Common MongoDB Authentication Issues and How to Fix Them
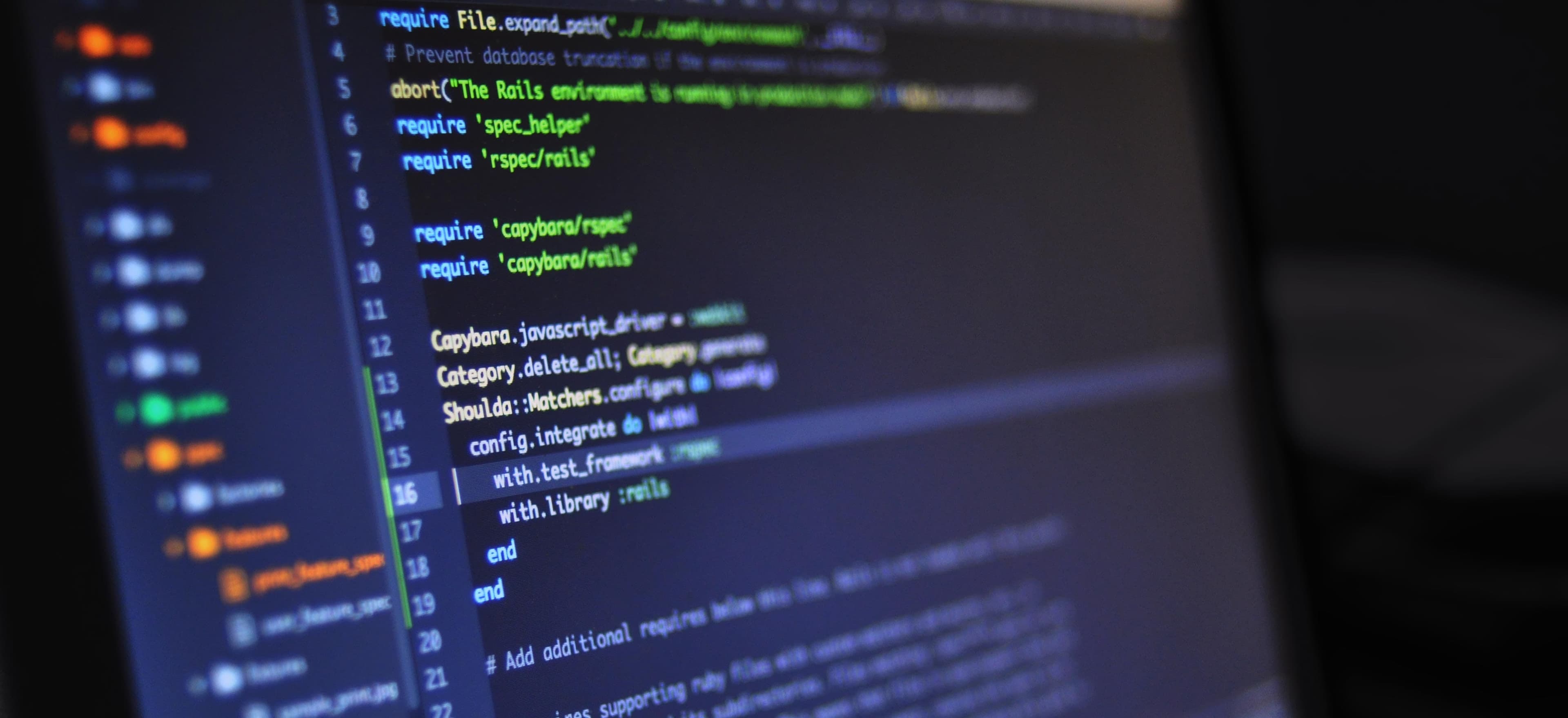
- Published on
Common MongoDB Authentication Issues and How to Fix Them
MongoDB, the popular NoSQL database, offers a flexible and powerful way to work with data. One of the crucial aspects of maintaining a secure database is user authentication. However, developers often encounter authentication issues that can prevent access to the database, causing significant delays in application development. In this blog post, we will explore common MongoDB authentication issues and provide actionable solutions to resolve them.
Understanding MongoDB Authentication
MongoDB provides various authentication mechanisms to ensure that only authorized users have access to the database. These mechanisms include:
- SCRAM (Salted Challenge Response Authentication Mechanism): The default authentication method.
- MONGODB-CR: An older method deprecated in MongoDB 4.0.
- x.509 Certificates: For client authentication using SSL/TLS.
- LDAP: For integration with existing directory services.
There are several factors that can lead to authentication issues, ranging from misconfigurations to network issues. Let's delve into some of the most common problems and their potential solutions.
Common Authentication Issues
1. Invalid Credentials
One of the most common errors encountered is the use of wrong username or password.
Solution
Double-check the username and password you are using. You can reset the password using the MongoDB shell with the following command:
use admin
db.updateUser("yourUsername", { pwd: "newPassword" })
This command is effective only if you have the privilege to change the user's password. Always ensure that you have the proper access rights.
2. Database Name Mismatch
Authentication issues can also occur if the database associated with the user is not specified correctly.
Solution
When creating users, you need to be aware of the database context in which they are created. To authenticate against a specific database, specify it in the connection string. If your user's credentials are tied to the ‘test’ database but you are attempting to connect to ‘admin’, you'll encounter issues.
Use this connection format:
mongodb://yourUsername:yourPassword@localhost:27017/test
3. Authentication Database Misconfiguration
MongoDB allows users to be created within a specific authentication database. If the client and server expect different databases for authentication, access will be denied.
Solution
Ensure you specify the authSource
parameter in your connection string:
mongodb://yourUsername:yourPassword@localhost:27017/test?authSource=admin
In this example, even if the user is created in the ‘admin’ database, they're authenticated against the ‘test’ database.
4. Wrong MongoDB URI Format
Using an incorrect URI format can lead to authentication protocol problems.
Solution
The MongoDB URI must follow specific structure rules. Refer to the official MongoDB URI documentation for correct formatting. Here is an example of a valid connection string:
mongodb://username:password@host:port/database
5. User Roles and Permissions
MongoDB users can be assigned various roles, and lacking appropriate permissions can lead to authentication errors.
Solution
Ensure that users have the correct roles assigned for the operations they are trying to perform. You can check existing user roles with:
use admin
db.getUsers()
If you find that a user lacks necessary permissions, you can assign roles like this:
db.grantRolesToUser("yourUsername", [{ role: "readWrite", db: "test" }])
6. Network Connectivity Issues
Sometimes, the connection might be denied due to a networking issue or firewall settings blocking the MongoDB server.
Solution
Check your firewall and network configurations to ensure that they allow connections to the MongoDB port (default is 27017). You can also use telnet
to verify connectivity:
telnet localhost 27017
If the command returns an error, further investigate your network settings.
7. MongoDB Configuration File Issues
Misconfigurations in the MongoDB configuration file (mongod.conf
) can lead to authentication problems.
Solution
Verify the authentication settings in your mongod.conf
. Make sure security.authorization
is set to enabled
for user authentication to function correctly.
security:
authorization: "enabled"
After making changes, restart the MongoDB service for the configurations to take effect.
8. Connection Using SSL/TLS without Proper Certificates
If you are attempting to connect to a replica set or shard cluster with SSL/TLS, and you have not provided the proper client certificates, you will face authentication issues.
Solution
Ensure you have the correct SSL certificates and include them in your connection string:
mongodb://yourUsername:yourPassword@host:port/?ssl=true&sslAllowInvalidCertificates=true
9. MongoDB Logs for Debugging
In both development and production environments, MongoDB logs can provide insight into authentication failures.
Solution
Examine the logs located typically in /var/log/mongodb/mongod.log
. Use commands like tail
to review real-time logs:
tail -f /var/log/mongodb/mongod.log
Look for entries related to authentication errors; they often include hints pointing towards what went wrong.
Closing Remarks
As discussed, MongoDB authentication issues can arise from a variety of factors including incorrect credentials, misconfiguration, or network limitations. Understanding these common pitfalls and their solutions can help streamline your development process and prevent downtime.
For additional reading and detailed information, check out the official MongoDB Security Documentation and MongoDB Client Connection Documentation.
Being aware of these common issues and their corresponding fixes will enhance your ability to manage MongoDB securely and effectively. Happy coding!