try {
InetAddress address = InetAddress.getByName("example.com");
System.out.println("Host Address: " + address.getHostAddress());
} catch (UnknownHostException e) {
System.err.println("Host not found.");
}
Why: This simple check will help verify whether the issue stems from name resolution before deep diving into your application logic.
3. Firewall and Security Settings
Issue: Firewalls can block access to certain ports, stopping your client-server communication in its tracks.
Resolution: Configure the local and server firewalls to allow traffic on the necessary ports. Consult with network security teams for comprehensive checks.
4. Network Configuration Changes
Issue: If your application runs in a dynamic network environment (e.g., cloud services), an IP address may change, or certain services may become unreachable.
Resolution: Utilize tools like ping
or Java's InetAddress.isReachable()
method to check for connectivity.
try {
InetAddress address = InetAddress.getByName("example.com");
if (address.isReachable(2000)) { // timeout in milliseconds
System.out.println("Host is reachable");
} else {
System.out.println("Host is NOT reachable");
}
} catch (IOException e) {
e.printStackTrace();
}
Why: Regular checks can help mitigate issues that arise from sudden network changes, keeping your application robust.
5. Load Balancing Problems
Issue: If your application interacts with a load-balanced server setup, misconfigurations may lead to failed connections.
Resolution: Verify the load balancer's health checks and ensure that your service is correctly registered and reachable.
Debugging Network Issues
When troubleshooting Java applications, employing systematic debugging processes is essential. Here are some robust strategies:
Use Logging
Implement comprehensive logging throughout your networking code to capture states, exceptions, and data flows. Utilize libraries like Log4j or SLF4J for flexible logging configurations.
Network Monitoring Tools
Tools like Wireshark can help you inspect the packets sent and received by your application. This insight can be invaluable when you suspect network-level issues.
Unit Testing
Develop unit tests for your networking components to ensure resilience against common network issues. JUnit can be a useful framework for this purpose.
import org.junit.Test;
import static org.junit.Assert.*;
public class NetworkTest {
@Test
public void testConnection() {
// Presume a method checkServerConnection() exists
assertTrue(checkServerConnection("example.com", 8080));
}
}
Why: Automated testing for connectivity reduces the risk of introducing new issues during application changes.
Additional Learning Resources
For developers who want to dig deeper into network-related issues, take a look at our article titled 5 Common Network Issues Beginners Face and Fix, which outlines practical solutions that can enhance your troubleshooting toolbox.
Bringing It All Together
Troubleshooting network issues in Java applications can be daunting, but with the right tools, techniques, and understanding of underlying principles, developers can navigate these challenges efficiently. Always remember to gather as much information as possible, leverage effective debugging practices, and implement preventive measures.
That said, tackling network issues is part of the development journey, and each resolved issue adds to your expertise and confidence as a developer. Whether you're working on a small project or a large-scale application, maintaining clear network protocols and error-handling procedures will lead to a smoother development experience.
Happy coding!