Troubleshooting Slow PageRank Jobs on AWS EMR
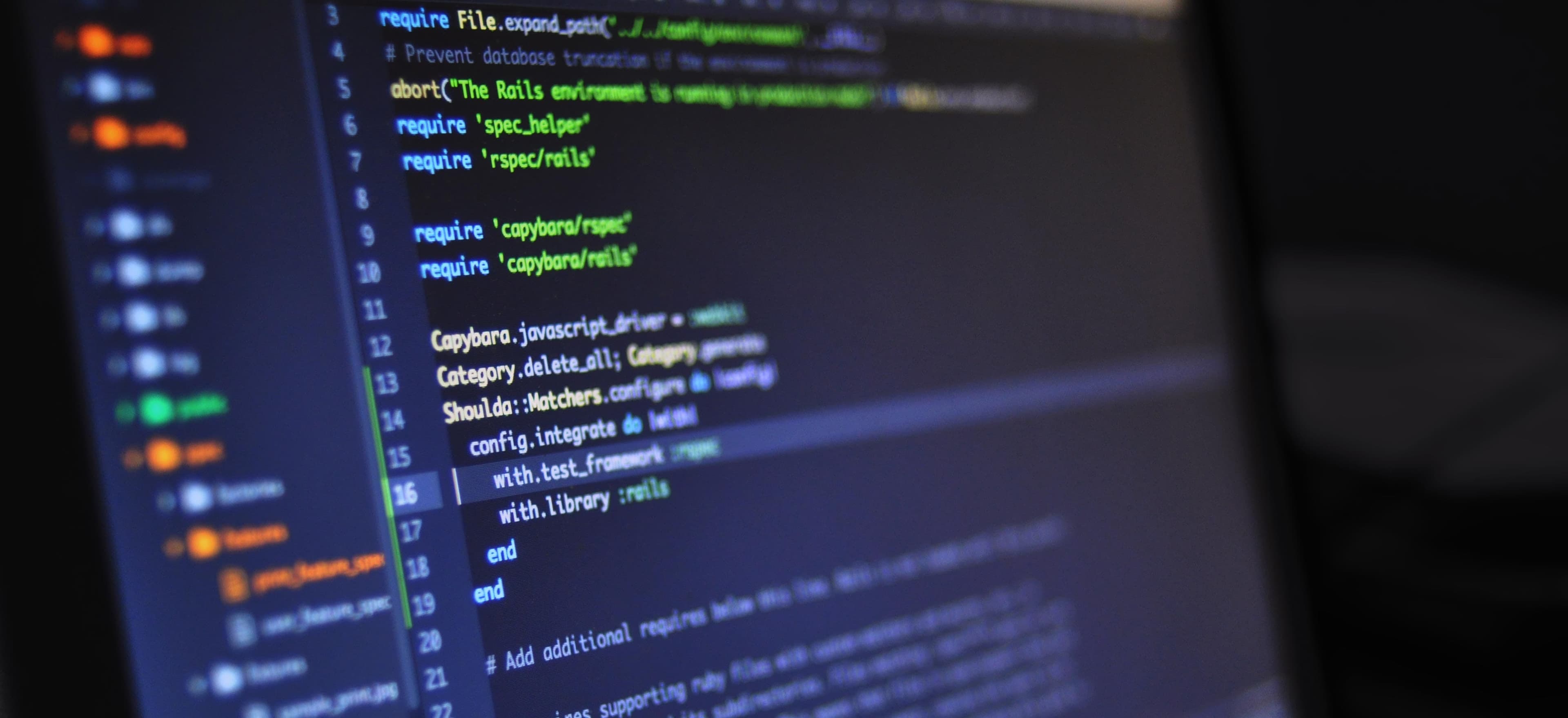
- Published on
Troubleshooting Slow PageRank Jobs on AWS EMR
In the era of big data, it's crucial to have efficient algorithms and frameworks to handle large datasets. One such algorithm is PageRank, a graph-based algorithm primarily used by search engines to rank web pages. Although powerful, running PageRank on AWS EMR can sometimes lead to unexpected slowdowns. This guide will discuss how to troubleshoot slow PageRank jobs on AWS EMR, providing actionable insights and practical solutions.
Understanding PageRank in Hadoop
PageRank is a graph algorithm that assigns a ranking to each element in a set of elements based on their connections. In the context of Hadoop's MapReduce framework, this is done by creating a directed graph where nodes represent pages, and edges represent links. AWS EMR simplifies this process by easing the deployment and scaling of such tasks.
Key Factors Leading to Slow PageRank Jobs
-
Data Size and Structure
- A larger dataset inherently increases processing time. Understanding the optimization of your dataset layout can greatly impact performance.
-
Cluster Type and Configuration
- The instance types and cluster configurations profoundly affect job performance. For instance, using underpowered instances will slow down your jobs.
-
Inefficient Resource Allocation
- AWS EMR has several tunable parameters that control how resources are allocated for each job. Failure to optimize these can lead to bottlenecks.
-
Shuffling Overhead
- Shuffling data between different nodes can introduce latency, especially if there is a large volume of data being transferred.
Initial Setup
Before diving into the troubleshooting process, ensure that you have set up your EMR cluster correctly with the necessary configurations.
Java provides an efficient way to run PageRank at scale in Hadoop. Below is a simplified version of the PageRank implementation:
import java.io.IOException;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.io.DoubleWritable;
import org.apache.hadoop.io.IntWritable;
import org.apache.hadoop.io.NullWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapred.JobConf;
import org.apache.hadoop.mapred.Mapper;
import org.apache.hadoop.mapred.Reducer;
import org.apache.hadoop.mapreduce.Job;
import org.apache.hadoop.mapreduce.lib.input.FileInputFormat;
import org.apache.hadoop.mapreduce.lib.output.FileOutputFormat;
public class PageRank {
public static class PageRankMapper extends Mapper<Object, Text, Text, DoubleWritable> {
public void map(Object key, Text value, Context context) throws IOException, InterruptedException {
// Parsing logic here
// Emit page and its rank
}
}
public static class PageRankReducer extends Reducer<Text, DoubleWritable, Text, DoubleWritable> {
public void reduce(Text key, Iterable<DoubleWritable> values, Context context) throws IOException, InterruptedException {
// Aggregation logic here
}
}
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "PageRank");
job.setJarByClass(PageRank.class);
job.setMapperClass(PageRankMapper.class);
job.setReducerClass(PageRankReducer.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(DoubleWritable.class);
FileInputFormat.addInputPath(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}
Code Commentary
-
The
PageRank
class contains mapper and reducer classes used for the PageRank algorithm. Themap
method calculates the rank for each page based on its connections, while thereduce
method aggregates these rankings. -
The configuration of the job is crucial; using a
Configuration
object allows for the customization of various parameters that can improve performance.
Troubleshooting Steps
Step 1: Monitor Cluster Metrics
AWS provides CloudWatch to monitor the performance of your EMR cluster. Check the following metrics:
- CPU Utilization: High CPU usage can indicate that your tasks are getting stuck on computation.
- Memory Usage: If memory usage is peaking towards 100%, jobs may be failing due to lack of resources.
- Disk I/O: Excessive read/write operations can slow down your jobs dramatically.
By identifying resource bottlenecks, you can take action, such as resizing instances or increasing memory allocation.
Step 2: Optimize the Input Dataset
The structure of your input dataset is critical. Consider:
- Partitioning your data properly to avoid skewness. Uneven data distribution can affect how data is processed across nodes.
- Use the compression of the input files to speed up processing times. For example, using Snappy or Gzip can reduce I/O times significantly.
Step 3: Tune EMR Settings
AWS EMR offers numerous configuration options. Here are some critical parameters:
- Yarn Scheduler: Consider using the Capacity or Fair Scheduler to optimize how resources are allocated across various jobs.
- EC2 Instance Types: Use instances optimized for high CPU or memory usage. For instance, r5.xlarge or c5.xlarge might be more efficient than the default m5.large.
- Number of Partitions: Adjust the levels of parallelism by increasing the number of partitions used for the data. The more partitions, the more parallel processing can occur.
Step 4: Inspect the Code
Examine your PageRank implementation thoroughly:
- Is your map function overly complex?
- Are you locally storing intermediate results? If so, consider external solutions like HDFS for better scalability.
- Ensure that your algorithms are implemented efficiently, avoiding any nested loops that could lead to increased processing time.
Step 5: Review Log Files
While AWS EMR provides metrics, sometimes you need a closer look. Utilize the Hadoop logging feature to dive into application logs:
- Errors might give clues about operations that are causing delays.
- Look for any warnings or error messages related to resource allocation and shuffling.
Additional Resources
For further reading on optimizing AWS EMR and Hadoop job performance, consider the following resources:
Wrapping Up
By utilizing these troubleshooting techniques, you can effectively address slow PageRank jobs on AWS EMR. Remember, efficiency often comes down to a combination of optimal configurations, monitoring, and effective coding practices. In the world of big data, small optimizations can lead to significant performance improvements. Take the time to assess your setup, and you will reap the rewards in processing speed and resource management.
With the complexity of Hadoop and massive datasets, troubleshooting can be daunting, but understanding the intricate details will position you for success on your data-processing journey. Happy coding!