Overcoming Adapter Pattern Confusion: A Clear Guide
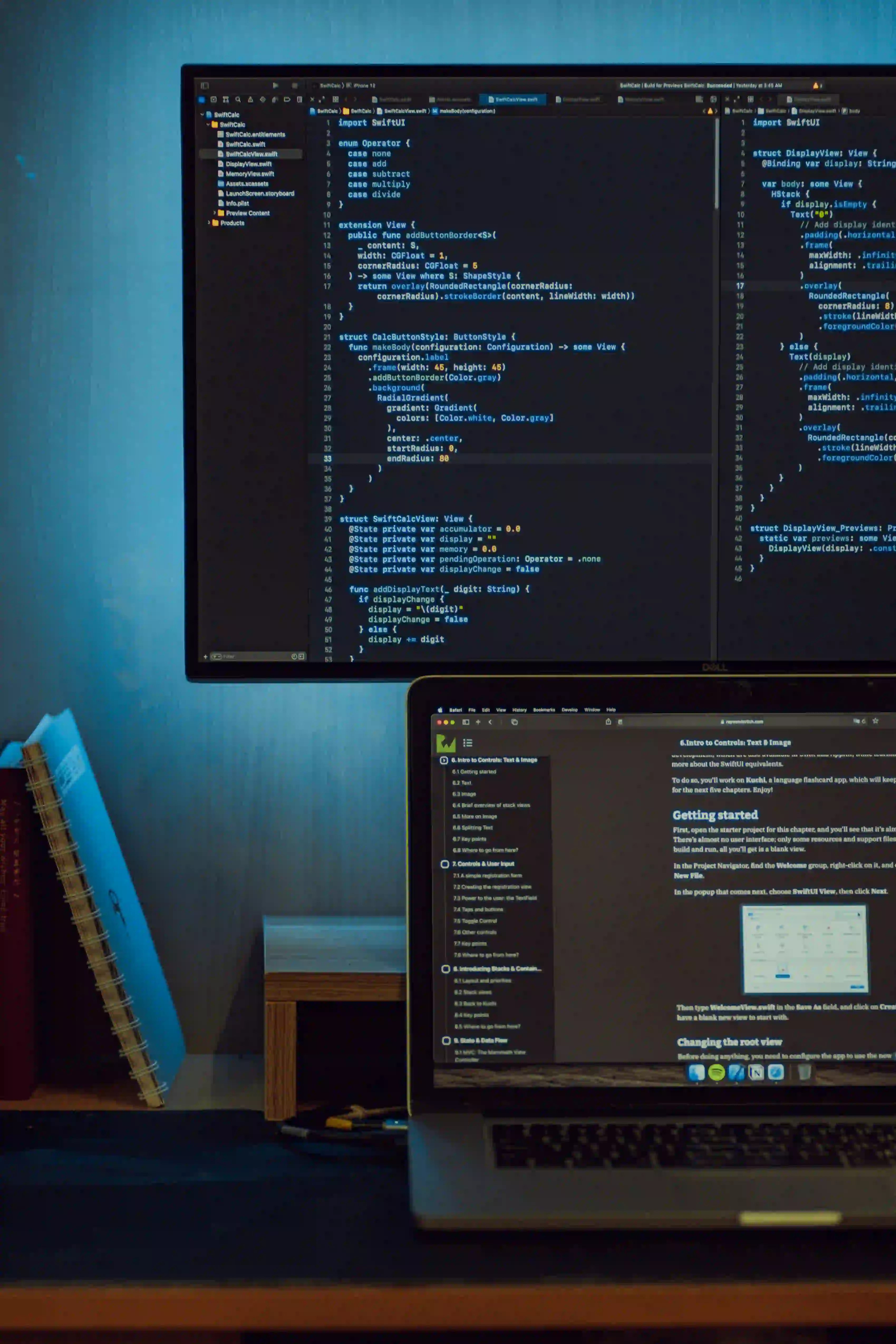
Overcoming Adapter Pattern Confusion: A Clear Guide
The Adapter Pattern is among those essential design patterns frequently appearing in software engineering discussions. Despite its prominence, many developers find themselves confused when they first encounter it. This post will clarify the Adapter Pattern, when to use it, and how to implement it effectively in Java.
What is the Adapter Pattern?
The Adapter Pattern is a structural design pattern that allows two incompatible interfaces to work together. It acts as a bridge between an interface and a class, enabling classes to communicate that otherwise wouldn't due to incompatible interfaces.
In simple terms, the Adapter Pattern converts the interface of a class into another interface clients expect. Using this pattern promotes flexibility and scalability in code, making it easier to maintain.
When to Use the Adapter Pattern
- Integration of Legacy Code: When working with legacy code that may not conform to new interfaces.
- Third-Party Libraries: To allow the use of external libraries that do not fit well with your existing code structure.
- Multiple Interfaces: In scenarios where you are compelled to use multiple interfaces from different sources.
With the basis of understanding in place, let's dive into a practical example using Java.
Java Implementation of the Adapter Pattern
Scenario Overview
Let's say you have different types of media players – audio and video. However, you have a client that only expects a media player interface that handles audio playback. The Adapter Pattern allows you to work with video players without altering the existing code structure.
Step 1: Define the Target Interface
We will first define the MediaPlayer
interface that our client uses.
public interface MediaPlayer {
void play(String audioType, String fileName);
}
Step 2: Create a Concrete Class for the Existing Functionality
Next, let's create an existing class, AudioPlayer
, that implements the target interface:
public class AudioPlayer implements MediaPlayer {
@Override
public void play(String audioType, String fileName) {
if(audioType.equalsIgnoreCase("mp3")) {
System.out.println("Playing mp3 file. Name: " + fileName);
} else {
System.out.println("Invalid audio type: " + audioType);
}
}
}
Why this matters: Here, AudioPlayer
can only play MP3 files. Our goal is to adapt it to handle video files through an adapter.
Step 3: Create an Incompatible Class
Now, let’s define a class called VideoPlayer
. The objective here is that VideoPlayer
plays video files but cannot implement the MediaPlayer
interface directly.
public class VideoPlayer {
public void playVideo(String fileName) {
System.out.println("Playing video file. Name: " + fileName);
}
}
Step 4: Implement the Adapter Class
Now, let's create an AudioVideoAdapter
class that will help connect the MediaPlayer
interface and the VideoPlayer
class.
public class AudioVideoAdapter implements MediaPlayer {
private VideoPlayer videoPlayer;
public AudioVideoAdapter(VideoPlayer videoPlayer) {
this.videoPlayer = videoPlayer;
}
@Override
public void play(String audioType, String fileName) {
if (audioType.equalsIgnoreCase("mp4")) {
videoPlayer.playVideo(fileName);
} else {
System.out.println("Invalid audio type: " + audioType);
}
}
}
Why this is significant: The AudioVideoAdapter
enables the MediaPlayer
interface to handle VideoPlayer
functionality seamlessly.
Step 5: Test the Adapter
Finally, we can test our implementation to see how it works in practice.
public class AdapterPatternDemo {
public static void main(String[] args) {
MediaPlayer audioPlayer = new AudioPlayer();
audioPlayer.play("mp3", "song.mp3");
VideoPlayer videoPlayer = new VideoPlayer();
MediaPlayer adapter = new AudioVideoAdapter(videoPlayer);
adapter.play("mp4", "movie.mp4");
adapter.play("mp3", "song.mp3");
}
}
Expected Output:
Playing mp3 file. Name: song.mp3
Playing video file. Name: movie.mp4
Invalid audio type: mp3
In Conclusion, Here is What Matters
In this demo, we showcased how the Adapter Pattern can unify different types of classes under a common interface, allowing them to function together seamlessly.
Benefits of the Adapter Pattern:
- Encapsulation: The client code knows nothing about the details of the
VideoPlayer
, enhancing encapsulation. - Flexibility: You can easily add more media types without changing existing classes.
- Interoperability: This pattern provides the means for diverse systems to communicate smoothly.
For a deeper understanding of design patterns, check out Refactoring Guru's Design Patterns and Java Design Patterns on GeeksforGeeks.
Final Thoughts
Now, you should feel more confident in using the Adapter Pattern in your Java applications. It remains a robust tool that can simplify your codebase, allowing for seamless integration of diverse components. Always remember that while design patterns provide proven solutions, the most effective implementations are those tailored to specific project requirements.
Happy coding!