Overcoming Challenges in Java Equivalence Testing
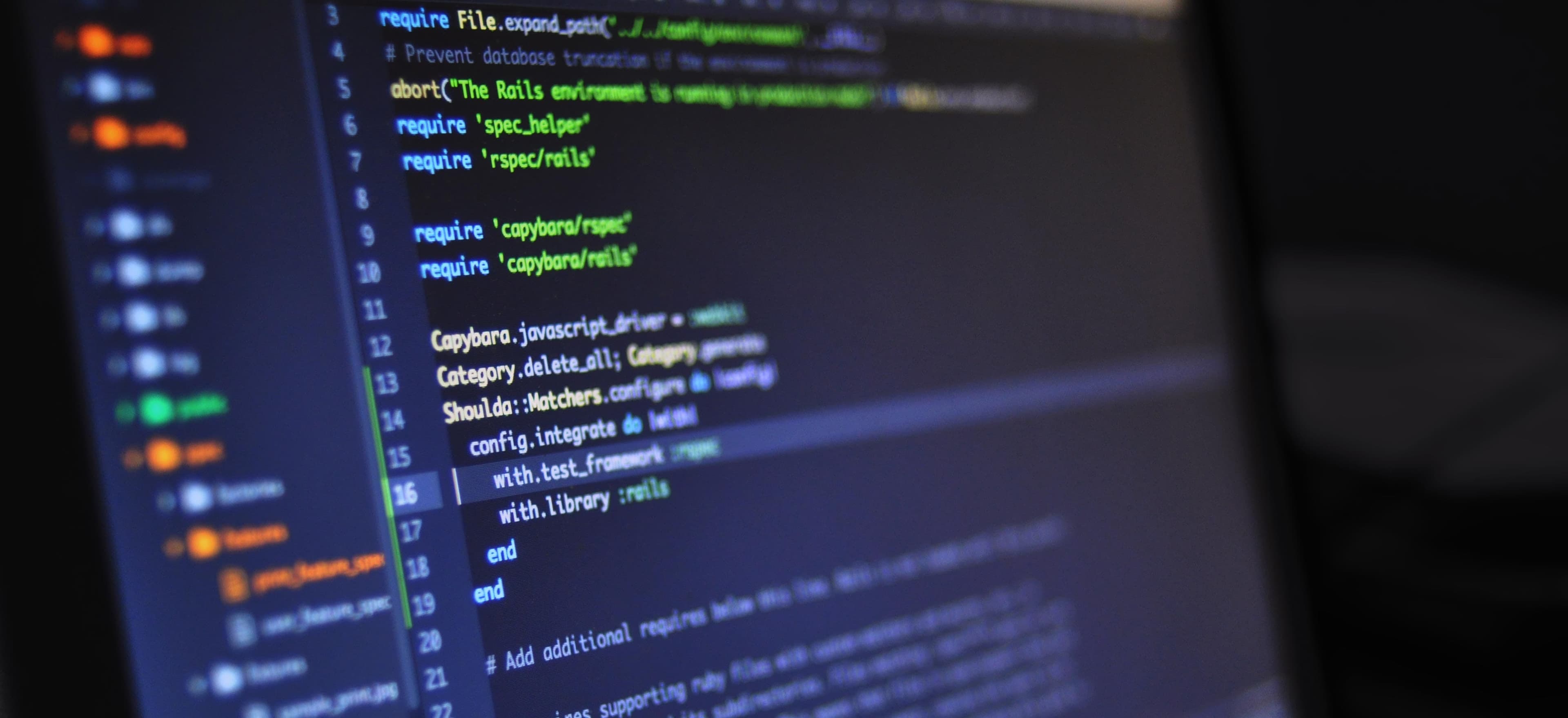
- Published on
Overcoming Challenges in Java Equivalence Testing
Java is one of the most widely used programming languages, known for its portability, performance, and extensive support for libraries. However, when developing robust applications, testing becomes crucial, especially equivalence testing. This blog post will explore the challenges associated with Java equivalence testing and provide strategies to overcome them.
What is Equivalence Testing?
Equivalence testing is a software testing methodology used to determine whether different inputs produce the same output. It plays a significant role in ensuring that the Java application is functioning correctly under various scenarios.
In Java, equivalence testing can help identify how the code behaves with various data types, including primitive data types, strings, and user-defined objects. This is critical for:
- Validating business logic
- Ensuring system performance
- Maintaining system stability
Understanding the basic principles of equivalence testing will help to identify when and where these tests need to be applied.
Challenges in Equivalence Testing
While Java provides many tools and frameworks for testing, equivalence testing comes with its own set of challenges. Here are a few:
1. Complex Object Comparisons
In Java, comparing objects is not as straightforward as comparing primitive data types. Consider the following code snippet:
public class Person {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (!(obj instanceof Person)) return false;
Person person = (Person) obj;
return this.age == person.age && (this.name != null ? this.name.equals(person.name) : person.name == null);
}
@Override
public int hashCode() {
return Objects.hash(name, age);
}
}
In this example, the equals
method is overridden to compare two Person
objects based on their name and age attributes. Failing to implement the equals
and hashCode
methods appropriately can lead to flawed test cases.
Solution
To tackle complex object comparisons, ensure you:
- Override both
equals
andhashCode
in custom objects. - Use libraries like Apache Commons Lang or Guava to simplify comparisons using utility methods.
2. Handling Null Values
In Java, null values can lead to unexpected behavior during equivalence testing. For instance:
public class EquivalenceTester {
public static void main(String[] args) {
String str1 = null;
String str2 = null;
System.out.println(str1.equals(str2)); // Throws NullPointerException
}
}
This code causes a NullPointerException
, as null cannot invoke methods.
Solution
Use null-safe comparisons to handle null values gracefully:
public static boolean areEqual(String a, String b) {
return a == null ? b == null : a.equals(b);
}
This method ensures that both references are compared safely, allowing for null values without crashing the application.
3. Immutable vs Mutable Objects
Java provides both immutable (like String
) and mutable classes (like most collection classes). This can complicate equivalence testing. Mutable objects can change state between tests, leading to inconsistent results.
Solution
Prefer using immutable objects or clone mutable objects when performing tests. For example, using the Collections
class to create an unmodifiable list:
List<String> originalList = new ArrayList<>();
originalList.add("Item1");
List<String> unmodifiableList = Collections.unmodifiableList(originalList);
This ensures that your test data remains constant throughout the testing process.
4. External Dependencies
Java applications often interact with external systems (databases, APIs, etc.). Such dependencies can inject variability into tests, thereby complicating equivalence testing.
Solution
To manage external dependencies:
- Use mocking frameworks like Mockito to create test doubles.
- Implement stubs or fakes for external interactions.
Here's a simple example using Mockito:
import static org.mockito.Mockito.*;
public class UserService {
private final UserRepository userRepository;
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public User findUser(String id) {
return userRepository.findById(id);
}
}
// In your test class
UserRepository mockRepository = mock(UserRepository.class);
UserService userService = new UserService(mockRepository);
when(mockRepository.findById("123")).thenReturn(new User("John Doe"));
Mocking allows you to test the UserService
without relying on a real database, making your tests more reliable.
Best Practices for Equivalence Testing in Java
1. Use Test Frameworks
Frameworks like JUnit provide excellent support for writing and running tests. Additionally, many IDEs come equipped with integrated testing tools.
2. Keep Tests Isolated
Ensure that each test is independent to minimize the impact of external factors. This will help in maintaining consistency in your tests.
3. Document Test Cases
Clearly document test cases for future developers and maintainers. This will help provide context and rationality behind your equivalence tests, simplifying maintenance when your code base evolves.
4. Continuous Integration
Integrate your tests into a Continuous Integration (CI) pipeline. Tools like Jenkins or GitHub Actions can automatically run tests with every commit, ensuring that all equivalence tests are executed continuously.
In Conclusion, Here is What Matters
Equivalence testing in Java is a vital part of the software development lifecycle, but it comes with its own set of challenges. With proper understanding and application of best practices, you can effectively navigate these challenges.
To summarize:
- Ensure to implement the
equals
andhashCode
methods correctly. - Use null-safe comparisons and avoid mutable object states during tests.
- Leverage external tools and libraries to mock dependencies.
By focusing on these aspects, your Java application will not only be robust but also reliable and maintainable in the long term. Happy coding!
For more information on testing strategies, consider checking out JUnit documentation and Effective Java by Joshua Bloch.