Mastering Java: Overcoming Zoom SDK Integration Challenges
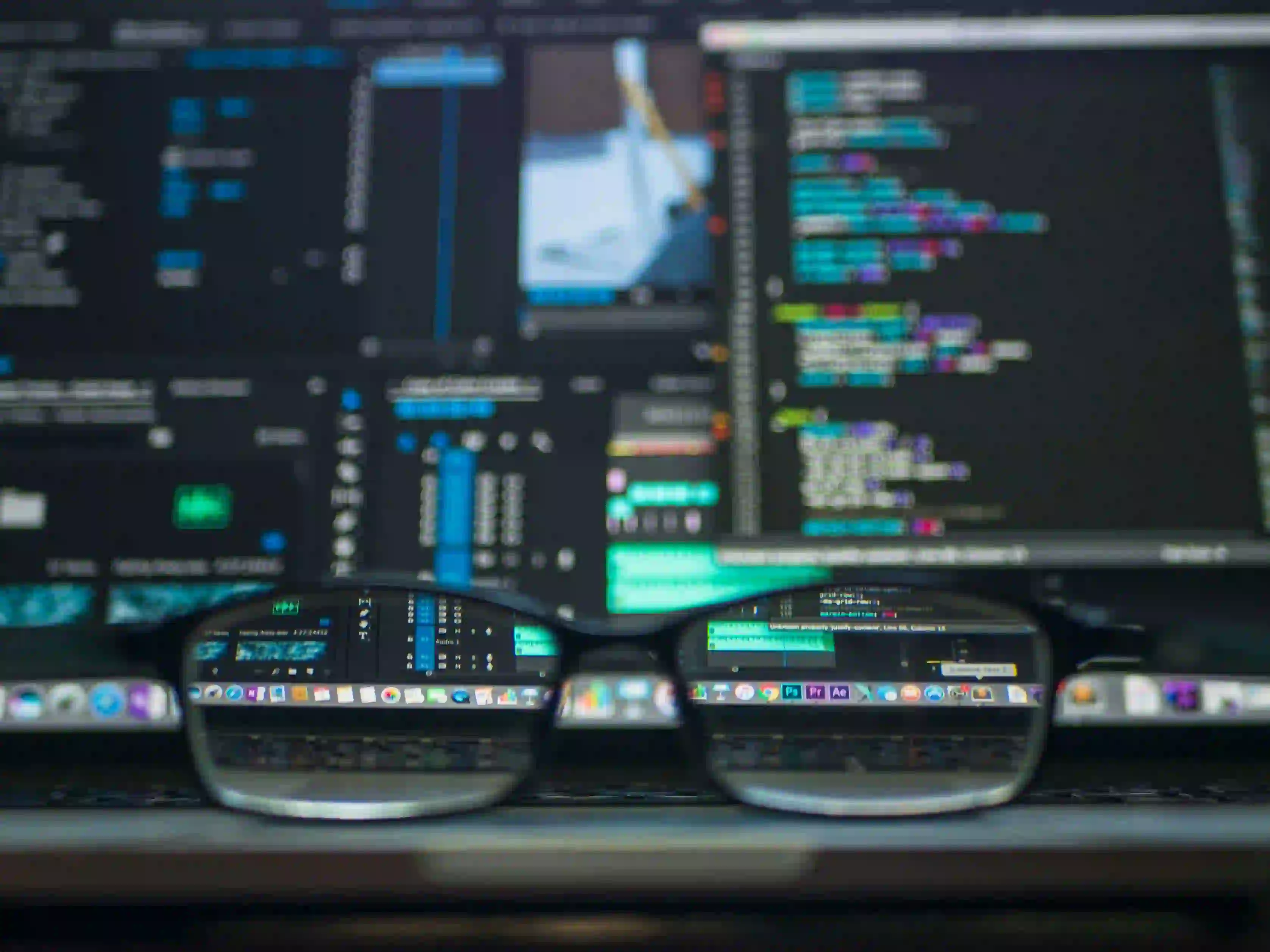
Mastering Java: Overcoming Zoom SDK Integration Challenges
In the modern world, video conferencing has become integral for both personal and professional interactions. As businesses increasingly turn to platforms like Zoom, integrating its features into Java applications has risen in demand. In this article, we will explore the common challenges you may face while implementing the Zoom SDK in Java and provide solutions to make your integration smoother.
Understanding Zoom SDK
Before diving into the challenges, it's crucial to understand what the Zoom SDK entails. The Zoom Software Development Kit (SDK) allows developers to integrate Zoom's video conferencing capabilities into their own applications. This enables features such as video meetings, webinars, and real-time chat, all embedded seamlessly into your Java applications.
To begin the integration process, developers often look for guidelines and troubleshooting tips. A valuable resource to reference is the article titled Zoom SDK Integration: Navigating Common Hurdles, which outlines several pitfalls and their solutions.
Prerequisites for Integration
Before we move forward, ensure you have the following:
- Java Development Kit (JDK) - version 8 or higher.
- Zoom SDK - the necessary libraries must be downloaded from the Zoom Developer Portal.
- Maven or Gradle - for handling dependencies.
Common Challenges and Solutions
Integrating the Zoom SDK comes with its set of challenges, including authentication, SDK initialization, and connection issues. Let's tackle them one by one, offering code snippets and explanations to solidify your understanding.
1. Authentication Issues
One of the primary hurdles developers face is authentication. The Zoom SDK requires a valid JWT or OAuth token for authentication. Here’s a Java-based example to obtain a JWT token:
import java.util.Base64;
import java.util.Date;
import io.jsonwebtoken.Jwts;
import io.jsonwebtoken.SignatureAlgorithm;
public class ZoomJWT {
private static final String API_KEY = "YOUR_API_KEY";
private static final String API_SECRET = "YOUR_API_SECRET";
public static String generateJWT() {
long now = System.currentTimeMillis();
Date nowDate = new Date(now);
// Define expiration time, e.g., 1 hour
Date exp = new Date(now + 3600000);
// Creating the token using JWT library
String jwt = Jwts.builder()
.setIssuedAt(nowDate)
.setExpiration(exp)
.setIssuer(API_KEY)
.signWith(SignatureAlgorithm.HS256, API_SECRET)
.compact();
return jwt;
}
}
In this snippet, we generate a JWT token using the API key and secret obtained from the Zoom Developer Portal. Why JWT? The JWT (JSON Web Token) format is stateless and makes the process quick and reliable.
2. SDK Initialization
Initializing the Zoom SDK can also be where things go awry. You need to ensure that the SDK is correctly initialized before performing operations. Below is an example.
import com.zipow.videobox.ZoomSDK;
public class ZoomIntegration {
public void initZoomSDK() {
ZoomSDK zoomSDK = ZoomSDK.getInstance();
if (zoomSDK.initialize(context, API_KEY, API_SECRET)) {
System.out.println("Zoom SDK initialized successfully.");
} else {
System.err.println("Failed to initialize Zoom SDK.");
}
}
}
Here, we check if the SDK initializes successfully. Why is this critical? If the SDK isn't initialized properly, none of the features will work, leading to frustrating debugging sessions.
3. Handling Meeting Status
After successful initialization, the next step is to join or start a meeting. This often leads to confusion since various meeting states need to be handled. Below is how you can manage these statuses:
import com.zipow.videobox.conf.ZoomSDKMeetingService;
public class MeetingHandler {
public void joinMeeting(String meetingID, String password) {
ZoomSDKMeetingService meetingService = ZoomSDK.getInstance().getMeetingService();
meetingService.joinMeeting(meetingID, password);
meetingService.addListener(new MeetingServiceListener() {
@Override
public void onMeetingStatusChanged(MeetingStatus status) {
if (status == MeetingStatus.MEETING_STATUS_ENDED) {
System.out.println("Meeting has ended.");
}
}
});
}
}
In this code snippet, the listener captures the meeting's status changes. This ensures that your application can respond appropriately, enhancing user experience.
4. Error Handling
Error handling plays a crucial role in making your application robust. Zoom SDK provides various error codes, and having proper error-handling mechanisms in place will save you from unexpected crashes. Here’s an example:
import com.zipow.videobox.conf.MeetingError;
public class ErrorHandler {
public void handleError(MeetingError error) {
switch (error) {
case MEETING_ERROR_NETWORK:
System.err.println("Network issue, please check your connection.");
break;
case MEETING_ERROR_INVALID_MEETING_ID:
System.err.println("Invalid Meeting ID entered.");
break;
default:
System.err.println("An unknown error occurred.");
break;
}
}
}
In this case, specific errors are caught, and appropriate messages are displayed. Why this matters: Providing feedback based on error types enhances user experience, making it easier for users to troubleshoot issues.
5. Testing and Debugging
Testing is often neglected, but it is crucial to ensure that the integration works under various scenarios. Using JUnit for unit tests is a good practice. Below is a simplistic example of how you could set up a unit test for your JWT generation:
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.*;
public class ZoomJWTTest {
@Test
public void testJWTGeneration() {
String token = ZoomJWT.generateJWT();
assertNotNull(token);
assertFalse(token.isEmpty());
// Further checks can be performed to decode the token
}
}
Here, we ensure the generated token isn't null or empty, laying the groundwork for more extensive tests. Why unit tests? They help catch errors early, making your development process smoother.
To Wrap Things Up
Integrating the Zoom SDK into Java applications can be rewarding, yet it comes with its share of challenges. We've outlined common hurdles and provided practical code snippets to guide you through the process. From authentication to error handling, a robust understanding and well-structured code will lead to a successful integration.
For more insights, don’t forget to check the article on Zoom SDK Integration: Navigating Common Hurdles for additional tips and tricks.
By mastering these challenges, you're not just improving your coding skills; you're transforming how people connect and collaborate in a hybrid world. Happy coding!