Mastering Java and Scala Synchronization: Common Pitfalls!
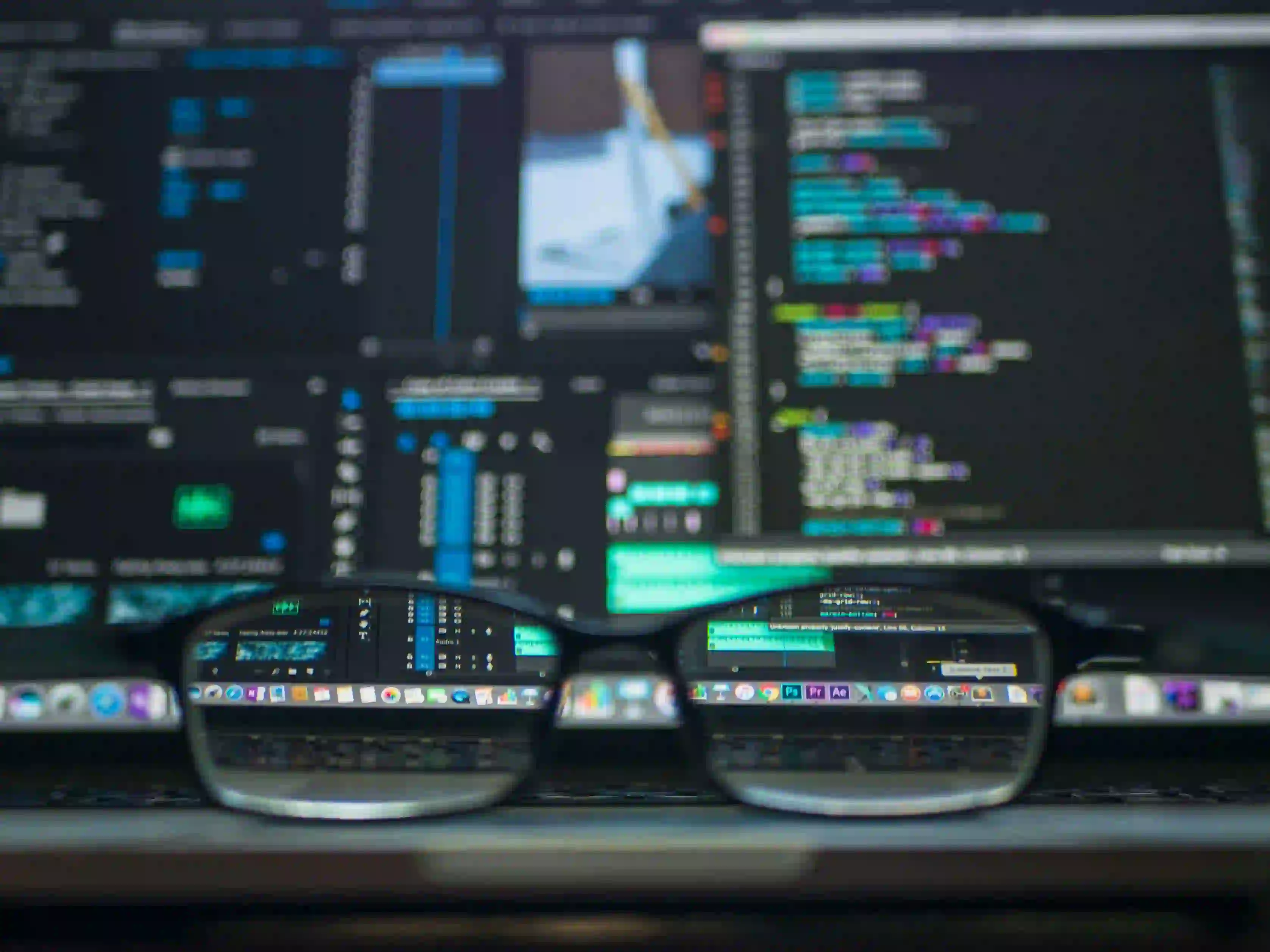
Mastering Java and Scala Synchronization: Common Pitfalls
In the world of concurrent programming, synchronization is crucial for maintaining data integrity and preventing race conditions. This is particularly true in languages like Java and Scala, which offer developers powerful concurrency tools. However, achieving efficient synchronization can be a labyrinth of pitfalls. Here, we'll explore the common pitfalls in synchronization within Java and Scala and provide practical solutions to avoid these issues.
Understanding Synchronization
Synchronization is a mechanism that ensures that two or more concurrent processes or threads do not simultaneously execute some particular program segment. It is key to protecting shared resources.
Why Synchronization is Important
- Data Integrity: Ensures that the same data isn't modified by different threads at the same time.
- Deadlocks: Helps prevent situations where threads become stuck waiting for each other.
- Order of Execution: Provides a structured way to guarantee the order of operations among threads.
Java Synchronization: Common Pitfalls
Java provides multiple ways to manage synchronization, including synchronized methods, synchronized blocks, and the Lock interface provided in the java.util.concurrent
package. However, common pitfalls can lead to issues.
1. Overusing Synchronized Methods
Using synchronized methods can lead to performance issues, especially if they are frequently called. It locks the entire method, which can delay the execution of other threads considerably.
Example:
public synchronized void increment() {
count++;
}
Why Not? While synchronized, multiple threads cannot run this method, potentially leading to a bottleneck. Instead, consider synchronizing only critical sections of your code.
2. Ignoring Fine-Grained Locking
Instead of using a single lock for the entire resource, consider using multiple locks. This allows finer control and can significantly improve performance.
Example:
class Counter {
private final Object lock1 = new Object();
private final Object lock2 = new Object();
public void incrementCounter1() {
synchronized (lock1) {
// Critical section for counter 1
counter1++;
}
}
public void incrementCounter2() {
synchronized (lock2) {
// Critical section for counter 2
counter2++;
}
}
}
Why? This approach allows different threads to access different counters without waiting for each other, ultimately leading to better throughput.
3. Neglecting Thread States and Deadlocks
Deadlocks can occur if two or more threads are waiting on conditions that will never be satisfied. Properly managing thread states and ensuring that a strict ordering is followed on acquiring locks is essential.
Example of a potential deadlock:
class A {
public synchronized void methodA(B b) {
b.last();
}
}
class B {
public synchronized void methodB(A a) {
a.last();
}
}
Why Not? Here, if Thread 1 holds a lock on A's method and waits for B, while Thread 2 holds a lock on B's method and waits for A, a deadlock occurs. Always try to define a predefined order when acquiring multiple locks.
4. Using wait() and notify() Incorrectly
In some situations, developers may use wait()
and notify()
improperly. Always remember to wrap these calls in a loop, as notifications can sometimes occur before a wait condition is valid.
Example:
synchronized (obj) {
while (conditionIsTrue) {
obj.wait();
}
// perform action
}
Why? By wrapping the wait in a loop, you can ensure that when the thread is notified, it only continues if the condition is indeed valid.
Scala Synchronization: Common Pitfalls
Scala provides many synchronization primitives, and also offers higher-level constructs like Futures
and Actors
. However, pitfalls can emerge from both the usual suspects in Java and due to Scala's functional programming nature.
5. Using Mutable State
Mutable state in concurrent applications in Scala can create race conditions. Prefer using immutable data whenever possible.
Example:
var counter = 0
def increment(): Unit = {
counter += 1 // Mutable state is a problem!
}
Why Not? Using mutable state can lead to unpredictable behavior in multi-threaded scenarios. Instead, opt for immutable structures or AtomicInteger
.
6. Relying Solely on synchronized
keyword
Just like in Java, solely using the synchronized
keyword is not efficient in all cases. Consider using java.util.concurrent
package or Scala’s ReentrantLock
for more advanced locking mechanisms.
Example:
val lock = new ReentrantLock()
lock.lock()
try {
// Critical section
} finally {
lock.unlock()
}
Why? This provides better control over how and when you acquire and release the lock, as opposed to using synchronized blocks.
7. Not Handling Futures Correctly
When using Scala's Futures
, neglecting to handle exceptions can cause your application to misbehave. Always account for failures.
Example:
val futureResult = Future {
// some computation
}
futureResult.onComplete {
case Success(value) => println(s"Got the result: $value")
case Failure(e) => println(s"An error occurred: ${e.getMessage}")
}
Why? This pattern ensures that any exceptions occurring inside the Future
are caught and handled properly, which is essential for building robust applications.
Final Thoughts
Mastering synchronization in Java and Scala is no small feat. While the pitfalls we discussed can hinder your application's performance and reliability, being aware of them can help you design better systems.
By using best practices such as avoiding over-synchronization, embracing fine-grained locks, and usability of functional constructs, you can create efficient, thread-safe applications.
If you're looking to deepen your understanding further, consider exploring resources like Java Concurrency in Practice by Brian Goetz for Java or Programming in Scala to understand how to better tackle concurrency in Scala.
Remember, the journey of mastering synchronization is a continuous learning process. Embrace the challenges, and your programs will reward you with stability and performance. Happy coding!