Troubleshooting Redis Connection Issues in Spring Applications
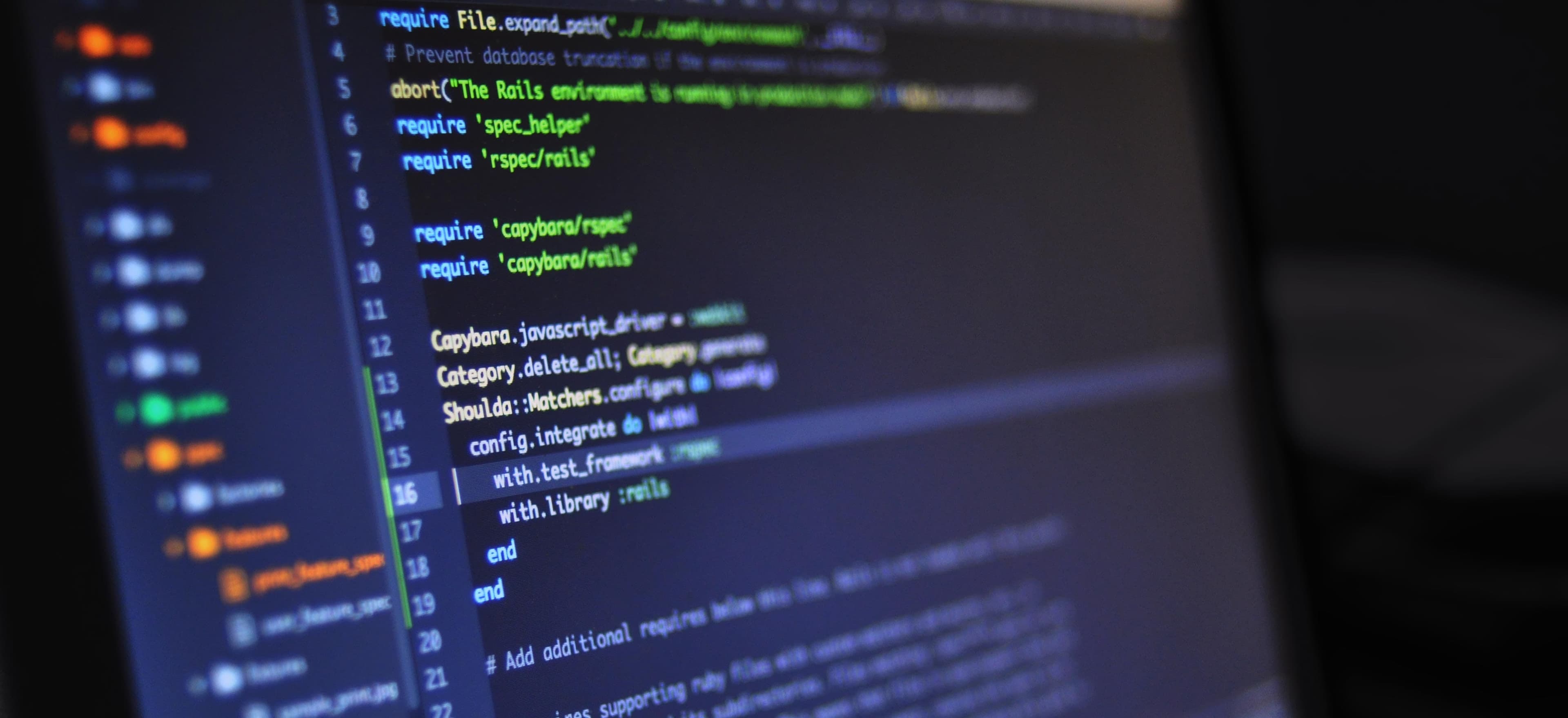
- Published on
Troubleshooting Redis Connection Issues in Spring Applications
Redis is a powerful in-memory data structure store that is widely used as a database, cache, and message broker. When integrating Redis with Spring applications, developers can enjoy high performance and scalability. However, issues can arise when setting up the connection, impacting your application's functionality. In this article, we will dive deep into troubleshooting common Redis connection issues in Spring applications, exploring their causes, potential solutions, and code examples.
Understanding Redis Connection Basics
Before we dive into troubleshooting, it’s crucial to understand the components of a Redis connection in a Spring application:
- RedisClient: This is your connection to the Redis server.
- RedisTemplate: This Spring abstraction simplifies interactions with Redis through various data structures.
- Configuration: Your Spring configuration setup (Java or XML) where you specify your Redis connection properties.
Common Redis Connection Issues
Here are some of the most common issues you might encounter while connecting Redis to Spring applications:
- Connection Refused
- Timeout Exceptions
- Authentication Issues
- Network Configuration Problems
1. Connection Refused
The "Connection Refused" error usually indicates that your application is trying to communicate with Redis, but no server is listening on the specified port. This can occur for several reasons:
- Redis Server Not Running: Ensure that the Redis server is up and running on the expected host and port.
- Incorrect Host/Port Configuration: Verify that the host and port in your Spring application match the Redis server settings.
Solution
First, ensure that Redis is installed and running. You can start Redis using the following command:
redis-server
Next, check your Spring configuration. Here’s a sample configuration in Java using RedisConnectionFactory
and RedisTemplate
:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.data.redis.connection.RedisConnectionFactory;
import org.springframework.data.redis.connection.RedisStandaloneConfiguration;
import org.springframework.data.redis.core.RedisTemplate;
@Configuration
public class RedisConfig {
@Bean
public RedisConnectionFactory redisConnectionFactory() {
// Replace "localhost" and "6379" with your Redis host and port
RedisStandaloneConfiguration config = new RedisStandaloneConfiguration("localhost", 6379);
return new LettuceConnectionFactory(config);
}
@Bean
public RedisTemplate<String, Object> redisTemplate() {
RedisTemplate<String, Object> template = new RedisTemplate<>();
template.setConnectionFactory(redisConnectionFactory());
return template;
}
}
In the configuration above, ensure that the hostname and port match your Redis server settings (default is localhost and 6379).
2. Timeout Exceptions
Timeout exceptions can occur if the connection to the Redis server takes too long or if the server is overwhelmed with requests.
Causes
- High Load: The Redis server could be under heavy load or running out of memory.
- Network Latency: If your application is running on a different machine or cloud service, network issues might cause delays.
Solution
You can increase the timeout settings in your Spring configuration. Here’s how:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.data.redis.connection.RedisConnectionFactory;
import org.springframework.data.redis.connection.RedisStandaloneConfiguration;
import org.springframework.data.redis.connection.lettuce.LettuceConnectionFactory;
import org.springframework.data.redis.core.RedisTemplate;
@Configuration
public class RedisConfig {
@Bean
public RedisConnectionFactory redisConnectionFactory() {
RedisStandaloneConfiguration config = new RedisStandaloneConfiguration("localhost", 6379);
LettuceConnectionFactory factory = new LettuceConnectionFactory(config);
// Set timeout (for example, 2000 milliseconds)
factory.setTimeout(Duration.ofMillis(2000));
return factory;
}
@Bean
public RedisTemplate<String, Object> redisTemplate() {
RedisTemplate<String, Object> template = new RedisTemplate<>();
template.setConnectionFactory(redisConnectionFactory());
return template;
}
}
Here, the timeout is set to 2000 milliseconds (2 seconds), which should help alleviate issues caused by latency.
3. Authentication Issues
If your Redis instance requires authentication and you're not supplying the correct password, you'll encounter authentication errors.
Solution
You can configure Redis authentication in your Spring application as follows:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.data.redis.connection.RedisConnectionFactory;
import org.springframework.data.redis.connection.RedisStandaloneConfiguration;
import org.springframework.data.redis.connection.lettuce.LettuceConnectionFactory;
@Configuration
public class RedisConfig {
@Bean
public RedisConnectionFactory redisConnectionFactory() {
RedisStandaloneConfiguration config = new RedisStandaloneConfiguration("localhost", 6379);
// Set the password if required
config.setPassword(RedisPassword.of("your-redis-password"));
return new LettuceConnectionFactory(config);
}
// ... (rest of the configuration)
}
4. Network Configuration Problems
Network issues can arise, especially if your Redis server is hosted in the cloud or in a separate container or VM.
Causes
- Firewalls blocking traffic.
- Incorrect network configuration in cloud services.
Solution
To resolve network-related issues:
- Check Firewall Settings: Ensure that the port Redis is running on (default 6379) is open.
- Network Security Groups: If you're using cloud providers, check your network security groups.
- Correct IP Binding: Ensure Redis is configured to bind to the correct IP address or set to bind to all interfaces (0.0.0.0).
Check the /etc/redis/redis.conf
file for the binding configuration:
bind 0.0.0.0
This setup allows Redis to accept connections from any IP address. Be cautious with this setting in production environments.
Best Practices for Redis Integration in Spring Applications
To enhance your Redis integration and avoid common pitfalls, consider following these best practices:
-
Use Connection Pooling: Utilize connection pooling to manage your connections efficiently.
import org.springframework.data.redis.connection.lettuce.LettucePoolingClientConfiguration; @Bean public RedisConnectionFactory redisConnectionFactory() { RedisStandaloneConfiguration config = new RedisStandaloneConfiguration("localhost", 6379); LettucePoolingClientConfiguration clientConfig = LettucePoolingClientConfiguration.builder().build(); return new LettuceConnectionFactory(config, clientConfig); }
-
Monitor Redis Performance: Use tools like
redis-cli
or Redis monitoring dashboards for insights. -
Handle Connection Failures Gracefully: Implement retry logic in your Spring services for handling transient connectivity issues.
-
Keep Redis Up-to-Date: Make sure to keep your Redis server updated to the latest stable version for security and performance improvements.
Closing Remarks
Troubleshooting Redis connection issues in Spring applications may seem daunting at first, but by understanding the common problems and their solutions, you can resolve these issues effectively. Proper configuration and monitoring can save you from headaches down the road, allowing you to focus on building great applications.
For additional resources, consider checking out the Spring Data Redis documentation and the Redis documentation. Happy coding!