Common Pitfalls in Structuring Your Akka Base Project
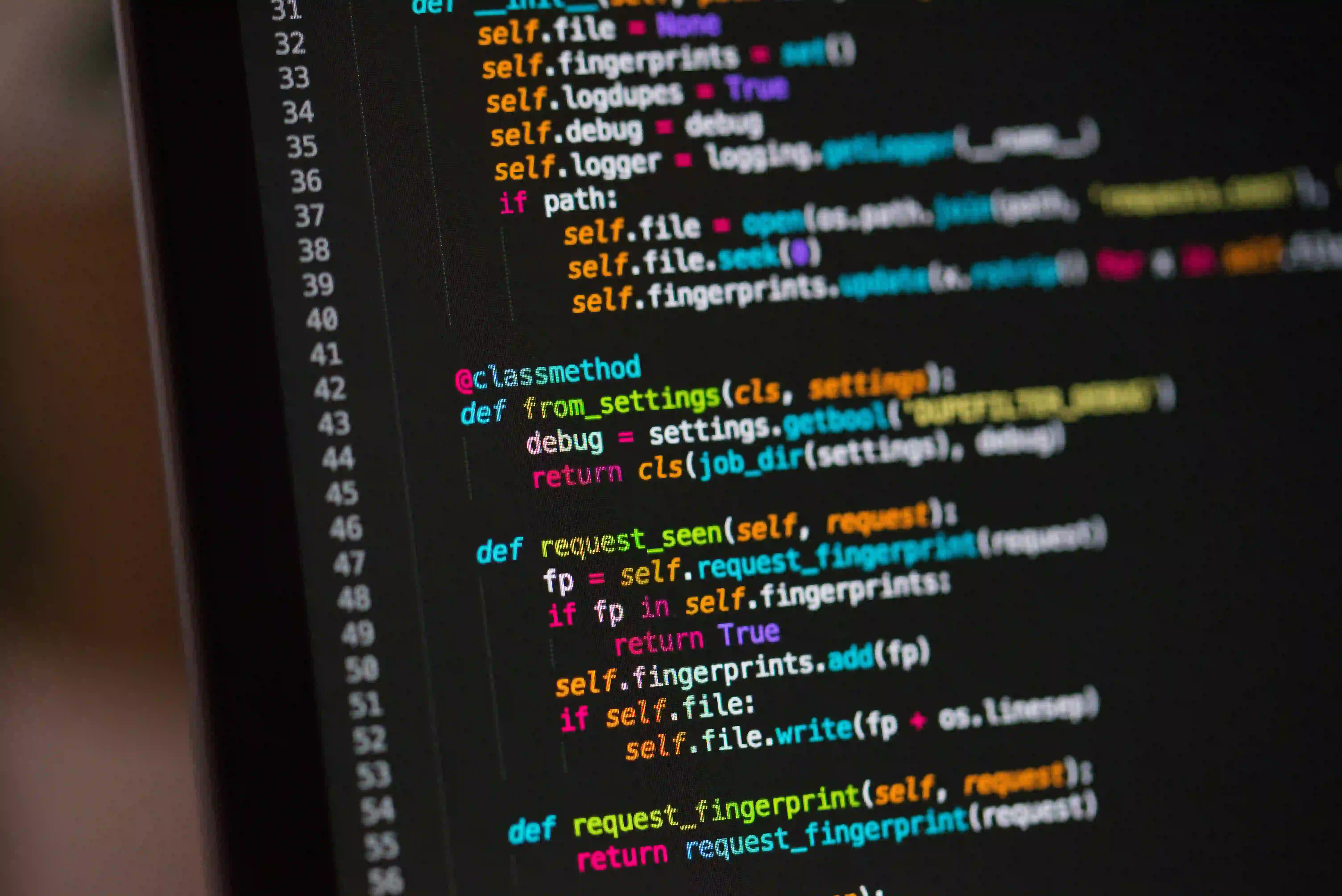
Common Pitfalls in Structuring Your Akka Base Project
When starting with Akka, a toolkit for building highly concurrent, distributed, and resilient message-driven applications, developers often face structural challenges that can make or break the success of their projects. This blog post discusses the common pitfalls in structuring an Akka base project and provides insights into how to avoid them.
Understanding Akka’s Actor Model
Before diving into project structure, it’s essential to grasp the foundational concepts of Akka's Actor Model. The Actor Model enables you to design your application as a collection of independent actors that communicate via asynchronous messages. This design pattern promotes scalability and fault tolerance.
Key Principles of Akka Actors:
- Encapsulation: Each actor has its own state and behavior.
- Asynchronous Communication: Actors do not call each other directly; they send messages instead.
- Location Transparency: Messages can be sent between actors regardless of their location in the distribution.
Pitfall 1: Monolithic Architecture
While it can be tempting to build an all-encompassing monolithic structure, this can lead to multiple issues, including difficulty in scaling and maintaining code. Instead, focus on creating a modular architecture.
Suggested Structure:
- Core Module: This should contain the business logic.
- Actor Module: Define your actors here.
- Service Module: Facilitate the interaction between actors and external systems.
- API Module: If your application exposes a REST or gRPC interface, keep it here.
Example Code:
// Core logic - UserService.scala
trait UserService {
def createUser(name: String): Future[User]
}
// Actor - UserActor.scala
class UserActor(userService: UserService) extends Actor {
def receive: Receive = {
case CreateUser(name) =>
userService.createUser(name).pipeTo(sender)
}
}
Why This Matters: By separating concerns, you not only improve maintainability but also facilitate testing and reusability.
Pitfall 2: Ignoring the Supervision Strategy
In Akka, actors can fail, but how you handle failures can make a significant difference. Developers often underestimate the importance of supervision strategies.
Recommended Strategies:
- One-for-One Strategy: Supervise individual actors; restart them on failure while allowing others to continue.
- All-for-One Strategy: If one actor fails, all its sibling actors are affected.
Example Code:
// Supervision Strategy - UserSupervisor.scala
class UserSupervisor extends Actor {
override val supervisorStrategy: SupervisorStrategy = OneForOneStrategy() {
case _: Exception => Resume
}
def receive: Receive = {
case createUser: CreateUser => // Create a child UserActor
}
}
Why This Matters: A well-thought-out supervision strategy ensures that your system remains resilient, greatly reducing downtime.
Pitfall 3: Overcomplicating Message Protocols
The message protocol among actors should be straightforward. Complicating this can lead to confusion and maintenance challenges. Instead, design a clean message interface.
Suggested Approach:
- Use case classes for messages.
- Keep messages immutable.
- Define messages in a dedicated package.
Example Code:
// Message Protocol - Messages.scala
case class CreateUser(name: String)
case class UserCreated(id: String)
// Actor Usage
case class UserActor extends Actor {
def receive: Receive = {
case CreateUser(name) =>
val userId = // save user and return ID
sender() ! UserCreated(userId)
}
}
Why This Matters: A well-defined message protocol reduces the chances of errors and enhances clarity between actors.
Pitfall 4: Overlooking Testing Strategies
Testing is crucial. However, many developers skip or neglect this aspect. In Akka, both unit tests for your actors and integration tests for your system are necessary.
Recommended Testing Frameworks:
- ScalaTest: Use for unit testing your actors.
- Akka TestKit: For testing interactions between actors.
Example Test Code:
// Actor Test - UserActorSpec.scala
class UserActorSpec extends TestKit(ActorSystem("UserActorSpec"))
with WordSpecLike with MustMatchers with AfterAll {
override def afterAll() {
TestKit.shutdownActorSystem(system)
}
"UserActor" should {
"create a user and respond with UserCreated" in {
val userActor = system.actorOf(Props[UserActor])
val probe = TestProbe()
probe.send(userActor, CreateUser("John Doe"))
probe.expectMsgType[UserCreated]
}
}
}
Why This Matters: Implementing solid testing strategies helps catch issues early and builds confidence in your application's stability.
Pitfall 5: Poor Configuration Management
Configuration management is an often-overlooked aspect of an Akka project. Hardcoding configurations can lead to inefficiencies and maintenance challenges.
Best Practices:
- Utilize Typesafe Config to manage configurations.
- Separate configurations for different environments (development, testing, production).
Example Configuration File:
# application.conf
akka {
loglevel = "DEBUG"
actor {
default-dispatcher {
throughput = 100
}
}
}
Why This Matters: Proper configuration management improves flexibility and adaptability in various environments.
In Conclusion, Here is What Matters
A well-structured Akka base project can elevate your application's capability to handle concurrency, distribution, and resilience effectively. By avoiding common pitfalls, such as monolithic architecture, improper supervision strategies, complex message protocols, inadequate testing, and poor configuration management, you can build a robust system.
Further Reading
- Akka Documentation
- Actor Model Fundamentals
With these insights and best practices, you are now better equipped to tackle the complexities of structuring your Akka base project. Building efficient, maintainable, and scalable applications is all about the right design choices from the very beginning. Happy coding!