Mastering Java: Convert File Contents to String Effortlessly
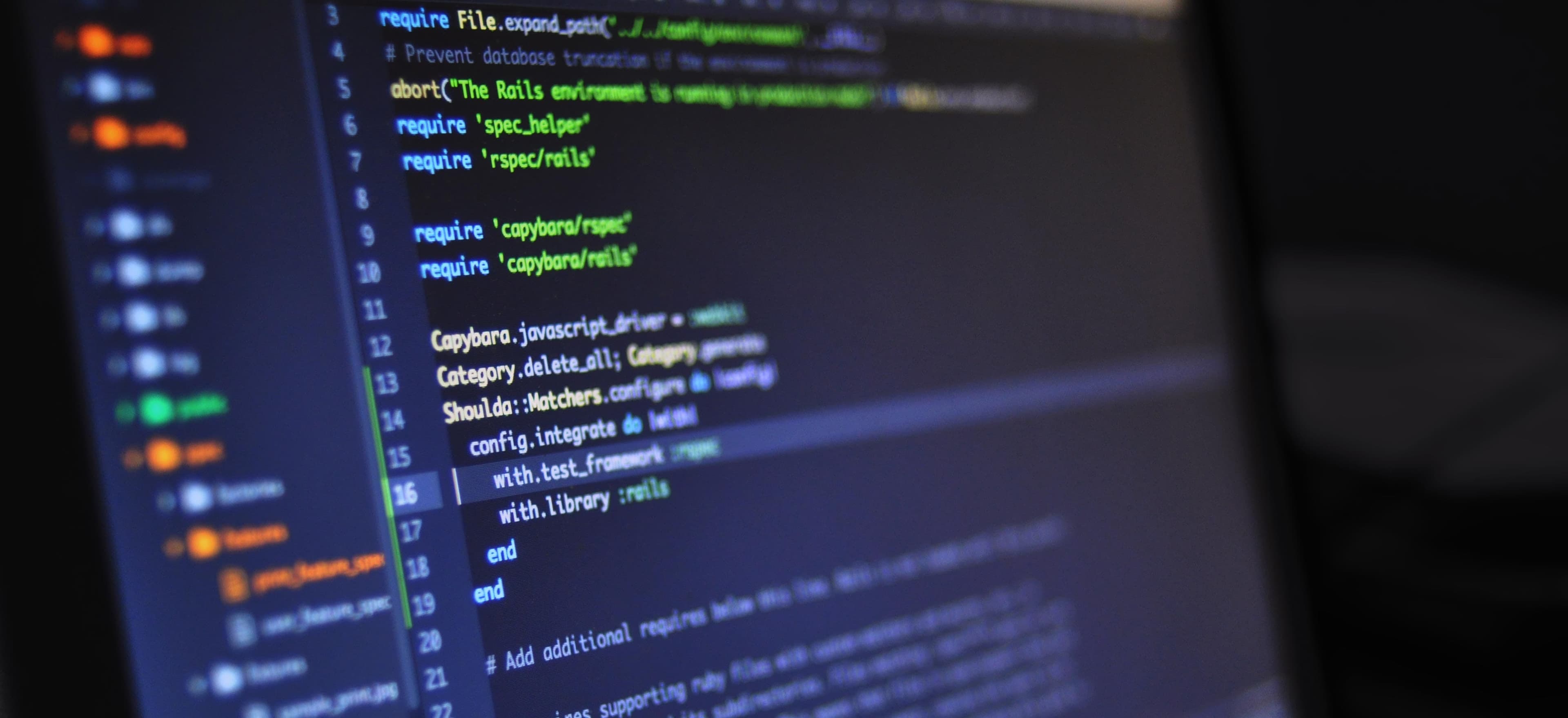
- Published on
Mastering Java: Convert File Contents to String Effortlessly
Java has long been recognized as a powerful, versatile programming language ideal for a wide range of applications. One task that regularly comes up in software development is reading the content of files. In this blog post, we will explore how to convert file contents to a String in Java, adding depth to our understanding of file handling while also keeping it straightforward and effective.
Why Read Files in Java?
Before we delve into the code, it is vital to understand the context for reading files. Files are ubiquitous in application development—they store configuration settings, user data, logs, and much more. Consequently, developers require robust means to handle file I/O (Input/Output) operations effectively.
Having an effortless way of reading files enhances productivity and application performance. In this discussion, we'll look at different methods to read file contents as a String and consider scenarios when each method is the most applicable.
Methods to Read File Content
Java offers several methods to read file contents. Here are a few common approaches:
- Using
BufferedReader
- Using
Files
Class (Java NIO) - Using File Input Stream
Each method has its benefits and ideal usage scenarios. Let’s discuss them one by one, providing exemplary code snippets to illustrate each approach.
1. Using BufferedReader
The BufferedReader
class is a part of java.io
package. It is often used for reading text from a character-based input stream. One main advantage of using BufferedReader
is that it reads a large chunk of data at once, improving performance for larger files.
Example Code
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class ReadFileWithBufferedReader {
public static void main(String[] args) {
String filePath = "example.txt";
// StringBuilder collects the entire content.
StringBuilder content = new StringBuilder();
try (BufferedReader br = new BufferedReader(new FileReader(filePath))) {
String line;
while ((line = br.readLine()) != null) {
content.append(line).append("\n"); // Append each line with a newline
}
} catch (IOException e) {
e.printStackTrace();
}
System.out.println(content.toString());
}
}
Commentary
-
Try-with-resources: The
try-with-resources
statement is a feature that ensures that each resource is closed at the end of the statement. It helps avoid memory leaks. -
Line by Line: Reading the file line by line minimizes memory consumption. Each line is appended to the
StringBuilder
to form the complete content.
2. Using Files
Class (Java NIO)
Java NIO (New I/O) introduced in Java 7 offers a simpler and more efficient way to handle file operations. The Files
class is particularly powerful, and it includes several static methods for file manipulation.
Example Code
import java.nio.file.Files;
import java.nio.file.Paths;
import java.io.IOException;
public class ReadFileWithFiles {
public static void main(String[] args) {
String filePath = "example.txt";
try {
// Read all bytes and convert byte array to String
String content = new String(Files.readAllBytes(Paths.get(filePath)));
System.out.println(content);
} catch (IOException e) {
e.printStackTrace();
}
}
}
Commentary
-
Read All Bytes:
Files.readAllBytes
reads the entire content of the file at once, making it efficient for smaller files. -
Simplicity: This method is more concise than using
BufferedReader
, especially when needing the entire file as a String with minimal code.
For those looking for further exploration into Java NIO, refer to the official documentation here.
3. Using FileInputStream
FileInputStream
is part of Java's original I/O classes. It is useful for reading raw byte streams, making it suitable for binary data. For reading text files, however, it is less convenient than the previous methods.
Example Code
import java.io.FileInputStream;
import java.io.IOException;
import java.nio.charset.StandardCharsets;
public class ReadFileWithFileInputStream {
public static void main(String[] args) {
String filePath = "example.txt";
StringBuilder content = new StringBuilder();
try (FileInputStream fis = new FileInputStream(filePath)) {
byte[] data = new byte[fis.available()]; // Read all available data
fis.read(data); // Read data into the byte array
content.append(new String(data, StandardCharsets.UTF_8)); // Convert byte array to String
} catch (IOException e) {
e.printStackTrace();
}
System.out.println(content.toString());
}
}
Commentary
-
Byte Processing: This approach reads data as bytes and converts them into a String using proper character encoding (UTF-8 in this case).
-
Less Efficient: Although this method can work with text files, it is generally considered less efficient than
BufferedReader
orFiles
.
Choosing the Right Method
The choice of method primarily depends on the use case:
-
Small to Medium Text Files: If your file size is reasonable and you want simple, clear code, using the
Files
class is recommended. You can read the entire file at once for quick processing. -
Larger Files: For larger files, using a
BufferedReader
is more efficient as it prevents high memory usage by reading the file line by line. -
Binary Files: If dealing with binary data files,
FileInputStream
would be the most suitable.
Error Handling
Always remember that reading from files can fail for various reasons: the file may not exist, permission issues, or disk failures. Thus, implementing robust error handling is critical. Using try-catch
blocks can help manage exceptions effectively.
Bringing It All Together
Java provides various methods to read file contents into a String, each with distinct advantages suited for different scenarios. From buffering lines of text with BufferedReader
to using the intuitive Files
class, Java facilitates seamless file handling.
Mastering file I/O operations in Java is essential for every developer. Explore more about Java file handling with comprehensive resources, such as Oracle's official documentation.
Now it's your turn! Experiment with these techniques by trying to read larger files or even parse CSVs. Understanding file handling is an important step in becoming a proficient Java developer. Happy coding!