Why Choose Server-Side Rendering for Your AngularJS App?
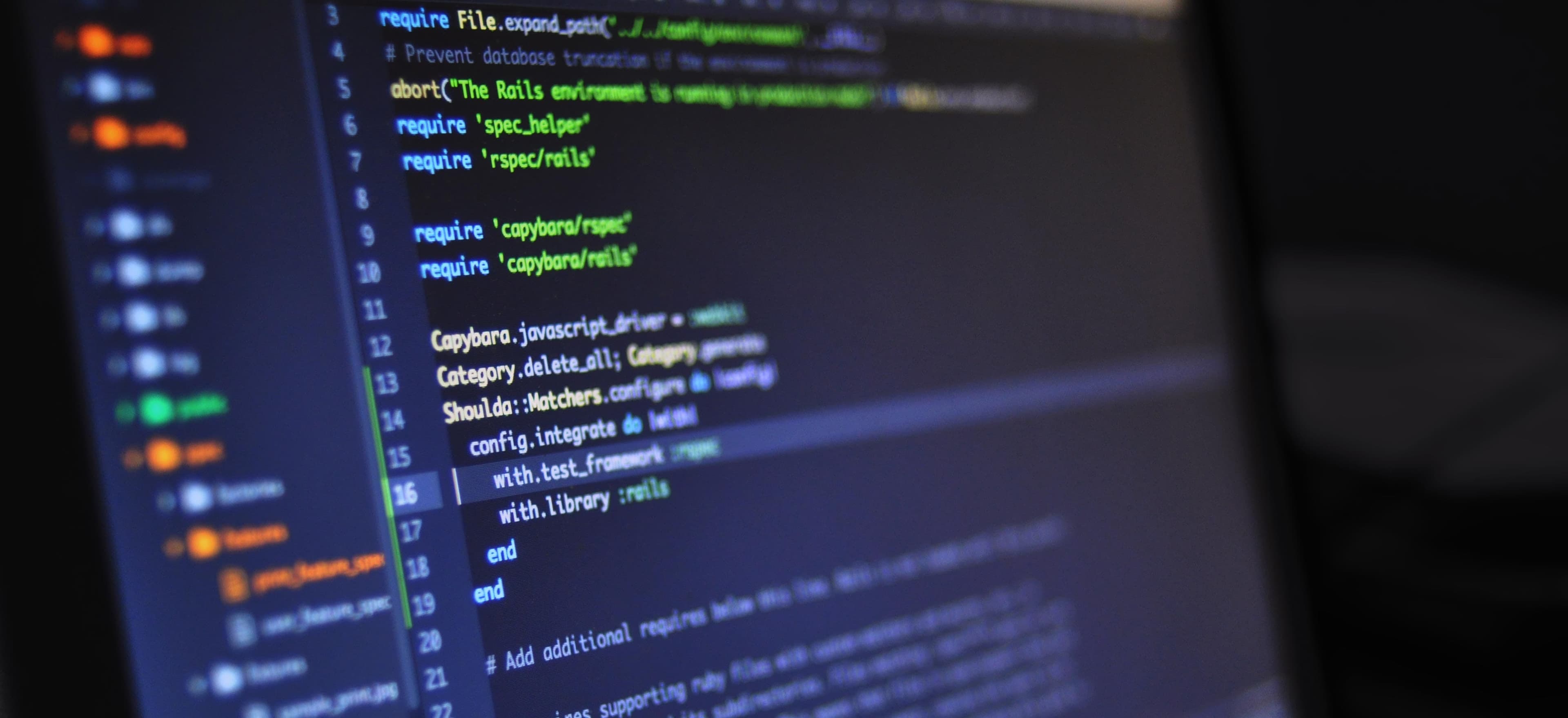
- Published on
Why Choose Server-Side Rendering for Your AngularJS App?
As web applications become increasingly complex, performance and user experience are paramount. AngularJS, a popular front-end framework, provides flexibility and power, but sometimes it needs a little help to ensure optimal performance. This is where Server-Side Rendering (SSR) comes into play. In this blog post, we will explore the benefits of implementing SSR in your AngularJS application, how it enhances performance, and provide code snippets to help you understand this approach better.
What is Server-Side Rendering?
Server-Side Rendering is a technique where the server generates the HTML for a web page and sends it to the client. Unlike traditional client-side rendering, where the browser requests JavaScript files and builds the page in the client's environment, SSR allows for the initial HTML to be fully rendered on the server. This means users receive a complete webpage upon their first request—saving time and improving the user experience from the outset.
Why is SSR Important?
- Improved Performance: Users receive a fully rendered page, speeding up the time to first paint (TTFP).
- Better SEO: Search engines can crawl and index the fully rendered HTML content, resulting in better visibility in search results.
- Enhanced User Experience: Users see content faster, making the application feel more responsive.
Getting Started with SSR in AngularJS
Implementing SSR in your AngularJS application typically involves the use of libraries like Angular Universal or custom server setups.
Example Implementation
Let's walk through a simple setup using Node.js and express to create an SSR-compatible AngularJS application.
Step 1: Setting Up the Environment
First, make sure you have Node.js installed on your machine. Next, create a new directory for your application and initialize a new Node project.
mkdir angularjs-ssr-example
cd angularjs-ssr-example
npm init -y
Step 2: Install Dependencies
Next, install Express, AngularJS, and any other necessary dependencies.
npm install express angular
Step 3: Create the Server
Create a file named server.js
and set up a basic Express server to serve your AngularJS application:
const express = require('express');
const path = require('path');
const app = express();
const PORT = process.env.PORT || 3000;
// Set static folder
app.use(express.static(path.join(__dirname, 'public')));
// SSR route
app.get('/', (req, res) => {
// Render HTML on server
const html = '<html><head><title>My AngularJS App</title></head><body><h1>Welcome to My SSR AngularJS App</h1></body></html>';
res.send(html);
});
// Start server
app.listen(PORT, () => {
console.log(`Server is running on http://localhost:${PORT}`);
});
In this snippet, we set up an Express server, serve static files, and create a simple route that returns HTML. The html
variable contains the initially rendered HTML that will be sent to the client.
Why We Did This
- Static Assets: By serving static files from the
public
directory, we enhance performance since static content can be cached. - Dynamic Routing: The server responds to specific paths, offering the ability to render different content based on the client's request.
Step 4: Rendering with AngularJS
Now let's integrate AngularJS into our application. You’ll typically want to include AngularJS in your public
directory and create an index file that initializes the Angular app.
public/index.html (Example)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>My AngularJS App</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.8.2/angular.min.js"></script>
<script src="app.js"></script>
</head>
<body ng-app="myApp">
<div ng-controller="MainController as main">
<h1>{{ main.title }}</h1>
</div>
</body>
</html>
public/app.js (Example)
angular.module('myApp', [])
.controller('MainController', function() {
this.title = 'Welcome to Server-Side Rendered AngularJS!';
});
This simple AngularJS application initializes with ng-app
and uses a controller to set the title on the page.
Why Use AngularJS in Conjunction with SSR?
- Dynamic Content: AngularJS excels at creating dynamic content that updates without full page reloads.
- Ease of Use: AngularJS has a simple learning curve, allowing developers to quickly build and manage their applications.
Key Benefits of SSR for AngularJS Apps
1. SEO Enhancement
As noted earlier, SSR improves SEO. AngularJS applications often struggle with search engine optimization due to their reliance on client-side rendering. By rendering HTML on the server, search engines can index your content correctly.
2. Faster Load Times
In a world where users expect quick load times, SSR delivers results. When users visit your site, they receive fully populated HTML, reducing load times significantly, especially on mobile devices.
3. Decreased Time to Interactive
Serving fully rendered HTML reduces the time it takes for the application to become interactive, thereby improving the overall user experience.
4. Universal Applications
With SSR, you can create a more universal application that works seamlessly across different devices and platforms. This capability is increasingly necessary as more users access web applications via mobile and tablets.
Challenges to Consider
While the benefits of SSR are substantial, there are challenges, including:
- Server Load: Rendering on the server can increase load, necessitating more powerful server infrastructure.
- Complexity: Introducing SSR to an existing AngularJS application can increase complexity. It’s important to plan the architecture carefully.
To Wrap Things Up
Server-Side Rendering significantly improves the performance, SEO, and user experience of AngularJS applications. While there are challenges to overcome, the benefits significantly outweigh the drawbacks. By using SSR, you can deliver fast, dynamic, and SEO-friendly applications that capture and retain user interest.
For further reading on optimizing AngularJS for performance, check out Google's Angular Performance Guide.
Implementing SSR in your AngularJS application may seem daunting, but as we've discussed, the payoff is well worth it. Start small, continuously optimize, and watch your application thrive in the competitive web landscape.
Feel free to share your experiences or ask questions in the comments below!
Checkout our other articles