Mastering Closures: Common Pitfalls to Avoid in JavaScript
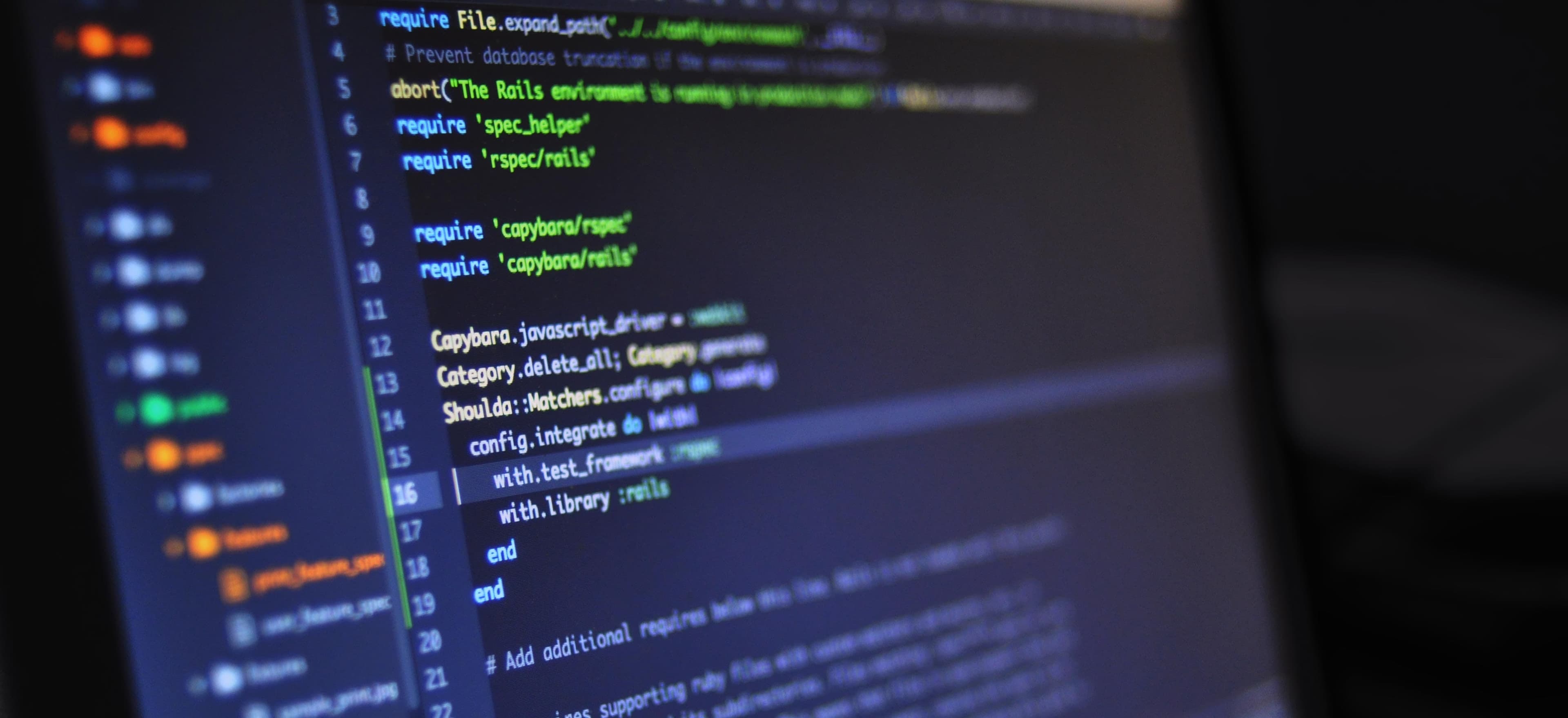
- Published on
Mastering Closures: Common Pitfalls to Avoid in JavaScript
JavaScript is a powerful language that incorporates a variety of concepts, one of which is closures. While closures empower developers to create robust and flexible functions, they can also be a source of confusion and bugs if not understood correctly. In this post, we will delve into the intricacies of closures, explore common pitfalls, and provide you with practical examples to enhance your JavaScript skill set.
What Are Closures?
A closure is created when a function captures the lexical scope in which it was defined, even when it is executed outside that scope. This means a function can remember and access its lexical scope even when the function is executed outside that scope.
Example of a Closure
Let's look at a simple example to understand closures:
function outerFunction() {
let outerVariable = 'I am from outer scope';
function innerFunction() {
console.log(outerVariable);
}
return innerFunction;
}
const myFunction = outerFunction();
myFunction(); // Outputs: I am from outer scope
Why Are Closures Useful?
Closures can be remarkably useful in many scenarios:
- Data Privacy: You can create private variables.
- Partial Application: Closures help in the creation of partially applied functions.
- Maintaining State: They allow you to maintain state in asynchronous programming.
- Functional Programming: Closures support functional programming paradigms effectively.
Common Pitfalls with Closures
While closures are powerful, there are instances where misuse can lead to bugs or performance issues. Here are several common pitfalls.
1. Unintended Variable Capture
The first pitfall arises from the way closures capture variables. If a closure captures a variable from its enclosing scope that changes during the function's lifecycle, it can lead to unexpected outcomes.
Example
function createCounter() {
let count = 0;
return function() {
count++;
return count;
}
}
const counter = createCounter();
console.log(counter()); // Outputs: 1
console.log(counter()); // Outputs: 2
In this example, the closure consistently returns the updated count
value because it captures the variable by reference, rather than by value.
Pitfall Explanation
If you use a loop with a closure, it may not behave as expected:
for (var i = 0; i < 3; i++) {
setTimeout(function() {
console.log(i);
}, 1000);
}
// Outputs: 3, 3, 3
This occurs because i
is captured as a reference. By the time the timeout runs, i
has already reached the loop's endpoint.
Solution
To avoid unintended captures from loops, utilize let
, which creates a block scope:
for (let i = 0; i < 3; i++) {
setTimeout(function() {
console.log(i);
}, 1000);
}
// Outputs: 0, 1, 2
2. Memory Leaks
An unintended closure can lead to memory leaks. When closures persist longer than necessary, they keep references to objects, preventing them from being garbage collected.
Example
function createLargeObject() {
const largeObject = new Array(1000000).fill('*');
return function() {
console.log(largeObject[0]);
}
}
const largeObjectClosure = createLargeObject();
// largeObject is now in memory, even though we don't need it anymore.
Solution
To mitigate this, relinquish references to larger objects when not needed.
function createLargeObject() {
const largeObject = new Array(1000000).fill('*');
return function() {
console.log(largeObject[0]);
// Clear the reference to largeObject
largeObject.length = 0;
}
}
3. Accidental Global Variables
Using closures incorrectly can also lead to the creation of global variables. This typically occurs when omitting the var
, let
, or const
keyword.
Example
function createGlobalVariable() {
globalVar = 'I am global'; // Accidentally creating a global variable
}
createGlobalVariable();
console.log(globalVar); // Outputs: I am global
Solution
Always declare variables with let
, const
, or var
:
function createScopedVariable() {
let scopedVar = 'I am scoped';
return function() {
console.log(scopedVar);
}
}
4. Overusing Closures
While closures can significantly optimize our code, overusing them can lead to confusing designs. Keeping code simple is often the best approach.
When to Use Closures
- To create private variables.
- When a function needs to remember its environment.
- In functional programming patterns or event handling.
Best Practices for Using Closures
- Be Mindful of Scope: Understand the scopes your closures are capturing.
- Clear References: Avoid memory leaks by ensuring large objects don’t linger in memory longer than needed.
- Minimize Nested Closures: Keep your functions clean and not overly nested unless required.
- Document Your Intentions: When using closures, provide documentation to clarify expected behavior.
Wrapping Up
Closures are an invaluable part of JavaScript that enable developers to create more maintainable and flexible code. They allow for encapsulation, data privacy, and a host of other functional programming benefits. However, as with all powerful tools, they come with their share of pitfalls. By understanding these pitfalls, along with implementing best practices, you can effectively use closures without introducing bugs or performance issues.
For further reading, explore MDN Web Docs on Closures or check out JavaScript.info: Closure for a deeper dive.
By mastering closures and being mindful of their pitfalls, you will become a more efficient and effective JavaScript developer, armed with the knowledge to avoid the common traps and create cleaner, safer code. Happy coding!